Azure Cosmos DB is a No SQL database service that supports flexible schemas and hierarchical data for modern applications. It is a globally distributed, multi-model database service that supports document, key-value, wide-column, and graph databases. Azure Cosmos DB service takes care of database administration, updates, patching, and scaling. It also provides cost-effective serverless and automatic scaling options that match capacity with demand. Cosmos DB integrates with other Azure services and tools, such as Azure Functions, IoT Hub, AKS (Azure Kubernetes Service), App Service, and more. The Azure SDK for JavaScript is a collection of libraries that you can use to interact with various Azure services from your JavaScript applications. It supports both Node.js and browser environments.
In this article, we will learn about working with ‘Azure Cosmos DB and the JavaScript SDK’. CRUD operations and other common operations on Azure Cosmos DB resources can be done using the JavaScript SDK. The Cosmos DB JavaScript SDK is primarily meant for use in Node.js applications.
To work with Azure Cosmos DB, the user needs to create the Cosmos DB by configuring it from the Azure Portal. If you already have an Azure Cosmos DB you can use it by following the steps after the ‘creation of Azure Cosmos DB’. As a prerequisite, users need to have an Azure subscription.
Steps to Create Azure Azure Cosmos DB
Step 1: Login to Azure Portal Select the Create Cosmos DB option and select the ‘Create’ option
Step 2: Select the option ‘Create’ from the ‘Azure Cosmos DB for NoSQL’ block
Step 3: This screen shows options to select/input for the cosmos db to be created. Select Resource Group Name, Add a name , Select Location, and Click ‘Review + Create
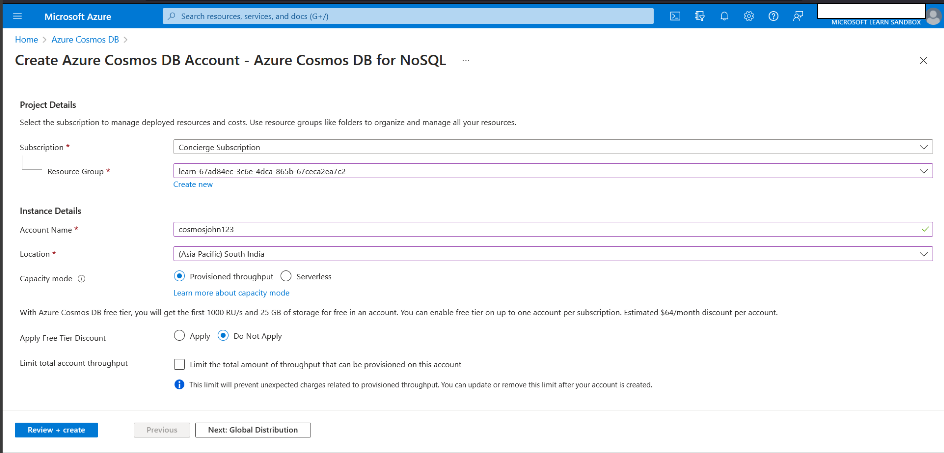
Step 4:Review and click ‘Create’
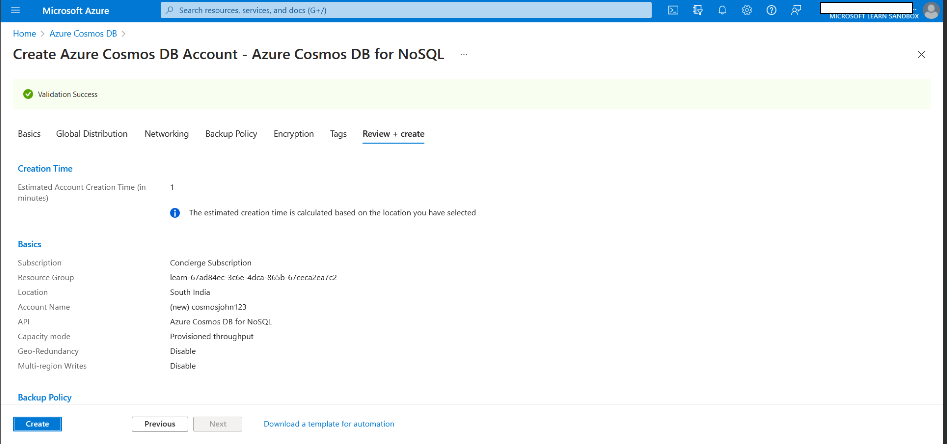
Step 5:The Cosmos DB creation progress will be displayed and will display the database details once done.
