Complete Guide to Redis Pipelining
Last Updated :
13 Oct, 2023
Redis is unbeatable when it comes to storing and retrieving data. It is open source and functions as an in-memory data structure store that provides performance, low latency data to different applications. Among others, one feature to effectuate this is Redis Pipelining – so stated by Redis. Here we analyze the concept of Redis pipelining
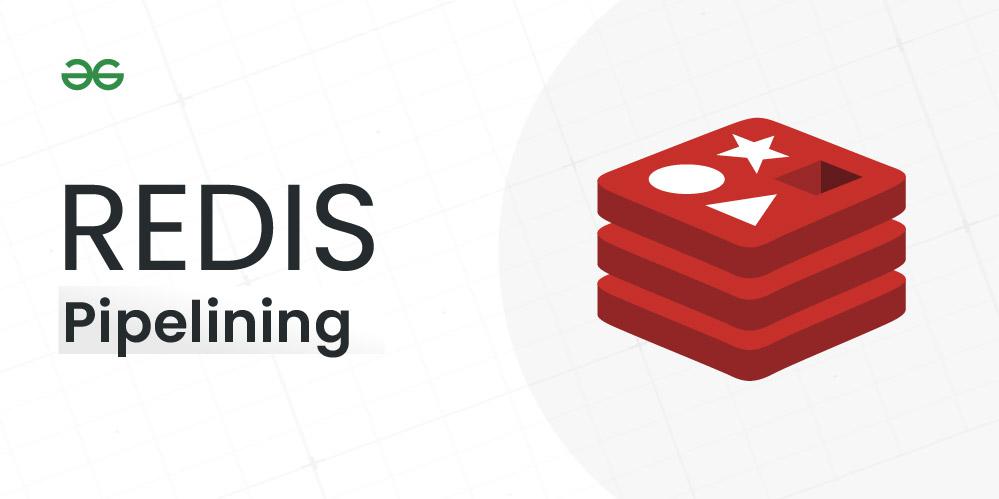
Important Topics for Redis Pipelining
What is Redis Pipelining?
Redis Pipelining is an optimization that helps execute multiple commands in Redis sequentially. Typically for a Redis interaction, a client would first issue a command to the server and then send another when processing of its command is done. The synchronous nature of this approach incurs a considerable delay, especially involving numerous commands.
In contrast with Redis Pipelining, clients can send multiple commands at once to the server without waiting for each answer. To this, the server stores such commands and processes them serially before returning the response after executing all the commands. By using such an approach, the round trip time between the client and server is substantially reduced, leading to quite fast Redis operations.
Request/Response Protocols and Round Trip Time (RTT)
Before diving deeper into Redis Pipelining, it’s essential to understand the concept of Request/Response protocols and Round Trip Time (RTT). In networking, when a client sends a request to a server, it must wait for the server’s response.
The time taken for the request to travel from the client to the server and back is called the Round Trip Time (RTT).
Reducing RTT is crucial for improving the responsiveness of networked applications. Redis Pipelining is a technique that helps minimize RTT in Redis interactions by batching commands.
Commands and Syntax of Redis Pipelining
With Redis pipelining, it is as easy as firing off a sequence of commands in succession without waiting for responses. Here’s a basic outline of how it works:
- First, it starts the TP transaction.
- Redis commands are sent one after the other.
- Finally, execute ‘EXEC’ for all queued transactions.
- Command sent, and received responses in return.
Here is an example sequence in Python using the popular `redis-py` library:
In our instance:
- We start with pipe = r.pipeline()
- Chain two commands (SET and GET)
- Then execute the pipeline through pipe.execute().
- The we can decode and interpret these responses.
Below is the implementation of the example:
Python3
import redis
r = redis.Redis(host = 'localhost' , port = 6379 )
pipe = r.pipeline()
pipe. set ( 'name' , 'Alice' )
pipe.get( 'name' )
responses = pipe.execute()
name = responses[ 1 ].decode( 'utf-8' )
print (f "Name: {name}" )
|
Output:
Name: Alice
Explanation of the output:
- The SET command sets the key ‘name’ with the value ‘Alice’.
- The GET command retrieves the value associated with the key ‘name’.
- The pipeline is executed with pipe.execute(), which sends both commands to the Redis server.
- The responses are stored in the responses list.
- The value retrieved from the GET command is decoded as a UTF-8 string and stored in the variable name.
- Finally, it prints the name, which should be “Alice” based on the input provided.
