YAML (YAML Ain’t Markup Language) and JSON (JavaScript Object Notation) are both popular data serialization formats. Both these formats are used to represent the data in various scenarios, We can convert the YAML to JSON format by using a mixture of Python Library and Shell Script for automation. We can convert the single YAML file along with a bunch of YAML files in one go and get the desired JSON file generated.
Prerequisites:
- Linux Environment: This tutorial assumes you are working in a Linux environment. The provided instructions and script are tailored for Linux systems.
- Terminal Access: You should have access to a terminal or command-line interface on your Linux system. Most Linux distributions provide this by default.
- Python Installed: The provided script in the tutorial uses Python. Python is commonly pre-installed on many Linux distributions.
Example:
Input:
Enter the numbers of the YAML files to convert to JSON (comma-separated) or 'a' for all: a
Output:
Converted /home/kali/Desktop/file.yaml to /home/kali/Desktop/file.json
Here our main task is to write the script which will take the input as one or more YAML files and convert these YAML files in the appropriate correct JSON file format. We are also saving the converted JSON file in the same directory where the YAML file is taken as input.
Creating the Script
Step 1: In this step, the script checks whether the PyYAML library is installed on the system. PyYAML is a Python library for working with YAML files. If it’s not installed, the script offers to install it using pip, the Python package manager. If we choose not to install PyYAML, the script exits with an error message.
#!/bin/bash
CYAN='\e[96m'
YELLOW='\e[93m'
GREEN='\e[92m'
RESET='\e[0m'
if ! python -c "import yaml" &>/dev/null; then
echo -e "${YELLOW}PyYAML is not installed. Do you want to install it? (y/n)${RESET}"
read -r install_choice
if [ "$install_choice" == "y" ]; then
echo -e "${CYAN}Installing PyYAML...${RESET}"
pip install pyyaml
else
echo -e "${YELLOW}YAML to JSON conversion requires PyYAML. Exiting.${RESET}"
exit 1
fi
fi
Step 2: In this step, the script lists the available YAML files in the user’s Desktop directory. It defines the desktop_dir variable to store the path to the user’s Desktop directory. It uses a wildcard (*.yaml) to create an array of YAML files in the Desktop directory and stores them in the yaml_files variable. If no YAML files are found, the script displays an error message and exits.
desktop_dir="$HOME/Desktop"
yaml_files=("$desktop_dir"/*.yaml)
if [ ${#yaml_files[@]} -eq 0 ]; then
echo -e "${YELLOW}No YAML files found on the Desktop.${RESET}"
exit 1
fi
Step 3: This step allows the user to select one or more YAML files for conversion to JSON. The user can choose individual files or select “a” to convert all available YAML files. It prompts the user to select YAML files for conversion. It displays the available YAML files on the Desktop with corresponding indices. The user can either specify the index of the file(s) to convert, separated by commas, or type “a” to convert all files. If the user chooses “a,” the script enters a loop to convert all available YAML files. If the user selects specific files, the script enters a loop to convert those selected files.
echo -e "${CYAN}Available YAML files on the Desktop:${RESET}"
for ((i=0; i<${#yaml_files[@]}; i++)); do
echo "[$i] $(basename "${yaml_files[$i]}")"
done
echo -e "${CYAN}Enter the numbers of the YAML files to convert to JSON (comma-separated) or 'a' for all:${RESET}"
read -r selection
if [ "$selection" == "a" ]; then
for ((i=0; i<${#yaml_files[@]}; i++)); do
selected_yaml="${yaml_files[$i]}"
output_json="${selected_yaml%.yaml}.json"
echo -e "\n${CYAN}Contents of $selected_yaml:${RESET}"
cat "$selected_yaml"
python -c "import yaml, json; print(json.dumps(yaml.load(open('$selected_yaml'), Loader=yaml.FullLoader), indent=4))" > "$output_json"
echo -e "${GREEN}Converted $selected_yaml to $output_json${RESET}"
echo -e "\n${CYAN}Contents of $output_json:${RESET}"
cat "$output_json"
done
else
IFS=',' read -ra selected_indices <<< "$selection"
for index in "${selected_indices[@]}"; do
if ! [[ $index =~ ^[0-9]+$ ]] || [ $index -ge ${#yaml_files[@]} ]; then
echo -e "${YELLOW}Invalid selection. Exiting.${RESET}"
exit 1
fi
Step 4: In this step, the script performs the actual YAML to JSON conversion for the selected files. It also displays the contents of the YAML files before and after conversion.
selected_yaml="${yaml_files[$index]}"
output_json="${selected_yaml%.yaml}.json"
echo -e "\n${CYAN}Contents of $selected_yaml:${RESET}"
cat "$selected_yaml"
python -c "import yaml, json; print(json.dumps(yaml.load(open('$selected_yaml'), Loader=yaml.FullLoader), indent=4))" > "$output_json"
echo -e "${GREEN}Converted $selected_yaml to $output_json${RESET}"
echo -e "\n${CYAN}Contents of $output_json:${RESET}"
cat "$output_json"
Steps to create and execute a bash script
Step 1: Open the terminal window using the keyboard shortcut “Ctrl + Alt + T“.
