C# | RichTextBox Class
Last Updated :
05 Sep, 2019
In C#, RichTextBox control is a textbox which gives you rich text editing controls and advanced formatting features also includes a loading rich text format (RTF) files. Or in other words, RichTextBox controls allows you to display or edit flow content, including paragraphs, images, tables, etc. The RichTextBox class is used to represent the windows rich text box and also provide different types of properties, methods, and events. It is defined under System.Windows.Forms namespace.
It does not have the same 64K character capacity limit like TextBox control. It is used to provide text manipulation and display features similar to word processing applications like Microsoft Word. In C# you can create a RichTextBox in the windows form by using two different ways:
1. Design-Time: It is the easiest way to create a RichTextBox as shown in the following steps:
- Step 1: Create a windows form as shown in the below image:
Visual Studio -> File -> New -> Project -> WindowsFormApp
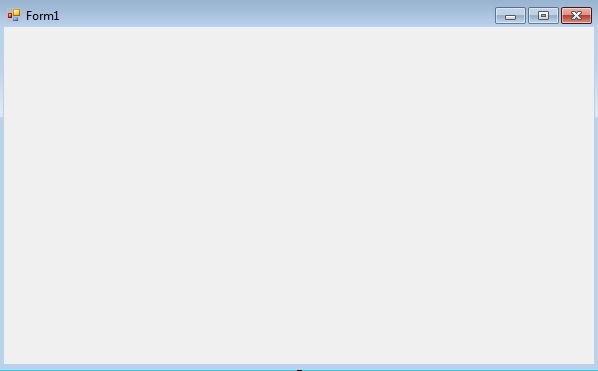
- Step 2: Next, drag and drop the RichTextBox control from the toolbox to the form.
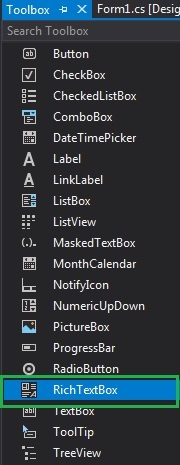
- Step 3: After drag and drop you will go to the properties of the RichTextBox control to modify RichTextBox according to your requirement.
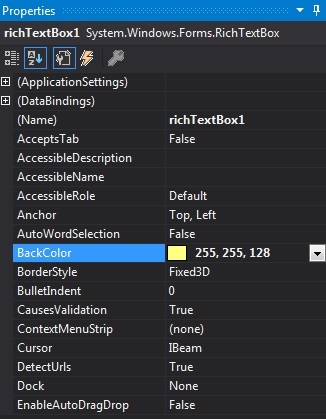
Output:
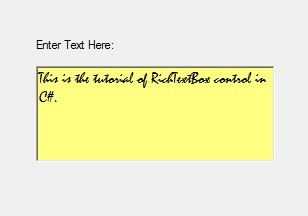
2. Run-Time: It is a little bit trickier than the above method. In this method, you can create a RichTextBox control programmatically with the help of syntax provided by the RichTextBox class. The following steps show how to set the create RichTextBox dynamically:
- Step 1: Create a RichTextBox control using the RichTextBox() constructor is provided by the RichTextBox class.
// Creating a RichTextBox control
RichTextBox box = new RichTextBox();
- Step 2: After creating a RichTextBox control, set the property of the RichTextBox control provided by the RichTextBox class.
// Setting the location
// of the RichTextBox
box.Location = new Point(236, 97);
// Setting the background
// color of the RichTextBox
box.BackColor = Color.Aqua;
// Setting the text
// in the RichTextBox
box.Text = "!..Welcome to GeeksforGeeks..!";
- Step 3: And last add this RichTextBox control to the form using the following statement:
// Adding this RichTextBox
// in the form
this.Controls.Add(box);
Example:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp30 {
public partial class Form1 : Form {
public Form1()
{
InitializeComponent();
}
private void Form1_Load( object sender, EventArgs e)
{
Label lb = new Label();
lb.Location = new Point(251, 70);
lb.Text = "Enter Text" ;
this .Controls.Add(lb);
RichTextBox box = new RichTextBox();
box.Location = new Point(236, 97);
box.BackColor = Color.Aqua;
box.Text = "!..Welcome to GeeksforGeeks..!" ;
this .Controls.Add(box);
}
}
}
|
Output:
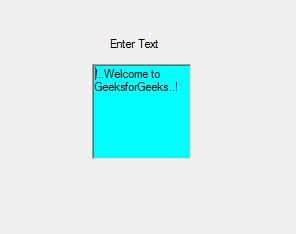
Constructor
Constructor |
Description |
RichTextBox() |
This Constructors is used to initialize a new instance of the RichTextBox class. |
Properties
Property |
Description |
AutoSize |
This property is used to get or set a value that indicates whether the control resizes based on its contents. |
BackColor |
This property is used to get or set the background color for the control. |
BorderStyle |
This property indicates the border style for the control. |
Font |
This property is used to get or set the font of the text displayed by the control. |
ForeColor |
This property is used to get or set the foreground color of the control. |
Height |
This property is used to get or set the height of the control. |
Location |
This property is used to get or set the coordinates of the upper-left corner of the RichTextBox control relative to the upper-left corner of its form. |
Name |
This property is used to get or set the name of the control. |
TabStop |
This property is used to get or set a value that shows whether the user can press the TAB key to provide the focus to the NumericUpDown. |
Size |
This property is used to get or set the height and width of the control. |
Text |
This property is used to get or set the text to be displayed in the RichTextBox control. |
Visible |
This property is used to get or set a value indicating whether the control and all its child controls are displayed. |
Width |
This property is used to get or set the width of the control. |
ZoomFactor |
This property is used to get or set the current zoom level of the RichTextBox. |
ShowSelectionMargin |
This property is used to get or set a value indicating whether a selection margin is displayed in the RichTextBox. |
SelectionTabs |
This property is used to get or set the absolute tab stop positions in a RichTextBox control. |
SelectedText |
This property is used to get or set the selected text within the RichTextBox. |
ScrollBars |
This property is used to get or set the type of scroll bars to display in the RichTextBox control. |
Multiline |
This property is used to get or set a value indicating whether this is a multiline RichTextBox control. |
Share your thoughts in the comments
Please Login to comment...