Create Binary Tree with Nodes as Cumulative sum of children
Last Updated :
04 Jan, 2024
Given a root of a Binary Tree, the task is to update each of its nodes with the sum of all the nodes below it (from its left and right subtree), inclusive of the current Node.
Example:
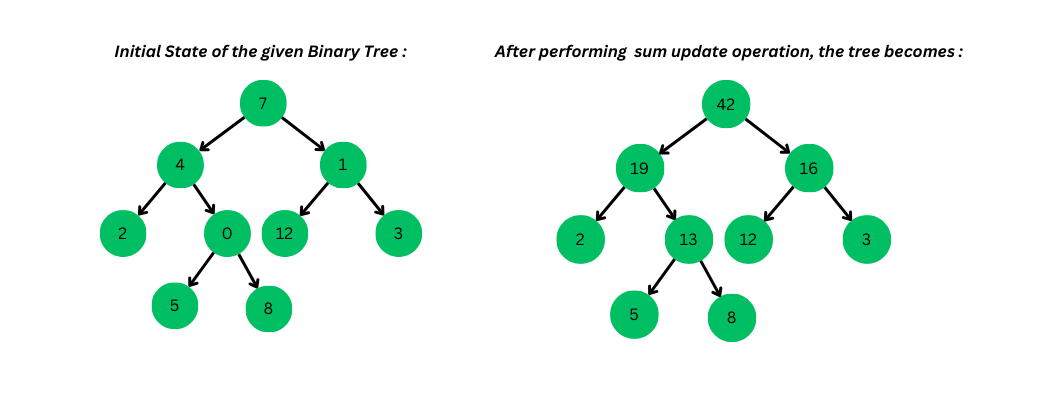
Example Test Case-I
Approach:
The idea is to simply traverse through left and right subtree, and calculate sum of Left Subtree & Right Subtree, and then update the current node with the sum returned from the left subtree and the right subtree + its own value.
Our base case would be the leaf node (The node which doesn’t have ANY child).
Here’s how to do this step-by-step:
- Traverse till leaf node (either left of right)
- Once you encounter a leaf node, the base case will be executed, and it will return the leaf node’s value
- Calculate sum of left subtree and right subtree, and add both of them (left Subtree + right Subtree’s values)
- The only catch we need to handle when our input tree is a binary tree(not necessarily a FULL Binary tree) is that, we need to check if the child exists before moving on to it.
- Update the current node’s value with the sum calculated in previous step.
Below is the implementation of the above approach:
C++
#include <iostream>
using namespace std;
class Node {
public :
Node* left;
int data;
Node* right;
Node( int data)
{
left = NULL;
this ->data = data;
right = NULL;
}
};
void inorder(Node* root)
{
if (root == NULL)
return ;
inorder(root->left);
cout << root->data << " ";
inorder(root->right);
}
int updateSum(Node*& root)
{
if (root->left == NULL && root->right == NULL)
return root->data;
int leftSum = 0;
if (root->left)
leftSum += updateSum(root->left);
int rightSum = 0;
if (root->right)
rightSum += updateSum(root->right);
root->data += leftSum + rightSum;
return root->data;
}
int main()
{
Node* root = new Node(1);
root->left = new Node(2);
root->left->left = new Node(4);
root->left->left->left = new Node(6);
root->left->right = new Node(5);
cout << "Inorder Before updating the Sum for every "
"Node :"
<< endl;
inorder(root);
updateSum(root);
cout << "\nInorder After updating the Sum for every "
"Node :"
<< endl;
inorder(root);
return 0;
}
|
Java
class Node {
public Node left;
public int data;
public Node right;
public Node( int data)
{
left = null ;
this .data = data;
right = null ;
}
}
public class BinaryTree {
static void inorder(Node root)
{
if (root == null )
return ;
inorder(root.left);
System.out.print(root.data + " " );
inorder(root.right);
}
static int updateSum(Node root)
{
if (root.left == null && root.right == null )
return root.data;
int leftSum = 0 ;
if (root.left != null )
leftSum += updateSum(root.left);
int rightSum = 0 ;
if (root.right != null )
rightSum += updateSum(root.right);
root.data += leftSum + rightSum;
return root.data;
}
public static void main(String[] args)
{
Node root = new Node( 1 );
root.left = new Node( 2 );
root.left.left = new Node( 4 );
root.left.left.left = new Node( 6 );
root.left.right = new Node( 5 );
System.out.println(
"Inorder Before updating the Sum for every Node:" );
inorder(root);
updateSum(root);
System.out.println(
"\nInorder After updating the Sum for every Node:" );
inorder(root);
}
}
|
Python3
class Node:
def __init__( self , data):
self .left = None
self .data = data
self .right = None
def inorder(root):
if root is None :
return
inorder(root.left)
print (root.data, end = " " )
inorder(root.right)
def update_sum(root):
if root.left is None and root.right is None :
return root.data
left_sum = 0
if root.left:
left_sum + = update_sum(root.left)
right_sum = 0
if root.right:
right_sum + = update_sum(root.right)
root.data + = left_sum + right_sum
return root.data
if __name__ = = "__main__" :
root = Node( 1 )
root.left = Node( 2 )
root.left.left = Node( 4 )
root.left.left.left = Node( 6 )
root.left.right = Node( 5 )
print ( "Inorder Before updating the Sum for every Node:" )
inorder(root)
update_sum(root)
print ( "\nInorder After updating the Sum for every Node:" )
inorder(root)
|
C#
using System;
public class Node
{
public Node left;
public int data;
public Node right;
public Node( int data)
{
left = null ;
this .data = data;
right = null ;
}
}
public class BinaryTree
{
public static void Inorder(Node root)
{
if (root == null )
return ;
Inorder(root.left);
Console.Write(root.data + " " );
Inorder(root.right);
}
public static int UpdateSum( ref Node root)
{
if (root.left == null && root.right == null )
return root.data;
int leftSum = 0;
if (root.left != null )
leftSum += UpdateSum( ref root.left);
int rightSum = 0;
if (root.right != null )
rightSum += UpdateSum( ref root.right);
root.data += leftSum + rightSum;
return root.data;
}
public static void Main()
{
Node root = new Node(1);
root.left = new Node(2);
root.left.left = new Node(4);
root.left.left.left = new Node(6);
root.left.right = new Node(5);
Console.WriteLine( "Inorder Before updating the Sum for every Node:" );
Inorder(root);
UpdateSum( ref root);
Console.WriteLine( "\nInorder After updating the Sum for every Node:" );
Inorder(root);
}
}
|
Javascript
class Node {
constructor(data) {
this .left = null ;
this .data = data;
this .right = null ;
}
}
function inorder(root) {
if (root === null ) return ;
inorder(root.left);
process.stdout.write(root.data + " " );
inorder(root.right);
}
function updateSum(root) {
if (root.left === null && root.right === null ) {
return root.data;
}
let leftSum = 0;
if (root.left) {
leftSum += updateSum(root.left);
}
let rightSum = 0;
if (root.right) {
rightSum += updateSum(root.right);
}
root.data += leftSum + rightSum;
return root.data;
}
const root = new Node(1);
root.left = new Node(2);
root.left.left = new Node(4);
root.left.left.left = new Node(6);
root.left.right = new Node(5);
console.log( "Inorder Before updating the Sum for every Node:" );
inorder(root);
updateSum(root);
console.log( "\nInorder After updating the Sum for every Node:" );
inorder(root);
|
Output
Inorder Before updating the Sum for every Node :
6 4 2 5 1
Inorder After updating the Sum for every Node :
6 10 17 5 18
Time Complexity: O(n)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...