Building an OTP Verification System with Node.js and MongoDB
Last Updated :
24 Apr, 2024
In the present digital world, Securing your website or internet could be very crucial. One manner to increase protection is by using One Time Password (OTP) for the verification system. This will help you to steady your software and defend your website from unauthorized get entry. This article will help you create an OTP verification device, which you can use in your upcoming initiatives. This venture was created using Nodejs and MongoDB, which is a completely famous technology in net improvement.
What’s OTP Verification?
OTP verification adds an extra layer of security to your application. When you log in or perform sensitive actions online this will help you to not allow anyone without your permission. Instead of using the same password whenever you get hold of a completely unique code for your cellphone or e-mail that you need to go into to verify your identity. This code is legitimate simplest to use and expires after a short time which makes it very steady.
This article will help you to study the technology and make the project from scratch. We will start from the Tech stack and then move to the project code for your better understanding.
Why Node.js and MongoDB?
Node.Js is a powerful JavaScript runtime that builds rapid and scalable net programs. MongoDB is a versatile and clean-to-use NoSQL database that stores our information in a JSON-like layout. Together, they make a terrific aggregate for building dynamic and efficient systems.
- Node.js: It is a JavaScript runtime environment constructed on Chrome version v8 JavaScript engine. It lets you run Javascript code on the server. In this utility, we use Node.js to create the backend server that handles requests from clients and generates OTPs.
- Express.js: Express.js is an internet utility framework for Node.js. It gives a set of capabilities for building internet packages and APIs, including routing, middleware support, and verifying OTP.
- MongoDB: MongoDB is a NoSQL database that shops records in a bendy, JSON-like format. It is used because the database for storing OTP and associated data on this software. MongoDB’s flexibility makes it suitable for storing unstructured or semi-structured information like OTPs.
- Mongoose: It is an Object Data Modeling(ODM) library for MongoDB and Node.js. It provides a straightforward schema-based solution for modeling utility information and interacting with MongoDB. In this application, we use Mongoose to define schemas and models for OTPs, making it less difficult to work with MongoDB.
- OTP-generator: OTP-generator is a library used for producing one-time passwords(OTPs). It allows us to create random OTPs of a specific length and layout. We use this library to generate particular OTPs for every verification try.
- Nodemailer: nodemailer is a module for sending emails from Node.Js packages. It supports diverse e-mail offerings and provides a smooth-to-use interface for sending emails programmatically. In this application, we use nodemailer to ship the generated OTPs to users through email for verification.
- CORS (Cross-Origin Resource Sharing): CORS is a security function implemented via web browsers to restrict pass-beginning HTTP requests. In this application, we use the CORS middleware to allow requests from distinctive origins to access our server’s resources. This is important because the frontend software may be served from a distinctive area or port than the backend server, and with out CORS, the browser could block such requests due to protection restrictions.
Project Structure and Dependency:
Here is the project structure which you have to follow to make otp verification system. You can also use another front-end frameworks to give a nice look, But for simplicity I am using HTML page.
.jpg)
Directory Structure
Here is the dependencies and their versions, you can refer them and learn. If error occur first check the version of these then try other things.
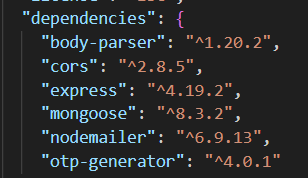
Dependencies
Steps to Create OTP Verification System
Step 1: Setting Up the Project
Create a new directory for your project and navigate into it:
mkdir otp-verification
cd otp-verification
Initialize a new Node.js project:
npm init -y
Install the required dependencies:
npm install express mongoose body-parser otp-generator nodemailer cors
Step 2: Creating the Server
Create a file name it as server.js and add the following code:
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>OTP Verification</title>
<style>
body {
font-family: Arial, sans-serif;
}
.container {
max-width: 400px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
input[type="text"], input[type="password"], button {
width: 100%;
padding: 10px;
margin-bottom: 10px;
box-sizing: border-box;
}
button {
background-color: #007bff;
color: #fff;
border: none;
cursor: pointer;
}
button:hover {
background-color: #0056b3;
}
.message {
margin-top: 10px;
text-align: center;
color: red;
}
</style>
</head>
<body>
<div class="container">
<h2>OTP Verification</h2>
<input type="email" id="email" placeholder="Enter your email">
<button onclick="generateOTP()">Generate OTP</button>
<input type="text" id="otp" placeholder="Enter OTP">
<button onclick="verifyOTP()">Verify OTP</button>
<p class="message" id="response"></p>
</div>
<script>
async function generateOTP() {
const email = document.getElementById('email').value;
const response = await fetch('http://localhost:3000/generate-otp', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ email })
