Correct the Random Pointer in Doubly Linked List
Last Updated :
13 Sep, 2023
Given a doubly linked list having exactly one of the node pointing to a random node in the list, the task is to correct this random pointer in the doubly linked list, such that it points to the expected node.
Examples:
Input:
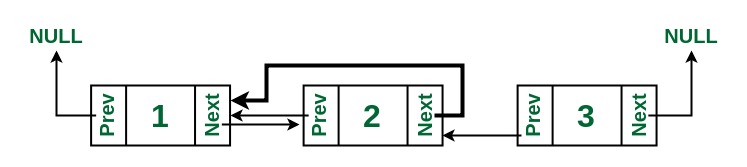
Output:
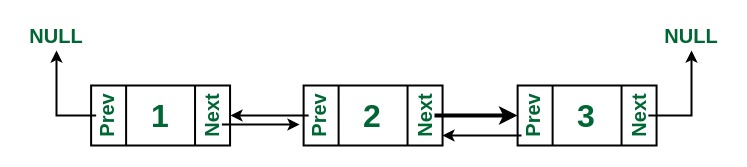
Explanation: 2’s next pointer has been corrected to point to 3. Earlier it was pointing to 1, which was incorrect.
Approach: This can be achieved by simply iterating the list and checking the individual pointers.
Below is the implementation for the above approach:
C++
#include<iostream>
using namespace std;
class Node
{
public :
int data;
Node *next;
Node *prev;
Node():prev(NULL),next(NULL){}
Node( int data):data(data),prev(NULL),next(NULL){}
};
class doublell
{
public :
Node *head;
void appendNode(Node *n)
{
Node *temp=head;
if (temp==NULL)
{
head=n;
}
else
{
while (temp->next!=NULL)
{
temp=temp->next;
}
temp->next=n;
n->prev=temp;
}
}
void print()
{
Node *temp=head;
while (temp!=NULL)
{
cout<<temp->data<< "->" ;
temp=temp->next;
}
cout<<endl;
}
void printReverse()
{
Node *temp=head;
while (temp->next!=NULL)
{
temp=temp->next;
}
while (temp!=NULL)
{
cout<<temp->data<< " ->" ;
temp=temp->prev;
}
cout<<endl;
}
void correctPointer()
{
if (!head)
{
return ;
}
Node *temp=head;
while (temp->next!=NULL)
{
if (temp->next->prev!=temp)
{
temp->next->prev=temp;
}
temp=temp->next;
}
}
};
int main()
{
doublell ll;
ll.head = new Node(1);
ll.head->next = new Node(2);
ll.head->next->prev = ll.head;
ll.head->next->next = new Node(3);
ll.head->next->next->prev =ll.head;
ll.head->next->next->next = new Node(4);
ll.head->next->next->next->prev = ll.head->next->next;
cout << "\nIncorrect Linked List: " ;
ll.print();
ll.printReverse();
ll.correctPointer();
cout << "\nCorrected Linked List: " ;
ll.print();
ll.printReverse();
return 0;
}
|
Java
class GFG
{
static class node
{
int data;
node next;
node prev;
};
static node newNode( int data)
{
node temp = new node();
temp.data = data;
temp.next = temp.prev = null ;
return temp;
}
static void correctPointer(node head)
{
if (head == null )
return ;
node temp = head;
if (head.next != null &&
head.next.prev != head)
{
head.next.prev = head;
return ;
}
if (head.prev != null )
{
head.prev = null ;
return ;
}
temp = temp.next;
while (temp != null )
{
if (temp.next != null &&
temp.next.prev != temp)
{
temp.next.prev = temp;
return ;
}
else if (temp.prev != null &&
temp.prev.next != temp)
{
temp.prev.next = temp;
return ;
}
System.out.print( "" );
temp = temp.next;
}
}
static void printList(node head)
{
node temp = head;
while (temp != null )
{
System.out.print(temp.data + " (" );
System.out.print((temp.prev != null ?
