Decision-making in C++ involves the usage of conditional statements (also called decision control statements) to execute specific blocks of code primarily based on given situations and their results.
So basically, in decision-making, we evaluate the conditions and make a decision about which part of the code should be executed or not. It allows selective code execution which is crucial for controlling the flow of a program and making it more dynamic.
Types of Decision-Making Statements in C++
In C++, the following decision-making statements are available:
- if Statement
- if-else Statement
- if-else-if Ladder
- Nested if Statement
- switch Statement
- Conditional Operator
- Jump Statements: break, continue, go, return
1. if in C++
In C++, the if statement is the simplest decision-making statement. It allows the execution of a block of code if the given condition is true. The body of the ‘if’ statement is executed only if the given condition is true.
Syntax of if in C++
if (condition) {
// code to be executed if the condition is true
}
Here if the condition is true then the code inside the if block will be executed otherwise not.
Flowchart of if in C++
Example of if in C++
The below example demonstrates the use of the if statement by finding if the age of a person is greater than 18 or not. if the condition is true then he/she is allowed to vote.
C++
#include <iostream>
using namespace std;
int main()
{
int age = 19;
if (age > 18) {
cout << "allowed to vote" << endl;
}
return 0;
}
|
2. if-else in C++
The if-else decision-making statement allows us to make a decision based on the evaluation of a given condition. If the given condition evaluates to true then the code inside the ‘if’ block is executed and in case the condition is false, the code inside the ‘else’ block is executed.
Syntax of if-else in C++
if (condition) {
// Code to be executed if the condition is true
}
else {
// Code to be executed if the condition is false
}
Flowchart of if-else in C++
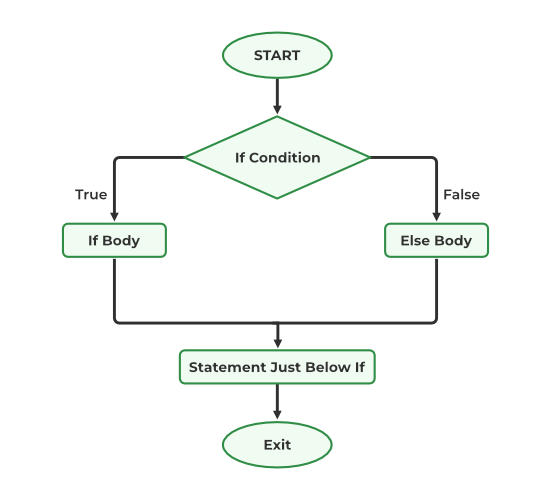
Flow diagram of if else
Example of if-else in C
The below example demonstrates the use of an if-else statement to find if the given number is positive or nonpositive.
C++
#include <iostream>
using namespace std;
int main()
{
int num = 5;
if (num > 0) {
cout << "number is positive." << endl;
}
else {
cout << "number is non-positive." << endl;
}
return 0;
}
|
Output
number is positive.
3. if-else if Ladder in C++
The if-else-if statements allow us to include additional situations after the preliminary if condition. The ‘else if’ condition is checked only if the above condition is not true. , and the `else` is the statement that will be executed if none of the above conditions is true. If some condition is true, then no only the associated block is executed.
Syntax if-else-if Ladder
if (condition1) {
// code to be executed if condition1 is true
}
else if (condition2) {
// code to be executed if condition2 is true
}
else {
// code to be executed if both the condition is false
}
We can use multiple else if statements with an if-else pair to specify different conditions.
Flowchart of if-else-if Ladder in C++
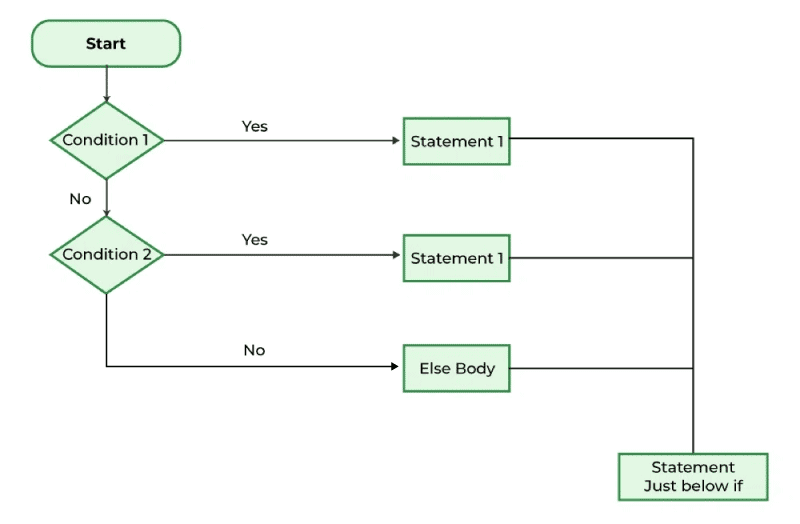
flow diagram of the if-else-if ladder
Example of if-else-ladder in C++
The below example demonstrates the use of an if-else-if ladder. In the program, you are given an age and if the age is less the 13 print child, if the age is between 13 to 18 print the growing stage, else print adult.
