Angular PrimeNG MegaMenu Styling
Last Updated :
26 Dec, 2022
A responsive website may be created with great ease using the open-source Angular PrimeNG framework, which has a wide range of native Angular UI components for superb style. In this tutorial, we will learn how to style the MegaMenu component in Angular PrimeNG. Additionally, we will learn about the styles and their syntaxes that will be used in the code.
A component with several menu options can be created using the MegaMenu navigation component.
Angular PrimeNG MegaMenu Styling:
- p-megamenu: This class will be used to style a container element.
- p-menu-list: This class will be used to style a list element.
- p-menuitem: This class will be used to style a menuitem element.
- p-menuitem-text: This class will be used to style a label of a menu item.
- p-menuitem-icon: This class will be used to style an icon of a menu item.
- p-submenu-icon: This class will be used to style the arrow icon of a submenu.
Syntax:
<p-megaMenu
[model]="...">
</p-megaMenu>
Creating Angular application and Installing the modules:
Step 1: Use the command below to create an Angular application.
ng new appname
Step 2: Use the following command to move to our project folder, appname, after creating it.
cd appname
Step 3: Install PrimeNG in the specified location.
npm install primeng --save
npm install primeicons --save
Project Structure: The project structure will look like this once the installation is finished
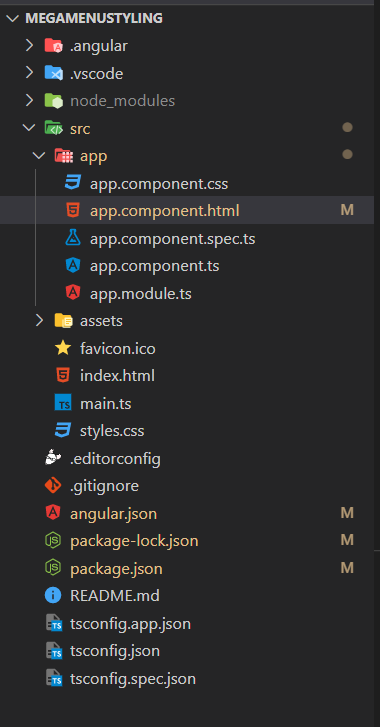
Â
Steps to run the application: Run the below command to see the output
ng serve --open
Example 1: This is a very basic example that explains how to style the MegaMenu Component.
HTML
< h1 >
< span >
< img src =
class = "gfg-logo" alt = "icon" />
</ span >
< span style = "color: green; font-size: 40px;" >
GeeksforGeeks
</ span >
</ h1 >
< h3 >PrimeNG MegaMenu Styling</ h3 >
< p-megaMenu [model]="gfg"></ p-megaMenu >
|
Javascript
import { Component } from "@angular/core" ;
import { MegaMenuItem } from "primeng/api" ;
@Component({
selector: "app-root" ,
templateUrl: "./app.component.html" ,
styleUrls: [ "./app.component.css" ]
})
export class AppComponent {
gfg: MegaMenuItem[] = [];
ngOnInit() {
this .gfg = [
