Dereference Pointer in C
Last Updated :
31 Oct, 2023
We know that the pointer variable can store the memory address in C language and in this article, we will learn how to use that pointer to access the data stored in the memory location pointed by the pointer.
What is a Pointer?
First of all, we revise what is a pointer. A pointer is a variable that stores the memory address of another variable. The pointer helps us to manipulate the data in the memory address that the pointer points. Moreover, multiple pointers can point to the same memory.
Consider the following example,
int num = 10;
int *ptr;
ptr = #
We have stored the address of the num variable in the ptr pointer, but now, how to access the value stored in the memory at the address pointed by ptr? Here, dereferencing comes into play.
Dereference Pointer in C
Accessing or manipulating the content that is stored in the memory address pointed by the pointer using dereferencing or indirection operator (*) is called dereferencing the pointer.
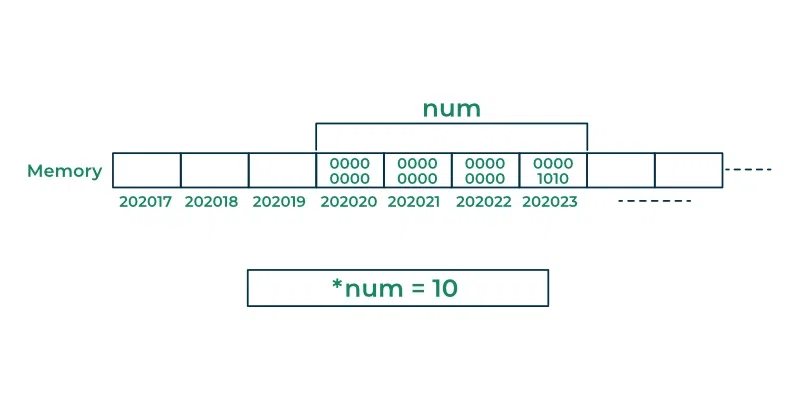
Dereferencing Pointer in C
Syntax for Dereferencing a Pointer
We use the indirection operator (*) as the prefix to dereference a pointer:
*(pointer_name)
For modifying the data stored in the memory, we use
*(pointer_name) = new_value;
It is to be noted that the new_value must be of the same type as the previous.
Consider the above examples where ptr points to num, the content in the memory address can be accessed by the dereferencing operator *. Now, the *ptr will fetch the content stored in the address which is 10.
The num and ptr memory address and values will look like this.
Variable |
Memory Address |
Value |
num = 10 |
202020 |
10 |
202021 |
202022 |
202023 |
ptr = &num |
202024 – 202032 |
202020 |
Note: We have assumed that the architecture in the above example is byte addressable i.e. minimum unit that has a separate address is a byte.
Examples of Pointer Dereferencing
Example 1:
Using a pointer to access and modify the value of an integer variable.
C
#include <stdio.h>
int main()
{
int num = 10;
int * ptr = #
printf ( "Value of num = %d \n" , num);
printf ( "Address of num = %d \n" , &num);
printf ( "Address stored in the ptr = %p \n\n" , ptr);
printf ( "Dereference content in ptr using *ptr\n\n" );
printf ( "Value of *ptr = %d \n" , *ptr);
printf ( "Now, *ptr is same as number\n\n" );
printf ( "Modify the value using pointer to 6 \n\n" );
*ptr = 6;
printf ( "Value of *ptr = %d \n" , *ptr);
printf ( "Value of number = %d \n" , num);
return 0;
}
|
Output
Value of num = 10
Address of num = 0x7ffe47d51b4c
Address stored in the ptr = 0x7ffe47d51b4c
Dereference content in ptr using *ptr
Value of *ptr = 10
Now, *ptr is same as number
Modify the value using pointer to 6
Value of *ptr = 6
Value of number = 6
Example 2: Dereferencing Double Pointer
The double pointers can also be dereferenced using the same logic but you will have to use the indirection operator two times: One for moving to the pointer the double pointer is pointing to and the other for accessing the actual value.
C
#include <stdio.h>
int main()
{
int var = 10;
int * ptr = &var;
int ** dptr = &ptr;
printf ( "Accesing value from double pointer using "
"**dptr: %d" ,
**dptr);
return 0;
}
|
Output
Accesing value from double pointer using **dptr: 10
Just like that, we can dereference pointers of any level.
How dereferencing work?
Whenever we ask the compiler to dereference a pointer, it does three things:
- It first looks up the address stored in the pointer.
- Then it looks for the type of pointer so that it can deduce the amount of memory to read. For example, 4 byes for int, 1 byte for char, etc. It is also the main reason why we need to specify the pointer type in the declaration even though the size of every pointer in a system is the same.
- Finally, it reads the memory and returns the data stored.
Note: From the above reasoning, we can also infer that we cannot dereference the void pointer as the size of the data it is pointing to is unknown. So we need to typecast the void pointer to dereference it.
Share your thoughts in the comments
Please Login to comment...