Express Returns Servers HTML Error Page Instead of res.json Error Message
Last Updated :
06 Jun, 2023
ExpressJS is a backend web application framework built on top of NodeJS which helps us manage the server and routing. It has some powerful functionalities like middleware, templates, and debugging which help us to write efficient and faster code. It helps us extensively in building web applications.
In this article, we will see why “Express returns servers’ automatic HTML error page, instead of a res.json error message” and how we can solve it.
When we don’t define a route for a particular path and the user tries to access it or something wrong happens on our webpage (like Could not access the database, server issue), Express returns a server automatic HTML error page.
A point to note that different developers would want their errors handled in their own way – some might want to create their own page for errors, some could give an error message to the user, and others might want to alert the user or redirect them to another page, or all of the above.
In this article, we will see how we can give a JSON error message instead of an HTML error page.
Example: Express generates a server automatic HTML page.
Javascript
const express = require( 'express' );
const app = express();
app.set( 'view engine' , 'ejs' );
app.get( '/' , (req, res) => {
res.render( 'home' )
})
app.get( '/about' , (req, res) => {
res.render( 'about' )
})
app.listen(3000);
|
In the above code, we have defined two routes (/ route) for the home page and (/about route) for the about page. But we haven’t defined a route /signin for the signin link in the navigation bar.
So our page functions like this:
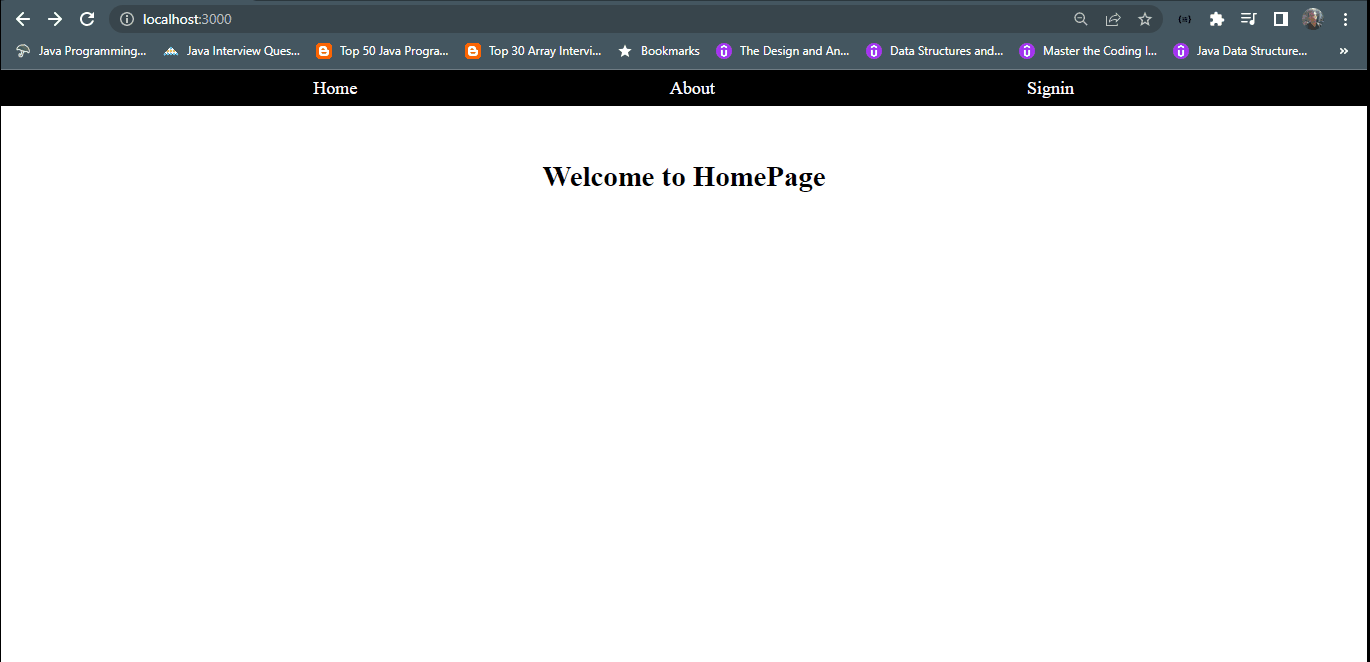
Web page which generates HTML Error Page
Output:
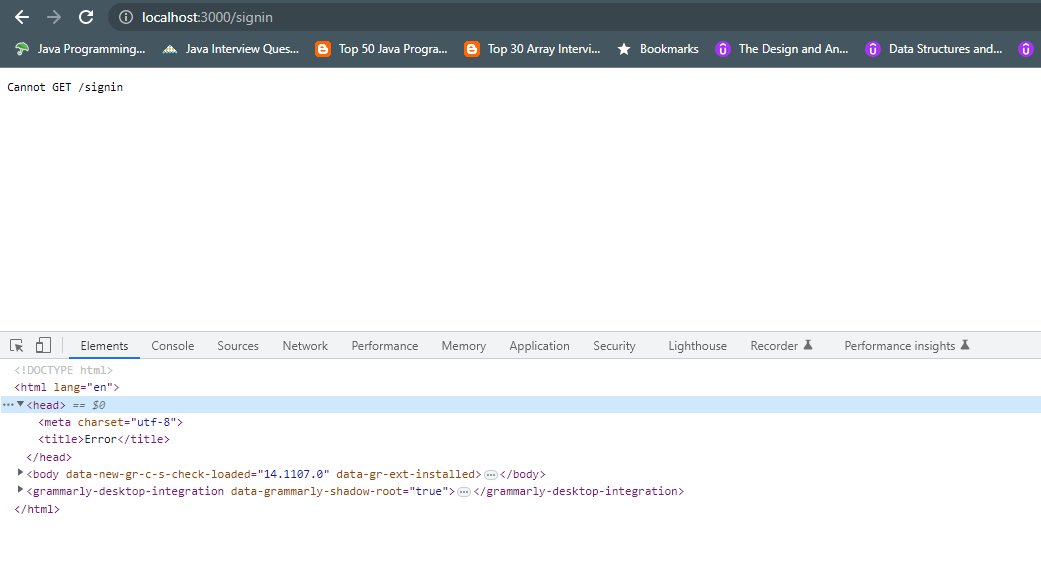
Express returning HTML-based error page
So Here we can see as we have not defined any route for /signin, So when the user tries to send a request to /signin route, Express returns a server automatic HTML page. So we somehow need to stop it and give our own JSON error message.
