C++ Nested if-else Statement
Last Updated :
31 Jul, 2023
In C++, there are various types of conditional statements such as if, if-else, nested if, nested if-else, and switch. In this article, we will learn about the nested if-else statement.
What are Nested if-else Statements?
Nested if-else statements are those statements in which there is an if statement inside another if else. We use nested if-else statements when we want to implement multilayer conditions(condition inside the condition inside the condition and so on). C++ allows any number of nesting levels.
Basic Syntax of Nested if-else
if(condition1)
{
// Code to be executed
if(condition2)
{
// Code to be executed
}
else
{
// Code to be executed
}
}
else
{
// code to be executed
}
In the above syntax of nested if-else statements, the inner if-else statement is executed only if ‘condition1’ becomes true otherwise else statement will be executed and this same rule will be applicable for inner if-else statements.
Flowchart of Nested if-else
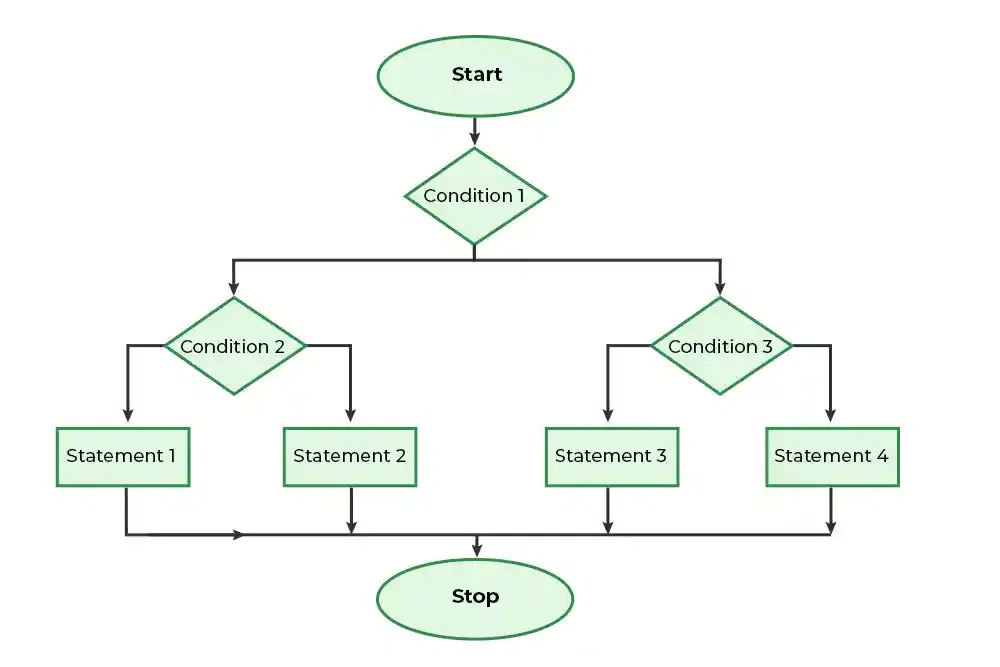
Flow Diagram of Nested if-else
Examples of Nested if-else
The following C++ programs demonstrate the use of nested if-else statements.
Example 1: Check the greatest of three numbers
In the below example, we will find the greatest number out of the three given numbers using nested if-else statements.
Algorithm
Assume that a, b, and c are three given numbers:
- If ( a < b ) evaluates to true, then
- If ( c < b ) is true, then b is greatest.
- else c is greatest.
- If ( a < b ) evaluates to false, then
- If ( c < a ) is true, then a is greatest.
- else c is greatest.
Implementation in C++
C++
#include <iostream>
using namespace std;
int main()
{
int a = 10;
int b = 2;
int c = 6;
if (a < b) {
if (c < b) {
printf ( "%d is the greatest" , b);
}
else {
printf ( "%d is the greatest" , c);
}
}
else {
if (c < a) {
printf ( "%d is the greatest" , a);
}
else {
printf ( "%d is the greatest" , c);
}
}
return 0;
}
|
Output
10 is the greatest
Example 2: Checking leap year using nested if-else
A year is a leap year if it follows the condition, the year should be evenly divisible by 4 then the year should be evenly divisible by 100 then lastly year should evenly divisible by 400 otherwise the year is not a leap year.
Algorithm
- If the year is divisible by 4,
- If the year is divisible by 100,
- If the year is divisible by 400, the year is a leap year
- else the year is not a leap year.
- else the year is a leap year
- else, the year is not a leap year.
Implementation in C++
C++
#include <iostream>
using namespace std;
int main()
{
int year = 2023;
if (year % 4 == 0) {
if (year % 100 == 0) {
if (year % 400 == 0) {
cout << year << " is a leap year." ;
}
else {
cout << year << " is not a leap year." ;
}
}
else {
cout << year << " is a leap year." ;
}
}
else {
cout << year << " is not a leap year." ;
}
return 0;
}
|
Output
2023 is not a leap year.
If the input year is 2023:
Output
2023 is not a leap year.
If the input year is 2024:
Output
2024 is a leap year.
Share your thoughts in the comments
Please Login to comment...