Creating GUI in Python – Labels, Buttons, and Message Box
Last Updated :
17 Apr, 2024
Tkinter offers various widgets that allow you to design your application’s interface, including labels for displaying text, buttons for user interaction, and message boxes for displaying information or receiving user input. In this article, we will see how we can create labels, buttons, and message boxes in Python using Tkinter.
Creating GUI in Python – Labels, Buttons, and Message Box
Below are the examples of labels, buttons, and message boxes that we are creating using Python:
Creating Labels Using Python Tkinter
In this example, a tkinter application window is created with a label widget displaying the text “This is a label”. The window is then launched and displayed with the label.
Python3
import tkinter as tk
# Create the main application window
root = tk.Tk()
root.title("Labels Example")
# Create a label
label = tk.Label(root, text="This is a label")
label.pack()
# Run the application
root.mainloop()
Output:
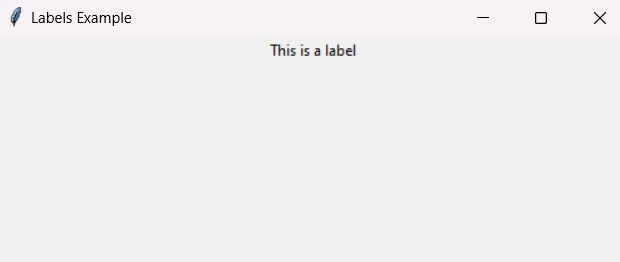
Creating Buttons Using Python Tkinter
In this example, a tkinter application window titled “Buttons Example” is created with a button widget labeled “Click Me”. When the button is clicked, it triggers the button_click function, which prints “Button clicked”. The application is then run, displaying the window with the button.
Python3
import tkinter as tk
def button_click():
print("Button clicked")
# Create the main application window
root = tk.Tk()
root.title("Buttons Example")
# Create a button
button = tk.Button(root, text="Click Me", command=button_click)
button.pack()
# Run the application
root.mainloop()
Output:
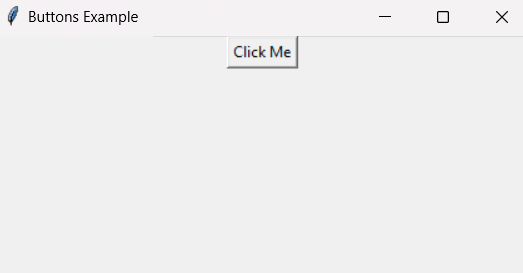
Creating Message Box Using Python Tkinter
In this example, a tkinter application window titled “Message Box Example” is created with a button widget labeled “Show Message”. When the button is clicked, it triggers the show_message function, which displays an information message box with the title “Message” and the text “Hello, this is a message!”.
Python3
import tkinter as tk
from tkinter import messagebox
def show_message():
messagebox.showinfo("Message", "Hello, this is a message!")
# Create the main application window
root = tk.Tk()
root.title("Message Box Example")
# Create a button to trigger the message box
button = tk.Button(root, text="Show Message", command=show_message)
button.pack()
# Run the application
root.mainloop()
Output:
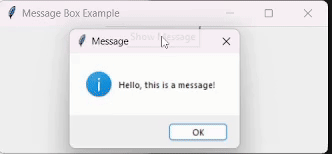
Share your thoughts in the comments
Please Login to comment...