{
label: "GFG Users" ,
icon: "pi pi-fw pi-users" ,
items: [
[
{
label: "User 1" ,
items: [
{ label: "User 1.1" },
{ label: "User 1.2" }
]
},
{
label: "User 2" ,
items: [
{ label: "User 2.1" },
{ label: "User 2.2" }
]
}
],
[
{
label: "User 3" ,
items: [
{ label: "User 3.1" },
{ label: "User 3.2" }
]
},
{
label: "User 4" ,
items: [
{ label: "User 4.1" },
{ label: "User 4.2" }
]
}
],
[
{
label: "User 5" ,
items: [
{ label: "User 5.1" },
{ label: "User 5.2" }
]
},
{
label: "User 6" ,
items: [
{ label: "User 6.1" },
{ label: "User 6.2" }
]
}
]
]
}
];
}
}
|
Javascript
import { NgModule } from "@angular/core" ;
import { BrowserModule } from "@angular/platform-browser" ;
import { BrowserAnimationsModule }
from "@angular/platform-browser/animations" ;
import { AppComponent } from "./app.component" ;
import { MegaMenuModule } from "primeng/megamenu" ;
@NgModule({
declarations: [AppComponent],
imports: [
BrowserModule,
BrowserAnimationsModule,
MegaMenuModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {}
|
CSS
:host ::ng-deep .p-megamenu {
background : #0da350 !important ;
}
:host
::ng-deep
.p-megamenu
.p-megamenu-root-list
> .p-menuitem.p-menuitem-active
> .p-menuitem-link,
.p-megamenu
.p-megamenu-root-list
> .p-menuitem.p-menuitem-active
> .p-menuitem-link:not(.p-disabled):hover {
background : #0da350 !important ;
}
:host
::ng-deep
.p-megamenu
.p-megamenu-root-list
> .p-menuitem
> .p-menuitem-link:not(.p-disabled):hover {
background : #0da350 !important ;
}
:host
::ng-deep
.p-megamenu
.p-megamenu-root-list
> .p-menuitem
> .p-menuitem-link
.p-menuitem- icon {
color : #fff !important ;
}
:host
::ng-deep
.p-megamenu
.p-megamenu-root-list
> .p-menuitem
> .p-menuitem-link
.p-menuitem-text {
color : #fff !important ;
}
:host
::ng-deep
.p-megamenu
.p-megamenu-root-list
> .p-menuitem
> .p-menuitem-link
.p-submenu- icon {
color : #fff !important ;
}
:host ::ng-deep .p-megamenu .p-menuitem-link:focus,
.p-megamenu .p-menuitem-link:active,
.p-megamenu .p-menuitem-link:hover {
box-shadow: inset 0 0 0 0.15 rem #0da350 !important ;
}
|
Output:
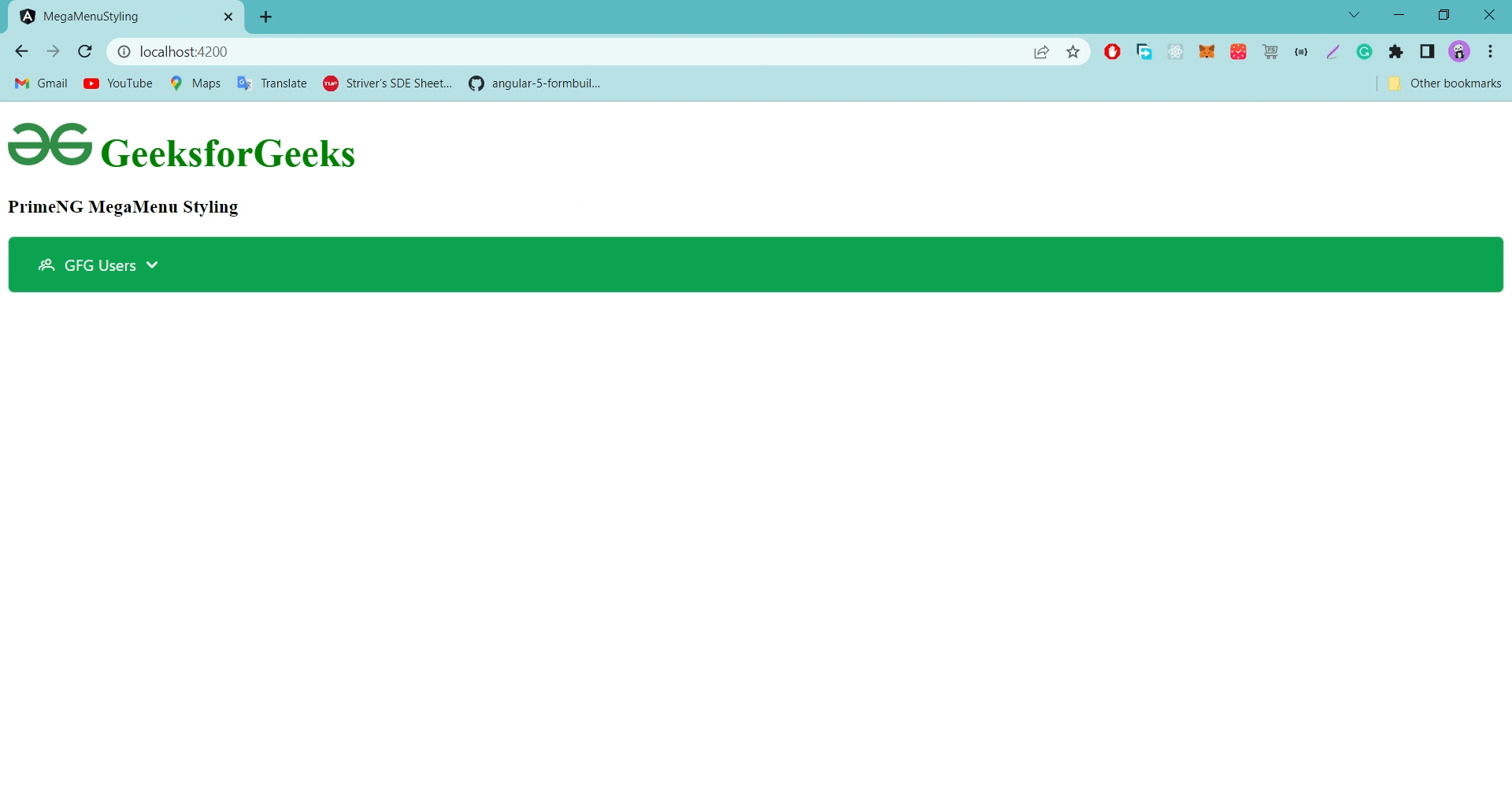
Â
Example 2: In this example, we will learn how to style the vertically oriented MegaMenu component on Focus and Hover view.
