Architecture for Mobile Development | Design Patterns
Last Updated :
14 Dec, 2023
Hey there, tech enthusiasts! Ever wonder what makes your favorite mobile apps so smooth and user-friendly? Well, the secret lies in their architecture – the behind-the-scenes structure that turns code into the awesome apps we can’t live without.
.jpg)
Important Topics for Mobile Development Architecture
Imagine you’re building your dream house. You wouldn’t just start putting things together randomly, right? You’d need a smart plan, like a blueprint, to make sure everything fits and works well. Well, the same goes for making apps, especially the ones you love on your phone.
In the big world of making apps, it’s a bit like building a house people want to live in. And right in the middle of all this is the plan – the fancy term for it is architecture. It’s like the secret recipe that turns your idea into a smooth and working app.
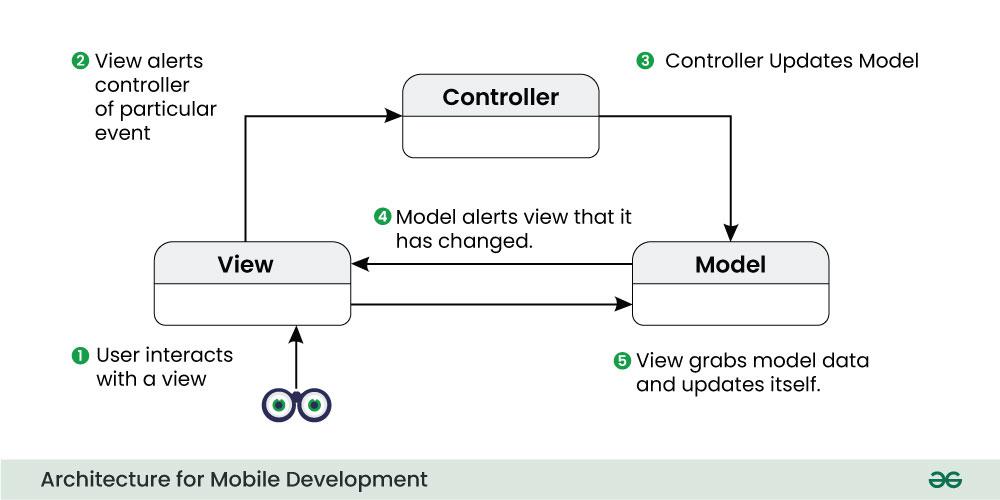
Classic MVC, or Model-View-Controller, is an architectural pattern used in software development to organize the code of an application in a structured way. Let’s break down each part of MVC:
- Model:
- Think of the Model as the brain of your application. It manages the data and the rules for how the data can be changed. For example, if you’re building a to-do list app, the Model would handle tasks, deadlines, and any other information related to the to-do items.
- View:
- The View is like the face of your app. It’s what the user sees and interacts with. In our to-do list example, the View would be the part of the app where you see your list of tasks, checkboxes, and maybe a button to add new tasks.
- Controller:
- The Controller is the middleman between the Model and the View. It takes input from the user (like clicking a button to add a new task), updates the Model based on that input, and then tells the View to refresh and show the updated information. In our to-do list, the Controller would handle things like adding or deleting tasks.
Example:
Suppose you have a basic weather app.
- Model: This would handle the weather data, like the current temperature, forecast, and other related information.
- View: The View would be what the user sees on the screen – maybe a simple interface showing the current temperature and a forecast.
- Controller: The Controller would handle user interactions. If a user wants to switch from viewing the current temperature to the forecast, the Controller takes that request, updates the Model (gets the forecast data), and tells the View to display the new information.
When to use Classic MVC Architecture
Use Classic MVC when your app is not too big or too small – it’s like the Goldilocks choice for moderate-sized projects. If you want a clear separation between how data is handled (Model), how it’s shown to the user (View), and how the user interacts with it (Controller), then Classic MVC is a good fit. It’s a balanced approach that works well for many different types of applications.
Apple’s MVC Architecture
Apple’s MVC (Model-View-Controller) architecture is a specific implementation of the classic MVC pattern tailored for iOS app development. Let’s break down Apple’s MVC in simple terms:
- Model:
- Just like in the classic MVC, the Model in Apple’s MVC is responsible for managing the data and business logic of your app. For example, in a note-taking app, the Model would handle tasks such as saving and retrieving notes.
