How to Exit after res.send() in Express JS
Last Updated :
16 Dec, 2023
In this article, we are going to learn how we can exit after the res.send() function in Express JS. In Express the res.send() method is mainly used to send a response to the client with the specified content. This function automatically sets the Content-Type Header which is based on the data provided.
We are going to implement the Exit after res.send() using below given two approaches.
Approach 1: Using next():
We are going to use the next() function after calling the res.send(), this will allow the request to proceed to the next middleware or the route handler and indirectly exit the current function but further processing will be done in subsequent middleware or routes.
Syntax:
app.get('/example', (req, res, next) => {
// Tasks
// Sending response to the client
res.send('Response sent to the client.');
// Using next() to exit the handler and pass control to the next middleware or route handler
next();
});
Example: In the below example the application responds with “Hello GeeksforGeeks” when the request is made to the root route. After sending the response using res.send(), the middleware is executed and exits the application using next().
Javascript
const express = require( 'express' );
const app = express();
app.get( '/' , (req, res, next) => {
res.send( 'Hello, GeeksforGeeks!' );
next();
});
app.use((req, res, next) => {
console.log( 'After res.send(), exiting using next()' );
});
app.listen(3000, () => {
console.log( 'Server is running on port 3000' );
});
|
Output:
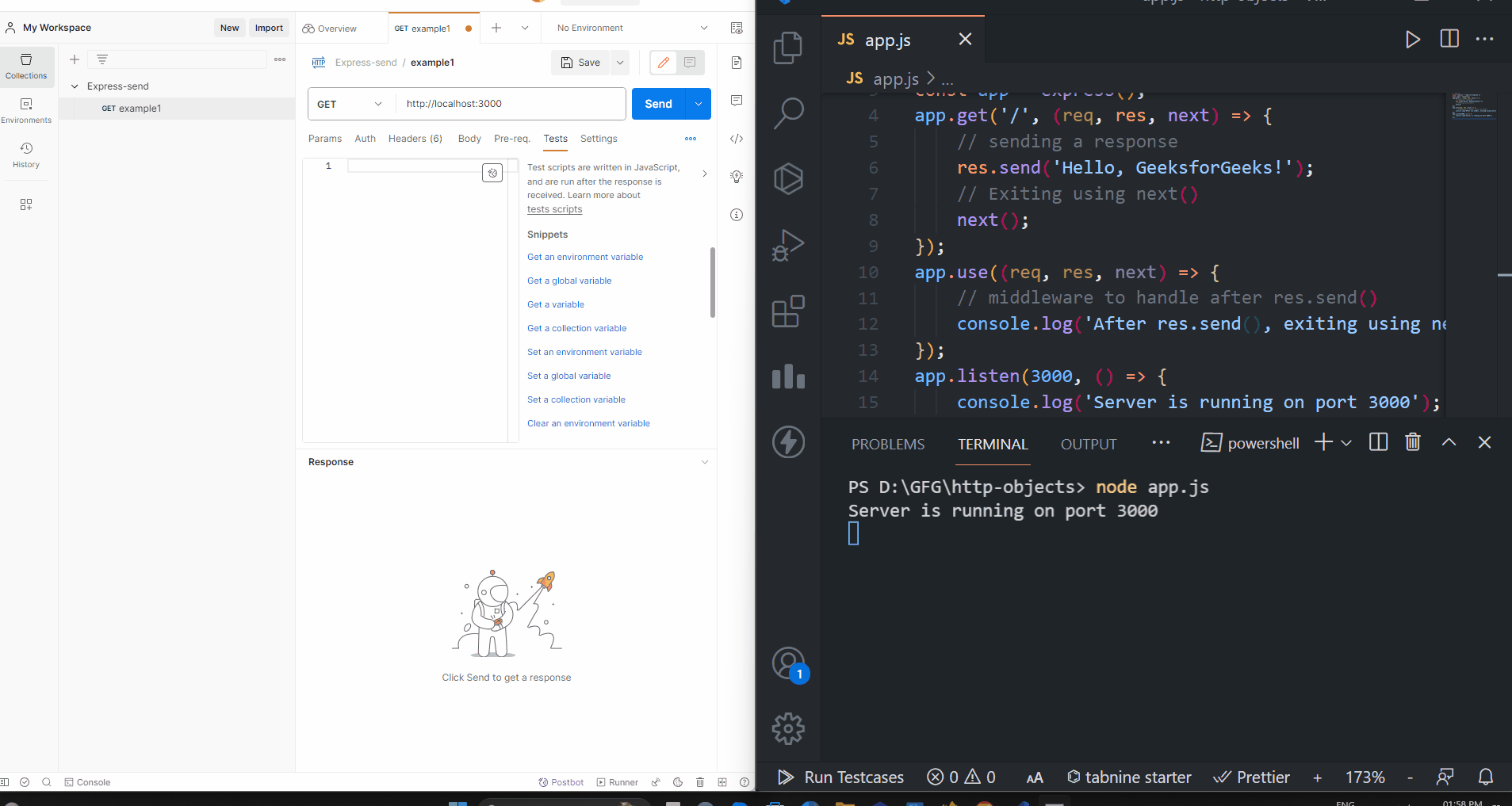
Approach 2: Using res.end():
We are going to use res.end() function to exit after res.send() response is been done. This function is mainly used to end or terminate the repose process. This will terminate the process and will not pass the control to the next middleware or route. The request is been terminated.