Redis Pipelining Commands
Here are some commonly used Redis Pipelining commands along with their explanations, syntax, and examples:
1. MULTI: Begins a transaction block for Redis Pipelining.
Syntax: `MULTI`
2. EXEC: Executes all the commands in the transaction block.
Syntax: `EXEC`
Below is an example Using MULTI and EXEC to perform a transaction with Redis Pipelining:
Python3
import redis
r = redis.StrictRedis(host = 'localhost' , port = 6379 , db = 0 )
pipe = r.pipeline()
pipe.multi()
pipe. set ( 'name' , 'Alice' )
pipe.get( 'name' )
pipe. exec ()
result = pipe.execute()
print ( "Name:" , result[ 1 ].decode( 'utf-8' ))
|
3. SET: Sets the value of a key.
Syntax: `SET key value`
4. GET: Retrieves the value associated with a key.
Syntax: `GET key`
Below is an example Using SET and GET commands in a Redis Pipelining:
Python3
import redis
r = redis.StrictRedis(host = 'localhost' , port = 6379 , db = 0 )
pipe = r.pipeline()
pipe. set ( 'name' , 'Alice' )
pipe.get( 'name' )
responses = pipe.execute()
name = responses[ 1 ].decode( 'utf-8' )
print ( "Name:" , name)
|
5. DISCARD: Cancels the transaction block created by MULTI, discarding all queued commands.
Syntax: DISCARD
Below is an example Using DISCARD to cancel a transaction:
Python3
import redis
r = redis.StrictRedis(host = 'localhost' , port = 6379 , db = 0 )
pipe = r.pipeline()
pipe.multi()
pipe. set ( 'name' , 'Alice' )
pipe.discard()
pipe.execute()
value = r.get( 'name' )
print ( "Name:" , value)
|
6. HSET: Sets the field in a hash stored at the given key to the specified value.
Syntax: HSET key field value
7. HGET: Retrieves the value of a field in a hash stored at the given key.
Syntax: HGET key field
Below is an example Using `HSET` and `HGET` commands in Redis Pipelining:
Python3
import redis
r = redis.StrictRedis(host = 'localhost' , port = 6379 , db = 0 )
pipe = r.pipeline()
pipe.hset( 'user:id1' , 'username' , 'Alice' )
pipe.hget( 'user:id1' , 'username' )
responses = pipe.execute()
username = responses[ 1 ].decode( 'utf-8' )
print ( "Username:" , username)
|
8. RPUSH: Appends one or more values to the end of a list.
Syntax: RPUSH key value [value …]
9. LPOP: Removes and returns the first element from a list.
Syntax: LPOP key
Below is an example Using `RPUSH` to add elements to a list and `LPOP` to remove and retrieve elements from the list in Redis Pipelining:
Python3
import redis
r = redis.StrictRedis(host = 'localhost' , port = 6379 , db = 0 )
pipe = r.pipeline()
pipe.rpush( 'tasks' , 'Task 1' , 'Task 2' )
pipe.lpop( 'tasks' )
responses = pipe.execute()
print ( "Task removed:" , responses[ 1 ].decode( 'utf-8' ))
|
Examples of Redis Pipelining
Let’s consider a few practical examples where Redis Pipelining can be beneficial:
1. E-commerce Inventory Management
In an e-commerce system, managing product inventory efficiently is crucial. Redis Pipelining can be used to update product quantities, retrieve stock status, and log inventory changes:
Python3
pipe = r.pipeline()
pipe.decrby( 'product:1:quantity' , 3 )
pipe.get( 'product:1:quantity' )
pipe.rpush( 'inventory:log' , 'Product 1 quantity reduced by 3' )
responses = pipe.execute()
new_quantity = int (responses[ 1 ])
print (f "New Quantity: {new_quantity}" )
|
Javascript
const Redis = require( 'ioredis' );
const redis = new Redis();
(async () => {
const pipeline = redis.pipeline();
pipeline.decrby( 'product:1:quantity' , 3);
pipeline.get( 'product:1:quantity' );
pipeline.rpush( 'inventory:log' , 'Product 1 quantity reduced by 3' );
const responses = await pipeline.exec();
const newQuantityResponse = responses[1][1];
const newQuantity = parseInt(newQuantityResponse, 10);
console.log(`New Quantity: ${newQuantity}`);
redis.quit();
})();
|
Output:
New Quantity: <updated quantity>
The output will be the updated quantity of product 1 after decrementing it by 3.