HTML
< h1 >
< span >
< img src =
class = "gfg-logo" alt = "icon" />
</ span >
< span style = "color: green; font-size: 40px;" >
GeeksforGeeks
</ span >
</ h1 >
< h3 >PrimeNG MegaMenu Styling</ h3 >
< p-megaMenu [model]="gfg" orientation = "vertical" ></ p-megaMenu >
|
Javascript
import { Component } from "@angular/core" ;
import { MegaMenuItem } from "primeng/api" ;
@Component({
selector: "app-root" ,
templateUrl: "./app.component.html" ,
styleUrls: [ "./app.component.css" ]
})
export class AppComponent {
gfg: MegaMenuItem[] = [];
ngOnInit() {
this .gfg = [
{
label: "GFG Users" ,
icon: "pi pi-fw pi-users" ,
items: [
[
{
label: "User 1" ,
items: [
{ label: "User 1.1" },
{ label: "User 1.2" }
]
},
{
label: "User 2" ,
items: [
{ label: "User 2.1" },
{ label: "User 2.2" }
]
}
],
[
{
label: "User 3" ,
items: [
{ label: "User 3.1" },
{ label: "User 3.2" }
]
},
{
label: "User 4" ,
items: [
{ label: "User 4.1" },
{ label: "User 4.2" }
]
}
],
[
{
label: "User 5" ,
items: [
{ label: "User 5.1" },
{ label: "User 5.2" }
]
},
{
label: "User 6" ,
items: [
{ label: "User 6.1" },
{ label: "User 6.2" }
]
}
]
]
}
];
}
}
|
Javascript
import { NgModule } from "@angular/core" ;
import { BrowserModule } from "@angular/platform-browser" ;
import { BrowserAnimationsModule }
from "@angular/platform-browser/animations" ;
import { AppComponent } from "./app.component" ;
import { MegaMenuModule } from "primeng/megamenu" ;
@NgModule({
declarations: [AppComponent],
imports: [