- View:
- The View is what the user interacts with on the screen. In an iOS app, this could be a button, a label, or any other interface element that users can see and touch. In the note-taking app, the View would be where you read and edit your notes.
- Controller:
- The Controller acts as a coordinator between the Model and the View. It takes user input from the View, updates the Model accordingly, and manages the communication between the two. In the note-taking app, the Controller would handle actions like creating a new note or deleting an existing one.
Example:
Imagine you’re building a basic calculator app.
- Model: The Model would handle the numbers and operations. If you press the buttons ‘2’ and ‘3’, the Model keeps track of these numbers and knows that the next operation is to add or multiply, for example.
- View: The View is what the user sees – the buttons for numbers and operations, and a display area showing the current calculation.
- Controller: The Controller responds to user actions. If the user taps the ‘5’ button, the Controller takes that input, updates the Model, and tells the View to refresh and show the updated calculation.
When to use Apple’s MVC Architecture
- Use Apple’s MVC when you’re developing an iOS app. It’s the default architecture recommended by Apple for building apps on their platform.
- If you’re comfortable working within the iOS development ecosystem and want a structure that aligns with Apple’s guidelines, Apple’s MVC is a straightforward and effective choice.
- It works well for a wide range of iOS applications, from simple utilities to more complex applications.
MVMM (Model-View-View-Model) Architecture
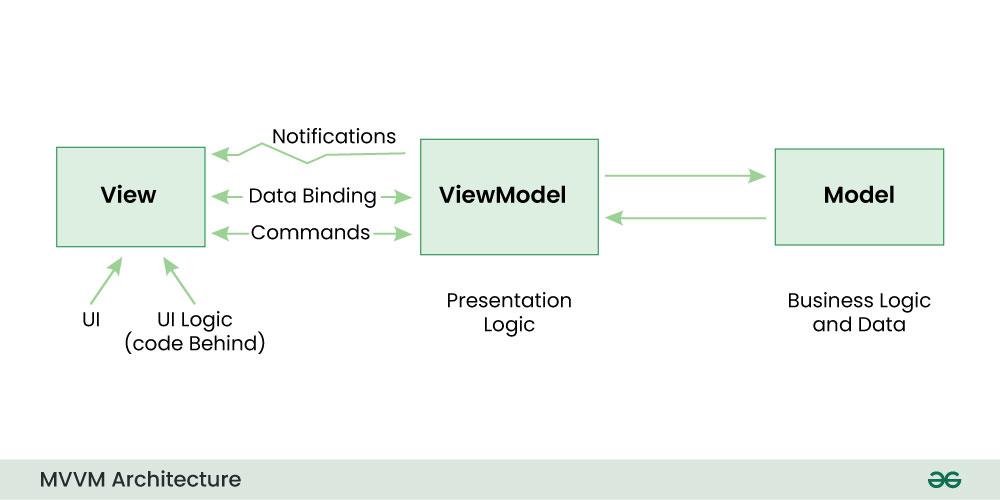
MVVM, or Model-View-View Model, is an architectural pattern commonly used in software development, especially for building user interfaces. Let’s break it down:
- Model:
- The Model is responsible for managing the data and the business logic of your application. If you’re building a weather app, for example, the Model would handle things like fetching weather data from the internet and storing it.
- View:
- The View is what the user sees on the screen – buttons, text, images, etc. In a weather app, the View would be the part of the app where you see the current temperature, weather conditions, and a forecast.
- View-Model:
- The View-Model is like a bridge between the Model and the View. It takes data from the Model and prepares it in a way that the View can easily display. It also handles user interactions and updates the Model accordingly. In the weather app, the View Model would take the raw weather data from the Model, format it, and then provide it to the View for display.
Example:
Imagine you are building a basic news app.
- Model: The Model would handle news articles – fetching them from an online source, storing them, and managing the data.
- View: The View is what the user interacts with – a screen displaying a list of news articles and maybe a button to read more details about a specific article.
- View-Model: The View-Model takes care of preparing the news data for display. It takes the raw data from the Model, formats it into a suitable form for the View, and handles interactions like tapping on an article to read more.
When to use MVVM Architecture
Use MVVM when you want a structured way to organize your code and keep a clear separation between how your data is managed (Model), how it’s presented to the user (View), and the logic that connects the two (View-Model). MVVM is particularly effective when used with data-binding frameworks, as it simplifies the synchronization between the UI and the underlying data. It’s a good choice for projects with complex user interfaces or applications where data presentation and manipulation are crucial.