2. Real-time Leaderboards:
Leaderboards are common in gaming and competitive applications. Redis is often used for leaderboard implementations. Pipelining can help fetch and update leaderboard scores efficiently:
Python3
pipe = r.pipeline()
pipe.zadd( 'leaderboard' , { 'player1' : 1500 , 'player2' : 1800 , 'player3' : 1600 })
pipe.zrevrange( 'leaderboard' , 0 , 9 , withscores = True )
responses = pipe.execute()
top_players = [(player.decode( 'utf-8' ), score)
for player, score in responses[ 1 ]]
print ( "Top Players:" )
for player, score in top_players:
print (f "{player}: {score}" )
|
Javascript
const Redis = require( 'ioredis' );
const redis = new Redis();
(async () => {
const pipeline = redis.pipeline();
pipeline.zadd( 'leaderboard' , { 'player1' : 1500, 'player2' : 1800, 'player3' : 1600 });
pipeline.zrevrange( 'leaderboard' , 0, 9, 'WITHSCORES' );
const responses = await pipeline.exec();
const topPlayersResponse = responses[1][1];
const topPlayers = [];
for (let i = 0; i < topPlayersResponse.length; i += 2) {
const player = topPlayersResponse[i];
const score = parseInt(topPlayersResponse[i + 1], 10);
topPlayers.push({ player, score });
}
console.log( 'Top Players:' );
topPlayers.forEach(({ player, score }) => {
console.log(`${player}: ${score}`);
});
redis.quit();
})();
|
Output:
Top Players:
player2: 1800.0
player3: 1600.0
player1: 1500.0
The output will be the top 10 players and their scores in descending order.
3. Rate Limiting for APIs
Rate limiting is essential for protecting APIs from abuse. Redis Pipelining can be used to enforce rate limits efficiently:
Python3
pipe = r.pipeline()
pipe.zscore( 'ratelimit:user123' , 'timestamp' )
pipe.multi()
pipe.zadd( 'ratelimit:user123' , { 'timestamp' : time.time()})
pipe.expire( 'ratelimit:user123' , 60 )
pipe.execute()
responses = pipe.execute()
timestamp = responses[ 0 ]
if timestamp:
remaining_time = 60 - (time.time() - float (timestamp))
print (f 'Rate limit exceeded. Try again in {remaining_time:.2f} seconds.' )
else :
print ( 'Request allowed.' )
|
Javascript
const Redis = require( 'ioredis' );
const redis = new Redis();
(async () => {
const pipeline = redis.pipeline();
pipeline.zscore( 'ratelimit:user123' , 'timestamp' );
pipeline.multi();
pipeline.zadd( 'ratelimit:user123' , { 'timestamp' : Math.floor(Date.now() / 1000) });
pipeline.expire( 'ratelimit:user123' , 60);
await pipeline.exec();
const timestampResponse = await redis.zscore( 'ratelimit:user123' , 'timestamp' );
const timestamp = parseFloat(timestampResponse);
if (timestamp) {
const currentTime = Math.floor(Date.now() / 1000);
const remainingTime = 60 - (currentTime - timestamp);
console.log(`Rate limit exceeded. Try again in ${remainingTime.toFixed(2)} seconds.`);
} else {
console.log( 'Request allowed.' );
}
redis.quit();
})();
|
Output:
If the user has exceeded the rate limit: Rate limit exceeded. Try again in <remaining_time> seconds.
If the user is within the rate limit: Request allowed.
Note: The output will depend on whether the user has exceeded the rate limit or not, and if exceeded, it will display the remaining time until the rate limit resets.
Pros of Redis Pipelining
- Reduced Latency: Redis Pipelining reduces the effects of network latencies by batching the requests and therefore allows for faster response times.
- Throughput: In this way, Redis becomes an even better solution for a variety of application processes by which it enables hundreds or thousands of operations per second.
- Atomic Transactions: The data is always consistent as all commands within a pipeline are carried out atomically.
Cons of Redis Pipelining
- Complexity: Synchronous Redis interactions may seem trivial as compared to implementing pipelines that are quite complex, especially with error handling.
- Limited Support for Some Commands: Most of the Redis commands are pipelined but certain commands that rely on former outcomes or deals could be avoided.
- Memory Consumption: If several commands are queued and not done quickly, pipelining may use up much memory on the side of clients.
Conclusion
The term Redis Pipelining stands for improving of Redis operations especially when there are several commands that follow each other one after another. With Redis pipelining you can greatly improve application performance by reducing network round- trips and increasing throughput. Nevertheless, it is crucial to evaluate time and place of using it, because there are cases when it may not work properly. In moderation, using Redis Pipelining could provide great benefits when leveraging the power of an ultra-high speed memory based data store
Share your thoughts in the comments
Please Login to comment...