Solution: The solution is pretty simple. We can fix it by adding a Middleware Function which is there in Express which can give us the desired result.
What are Middleware Functions?
It gets triggered when the server receives the request and before the response is sent to the client. So there are different purposes for using middleware functions like making API calls, getting some data from the database, Error Handling, etc. Here we need middleware to handle our error which will come when we will send a request to /signin route.
So what we need to do is just we have to update our app.js by adding a middleware function that gets triggered when no route matches. It gives a JSON error message(i.e. page not found) instead of an HTML Page.
Javascript
const express = require( 'express' );
const app = express();
app.set( 'view engine' , 'ejs' );
app.get( '/' , function (req, res) {
res.render( 'home' );
});
app.get( '/about' , function (req, res) {
res.render( 'about' );
});
app.use( function (req, res) {
res.status(401).json({ error: "Page not Found" });
})
app.listen(3000);
|
Output:
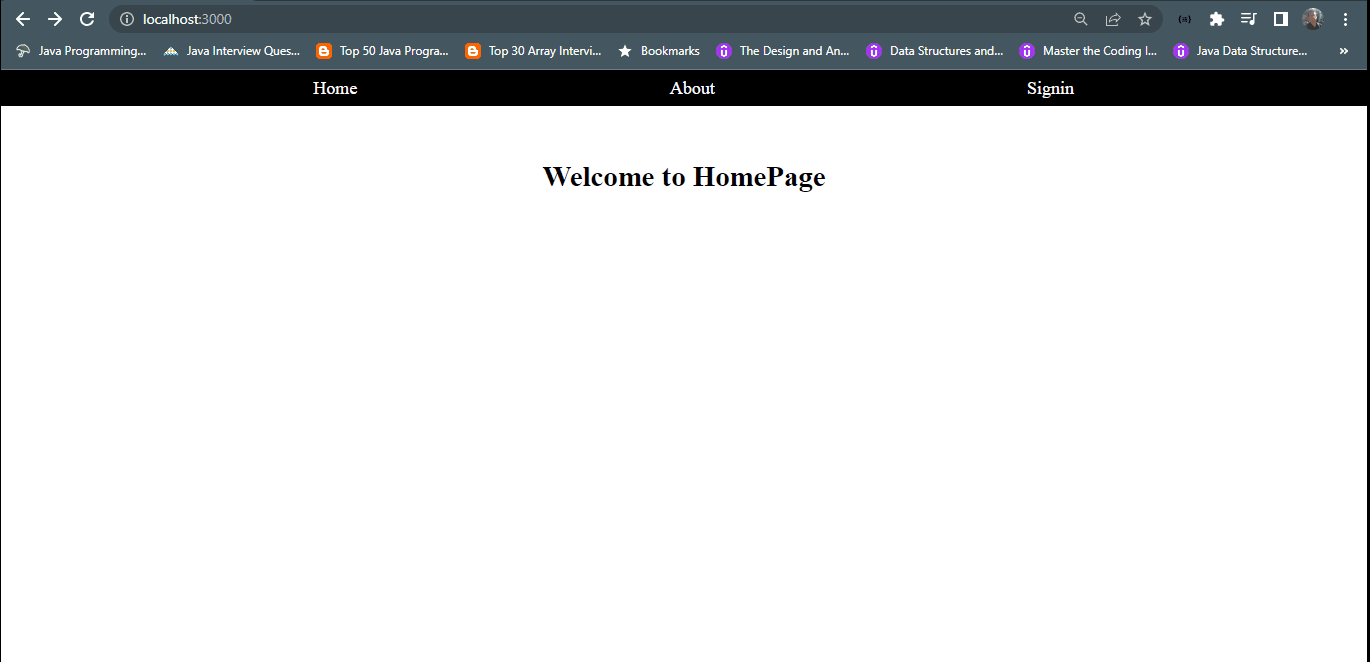
JSON Error Message
In this way, we are able to return the user a JSON error message instead of a server-generated HTML Page. This comes in handy when we want to deal with the error and want to send a JSON Format to the user.
Share your thoughts in the comments
Please Login to comment...