C++
#include <iostream>
using namespace std;
int main()
{
int age = 18;
if (age < 13) {
cout << "child" << endl;
}
else if (age >= 1 and age <= 18) {
cout << "Growing stage" << endl;
}
else {
cout << "adult" << endl;
}
return 0;
}
|
4. Nested if-else in C++
The Nested if-else statement contains an ‘if’ statement inside another ‘if’ statement. This structure lets in more complex selection-making by way of comparing multiple conditions. In this type of statement, multiple conditions are checked, and then the body of the last if statement is executed.
Syntax of Nested if-else in C++
if (condition1) {
// code to be executed if condition 1 is true
if (condition2) {
// code to be executed when condition 2 is true.
}
else {
// code to be executed if condition1 is true but condition2 is false.
}
}
else {
// code to be executed when condition 1 is false
}
Flowchart of Nested if-else
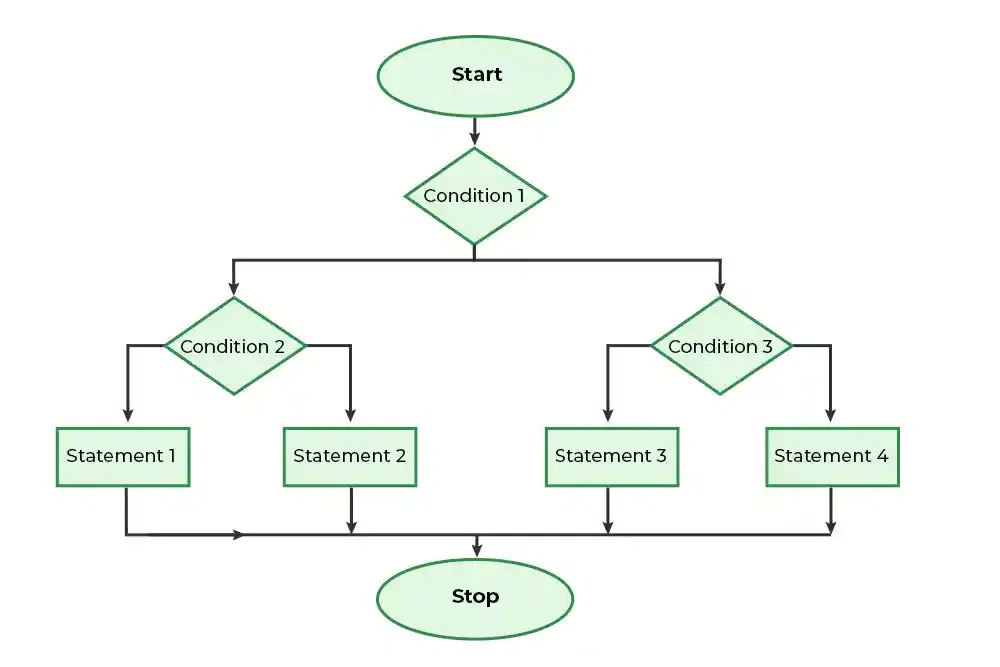
Flow diagram of Nested if-else
Example of Nested if-else in C++
The below example demonstrates the use of nested if-else. In the below program, we are given a number and we have to check whether the number is positive or negative or zero if the number is positive check whether it is even or odd.
C++
#include <iostream>
using namespace std;
int main()
{
int number = 44;
if (number > 0) {
if (number % 2 == 0) {
cout << "positive and even number" << endl;
}
else {
cout << "positive and odd number" << endl;
}
}
else if (number == 0) {
cout << "the number is zero" << endl;
}
else {
cout << "the number is negative" << endl;
}
return 0;
}
|
Output
positive and even number
5. Switch Statement in C++
In C++, the switch statement is used when multiple situations need to be evaluated primarily based on the value of a variable or an expression. switch statement acts as an alternative to multiple if statements or if-else ladder and has a cleaner structure and it is easy for handling multiple conditions.
