Angular PrimeNG Table Cell Editing
Last Updated :
26 Sep, 2022
Angular PrimeNG is an open-source library that consists of native Angular UI components that are used for great styling and this framework is used to make responsive websites with very much ease. In this article, we will see Angular PrimeNG Table Cell Editing.
The Table Component is used to show some data to the user in the tabular form. Cell editing in a table can be enabled by adding the pEditableColumn directive to a cell that has a p-cellEditor component with input-output templates.
Syntax:
<p-table [value]="books" responsiveLayout="scroll">
<ng-template pTemplate="header">
<tr>
<th>Name</th>
...
</tr>
</ng-template>
<ng-template pTemplate="body"
let-book
let-rowData
let-x="rowIndex">
<tr>
<td pEditableColumn>
<p-cellEditor>
<ng-template pTemplate="input">
<input
pInputText
type="text"
[(ngModel)]="rowData.name">
</ng-template>
<ng-template pTemplate="output">
{{rowData.name}}
</ng-template>
</p-cellEditor>
</td>
...
</tr>
</ng-template>
</p-table>
Creating Angular application and Installing the Modules:
Step 1: Create an Angular application using the following command.
ng new myapp
Step 2: After creating your project folder i.e. myapp, move to it using the following command.
cd myapp
Step 3: Install PrimeNG in your given directory.
npm install primeng --save
npm install primeicons --save
Project Structure: After completing the above steps the project structure will look like the following.
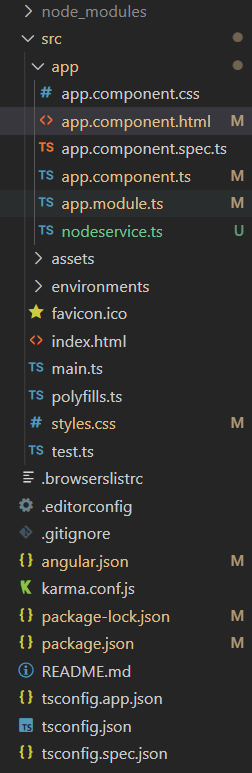
Project Structure
Run the Application:
ng serve --open
Example 1: This example shows how to enable cell editing in a simple table.
app.component.html
< div style = "text-align: center" >
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h4 >Angular PrimeNG Table Cell Editing</ h4 >
< p-table [value]="books" responsiveLayout = "scroll" >
< ng-template pTemplate = "header" >
< tr >
< th >Name</ th >
< th >Author</ th >
< th >Year</ th >
</ tr >
</ ng-template >
< ng-template pTemplate = "body" let-book let-rowData>
< tr >
< td pEditableColumn>
< p-cellEditor >
< ng-template pTemplate = "input" >
< input
pInputText
type = "text"
[(ngModel)]="rowData.name">
</ ng-template >
< ng-template pTemplate = "output" >
{{rowData.name}}
</ ng-template >
</ p-cellEditor >
</ td >
< td pEditableColumn>
< p-cellEditor >
< ng-template pTemplate = "input" >
< input
pInputText
type = "text"
[(ngModel)]="rowData.author">
</ ng-template >
< ng-template pTemplate = "output" >
{{rowData.author}}
</ ng-template >
</ p-cellEditor >
</ td >
< td pEditableColumn>
< p-cellEditor >
< ng-template pTemplate = "input" >
< input
pInputText
type = "number"
[(ngModel)]="rowData.year">
</ ng-template >
< ng-template pTemplate = "output" >
{{rowData.year}}
</ ng-template >
</ p-cellEditor >
</ td >
</ tr >
</ ng-template >
</ p-table >
</ div >
|
app.component.ts
import { Component } from '@angular/core' ;
interface Book {
name: String,
author: String,
year: Number
}
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
})
export class AppComponent {
books: Book[] = [];
ngOnInit() {
this .books = [
{
name: "Clean Code" ,
author: "Robert Cecil Martin" ,
year: 2008
},
{
name: "Introduction to Algorithms" ,
author: "Thomas H Corman" ,
year: 1989
},
{
name: "Refactoring" ,
author: "Martin Fowler" ,
year: 1999
},
{
name: "Code Complete" ,
author: "Steve McConnell" ,
year: 1993
},
{
name: "Programming Pearls" ,
author: "John Bentley" ,
year: 1986
},
{
name: "The Clean Coder" ,
author: "Robert Cecil Martin" ,
year: 2011
},
{
name: "Coders at Work" ,
author: "Peter Seibel" ,
year: 2009
},
{
name: "Effective Java" ,
author: "Joshua Bloch" ,
year: 2001
},
{
name: "Head First Java" ,
author: "Bert Bates" ,
year: 2003
}
];
}
}
|
app.module.ts
import { NgModule } from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { BrowserAnimationsModule }
from '@angular/platform-browser/animations' ;
import { AppComponent } from './app.component' ;
import { TableModule } from 'primeng/table' ;
import { FormsModule } from '@angular/forms' ;
import { InputTextModule } from 'primeng/inputtext' ;
@NgModule({
imports: [
BrowserModule,
BrowserAnimationsModule,
TableModule,
InputTextModule,
FormsModule
],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule { }
|
Output:
Example 2: In this example, we enabled cell editing in a striped table.
