Create an Online Art Auction using HTML, CSS and JavaScript
Last Updated :
17 Nov, 2023
In this article, we’ll guide you through the process of creating an online art auction website using HTML, CSS, and JavaScript. Art auctions are exciting events where collectors and enthusiasts bid on prized artworks. It will provide a foundation for displaying art listings and creating an appealing user interface.
Preview of final output: Let us have a look at how the final output will look like.
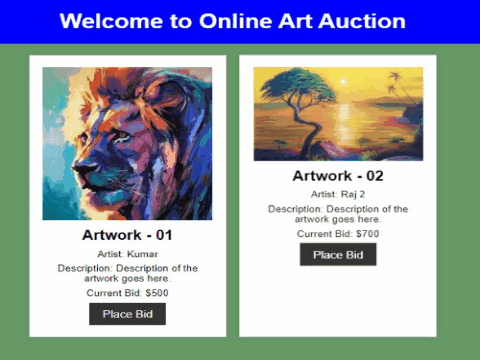
Prerequisites:
Step-Wise Approach to create Online Art Auction:
- Step 1: Setting Up the Project:
- Let’s start by creating a new folder for our project. Inside this folder create two files: index.html, styles.css and script.js. The HTML file will contain the structure of our auction site and CSS file will handle the styling.
- Step 2: Creating the HTML Structure:
- In the index.html file set up the basic structure of your online art auction website.The HTML code sets up the basic structure of your art auction website with the header, auction listings section and footer.
- Step 3: Styling with CSS:
- In the styles.css file you can add styles to make your art auction site visually appealing. The CSS code provides basic styling for header, auction listings section, individual art listing cards and footer. You can customize these styles to match your design preferences.
- Step 4: Adding Art Listings:
- To make your online art auction functional you need to add art listings to auction listings section. Each art listing can be represented as a card within auction-listings section.
- Step 5: Javascript code:
- This javascript code provides the message like once click the button then showing successful message
- Step 6: Testing and Refining:
- After adding content, it’s essential to test your online art auction website in the different web browsers to ensure compatibility. Make refinements to HTML , CSS and javascript code as needed to improve the user experience and styling.
Example: Below is the basic implementaion to create an Online Art Auction using HTML & CSS
Javascript
function placeBid() {
alert( "Successfully Bid!" );
}
const Buttons =
document.querySelectorAll( ".art-card button" );
Buttons.forEach(button => {
button.addEventListener( "click" , placeBid);
});
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >The Online Art Auction</ title >
< link rel = "stylesheet" href = "style.css" >
</ head >
< body >
< header >
< h1 >
Welcome to Online
Art Auction
</ h1 >
</ header >
< section class = "art-listings" >
< div class = "art-card" >
< img src = "a.jpg" alt = "Artwork 1" >
< h2 >Artwork - 01</ h2 >
< p >Artist: Kumar </ p >
< p >
Description: Description of
the artwork goes here.
</ p >
< p >Current Bid: $500</ p >
< button >Place Bid</ button >
</ div >
< div class = "art-card" >
< img src = "b.jpg" alt = "Artwork 2" >
< h2 >Artwork - 02</ h2 >
< p >Artist: Raj 2</ p >
< p >
Description: Description of
the artwork goes here.
</ p >
< p >Current Bid: $700</ p >
< button >Place Bid</ button >
</ div >
</ section >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
* {
margin : 0 ;
padding : 0 ;
box-sizing: border-box;
}
body {
font-family : Arial , sans-serif ;
background-color : #669966 ;
margin : 0 ;
padding : 0 ;
}
header {
background-color : #0000ff ;
color : #fff ;
text-align : center ;
padding : 20px 0 ;
}
h 1 {
font-size : 36px ;
margin : 0 ;
}
.art-listings {
display : flex;
justify- content : center ;
flex-wrap: wrap;
gap: 20px ;
padding : 20px ;
}
.art-card {
background-color : #fff ;
border : 1px solid #ddd ;
padding : 20px ;
text-align : center ;
box-shadow: 0 0 10px rgba( 0 , 0 , 0 , 0.1 );
transition: transform 0.2 s ease;
max-width : 300px ;
}
.art-card:hover {
transform: translateY( -5px );
}
img {
max-width : 100% ;
height : auto ;
}
h 2 {
font-size : 24px ;
margin : 10px 0 ;
}
p {
font-size : 16px ;
margin : 8px 0 ;
}
button {
background-color : #333 ;
color : #fff ;
border : none ;
padding : 10px 20px ;
font-size : 18px ;
cursor : pointer ;
}
footer {
text-align : center ;
background-color : #333 ;
color : #fff ;
padding : 10px 0 ;
}
|
Output:
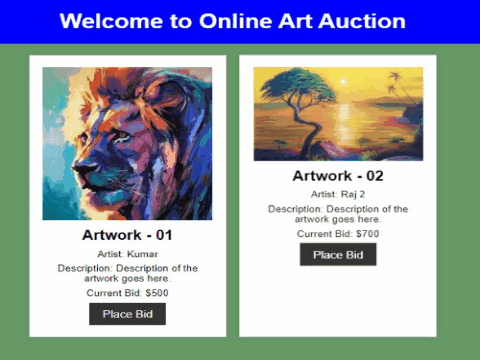
Share your thoughts in the comments
Please Login to comment...