Syntax of C++ switch
switch (expression) {
case value1:
// code to be executed if the value of expression is value1.
break;
case value2:
// code to be executed if the value of expression is value2.
break;
//…..
default:
// code to be executed if expression doesn't match any case.
}
Flowchart of switch in C++
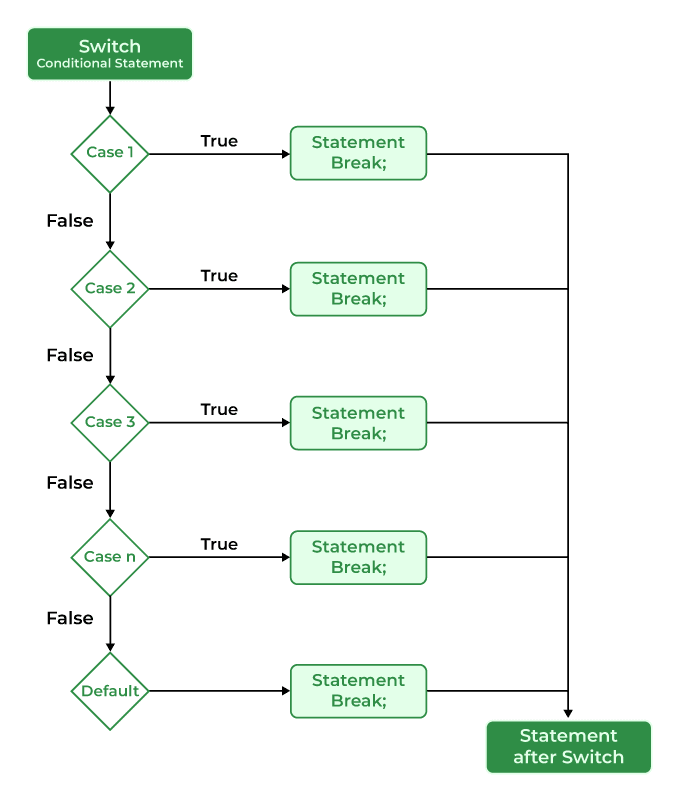
Flowchart of switch in C++
Example pf switch in C++
The below example demonstrates the use of switches in decision-making. In the below program, we are given a character and you have to give output as per the given condition: if the given input is A then print GFG, and if the given input is B print GeeksforGeeks else print invalid input.
C++
#include <iostream>
using namespace std;
int main()
{
char input = 'B' ;
switch (input) {
case 'A' :
cout << "GFG" << endl;
break ;
case 'B' :
cout << "GeeksforGeeks" << endl;
break ;
default :
cout << "invalid input" << endl;
}
return 0;
}
|
6. Ternary Operator ( ? : ) in C++
The conditional operator is also known as a ternary operator. It is used to write conditional operations provided by C++. The ‘?’ operator first checks the given condition, if the condition is true then the first expression is executed otherwise the second expression is executed. It is an alternative to an if-else condition in C++.
Syntax of Ternary Operator in C++
condition ? expression1 : expression2
Flowchart of Conditional Operator in C++
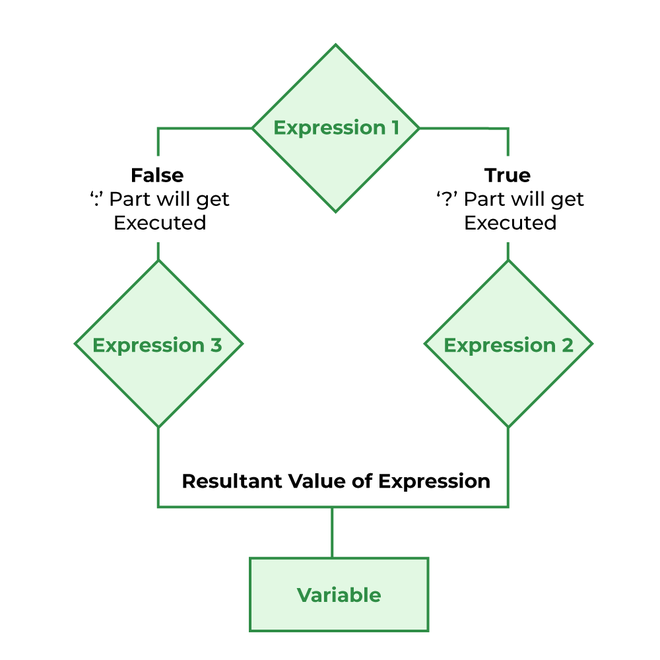
Flow Diagram of Conditional operator
Example of Ternary Operator in C++
The below program demonstrates the use of a conditional operator to find the maximum of two numbers.
