Difference between Node.js and React.js
Last Updated :
06 Apr, 2023
Node.js: Node.js is an open-source and cross-platform runtime environment for executing JavaScript code outside a browser. You need to remember that NodeJS is not a framework and it’s not a programming language. Most people are confused and understand it’s a framework or a programming language. We often use Node.js for building back-end services like APIs like Web App or Mobile App.
Features of Node.js: There are other programming languages also that we can use to build back-end services so what makes Node.js different I am going to explain.
- It’s easy to get started and can be used for prototyping and agile development
- It provides fast and highly scalable services
- It uses JavaScript everywhere so it’s easy for a JavaScript programmer to build back-end services using Node.js
- The source code is cleaner and consistent.
- Large ecosystem for open source library.
- It has an Asynchronous or Non-blocking nature.
Code: Here is an example of how to include the HTTP module to build the server.
Filename: App.js
Javascript
const http = require( 'http' );
http.createServer( function (req, res) {
res.write( 'GeeksforGeeks' );
res.end();
}).listen(8080);
|
Output: For compiling the nodejs file go to the terminal and follow the command:
node foldername.js
Now, open the localhost:8080 in your browser to see the output
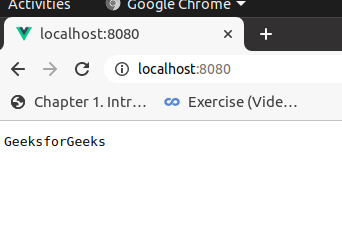
ReactJS: It is an open-source JavaScript library for building single-page user interfaces. It is declarative, efficient, and flexible, and allows us to create reusable UI components, it is used as a base in single-page, complex, interactive web projects, and react components are difficult to reuse. The virtual DOM algorithm of React is a time-consuming and imprecise writing code. A React application is made of multiple components, each responsible for rendering a small, reusable piece of HTML. Components can be nested within other components to allow complex applications to be built out of simple building blocks.
Features of React.js: Here are some features that make React.js different from other programming languages:
- React components have reusable codes that make it simple to use and learn.
- React library has JSX (JavaScript XML), which is HTML-like syntax, which is processed into JavaScript calls.
- React components are reusable which helps while working on larger scale projects and have its own logic and controls.
- One-way data binding provides better control throughout the application.
- The virtual DOM uses the ReactDOM library which ideally/virtually, represents UI and keeps in the memory and syncs with the real DOM.
- DOM has a smoother and faster performance due to virtual components.
Create a react app project and edit the App.js file in the src folder as:
FilePath:- src/App.js:
Javascript
import React, { Component } from 'react' ;
class App extends Component {
render() {
return (
<div className= "App" >
<>
<h1>Hello from GeeksforGeeks!!</h1>
</>
</div>
);
}
}
export default App;
|
Output:
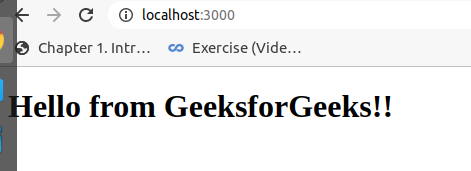
Difference between Node.js and React.js:
Node.js |
React.js |
Node.js used as a back-end framework |
React is used for developing user interfaces. |
It supports the Model–view–controller (MVC) framework. |
Does not support the Model–view–controller (MVC) framework. |
It runs on chrome’s v8 engine and uses an event-driven, non-blocking I/O model, which is written in C++. |
It uses Node.js to compile and optimize the JavaScript code and easy to create UI Test cases. |
Node.js handles requests and authentication from the browser, make database calls, etc. |
It makes API calls and processes in-browser data. |
Here the Real-time data streaming is handled easily. |
In React complex architecture makes it hard to keep track of the traditional approach. |
Framework for JavaScript execution having the largest ecosystem of open source libraries. |
Facebook-backed Open Source JS library. |
The language used is only JavaScript. |
The language used is JSX and JavaScript. |
There is no DOM (Document Object Model) concept that is Used. |
Here the Virtual DOM (Document Object Model) is Used that makes it faster. |
It is easy to write microservices in Node.Js |
Microservices are difficult to be written in React.Js |
It is highly scalable. |
Scalability is still a challenge. |
It has a simple architecture. |
It has a complex architecture. |
Share your thoughts in the comments
Please Login to comment...