Create a Calculator App Using Next JS
Last Updated :
15 Mar, 2024
Creating a Calculator app is one of the basic projects that clears the core concept of a technology. In this tutorial, we’ll walk you through the process of building a calculator app using Next.js.
Output Preview: Let us have a look at how the final output will look like.
.png)
Prerequisites:
Approach to Create a Calculator App Using NextJS:
- Setup the Project by Creating a new Next JS project.
- Create Calculator component for the calculator layout, including buttons for numbers, operators, and other functionality.
- We will use React useState hook to manage the calculator’s state such as current input, operator, and result.
- We will create handleClick function to handle arithmetic operations like addition, subtraction, multiplication, and division.
- Use CSS to style the calculator layout and buttons.
Steps to create the Calculator Application:
Step 1: Create a application of NextJS using the following command.
npx create-next-app calculator
Step 2: Navigate to project directory
cd calculator
Project Structure:
.png)
The updated Dependencies in package.json file will look like:
"dependencies": {
"react": "^18",
"react-dom": "^18",
"next": "14.1.3"
}
Example: Below are the files which describes the basic implementation of theCalculator App.
CSS
/* globals.css */
.calculator {
max-width: 400px;
margin: 0 auto;
padding: 20px;
background-color: #f9f9f9;
border-radius: 10px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
}
.calculator input[type="text"] {
width: 100%;
padding: 10px;
margin-bottom: 10px;
font-size: 24px;
text-align: right;
border: none;
border-bottom: 1px solid #ccc;
outline: none;
background-color: #fff;
}
.buttons {
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 10px;
}
button {
padding: 15px;
font-size: 20px;
border: none;
outline: none;
cursor: pointer;
border-radius: 5px;
background-color: #f0f0f0;
}
button:hover,
button.operator:hover {
background-color: #e0e0e0;
}
button.operator {
font-size: 1.5rem;
font-weight: bold;
background-color: #f0f0f0;
color: #f0a500;
}
button.wide {
grid-column: span 2;
}
button.clear {
grid-column: span 2;
background-color: #f0a500;
color: white;
}
button.clear:hover {
background-color: #d18d00;
}
button.wide {
grid-column: span 2;
}
h1 {
text-align: center;
color: green;
}
h2 {
text-align: center;
}
Javascript
// page.js
import Calculator from './components/Calculator';
export default function Home() {
return (
<div>
<Calculator />
</div>
);
}
Javascript
// Calculator.js
'use client'
import '../globals.css';
import React, { useState } from 'react';
const Calculator = () => {
const [result, setResult] = useState('');
const handleClick = (value) => {
if (value === '=') {
try {
setResult(eval(result) || '');
} catch (error) {
setResult('Error');
}
} else if (value === 'C') {
setResult('');
} else if (value === 'CE') {
setResult(result.slice(0, -1));
} else {
setResult(result + value);
}
};
return (
<>
<h1>GeeksForGeeks</h1>
<h2>Calculator App</h2>
<div className="calculator">
<input type="text" className="form-control mb-3"
value={result} readOnly />
<div className="buttons">
<button onClick={() =>
handleClick('7')}>7</button>
<button onClick={() =>
handleClick('8')}>8</button>
<button onClick={() =>
handleClick('9')}>9</button>
<button className="operator" onClick={() =>
handleClick('CE')}>CE</button>
<button onClick={() =>
handleClick('4')}>4</button>
<button onClick={() =>
handleClick('5')}>5</button>
<button onClick={() =>
handleClick('6')}>6</button>
<button className="operator" onClick={() =>
handleClick('/')}>/</button>
<button onClick={() =>
handleClick('1')}>1</button>
<button onClick={() =>
handleClick('2')}>2</button>
<button onClick={() =>
handleClick('3')}>3</button>
<button className="operator" onClick={() =>
handleClick('*')}>*</button>
<button onClick={() =>
handleClick('0')}>0</button>
<button onClick={() =>
handleClick('.')}>.</button>
<button onClick={() =>
handleClick('00')}>00</button>
<button className="operator" onClick={() =>
handleClick('-')}>-</button>
<button className="operator wide" id='clear' onClick={() =>
handleClick('C')}>C</button>
<button className="operator" onClick={() =>
handleClick('=')}>=</button>
<button className="operator" onClick={() =>
handleClick('+')}>+</button>
</div>
</div>
</>
);
};
export default Calculator;
To run the application, type the following command in terminal:
npm run dev
Output: Naviage to the URL http://localhost:3000.
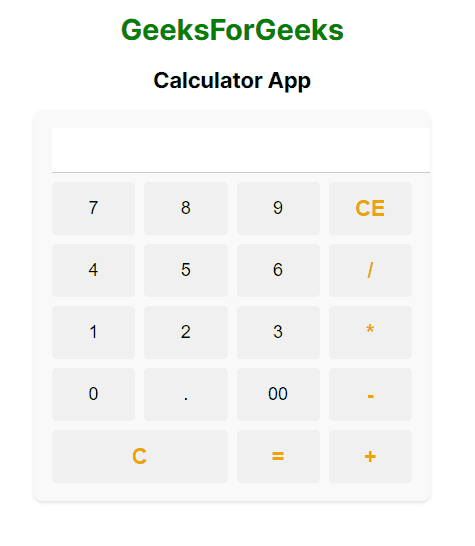
Share your thoughts in the comments
Please Login to comment...