Syntax:
// Inside a route handler
app.get('/', (req, res) => {
// Sending a response
res.send('Hello, World!');
// Exiting the application using res.end()
res.end();
});
Example: In the below example, we are sending the response with the res.send() function in the root route (‘/’). The middleware that is used to handle after res.send() is not executed because the res.end() stops the response process immediately.
Javascript
const express = require( 'express' );
const app = express();
app.get( '/' , (req, res) => {
res.send( 'Hello, Geeks!' );
res.end();
});
app.use((req, res, next) => {
console.log( 'After res.send(), exiting using res.end()' );
});
app.listen(3000, () => {
console.log( 'Server is running on port 3000' );
});
|
Output:
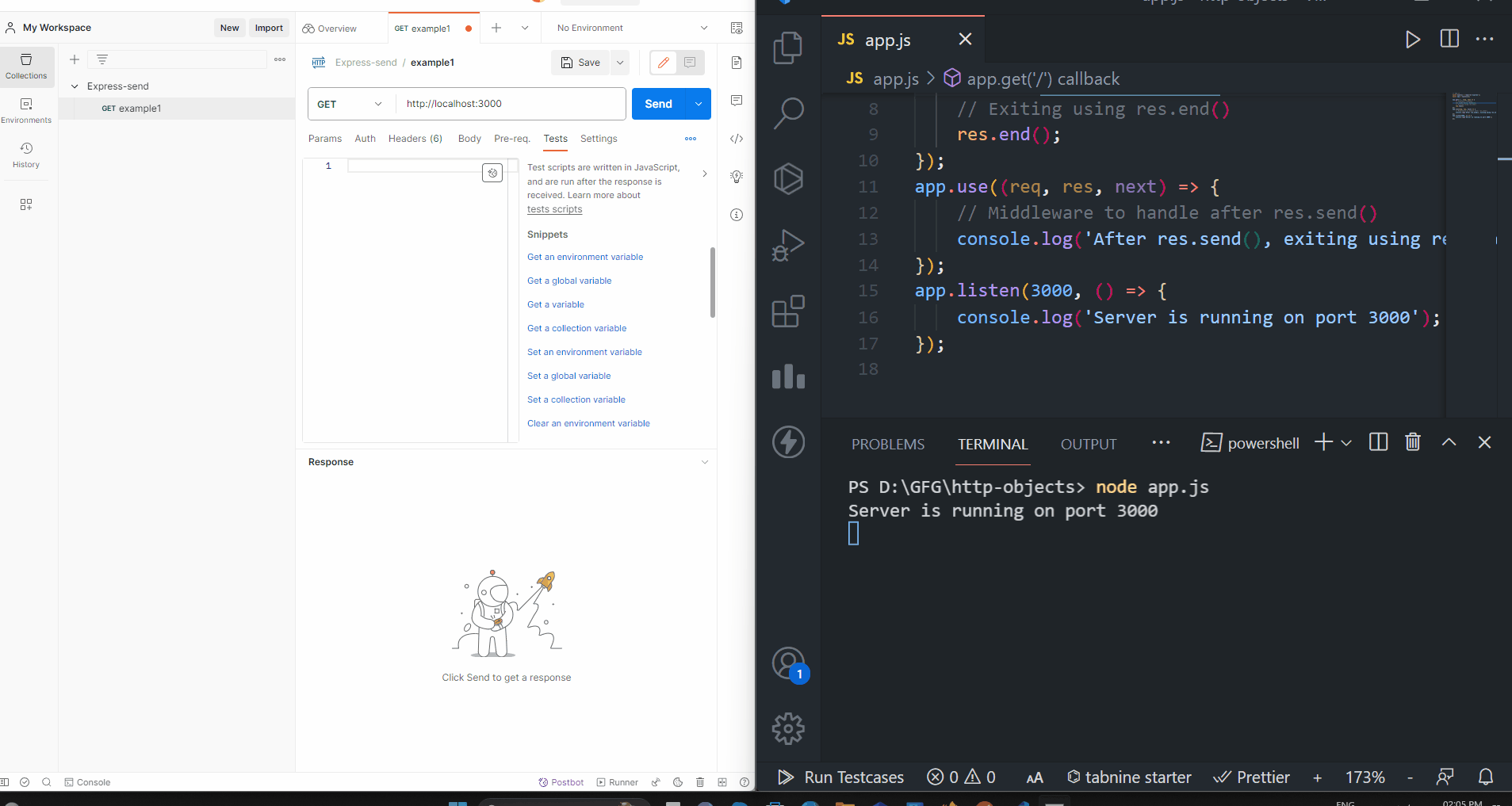
Share your thoughts in the comments
Please Login to comment...