Redis, which stands for “Remote Dictionary Server,” is an open-source, in-memory data store that has become a cornerstone technology in modern application development. Its significance lies in its ability to provide fast, efficient, and versatile data storage and caching solutions.
At its core, Redis is a key-value store that stores data in RAM, which allows for incredibly fast data retrieval and manipulation. This makes it ideal for use cases requiring low-latency access to frequently used data, such as session management, real-time analytics, and caching.
Important Topics for Redis Scripting
Redis offers several key features that contribute to its prominence:
- In-Memory Storage: Redis stores data in RAM, enabling sub-millisecond response times. This makes it ideal for applications that demand high-speed data access.
- Data Structures: Redis supports various data structures like strings, sets, lists, and hashes. This versatility allows developers to model and manipulate data in ways that are natural for their application’s needs.
- Pub/Sub Messaging: Redis provides publish/subscribe messaging capabilities, facilitating real-time communication between components of an application.
- Persistence: Redis can be configured to persist data to disk, ensuring data durability while still benefiting from in-memory performance.
- Clustering: Redis supports clustering, making it horizontally scalable for high availability and fault tolerance in distributed systems.
- Atomic Operations: Redis supports atomic operations, making it an excellent choice for implementing counters, locks, and distributed task queues.
Redis scripting offers two primary benefits:
- Reduced Network Overhead: Traditional Redis operations require multiple round trips between the client and server for complex tasks. Redis scripting, on the other hand, allows developers to bundle commands into a single script. This reduces the number of network requests, minimizing latency and conserving bandwidth, making it ideal for applications where speed and efficiency are highly necessary.
- Improved Performance: By executing code on the server side, Redis scripting uses the server’s computational power and memory, resulting in faster execution of complex tasks. This enhanced performance is especially valuable for real-time analytics, high-speed data processing, and scenarios where rapid response times are crucial, making Redis scripting a powerful tool in optimizing application performance.
Supported Scripting Languages
Redis supports multiple scripting languages, but the primary and most widely used scripting language is Lua. Here’s an overview of scripting languages in Redis:
Lua Scripting:
- Primary Language: Redis’s native scripting language is Lua, a lightweight, fast, and embeddable scripting language.
- Advantages: Lua’s simplicity, speed, and safety make it an ideal choice for Redis scripting. It offers a well-defined API for interacting with Redis data structures, ensuring secure execution.
- Usage: Lua scripts are executed using the `EVAL` or `EVALSHA` commands in Redis. These scripts are atomic and can interact with Redis data, providing tremendous flexibility and control.
Other Supported Languages (Deprecated):
Redis has supported other languages like JavaScript, Ruby, and Python in the past through third-party modules. However, these languages are less common and considered deprecated in favor of Lua due to Lua’s performance and security advantages.
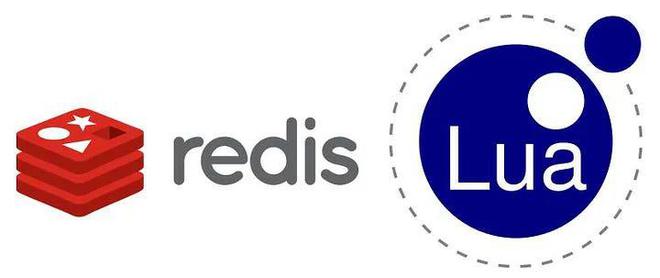
Lua-The primary scripting language for Redis
Loading and Executing Scripts
Loading and executing Redis scripts involves using the SCRIPT LOAD command to load the script into the server and the EVAL or EVALSHA command to execute it.
Here’s a step-by-step guide on how to do this:
- Writing the Redis Script: First, create your Redis script using the Lua scripting language. Ensure that your script contains the necessary logic to perform the desired operations on Redis data.
- Loading the Script: To load the script into Redis, use the SCRIPT LOAD command. This command accepts the script as a parameter and returns a unique SHA-1 hash identifier for the script.
Shell Script:
redis> SCRIPT LOAD “your_lua_script_here”
“1234567890abcdef”
Save the returned SHA-1 hash as it will be used to execute the script.
Executing the Script:
To execute the script, use either the EVAL or EVALSHA command, depending on whether you want to use the script’s hash or the script itself.
Using EVAL (with script source):
redis> EVAL "your_lua_script_here" 0
Using EVALSHA (with script hash):
redis> EVALSHA 1234567890abcdef 0
In both commands, the 0 represents the number of keys the script will access. If your script requires keys, you can specify them after the 0.