temp.prev.data: - 1 ) + ") " );
temp = temp.next;
}
System.out.print( "\n" );
}
public static void main(String[] args)
{
node head = newNode( 1 );
head.next = newNode( 2 );
head.next.prev = head;
head.next.next = newNode( 3 );
head.next.next.prev = head;
head.next.next.next = newNode( 4 );
head.next.next.next.prev = head.next.next;
System.out.print( "\nIncorrect Linked List: " );
printList(head);
correctPointer(head);
System.out.print( "\nCorrected Linked List: " );
printList(head);
}
}
|
Python3
class Node:
def __init__( self , data):
self .data = data
self .prev = None
self . next = None
def correctPointer(head):
if head = = None :
return
temp = head
if (head. next ! = None and
head. next .prev ! = head):
head. next .prev = head
return
if head.prev ! = None :
head.prev = None
return
temp = temp. next
while temp ! = None :
if (temp. next ! = None and
temp. next .prev ! = temp):
temp. next .prev = temp
return
elif (temp.prev ! = None and
temp.prev. next ! = temp):
temp.prev. next = temp
return
temp = temp. next
def printList(head):
temp = head
while temp ! = None :
print (temp.data, "(" , end = "")
if temp.prev = = None :
print ( - 1 , end = ") " )
else :
print (temp.prev.data, end = ") " )
temp = temp. next
print ()
if __name__ = = "__main__" :
head = Node( 1 )
head. next = Node( 2 )
head. next .prev = head
head. next . next = Node( 3 )
head. next . next .prev = head
head. next . next . next = Node( 4 )
head. next . next . next .prev = head. next . next
print ( "Incorrect Linked List:" ,
end = " " )
printList(head)
correctPointer(head)
print ( "\nCorrected Linked List:" ,
end = " " )
printList(head)
|
C#
using System;
class GFG
{
class node
{
public int data;
public node next;
public node prev;
};
static node newNode( int data)
{
node temp = new node();
temp.data = data;
temp.next = temp.prev = null ;
return temp;
}
static void correctPointer(node head)
{
if (head == null )
return ;
node temp = head;
if (head.next != null &&
head.next.prev != head)
{
head.next.prev = head;
return ;
}
if (head.prev != null )
{
head.prev = null ;
return ;
}
temp = temp.next;
while (temp != null )
{
if (temp.next != null &&
temp.next.prev != temp)
{
temp.next.prev = temp;
return ;
}
else if (temp.prev != null &&
temp.prev.next != temp)
{
temp.prev.next = temp;
return ;
}
Console.Write( "" );
temp = temp.next;
}
}
static void printList(node head)
{
node temp = head;
while (temp != null )
{
Console.Write(temp.data + " (" );
Console.Write((temp.prev != null ?
temp.prev.data: -1) + ") " );
temp = temp.next;
}
Console.Write( "\n" );
}
public static void Main(String[] args)
{
node head = newNode(1);
head.next = newNode(2);
head.next.prev = head;
head.next.next = newNode(3);
head.next.next.prev = head;
head.next.next.next = newNode(4);
head.next.next.next.prev = head.next.next;
Console.Write( "\nIncorrect Linked List: " );
printList(head);
correctPointer(head);
Console.Write( "\nCorrected Linked List: " );
printList(head);
}
}
|
Javascript
class Node {
constructor(data) {
this .data = data;
this .prev = null ;
this .next = null ;
}
}
class doublell {
constructor() {
this .head = null ;
}
appendNode(n) {
let temp = this .head;
if (!temp) {
this .head = n;
} else {
while (temp.next) {
temp = temp.next;
}
temp.next = n;
n.prev = temp;
}
}
print() {
let temp = this .head;
let result = "" ;
while (temp) {
result += temp.data + " -> " ;
temp = temp.next;
}
console.log(result);
}
printReverse() {
let temp = this .head;
while (temp && temp.next) {
temp = temp.next;
}
let result = "" ;
while (temp) {
result += temp.data + " -> " ;
temp = temp.prev;
}
console.log(result);
}
correctPointer() {
if (! this .head) {
return ;
}
let temp = this .head;
while (temp.next) {
if (temp.next.prev !== temp) {
temp.next.prev = temp;
}
temp = temp.next;
}
}
}
const ll = new doublell();
ll.head = new Node(1);
ll.head.next = new Node(2);
ll.head.next.prev = ll.head;
ll.head.next.next = new Node(3);
ll.head.next.next.prev = ll.head;
ll.head.next.next.next = new Node(4);
ll.head.next.next.next.prev = ll.head.next.next;
console.log( "\nIncorrect Linked List: " );
ll.print();
ll.printReverse();
ll.correctPointer();
console.log( "\nCorrected Linked List: " );
ll.print();
ll.printReverse();
|
Output
Incorrect Linked List: 1 (-1) 2 (1) 3 (1) 4 (3)
Corrected Linked List: 1 (-1) 2 (1) 3 (2) 4 (3)
Time Complexity: O(n)
Auxiliary Space: O(n)
Share your thoughts in the comments
Please Login to comment...