C++
#include <iostream>
using namespace std;
int main()
{
int num1 = 10, num2 = 40;
int max;
max = (num1 > num2) ? num1 : num2;
cout << max;
return 0;
}
|
7. Jump Statements in C++
Jump statements are used to alter the normal flow of the code. If you want to break the flow of the program without any conditions then these jump statements are used. C++ provides four types of jump statements.
- break
- continue
- goto
- return
A) break
break is a control flow statement that is used to terminate the loop and switch statements whenever a break statement is encountered and transfer the control to the statement just after the loop.
Syntax
break;
break statement is generally used when the actual number of iterations are not predefined so we want to terminate the loop based on some conditions.
Flowchart of break
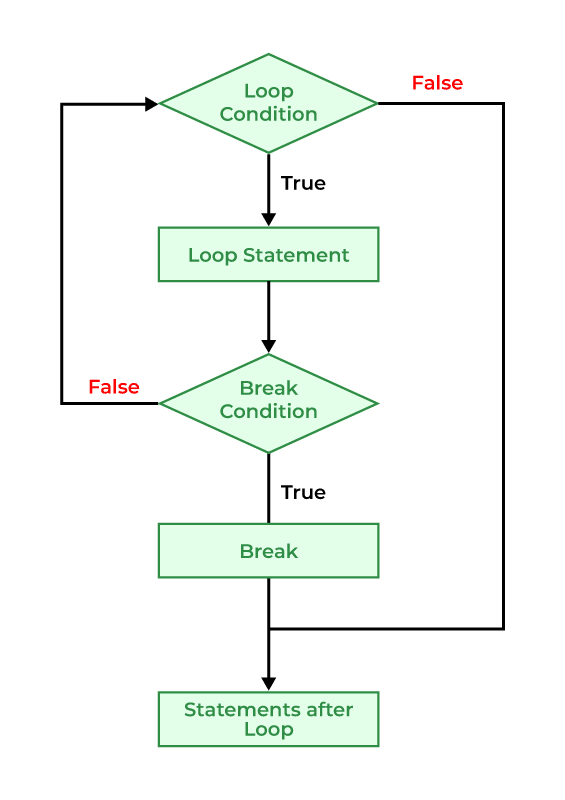
Flow diagram of break
Example
The below example demonstrates the use of breaks to manage the control flow.
C++
#include <iostream>
using namespace std;
int main()
{
for ( int i = 0; i < 5; i++) {
if (i == 3) {
break ;
}
cout << i << endl;
}
return 0;
}
|
B) continue
The continue statement is used to skip the loop body for the current iteration and continue from the next iteration. Unlike the break statement which terminates the loop completely, continue allows us just to skip one iteration and continue with the next iteration.
Syntax
continue;
Flowchart of continue
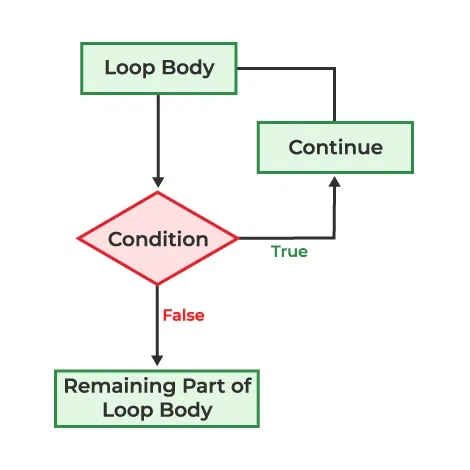
Flow diagram of continue
Example
The below example demonstrates the use of continue to manage the control flow.
C++
#include <iostream>
using namespace std;
int main()
{
for ( int i = 0; i < 5; i++) {
if (i == 3) {
continue ;
}
cout << i << endl;
}
return 0;
}
|
C) goto
It is a jump statement that is used to transfer the control to another part of the program. It transfers the control unconditionally to the labeled statement.