BrowserModule,
BrowserAnimationsModule,
MegaMenuModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {}
|
CSS
:host ::ng-deep .p-megamenu {
background : #0da350 !important ;
}
:host
::ng-deep
.p-megamenu
.p-megamenu-root-list
> .p-menuitem.p-menuitem-active
> .p-menuitem-link,
.p-megamenu
.p-megamenu-root-list
> .p-menuitem.p-menuitem-active
> .p-menuitem-link:not(.p-disabled):hover {
background : #0da350 !important ;
}
:host
::ng-deep
.p-megamenu
.p-megamenu-root-list
> .p-menuitem
> .p-menuitem-link:not(.p-disabled):hover {
background : #0da350 !important ;
}
:host
::ng-deep
.p-megamenu
.p-megamenu-root-list
> .p-menuitem
> .p-menuitem-link
.p-menuitem- icon {
color : #fff !important ;
}
:host
::ng-deep
.p-megamenu
.p-megamenu-root-list
> .p-menuitem
> .p-menuitem-link
.p-menuitem-text {
color : #fff !important ;
}
:host
::ng-deep
.p-megamenu
.p-megamenu-root-list
> .p-menuitem
> .p-menuitem-link
.p-submenu- icon {
color : #fff !important ;
}
:host ::ng-deep .p-megamenu .p-menuitem-link:focus {
box-shadow: inset 0 0 0 0.15 rem #0da350 !important ;
}
:host ::ng-deep .p-megamenu .p-menuitem-link:not(.p-disabled):hover {
background : #0da350 !important ;
border-radius: 5px ;
}
:host
::ng-deep
.p-megamenu
.p-menuitem-link:not(.p-disabled):hover
.p-menuitem-text {
color : #fff !important ;
}
|
Output:
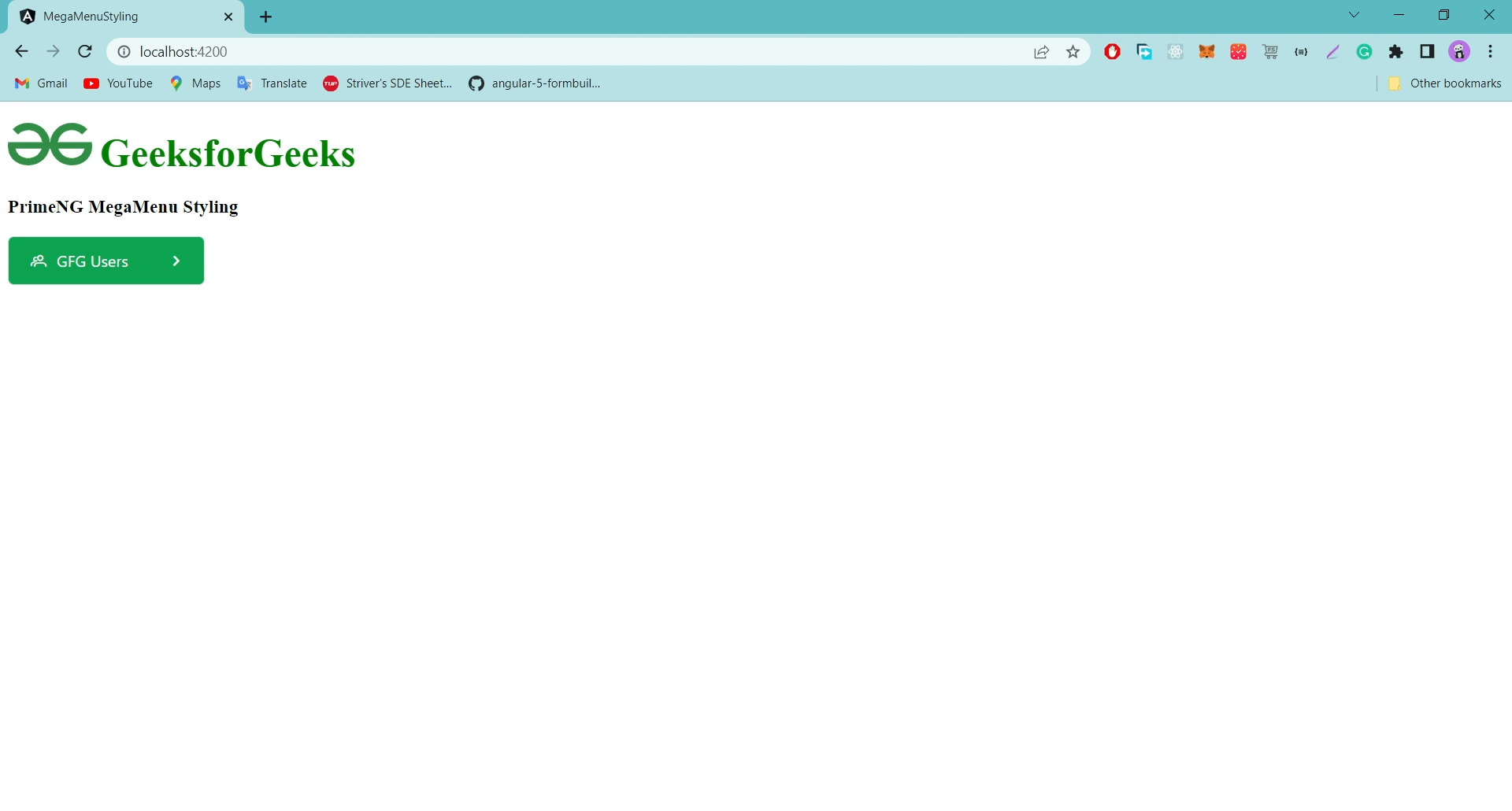
Â
Reference: https://primefaces.org/primeng/megamenu
Share your thoughts in the comments
Please Login to comment...