8086 program to search a number in a string
Last Updated :
22 May, 2018
Problem – Write an assembly language program in 8086 microprocessor to search a number in a string of 5 bytes, store the offset where the element is found and the number of iterations used to find the number.
Example –
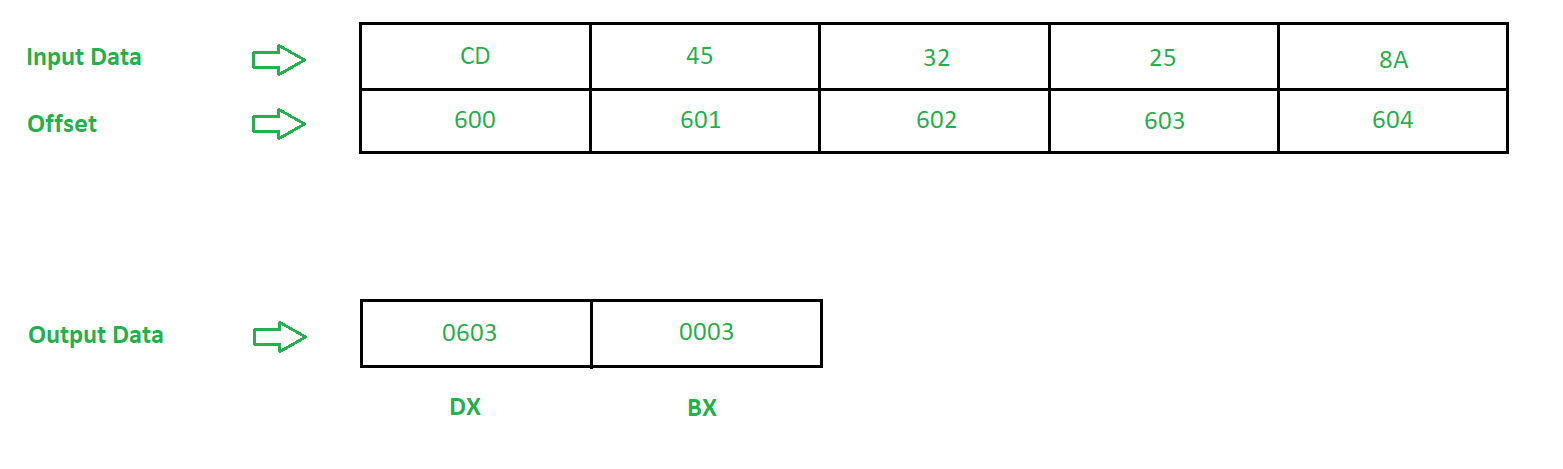
Algorithm –
- Move 2000 in AX and assign it to ES
- Assign value 600 to DI
- Move 25 in AL
- Move 0005 in CX
- Move the contents of CX to BX
- Clear the value of Directional Flag (DF)
- Repeat step 7 till Zero Flag (ZF) is not set
- Scan byte from [DI] and check its difference with contents of AL. Update the value of DI
- Decrements the value of DI by 1
- Subtract the value of BX by CX
- Decrements the value of BX by 1
- Halt the program
Program –
OFFSET |
MNEMONICS |
COMMENT |
400 |
MOV AX, 2000 |
AX <- 2000 |
403 |
MOV ES, AX |
ES <- AX |
405 |
MOV DI, 600 |
DI <- 600 |
408 |
MOV AL, 25 |
AL = 25 |
40A |
MOV CX, 0005 |
CX = 0005 |
40D |
MOV BX, CX |
BX <- CX |
40F |
CLD |
DF = 0 so that memory is accessed
from lower byte to higher byte |
410 |
REPNE SCAS B |
Repeat till ZF = 0. Scan value from [DI] and
compare with AL, Increment DI |
414 |
DEC DI |
DI = DI – 1 |
415 |
MOV DX, DI |
DX = DI |
417 |
SUB BX, CX |
BX = BX – CX |
419 |
DEC BX |
BX = BX – 1 |
41B |
HLT |
Stop |
Explanation –
- MOV AX, 2000 is used to move data to register AX.
- MOV ES, AX is used to move the data of AX to segment register ES.
- MOV DI, 600 is used to move offset 600 to Destination Index(DI).
- MOV AX, 25 is used to move data to AL.
- MOV CX, 0005 is used to move data to CX.
- MOV BX, CX is used to copy contents of CX to BX.
- CLD is used to clear the value of Directional Flag (DF), so that memory is accessed from lower byte to higher byte.
- REPNE SCAS B is used to scan data from DI and compare it with data from AL, if equal go to next step else increment the value of DI by 1 and repeat this step.
- DEC DI is used to decrement contents of DI by 1.
- MOV DX, DI is used to move the contents of DI to DX.
- SUB BX, CX is used to subtract the contents of BX by CX.
- DEC BX is used to decrement contents of BX by 1.
- HLT stops executing the program and halts any further execution.
Share your thoughts in the comments
Please Login to comment...