});
const result = await response.text();
document.getElementById('response').innerText = result;
}
async function verifyOTP() {
const email = document.getElementById('email').value;
const otp = document.getElementById('otp').value;
const response = await fetch('http://localhost:3000/verify-otp', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ email, otp })
});
const result = await response.text();
document.getElementById('response').innerText = result;
}
</script>
</body>
</html>
JavaScript
// server.js
const express = require('express');
const bodyParser = require('body-parser');
const mongoose = require('mongoose');
const otpGenerator = require('otp-generator');
const nodemailer = require('nodemailer');
const cors = require('cors');
const app = express();
const PORT = process.env.PORT || 3000;
app.use(bodyParser.json());
app.use(cors());
// MongoDB connection
mongoose.connect('mongodb+srv://<username>:<password>@cluster0.d6t5od6.mongodb.net/', { useNewUrlParser: true, useUnifiedTopology: true });
const db = mongoose.connection;
db.on('error', (err) => {
console.error('MongoDB connection error:', err);
});
db.once('open', () => {
console.log('Connected to MongoDB');
});
// Define schema and model for OTP
const otpSchema = new mongoose.Schema({
email: String,
otp: String,
createdAt: { type: Date, expires: '5m', default: Date.now }
});
const OTP = mongoose.model('OTP', otpSchema);
// Generate OTP and send email
app.post('/generate-otp', async (req, res) => {
const { email } = req.body;
const otp = otpGenerator.generate(6, { digits: true, alphabets: false, upperCase: false, specialChars: false });
try {
await OTP.create({ email, otp });
// Send OTP via email (replace with your email sending logic)
const transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: 'your-mail@gmail.com',
pass: 'your-app-password'
}
});
await transporter.sendMail({
from: 'your-mail@gmail.com',
to: email,
subject: 'OTP Verification',
text: `Your OTP for verification is: ${otp}`
});
res.status(200).send('OTP sent successfully');
} catch (error) {
console.error(error);
res.status(500).send('Error sending OTP');
}
});
// Verify OTP
app.post('/verify-otp', async (req, res) => {
const { email, otp } = req.body;
try {
const otpRecord = await OTP.findOne({ email, otp }).exec();
if (otpRecord) {
res.status(200).send('OTP verified successfully');
} else {
res.status(400).send('Invalid OTP');
}
} catch (error) {
console.error(error);
res.status(500).send('Error verifying OTP');
}
});
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Step 3: Running the Server
Run the server using the following command:
node server.js
Step 4: Testing the Endpoints
You can now test the OTP generation and verification endpoints using a tool like Postman. You can also try it using the start server of HTML or by making HTTP requests from your frontend application.
- To generate an OTP you have to send a POST. Request should be send to http://localhost:3000/generate-otp with the email. Email should attached in the requested body.
- To verify an OTP you have to send a POST. Request should be send to http://localhost:3000/verify-otp with the email and OTP. Email and OTP is also attached with the requested body.
That’s it! You’ve successfully built a basic OTP verification system using Node.js and MongoDB. You can further enhance this system by adding error handling, validation, and additional security measures.
Output-
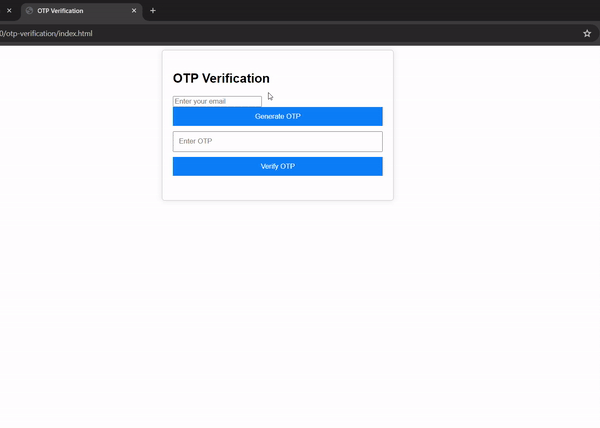
Conclusion
In conclusion, building an OTP verification gadget with Node.Js and MongoDB adds a essential layer of safety to web programs. By requiring customers to verify their identity with a completely unique, one-time code, we are able to substantially reduce the hazard of unauthorized access and protect sensitive consumer information. With the technology used in this utility, including Node.Js, Express.Js, MongoDB, otp-generator, and nodemailer. Now your Otp verification system is ready to use. You can also add this feature to your application for securing your application. This application will help you to add security to you upcoming application. It also ensure that your application will not affected by other users/middle-man.
FAQs
Why is OTP verification important for web applications?
OTP verification provides an additional layer of security to net packages by requiring customers to provide a unique, one-time code to confirm their identity. This allows save you unauthorized get admission to and protects sensitive consumer information from being compromised.
How does the OTP generation process work in this application?
In this application, OTPs are generated using the otp-generator library in Node.Js. When a consumer requests OTP verification, a random code is generated and stored inside the database along with the person’s e-mail or smartphone wide variety. The code is then despatched to the person thru e-mail the usage of the nodemailer module for verification.
What measures are taken to ensure the security of OTPs in transit and storage?
OTPs are securely generated the use of a random code generator and stored within the database with a brief expiration time to reduce the risk of unauthorized get entry to. Additionally, the OTPs are despatched to users through e mail using encrypted verbal exchange channels to shield them from interception throughout transit. The backend server is likewise covered from unauthorized access thru measures consisting of CORS policy implementation and right authentication.
Share your thoughts in the comments
Please Login to comment...