app.component.html
< div style = "text-align: center" >
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h4 >Angular PrimeNG Table Cell Editing</ h4 >
< p-table [value]="books" responsiveLayout = "scroll" >
< ng-template pTemplate = "header" >
< tr >
< th >Name</ th >
< th >Author</ th >
< th >Year</ th >
</ tr >
</ ng-template >
< ng-template pTemplate = "body"
let-book
let-rowData
let-x = "rowIndex" >
< tr [ngClass]="{'even' : x%2 == 0}">
< td pEditableColumn>
< p-cellEditor >
< ng-template pTemplate = "input" >
< input
pInputText
type = "text"
[(ngModel)]="rowData.name">
</ ng-template >
< ng-template pTemplate = "output" >
{{rowData.name}}
</ ng-template >
</ p-cellEditor >
</ td >
< td pEditableColumn>
< p-cellEditor >
< ng-template pTemplate = "input" >
< input
pInputText
type = "text"
[(ngModel)]="rowData.author">
</ ng-template >
< ng-template pTemplate = "output" >
{{rowData.author}}
</ ng-template >
</ p-cellEditor >
</ td >
< td pEditableColumn>
< p-cellEditor >
< ng-template pTemplate = "input" >
< input
pInputText
type = "number"
[(ngModel)]="rowData.year">
</ ng-template >
< ng-template pTemplate = "output" >
{{rowData.year}}
</ ng-template >
</ p-cellEditor >
</ td >
</ tr >
</ ng-template >
</ p-table >
</ div >
|
app.component.ts
import { Component } from '@angular/core' ;
interface Book {
name: String,
author: String,
year: Number
}
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styles: [
`
tr.even{
background-color: green;
color: white;
}
`
]
})
export class AppComponent {
books: Book[] = [];
ngOnInit() {
this .books = [
{
name: "Clean Code" ,
author: "Robert Cecil Martin" ,
year: 2008
},
{
name: "Introduction to Algorithms" ,
author: "Thomas H Corman" ,
year: 1989
},
{
name: "Refactoring" ,
author: "Martin Fowler" ,
year: 1999
},
{
name: "Code Complete" ,
author: "Steve McConnell" ,
year: 1993
},
{
name: "Programming Pearls" ,
author: "John Bentley" ,
year: 1986
},
{
name: "The Clean Coder" ,
author: "Robert Cecil Martin" ,
year: 2011
},
{
name: "Coders at Work" ,
author: "Peter Seibel" ,
year: 2009
},
{
name: "Effective Java" ,
author: "Joshua Bloch" ,
year: 2001
},
{
name: "Head First Java" ,
author: "Bert Bates" ,
year: 2003
}
];
}
}
|
app.module.ts
import { NgModule } from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { BrowserAnimationsModule }
from '@angular/platform-browser/animations' ;
import { AppComponent } from './app.component' ;
import { TableModule } from 'primeng/table' ;
import { FormsModule } from '@angular/forms' ;
import { InputTextModule } from 'primeng/inputtext' ;
@NgModule({
imports: [
BrowserModule,
BrowserAnimationsModule,
TableModule,
InputTextModule,
FormsModule
],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule { }
|
Output:
Reference: http://primefaces.org/primeng/table
Share your thoughts in the comments
Please Login to comment...