Design a Decimal to Binary Converter in VueJS
Last Updated :
24 Apr, 2024
The Decimal to Binary Converter is a simple web application built using Vue.js that allows users to convert decimal numbers to binary format. It provides a user-friendly interface for the easy conversion of decimal numbers into a binary representation.
Prerequisites
Approach
- Create a new HTML file named index.html. Include the necessary CDN links for the Vue.js and Tailwind CSS.
- Design the layout using HTML elements such as the <div>, <input>, <button>, and <p>. Use appropriate classes from the Tailwind CSS for styling.
- Apply Tailwind CSS classes to style the elements for a modern and responsive design.
- Use utility classes for the spacing, borders, and colors as needed. Initialize a new Vue instance with the el option set to #app.
- Define data properties for the storing the decimal number input and binary conversion result.
- Implement a method to convert the decimal number to the binary upon button click. Use the parseInt() and toString(2) functions to the perform the conversion.
- Add validation to the ensure that a valid decimal number is entered before conversion. Display an alert message if the input is invalid.
- Show the binary equivalent of the entered decimal number below the input field after conversion.
CDN link:
https://cdn.jsdelivr.net/npm/vue@2.6.14/dist/vue.js
Example: This example shows the implementation of the above-explained approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>
The Decimal to Binary Converter
</title>
<script src=
"https://cdn.jsdelivr.net/npm/vue@2.6.14/dist/vue.js">
</script>
<link rel="stylesheet" href=
"https://cdn.jsdelivr.net/npm/tailwindcss@2.2.19/dist/tailwind.min.css">
</head>
<body class="bg-gray-200 py-10">
<div id="app" class="max-w-md mx-auto
bg-white p-8 rounded shadow-md">
<h1 class="text-2xl font-semibold mb-4">
Decimal to Binary Converter
</h1>
<div class="mb-4">
<label for="decimalInput" class="block mb-1">
Enter Decimal Number:
</label>
<input type="number" id="decimalInput"
v-model.number="decimalNumber"
class="w-full px-4 py-2 border rounded
focus:outline-none focus:border-blue-500"
placeholder="Enter decimal number">
</div>
<button @click="convertToBinary"
class="bg-blue-500 text-white px-4 py-2
rounded hover:bg-blue-600">
Convert
</button>
<div v-if="binaryNumber" class="mt-4">
<p>
<strong>
Binary Equivalent:
</strong>
{{ binaryNumber }}
</p>
</div>
</div>
<script>
new Vue({
el: '#app',
data: {
decimalNumber: null,
binaryNumber: null
},
methods: {
convertToBinary() {
if (!this.decimalNumber) {
alert('Please enter a decimal number');
return;
}
const decimal = parseInt(this.decimalNumber);
if (isNaN(decimal)) {
alert(`Invalid input. Please enter a
valid decimal number`);
return;
}
this.binaryNumber = decimal.toString(2);
}
}
});
</script>
</body>
</html>
Output:
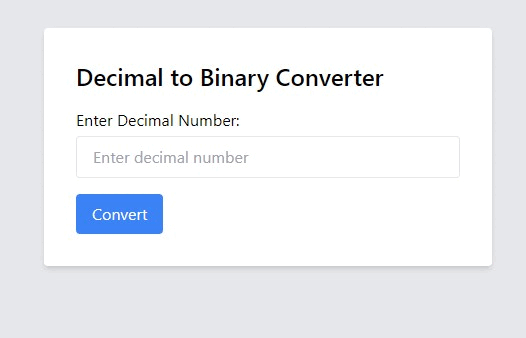
Share your thoughts in the comments
Please Login to comment...