-min.png)
Opening Terminal
Step 2: Using any text editor like Vim, vi, or Nano, open a new blank file in the text editor.
nano yamlToJson.sh
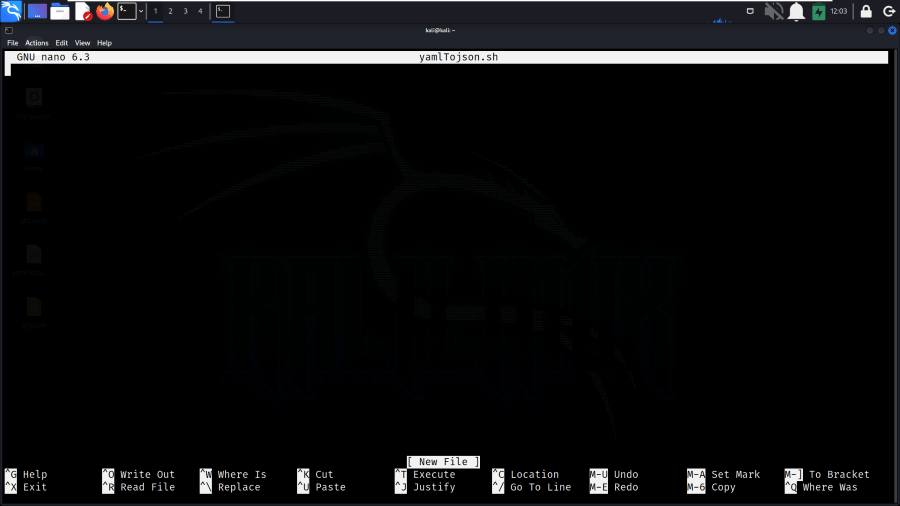
Creating script in the nano editor
Step 3: Now, we need to write the below script in the created file yamlToJson.sh.
#!/bin/bash
# ANSI escape codes for text formatting
CYAN='\e[96m'
YELLOW='\e[93m'
GREEN='\e[92m'
RESET='\e[0m'
# Check if PyYAML is installed, and if not, install it.
if ! python -c "import yaml" &>/dev/null; then
echo -e "${YELLOW}PyYAML is not installed. Do you want to install it? (y/n)${RESET}"
read -r install_choice
if [ "$install_choice" == "y" ]; then
echo -e "${CYAN}Installing PyYAML...${RESET}"
pip install pyyaml
else
echo -e "${YELLOW}YAML to JSON conversion requires PyYAML. Exiting.${RESET}"
exit 1
fi
fi
# List available YAML files on the Desktop
desktop_dir="$HOME/Desktop"
yaml_files=("$desktop_dir"/*.yaml)
if [ ${#yaml_files[@]} -eq 0 ]; then
echo -e "${YELLOW}No YAML files found on the Desktop.${RESET}"
exit 1
fi
# Prompt the user to select YAML files for conversion
echo -e "${CYAN}Available YAML files on the Desktop:${RESET}"
for ((i=0; i<${#yaml_files[@]}; i++)); do
echo "[$i] $(basename "${yaml_files[$i]}")"
done
echo -e "${CYAN}Enter the numbers of the YAML files to convert to JSON (comma-separated) or 'a' for all:${RESET}"
read -r selection
if [ "$selection" == "a" ]; then
for ((i=0; i<${#yaml_files[@]}; i++)); do
selected_yaml="${yaml_files[$i]}"
output_json="${selected_yaml%.yaml}.json"
# Display the contents of the YAML file
echo -e "\n${CYAN}Contents of $selected_yaml:${RESET}"
cat "$selected_yaml"
# Convert YAML to JSON using PyYAML
python -c "import yaml, json; print(json.dumps(yaml.load(open('$selected_yaml'), Loader=yaml.FullLoader), indent=4))" > "$output_json"
echo -e "${GREEN}Converted $selected_yaml to $output_json${RESET}"
# Display the contents of the generated JSON file
echo -e "\n${CYAN}Contents of $output_json:${RESET}"
cat "$output_json"
done
else
IFS=',' read -ra selected_indices <<< "$selection"
for index in "${selected_indices[@]}"; do
if ! [[ $index =~ ^[0-9]+$ ]] || [ $index -ge ${#yaml_files[@]} ]; then