Security considerations and best practices for loading scripts in Redis:
- Authentication and Access Control: Secure Redis with strong authentication and limit access to trusted clients.
- Use EVALSHA for Repeated Scripts: Employ `EVALSHA` with script hashes to avoid exposing script source code repeatedly.
- Input Validation: Validate and sanitize inputs to prevent code injection vulnerabilities.
- Script Isolation: Isolate scripts with unique data keys to prevent unintended interactions.
- Rate Limiting: Implement rate limiting to prevent resource exhaustion or denial-of-service attacks.
Redis Scripting Commands
In-depth exploration of key Redis scripting commands, including `EVAL`, `EVALSHA`, and `SCRIPT EXISTS`.
EVAL Command:
The EVAL command is used to evaluate a Lua script on the Redis server.
Syntax:
EVAL script numkeys key [key ...] arg [arg ...]
Parameters:
- script: The Lua script to execute.
- numkeys: The number of keys in the keyspace that the script will access.
- key [key …]: The keys in the keyspace that the script will access.
- arg [arg …]: Arguments passed to the script.
- This command executes the provided Lua script with access to one key (key1) and one argument (val1), returning the result.
EVAL "return {KEYS[1],ARGV[1]}" 1 key1 val1
EVALSHA Command:
The EVALSHA command is similar to EVAL but uses a precomputed SHA-1 hash of the Lua script instead of the script source.
Syntax:
EVALSHA sha1 numkeys key [key ...] arg [arg ...]
Parameters:
- sha1: The SHA-1 hash of the Lua script.
- numkeys, key [key …], and arg [arg …] are the same as in EVAL.
- This command executes a script using its SHA-1 hash, providing the same functionality as EVAL.
EVALSHA "12345..." 1 key1 val1
SCRIPT EXISTS Command:
The SCRIPT EXISTS command checks if scripts with given SHA-1 hashes exist in the script cache.
Syntax:
SCRIPT EXISTS sha1 [sha1 ...]
Parameters:
- sha1 [sha1 …]: One or more SHA-1 hashes of scripts to check.
SCRIPT EXISTS "12345..." "67890..."
- This command checks if scripts with the specified SHA-1 hashes exist in the script cache. It returns an array of integers, with each integer corresponding to a script’s existence status.
Real-world examples of using these commands to solve problems:
Atomic Counter with EVAL:
Suppose you want to implement an atomic counter in Redis. You can use the EVAL command to ensure that the increment operation is atomic.
EVAL "return redis.call('INCRBY', KEYS[1], ARGV[1])" 1 my_counter 5
In this example, the Lua script increments the value stored in the my_counter key by 5.
Caching Complex Computation with EVALSHA:
When you have a complex computation that you want to cache to improve performance, you can use EVALSHA to execute a preloaded script. First, you need to load the script using SCRIPT LOAD, and then you can call it using EVALSHA. For example, let’s say you have a script to calculate Fibonacci numbers:
SCRIPT LOAD "local a, b = 0, 1; for i = 1, tonumber(ARGV[1]) do local tmp = a; a = b; b = tmp + b; end; return a"
Then you can call it with EVALSHA:
EVALSHA <script_sha1> 1 10
This would calculate the 10th Fibonacci number using the cached script.
Checking Existence of a Script with SCRIPT EXISTS:
You can use SCRIPT EXISTS to check whether a specific script exists in the Redis server or not. This can be useful when you want to avoid loading a script multiple times.
SCRIPT EXISTS <script_sha1>
If the script with the given SHA1 hash exists, it will return 1; otherwise, it will return 0.
Using EVAL to Implement Conditional Updates:
You can use EVAL to implement conditional updates. For example, suppose you have a distributed lock implemented with Redis. You can use a Lua script to release the lock only if the current owner matches the requesting client.
local current_owner = redis.call('GET', KEYS[1])
if current_owner == ARGV[1] then
redis.call('DEL', KEYS[1])
end
return current_owner
This script checks if the current owner of the lock (stored in KEYS[1]) matches the requesting client (ARGV[1]). If they match, it releases the lock; otherwise, it returns the current owner.
These are just a few examples of how you can use EVAL, EVALSHA, and SCRIPT EXISTS to solve specific problems in Redis scripting.
Data Access and Manipulation
Method to access and manipulate Redis data within a script.
