Detect Encoding of a Text file with Python
Last Updated :
11 Mar, 2024
Python provides a straightforward way to determine the encoding of a text file, essential for the proper handling of diverse character sets. The chardet
library is a popular choice for automatic character encoding detection. By analyzing the statistical distribution of byte values, it accurately identifies the encoding scheme used in a given text file. In this guide, we’ll explore a simple yet effective approach to detect and work with text file encodings using Python and the chardet
library.
How to detect the encoding of a text file with Python?
Below, are the step-by-step implementation of How to detect the encoding of a text file with Python.
Step 1: Create a Virtual Environment
First, create the virtual environment using the below commands
python -m venv env
.\env\Scripts\activate.ps1
Step 3:Install the library chardet
First, you need to install the chardet library. Open your terminal or command prompt and run the following command:
pip install chardet

Step 3: Implement the Logic
Below, Python code defines a function, `detect_encoding(file_path)`, that uses the `chardet` library to automatically determine the encoding of a text file specified by its path. It reads the file in binary mode, feeds each line to a universal detector from `chardet`, and stops when the detector is done or the file ends. The function then returns the detected encoding extracted from the detector’s result, facilitating proper handling of diverse character sets during file processing..
Python
import chardet
def detect_encoding(file_path):
with open (file_path, 'rb' ) as file :
detector = chardet.universaldetector.UniversalDetector()
for line in file :
detector.feed(line)
if detector.done:
break
detector.close()
return detector.result[ 'encoding' ]
|
Step 4: Add the File Path
Finally, let us use our function to identify the coding of a sample text file. Change the file path in the code below to match where your text file is stored.
Python
file_path = 'path/to/your/textfile.txt'
encoding = detect_encoding(file_path)
print (f 'The encoding of the file is: {encoding}' )
|
Step 6: Run the server
Save the whole script in a Python file (such as detect_encoding.py) and run it with your preferred Python interpreter, make sure to replace detect_encoding.py by the name of your actual script.
python detect_encoding.py
Output :
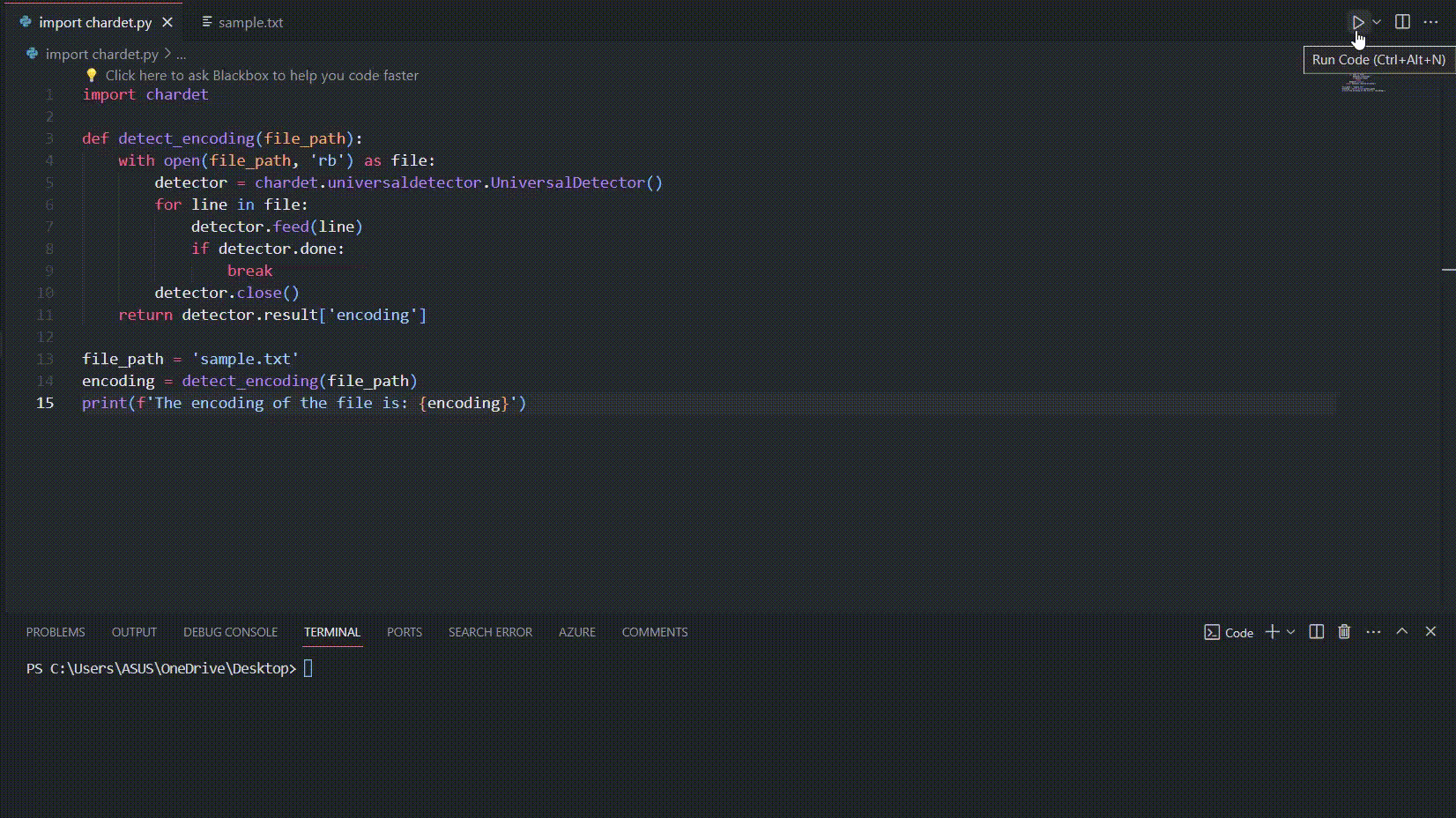
Conclusion
In this article, we discussed how the chardet library could be used for automatic text file encoding detection in the Python. In this way, following the steps provided above, you will be able to incorporate the encoding detection into your Python scripts and enhance their efficiency in processing text files encoded differently.
Share your thoughts in the comments
Please Login to comment...