echo -e "${YELLOW}Invalid selection. Exiting.${RESET}"
exit 1
fi
selected_yaml="${yaml_files[$index]}"
output_json="${selected_yaml%.yaml}.json"
# Display the contents of the YAML file
echo -e "\n${CYAN}Contents of $selected_yaml:${RESET}"
cat "$selected_yaml"
# Convert YAML to JSON using PyYAML
python -c "import yaml, json; print(json.dumps(yaml.load(open('$selected_yaml'), Loader=yaml.FullLoader), indent=4))" > "$output_json"
echo -e "${GREEN}Converted $selected_yaml to $output_json${RESET}"
# Display the contents of the generated JSON file
echo -e "\n${CYAN}Contents of $output_json:${RESET}"
cat "$output_json"
done
fi
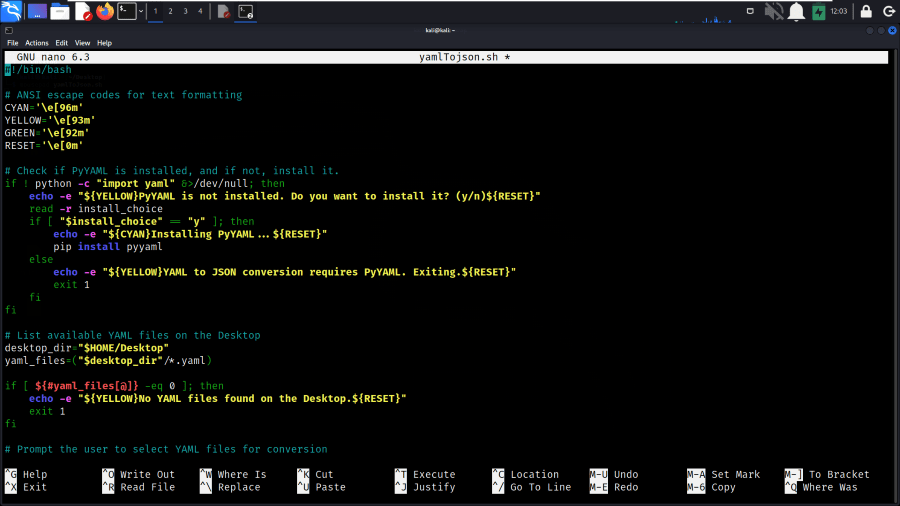
Script Written in File
Step 4: Now, as we have created the script, we need to make the script executable by running the chmod command in the terminal.
chmod +x yamlToJson.sh
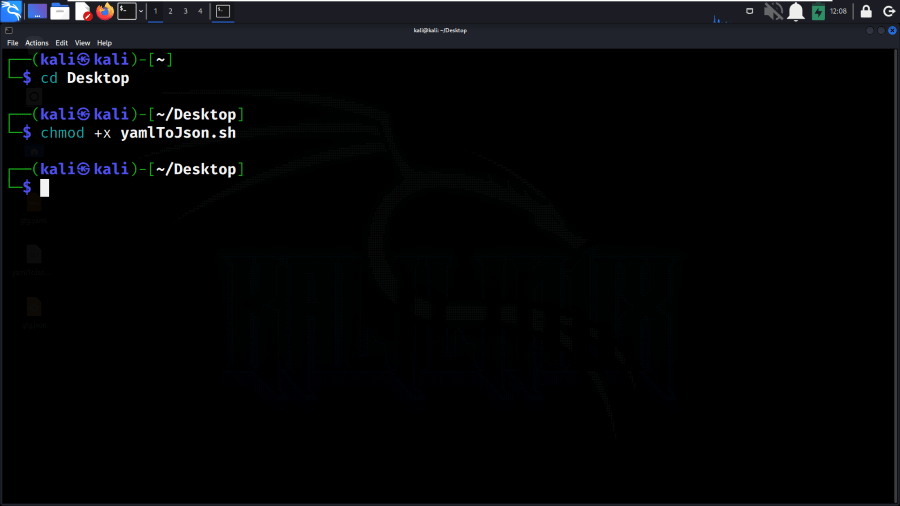
Granting Executable Permissions
Step 5: Create the sample YAML file as the input file for the conversion process.
.png)
Sample YAML File as Input
Step 6: Finally, we need to execute the script by using the below command.
./yamlToJson.sh
Output:
-min.png)
Conversion Completed
Explanation:
In this above output, we can see that we have eexecutedthe script and once the script is been executed, the script has detected all the YAML files in the present directory. As we have created the “gfg.yaml” file, it is been shown in the script. We have given the number of YAML files as “0” and the script has successfully converted the “gfg.yaml” file to the “gfg.json” file. The contents of the output JSON file have also been printed in the script itself. The converted JSON file is been stored in the present directory in which the input YAML file has been stored.
Conclusion
In conclusion, the Linux YAML to JSON conversion script describes the process of converting YAML files to JSON. It checks for and installs the necessary PyYAML library, lists available files, and offers user-friendly options for selecting and converting YAML files. With detailed output, this script simplifies the handling of data in different formats, making it a valuable tool for Linux users.
Share your thoughts in the comments
Please Login to comment...