In Redis, you can access and manipulate data within a Lua script using the redis.call and redis.pcall functions. These functions allow you to interact with Redis commands and data structures. Here’s a demonstration of how to access and manipulate Redis data within a Redis script.
Let’s say you have a Redis list named my_list, and you want to pop an element from the list, increment its value by 1, and then push it back to the list.
Lua script:
-- Define the key of the list
local key = 'my_list'
-- Pop an element from the list
local popped_value = redis.call('LPOP', key)
-- Check if the list was not empty
if popped_value then
-- Convert the popped value to a number
local num = tonumber(popped_value)
-- Increment the value by 1
num = num + 1
-- Push the updated value back to the list
redis.call('RPUSH', key, num)
-- Return the updated value
return num
else
-- Return an error or a sentinel value if the list was empty
return "List is empty"
end
In this Lua script:
- We define the key of the Redis list as my_list.
- We use redis.call(‘LPOP’, key) to pop an element from the list.
- We check if the list was not empty (i.e., popped_value is not nil).
- If the list was not empty, we convert the popped value to a number, increment it by 1, and then push it back to the list using redis.call(‘RPUSH’, key, num).
- Finally, we return the updated value.
You can execute this script using the EVAL command in Redis:
EVAL "<Lua Script Here>" 0
Replace <Lua Script Here> with the Lua script code provided above.
Common Operation of Redis Scripting
Examples of common operations, such as setting values, retrieving data, and modifying keys.
Setting Values:
Set a String Value:
Syntax:
SET my_key "Hello, Redis!"
This command sets the value of the key “my_key” to the string “Hello, Redis!”. In Redis, SET is used to assign a value to a key.
Set an Integer Value:
Syntax:
SET counter 42
This command sets the value of the key “counter” to the integer 42. Redis keys can hold different types of values, including strings, integers, and more.
Set with Expiration (in seconds):
Syntax:
SETex my_key 3600 "This value will expire in 1 hour"
This command is similar to SET, but it also includes a time-to-live (TTL) in seconds. In this case, the key “my_key” is set to the string “This value will expire in 1 hour” and will automatically expire after 3600 seconds (1 hour).
Retrieving Data:
Retrieve a String Value:
Syntax:
GET my_key
This command retrieves the value stored at the key “my_key”. In this example, it would return the string “Hello, Redis!” if the previous SET command has been executed.
Retrieve an Integer Value:
Syntax:
GET counter
This command retrieves the value stored at the key “counter”. In this example, it would return the integer 42 if the previous SET command has been executed.
Modifying Keys:
Increment a Key’s Value (Atomic):
Syntax:
INCR my_counter
This command increments the value stored at the key “my_counter” by 1. If the key doesn’t exist, it’s set to 1.
Decrement a Key’s Value (Atomic):
Syntax:
DECR my_counter
This command decrements the value stored at the key “my_counter” by 1. If the key doesn’t exist, it’s set to -1.
Append to a String Value:
Syntax:
APPEND my_key ", Redis is awesome!"
This command appends the specified string (“, Redis is awesome!”) to the value stored at the key “my_key”.
Rename a Key:
Syntax:
RENAME old_key new_key
This command renames the key “old_key” to “new_key”. It’s used for changing the name of a key.
Delete a Key:
Syntax:
DEL my_key
This command deletes the key “my_key” and its associated value from the Redis database.
Expire a Key (in seconds):
Syntax:
EXPIRE my_key 60
This command sets a time-to-live (TTL) for the key “my_key” to 60 seconds. After 60 seconds, the key will be automatically deleted.
Check if a Key Exists:
Syntax:
EXISTS my_key
This command checks whether the key “my_key” exists in the Redis database. If the key exists, the command returns 1; otherwise, it returns 0.
Hash Data Structure Operations:
Set a Field in a Hash:
Syntax:
HSET user:id123 name "Alice"
This command sets the field “name” in the hash “user:id123” to the value “Alice”. Hashes in Redis are maps between string field and string values.
Retrieve a Field from a Hash:
Syntax:
HGET user:id123 name
This command retrieves the value of the field “name” in the hash “user:id123”. In this example, it would return “Alice”.
Increment a Field Value in a Hash (Atomic):
Syntax:
HINCRBY user:id123 age 1
This command increments the value of the field “age” in the hash “user:id123” by 1. If the field doesn’t exist, it’s set to 1.
Get All Fields and Values from a Hash:
Syntax:
HGETALL user:id123
This command retrieves all fields and values in the hash “user:id123”.
