GraphQL is an open-source technology that allows us to query only the data that we require, unlike the traditional REST architecture which returns us the entire resources and data with specific endpoints configured for the same. Using GraphQL, we specify using the query the data that we want, and it returns the specified data in the specified format. It helps reduce the amount of data transfer over the network and saves network bandwidth.
This article will teach us how to fetch data using GraphQL, with React as our front-end UI library.
Fetching GraphQL Data with React as a UI Library
Step 1: Create an open-source application
npx create-react-app graphql
Step 2: Move into the project folder
cd graphql
Step 3: The project Structure will look like
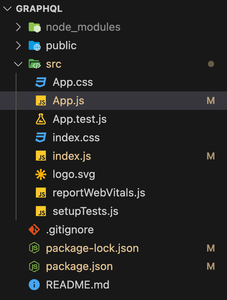
Step 4: Let’s modify App.js component to include ApolloClient and ApolloProvider
In the App.js component, we will use the ApolloProvider to utilise the GraphQL queries in our react app. We will wrap the entire application inside an ApolloProvider component, and give it an ApolloClient with a graphQL API pointing to a Pokemon API, which we will eventually use to fetch the list of the pokemons and display the same on the web page.
We will create a new ApolloClient like below
Javascript
const client = new ApolloClient({
cache: new InMemoryCache(),
});
|
We will wrap the entire App.js in the ApolloProvider like below –
Wrapping the entire App component inside the ApolloProvider ensures all the child components, or rather, the entire App components, to use the GraphQL API seamlessly. Because of this, we will be able to call the GraphQL API endpoint inside the App component.
Javascript
function ApolloApp() {
return (
<ApolloProvider client={client}>
<App />
</ApolloProvider>
);
}
|
We will use the below GraphQL query to fetch the list of all the pokemons, with a total limit of upto 10. In the below query, we specify that we want to get the list of pokemons, and that the individual pokemon data in the list should only contain the id, name, and image, and the list as a whole should only return the total count, next, previous, status, and message.
const GET_POKEMONS = gql`
query getPokemons {
pokemons(limit: 10, offset: 0) {
count
next
previous
status
message
results {
id
name
image
}
}
}
`;
Now, we will use the above query to fetch the list and display the same in the App component –
In the below code, we use the useQuery hook, provided by the ApolloClient, to get the loading state, error, and data values from the GET_POKEMONS GraphQL query. We will then loop over the pokemon data, present in the data object, and display the pokemon’s names in the form of a list in the UI.
Javascript
function App() {
const { loading, error, data } = useQuery(GET_POKEMONS);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return (
<div>
<h1>Pokemons</h1>
<ul>
{data?.pokemons?.results?.map(pokemon => (
<li key={pokemon.id}>
{pokemon.name}
</li>
))}
</ul>
</div>
);
}
|
Our final App.js component will look like below
Javascript
import React from 'react' ;
import { ApolloProvider, useQuery, gql } from '@apollo/client' ;
import { ApolloClient, InMemoryCache } from '@apollo/client' ;
const client = new ApolloClient({
cache: new InMemoryCache(),
});
const GET_POKEMONS = gql`
query getPokemons {
pokemons(limit: 10, offset: 0) {
count
next
previous
status
message
results {
id
name
image
}
}
}
`;
function App() {
const { loading, error, data } = useQuery(GET_POKEMONS);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return (
<div>
<h1>Pokemons</h1>
<ul>
{data?.pokemons?.results?.map(pokemon => (
<li key={pokemon.id}>
{pokemon.name}
</li>
))}
</ul>
</div>
);
}
function ApolloApp() {
return (
<ApolloProvider client={client}>
<App />
</ApolloProvider>
);
}
export default ApolloApp;
|
Output:
The output for the above code will look like below
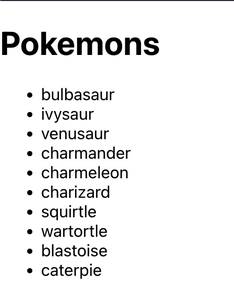
Fetching GraphQL Data Using Fetch API
Let’s look at how we can fetch the GraphQL data using the fetch API.
