<%= vs <%- in EJS Template Engine
Last Updated :
27 Mar, 2024
EJS stands for Embeded JavaScript. It’s a simple templating language that lets you generate HTML with plain JavaScript. EJS allows you to embed JavaScript code directly into your HTML. It is often used directly in your HTML markup, making it easy to generate dynamic content.
In this article, we will discuss <%= and <%- in EJS, along with discussing significant differences that distinct them from each other.
What is <%=?
‘<%= %>’ is used to give out the output value of JavaScript expression into the HTML markup language. They directly output the result of the JavaScript expression and escape the HTML entity to prevent XSS(Cross-site-scripting) attacks.
Syntax:
<%= JavaScript expression %>
Featuers of <%=
- The purpose of ‘ <%= ‘ is to output the variable or the result of the JavaScript expression directly into the HTML document.
- <%= supports the feature of interpolation of JavaScript variables or expressions within HTML.
- We can use <%= in a control flow statement like ‘if‘, ‘else‘, and ‘switch‘ in JavaScript.
- We can use <%= in a looping statement like ‘for‘ , ‘while‘ and ‘do-while‘ in JavaScript.
- <%= can be nested within other <%= tags to create complex output structure.
Steps to Create an EJS application:
Step 1: Firstly, we will make the folder named root by using the below command in the VScode Terminal. After creation use the cd command to navigate to the newly created folder.
mkdir root
cd root
Step 2: Once the folder is been created, we will initialize NPM using the below command, this will give us the package.json file.
npm init -y
Step 3: Once the project is been initialized, we need to install Express and EJS dependencies in our project by using the below installation command of NPM.
npm i express ejs
Project Structure:
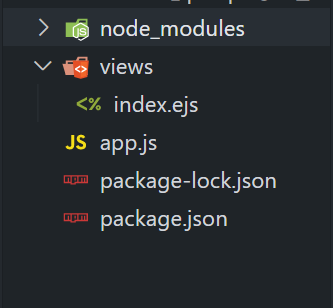
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2",
"ejs": "^3.1.9",
}
Example: Below is an example of <%= in EJS.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>EJS Example</title>
</head>
<body>
<h1>Hello, <%= username %>!</h1>
<p>Your age is <%= age %> and your email is <%= email %>.</p>
</body>
</html>
JavaScript
const express = require('express');
const app = express();
const port = 3000;
// Set EJS as the view engine
app.set('view engine', 'ejs');
// Sample user data
const userData = {
username: 'Anshul Ojha',
age: 21,
email: 'anshul@example.com'
};
// Route to render the EJS template
app.get('/', (req, res) => {
res.render('index', userData);
});
// Start the server
app.listen(port, () => {
console.log(`Server is running at http://localhost:${port}`);
});
Output:
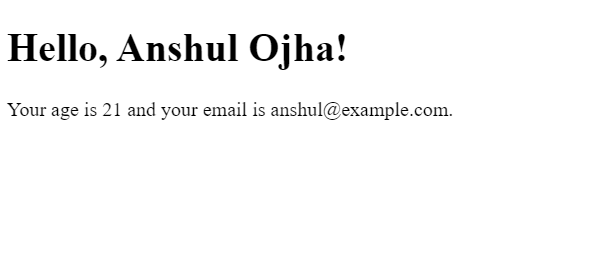
What is <%-?
‘<%- %>’ is majorly used for unescaped output, it directly injects the raw value of a javaScript expression into the HTML markup. It is useful for rendering HTML content generating the user input.
Syntax:
<%- JavaScript expression %>
Featuers of <%-
- The propose of ‘ <%-‘ is to output the content without escaping HTML entities. This is used when we want to show content with raw HTML.
- <%- supports feature to directly insert HTML markup into your templates.
- We can use <%- in a control flow statement like ‘if‘ , ‘else’ , and ‘switch‘ in JavaScript.
- We can use <%- in a looping statement like ‘for‘ , ‘while‘ and ‘do-while‘ in JavaScript.
- <%- can be used with other EJS tags like <%, <%#, and <%= to create complex dynamic template.
Example: Below is an example of <%- in EJS.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>EJS Example</title>
</head>
<body>
<h1>Hello, <%- username %>!</h1>
<p>Your age is <%- age %> and your email is <%- email %>.</p>
<p>Raw HTML content: <%- rawHTML %></p>
</body>
</html>
JavaScript
const express = require('express');
const app = express();
const port = 3000;
// Set EJS as the view engine
app.set('view engine', 'ejs');
// Sample user data
const userData = {
username: 'Anshul Ojha',
age: 20,
email: 'anshul@example.com',
rawHTML: '<strong>This is <em>raw</em> HTML content.</strong>'
};
// Route to render the EJS template
app.get('/', (req, res) => {
res.render('index', userData);
});
// Start the server
app.listen(port, () => {
console.log(`Server is running at http://localhost:${port}`);
});
Output:
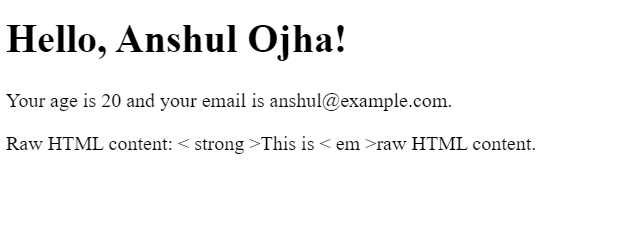
Difference between <%= and <%- in EJS:
Features
|
<%= %>
|
<%- %>
|
---|
Output
| Escapes HTML entities
| Does not escapes HTML entities
|
---|
HTML escape
| Yes
| No
|
---|
Use Case
| Safer for user input
| Rendering raw HTML from user input.
|
---|
When to use
| Rendering plain text
| Rendering HTML content
|
---|
Security consdieraaation
| Safer, prevents XSS attacks
| Requires necessary caution to avoid XSS inssues.
|
---|
Syntax
| <%= username %>
| <%- username %>
|
---|
Conclusion:
Understanding difference between these synatax is crucial for developers when working with EJS, as it maintains proper handling of data. These tags in EJS are important to determine how data is rendered on WEB PAGE. <%= %> is used for direct general output, maintain the safer rendering environment, while <%- %> is used when raw HTML output is required. Understanding the use case of these tag is important to maintain secure web development while using EJS.
Share your thoughts in the comments
Please Login to comment...