List Data Structure Operations:
Push an Element to the Head of a List:
LPUSH my_list "item1"
This command pushes the value “item1” to the left end of the list “my_list”.
Push an Element to the Tail of a List:
RPUSH my_list "item2"
This command pushes the value “item2” to the right end of the list “my_list”.
Pop an Element from the Head of a List:
LPOP my_list
This command removes and returns the leftmost item from the list “my_list”.
Retrieve a Range of Elements from a List:
LRANGE my_list 0 -1
This command returns all elements of the list “my_list” from index 0 to the last index (-1). It effectively retrieves all items in the list.
Error Handling:
Error handling in Redis scripting with Lua is important to ensure the robustness and reliability of your Redis operations. Redis provides several mechanisms for handling errors within scripts:
Error Return Values:
Most Redis commands in Lua scripts return a special error value when something goes wrong. For example, if you try to access a non-existent key, the redis.call function will return nil.
You can check for error conditions by explicitly comparing the return value with nil. For instance:
Lua script:
local value = redis.call('GET', 'non_existent_key')
if value == nil then
return "Key not found"
end
Error Messages:
You can use error(“message”) in your Lua script to throw an error with a custom error message.
Redis will capture this error message and return it as part of the script’s result. You can access it using the pcall function when calling the script.
Lua script:
if some_condition then
error("Something went wrong")
end
Error Handling with pcall:
You can use the pcall function in Redis to execute a Lua script and capture any errors that occur during execution.
local success,
result = pcall(function()
-- Your Lua script here end)
if not success then
return "Script error: " .. result
end
Error Handling with assert:
The assert function can be used to check for conditions and throw an error if the condition is not met.
Lua script:
local result = redis.call('GET', 'some_key')
assert(result, "Key not found")
Transaction Rollback:
In a Redis transaction (MULTI and EXEC), if an error occurs during the execution of the transaction, the entire transaction is rolled back, and no changes are applied.
This ensures that Redis operations within a transaction are atomic, and either all succeed or none do.
Error Logging:
Redis logs errors and exceptions related to Lua script execution. These logs can be useful for debugging and monitoring script behavior.
Atomic Transactions
Redis scripting, specifically through the use of the MULTI, EXEC, and WATCH commands in conjunction with Lua scripts, enables atomic transactions. This mechanism ensures that a series of Redis commands are executed atomically, meaning they are either all executed together or none at all. Here’s how Redis scripting achieves atomic transactions:
MULTI and EXEC Commands:
- Redis provides a way to group multiple commands into a transaction using the MULTI and EXEC commands.
- When you send a MULTI command, Redis enters into a transaction mode, where subsequent commands are not immediately executed but are queued for execution.
- When you send the EXEC command, Redis executes all the commands queued within the transaction atomically.
Lua Script Execution:
- Instead of executing individual commands within a transaction, you can also use a Lua script to perform a sequence of Redis operations atomically.
- You can send the Lua script to Redis using the EVAL or EVALSHA command. Redis executes the entire script atomically, without interleaving other client commands.
Isolation and Atomicity:
During the execution of a Redis transaction or Lua script, Redis ensures isolation from other client commands. No other commands from different clients can execute in the middle of a transaction. If any error occurs within a transaction or script, Redis aborts the entire transaction, and no changes are applied to the data.
WATCH for Optimistic Locking:
Redis also provides the WATCH command, which allows you to implement optimistic locking within a transaction. By using WATCH, you can monitor one or more keys. If any of the watched keys are modified by another client before your transaction is executed, Redis will abort your transaction.
Here’s an example of how Redis scripting enables atomic transactions:
Lua script:
In this example, the Lua script ensures that the transfer of funds between two accounts (from_account and to_account) happens atomically. If the balance in the “from_account” is sufficient, the transaction succeeds; otherwise, it fails. Regardless of the outcome, the entire script executes as a single, atomic operation.
-- Lua script to transfer funds between two accounts atomically
local from_account = 'account:123'
local to_account = 'account:456'
local amount = 50
-- Check if the "from_account" has enough balance
local from_balance = tonumber(redis.call('GET', from_account))
if from_balance >= amount then
-- Deduct the amount from "from_account"
redis.call('DECRBY', from_account, amount)
-- Add the amount to "to_account"
redis.call('INCRBY', to_account, amount)
-- Transaction is successful
return "Transaction successful
else
-- Insufficient balance, transaction fails
return "Insufficient balance"
end
Use cases and practical examples of maintaining data consistency with scripts:
Atomic Counters:
- Use case: Keeping track of counts or statistics that need to be updated concurrently.