Syntax
goto label;
// ...
label:
// Statement or block of code
Flowchart of goto
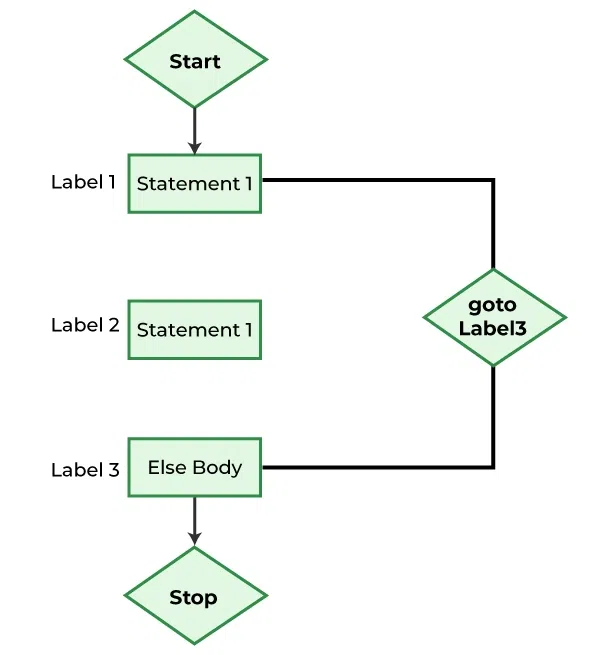
Flow Diagram of goto
Example
The below example demonstrates the use of the goto statement.
C++
#include <iostream>
using namespace std;
int main()
{
int age = 17;
if (age < 18) {
goto Noteligible;
}
else {
cout << "You can vote!" ;
}
Noteligible:
cout << "You are not eligible to vote!\n" ;
return 0;
}
|
Output
You are not eligible to vote!
Note Use of goto is generally avoided in modern programming practices because it may disturb the readability of the code and make the code error-prone, although it is still valid and used occasionally.
D) return
The return statement is used to exit the function immediately and optionally returns a value to the calling function. It returns to the function from where it was called and if it is the ‘main’ function then it marks the end of the execution. So basically, return is a mechanism used to communicate the results back to the calling function.
Syntax
return expression;
Flowchart of return
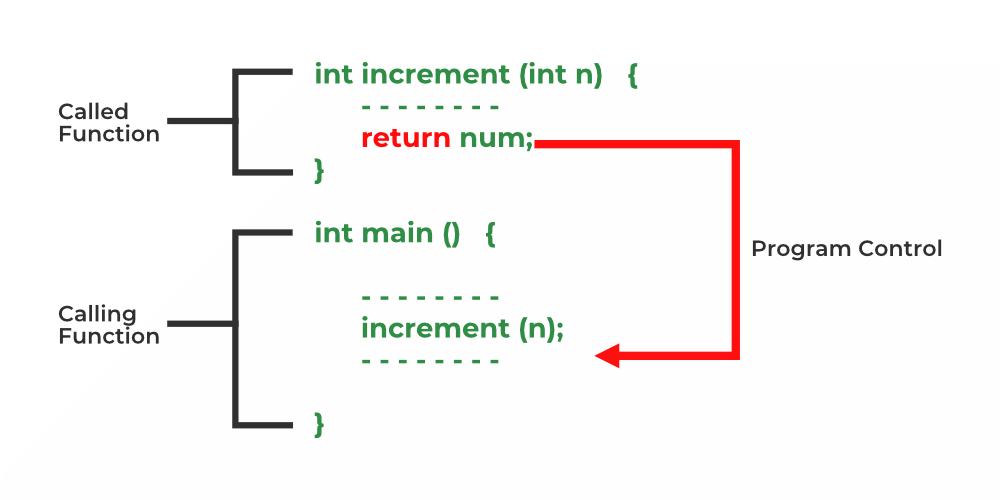
Flow diagram of return
Example
The below example demonstrates the use of a return statement to return a value from a function.
C++
#include <iostream>
using namespace std;
int add( int a, int b)
{
int sum = a + b;
return sum;
}
int main()
{
int res = add(3, 5);
cout << "The sum is: " << res << endl;
return 0;
}
|
Conclusion
Decision-making is an essential factor of programming in C++, presenting flexibility and management over program execution. When used correctly, it enhances application performance and adaptability. However, overuse or mismanagement of decision-making statements can lead to code complexity, decreased clarity, and protection challenges. Striking a balance and using those statements properly is essential to enhance your code.
Share your thoughts in the comments
Please Login to comment...