VIPER (View Interactor Presenter Entity Router) Architecture
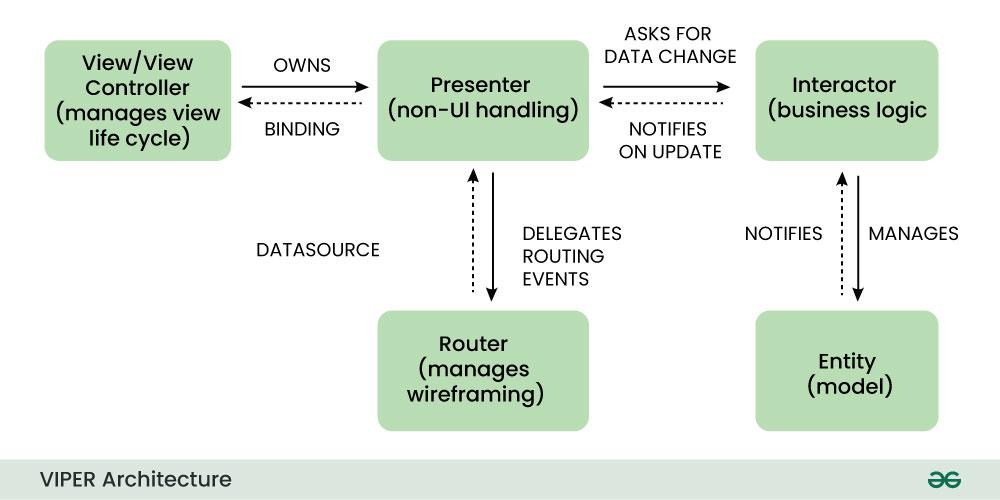
VIPER is an architectural pattern used in software development, especially in mobile app development, to organize code in a modular and scalable way. Let’s break down:
- View:
- The View is what the user sees on the screen – buttons, text, images, etc. In a messaging app, for instance, the View would be the part of the app where you see your chat interface.
- Interactor:
- The Interactor contains the business logic of your application. It’s responsible for handling data and performing operations. In a messaging app, the Interactor would manage tasks like sending and receiving messages.
- Presenter:
- The Presenter acts as a mediator between the View and the Interactor. It handles user input from the View, communicates with the Interactor to perform actions, and then updates the View with the results. In the messaging app, the Presenter would manage things like handling a user’s tap on a message to display more details.
- Entity:
- The Entity represents the data objects used by the Interactor. In the messaging app, an Entity could be the structure that holds information about a message, such as the sender, timestamp, and content.
- Router:
- The Router manages navigation between different modules (screens or features) of your app. It decides which screen to show based on user actions. In the messaging app, the Router could handle navigating from the chat screen to the settings screen.
Example
Imagine you are building a basic social media app.
- View: The View would be the part of the app where users see their feed – posts, comments, and likes.
- Interactor: The Interactor would handle the logic for loading posts, managing user interactions (like adding a comment), and interacting with the app’s backend to fetch or update data.
- Presenter: The Presenter would take user input from the View (like tapping on a post), communicate with the Interactor to perform the necessary actions (like fetching detailed information about the post), and then update the View to show the results.
- Entity: The Entity would be the data structure representing a post – with fields like the post content, author, and timestamp.
- Router: The Router would handle navigation between screens, for instance, moving from the feed to the user’s profile page.
When to use VIPER Architecture
- Use VIPER when you are working on a large and complex project, especially if you want to keep your code modular and easily maintainable.
- VIPER is suitable for applications with multiple features that need to be developed and modified independently.
- It’s a good choice when you have a team of developers working on different parts of the app or when scalability and maintainability are top priorities.
Conclusion
Choosing the right architecture for your mobile app is a critical decision that can significantly impact the development process and the long-term maintainability of your application. Each architecture has its strengths and weaknesses, and the best choice depends on the specific requirements of your project.
For smaller applications or when developing for a specific platform, Classic MVC, Apple’s MVC, or Extended MVC for Android might be sufficient. If testability and a clear separation of concerns are priorities, MVP could be a good fit. MVVM, with its data-binding capabilities, is well-suited for projects with complex UI logic. For large and scalable projects, especially those involving collaborative development, VIPER provides a robust and modular structure.
Ultimately, the key is to understand the specific needs of your project and choose an architecture that aligns with those needs while considering factors such as scalability, maintainability, and testability.
Share your thoughts in the comments
Please Login to comment...