- Example: Incrementing a view counter for a web page.
local key = 'page_views:123'
redis.call('INCR', key)
Distributed Locks:
- Use case: Ensuring exclusive access to a shared resource among multiple clients.
- Example: Implementing a distributed lock to prevent concurrent access to a critical section of code or data.
local lockKey = 'resource_lock'
local acquired = redis.call('SET', lockKey, 'LOCKED', 'NX', 'EX', 10)
if acquired then
-- Execute critical section
-- Release the lock when done
redis.call('DEL', lockKey)
else
return "Resource is locked"
end
Unique IDs Generation:
- Use case: Generating unique identifiers without duplication in a distributed system.
- Example: Generating unique order IDs.
local uniqueID = redis.call('INCR', 'next_order_id')
Conditional Updates:
- Use case: Modifying data only if certain conditions are met.
- Example: Updating a user’s email address if the provided verification code matches.
local verificationCodeKey = 'user:123:verification_code'
local emailKey = 'user:123:email'
local providedCode = ARGV[1]
if redis.call('GET', verificationCodeKey) == providedCode then
redis.call('SET', emailKey, ARGV[2])
return "Email updated successfully"
else
return "Verification code is invalid"
end
Rate Limiting:
- Use case: Enforcing rate limits to prevent excessive requests or abuse.
- Example: Implementing a simple rate limiter for an API endpoint.
local userKey = 'user:123:request_count'
local limit = 100
local currentCount = tonumber(redis.call('INCR', userKey))
if currentCount > limit then
return "Rate limit exceeded"
else
return "Request accepted"
end
Conditional Data Deletion:
- Use case: Deleting data based on certain conditions.
- Example: Deleting expired sessions or cache entries.
local sessionKey = 'session:123'
local expirationTime = tonumber(redis.call('GET', sessionKey .. ':expiration'))
if expirationTime and expirationTime < os.time() then
redis.call('DEL', sessionKey)
return "Session deleted"
else
return "Session still valid"
end
Scripting in a Distributed Environment
- When employing Redis scripting in a distributed or clustered Redis setup, there are crucial considerations to ensure efficient and reliable script execution. Redis Cluster uses hash slots to distribute data across nodes, and keys must be located within the same hash slot to work properly. Use the `EVAL` or `EVALSHA` command to execute scripts, allowing Redis to route them correctly based on key distribution.
- Handle failures gracefully, as network partitions or node outages can occur in such setups. Ensure scripts are resilient and can manage scenarios where certain nodes become unavailable. It’s essential to test your scripts in an environment that closely resembles your production setup to catch any unexpected issues.
- Additionally, be cautious with certain Redis commands in clustered environments. For example, `SORT` with `BY` clauses may not behave as expected if keys are distributed across multiple slots.
- Monitoring and observability tools like Redis Sentinel and Redis Cluster Manager can help track script performance and identify bottlenecks. Finally, consult Redis documentation, which offers specific guidance and best practices for scripting in Redis Cluster and Sentinel setups. By following these guidelines, you can harness the power of Redis scripting while maintaining data consistency and reliability in distributed environments.
Considerations for data sharding and consistency in a distributed system
- Sharding Strategy: Choose an appropriate sharding method, such as range-based or hash-based, aligning with your specific use case.
- Data Distribution: Equally distribute data across shards to prevent hotspots, employing efficient hash functions or distribution techniques.
- Replication: Ensure data redundancy within each shard to enhance fault tolerance and high availability, replicating data across multiple nodes or data centers.
- Consistency Models: Decide on the desired consistency model, whether strong or eventual, aligning it with your application’s requirements.
- Conflict Resolution: Define strategies for resolving conflicts, utilizing timestamps, vector clocks, or application-specific logic.
- Scaling and Load Balancing: Prepare for horizontal scaling and implement load balancing mechanisms for even request distribution.
Scaling Redis Scripting
To scale Redis scripting as your app grows:
- Horizontal Scaling: Add more Redis nodes or clusters.
- Caching: Use Redis for read-heavy tasks.
- Clustering: Implement Redis Cluster for high availability.
- Optimize Lua Scripts: Continuously optimize scripts for efficiency.
- Monitoring: Use metrics for insights.
Share your thoughts in the comments
Please Login to comment...