Step 1: Creating GraphQL Query
In this step, we will create the GraphQL query to fetch only the specified pokemon data from the server, and we will keep the limit as 10, and offset as 0.
Javascript
const graphqlQuery = `
query getPokemons {
pokemons(limit: 10, offset: 0) {
count
next
previous
status
message
results {
id
name
image
}
}
}
`;
|
Step 2: Defining fetch options, changing the HTTP verb to POST, and passing the GraphQL query in the request body.
In this step, we will create the fetchOptions object and assign the method to be POST, headers as Content=Type of application/json, and in the body we will pass the graphQL Query
Javascript
const fetchOptions = {
method: 'POST' ,
headers: {
'Content-Type' : 'application/json' ,
},
body: JSON.stringify({ query: graphqlQuery }),
};
|
Step 3: Hitting the Query using the Fetch API
In this step, we will hit the query endpoint using the fetch API and log the data into the console. The whole code should look like below
Javascript
const graphqlQuery = `
query getPokemons {
pokemons(limit: 10, offset: 0) {
count
next
previous
status
message
results {
id
name
image
}
}
}
`;
const fetchOptions = {
method: 'POST' ,
headers: {
'Content-Type' : 'application/json' ,
},
body: JSON.stringify({ query: graphqlQuery }),
};
fetch(graphqlEndpoint, fetchOptions)
.then(response => response.json())
.then(data => {
console.log( 'GraphQL Data:' , data);
})
. catch (error => {
console.error( 'Error:' , error);
});
|
Output:
The output for the above code will look like below
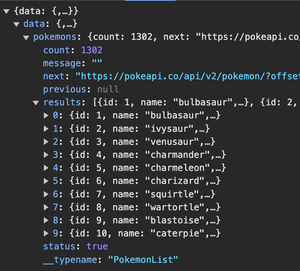
Fetching Data with GraphiQL
GraphiQL is an interactive platform that allows user to query the GraphQL API from the browser itself, without the need to download, or go to another platform. GraphiQL also provides other features like auto-completion, syntax highlighting, etc.
Let’s look at how we can fetch the GraphQL data using GraphiQL.
Step 1: We will create a basic NodeJS server to setup up our GraphQL API
In the below code, we will create a basic GraphQL API, with only one query, “hello”, and we will create a resolver that returns “Hello, GFG!”, when the query is called. We will use express to setup and create our server, and we will use the “graphqlHTTP” express middleware to create a graphQL endpoint for our server.
Filename: server.js
Javascript
const express = require( 'express' );
const { graphqlHTTP } = require( 'express-graphql' );
const { buildSchema } = require( 'graphql' );
const schema = buildSchema(`
type Query {
hello: String
}
`);
const root = {
hello: () => {
return 'Hello, GFG!' ;
},
};
const app = express();
app.use( '/graphql' , graphqlHTTP({
schema: schema,
rootValue: root,
graphiql: true ,
}));
const PORT = process.env.PORT || 4000;
app.listen(PORT, () => {
console.log(`Server is running on http:
});
|
Step 2: Start the server
To start the server run the below command in the terminal.
node server.js
Output:
Server running on http://localhost:4000/graphql
Step 4: Test the query in GraphiQL interface.
To test the query, execute the below query in the GraphiQL interface.
query {
hello
}
Output:
The output of the above query will look like below
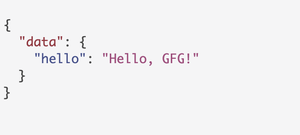
Conclusion
In this article, we learned about data fetching in GraphQL, and how we can use UI Libraries, fetch API, or the GraphiQL interface to query the data from the GraphQL server endpoint. GraphQL query helps us only retrieve the required data from the server, instead of bloating the network bandwidth with the unnecessary data on the client side.
Share your thoughts in the comments
Please Login to comment...