Next Steps:
Select the database created.
- Add the Collection Name
- Add the Partition Key
- Create a new Document and add some data.
Example data:
{
"id": "1",
"category": "personal",
"name" : "groceries",
"description" : "Pick up apples and strawberries",
"isComplete" : false
}
In the data Explorer window of Azure Portal, from the ‘Read-write Keys’ tab, you can get the URI which will go to the ‘endpoint’ and the primary key value will go to the ‘masterKey’ .
Working With the JavaScript SDK
The Javascript SDK is a package that can be installed via npm to create,read,update and delete items in an Azure Cosmos DB container. It provides features and options to perform optimized queries to fetch data from documents in your database.
Install the required dependency for the Azure Cosmos DB for NoSQL JavaScript SDK using the below bash command.
npm install @azure/cosmos
Write the Javascript SDK scripts for connecting and working with Cosmos DB from Visual Studio Code Editor in a file called ‘App.js’.Initially, CosmosClient class need to be created to interact with CosmosDB and then the required connection string details are added to access the Azure Cosmos DB. Once the connection is established, the script to fetch data and write it to console log is done using the iteration statement.
Node
const { CosmosClient } = require(“@azure/cosmos”);
const endpoint = “https://cosmosjohndatabase.documents.azure.com:443”; //Add the URI value
const masterkey = “
“; // add the key value for the end point
const client = new CosmosClient({ endpoint, auth: {masterkey } });
const databaseId = “cosmosjohndatabase”;
const containerId=”cosmosjohncollection”;
// Below is the code to connect to Cosmos DB and fetch data
async function main() {
const { result: results } = await client.database(databaseId).container(containerId).items.query(querySpec, { enableCrossPartitionQuery: true }).toArray();
//looping through data and writing to console
for (var queryResult of results){
let resultString = JSON.stringify(queryResult);
console.log(‘\tQuery returned ${resultString}\n);
}
}
main().catch((error) => {
console.error(error);
});
Once this code is run the resuts are printed in the console using the below command from Windows Powershell:
node App.js
Output

Best Practices For Working With Azure Cosmos Db
1. Choose The Appropriate Data Model And Right Api
- Use the appropriate data model (e.g., document, key-value, graph, column-family) based on your application’s needs.
- Use the right APIs that best suits your application’s requirements and preferences. Azure Cosmos DB supports various APIs such as SQL API, MongoDB API, Cassandra API, Gremlin API, and Table API.
2. Choose The Best Consistency Level.
- Azure Cosmos DB offers five consistency levels such as: strong, bounded staleness, session, consistent prefix, and eventual.
- Choose the appropriate consistency level based on your application’s requirements and trade-offs between consistency and performance.
3. Optimize The Partition Key To Improve Performance And Scalability.
- Azure Cosmos DB automatically partitions your data across multiple physical partitions based on the partition key you specify.
- A good partition key should have high cardinality, even distribution, and align with your query patterns.
4. Monitor And Tune Performance Regularly To Ensure Optimal Performance.
- Set up Cosmos DB diagnostic logs and metrics to monitor performance, errors, and resource usage.
- Integrate with Azure Monitor and Application Insights for advanced monitoring and alerting.
5. Implement Data Retention Policies And Archival Strategies To Manage Data Growth And Costs Effectively.
- Cosmos DB retention policy refers to how long and how often Azure Cosmos DB backs up your data.
- By default, Cosmos DB takes a full backup every 4 hours and retains the last 2 backups, which are free of charge.
- You can change the backup interval and retention period, but you will be charged extra for more than 2 backups.
- Utilize time-to-live (TTL) settings for automatic data expiration.
6. Multi-Region Data Distribution (Geo-Replication) And Multi-Region Writes (Multi-Master)
- Azure Cosmos DB can replicate and distribute your data to any or all Azure regions.
- Single region Azure Cosmos DB accounts have guaranteed 99.99% availability and adding additional regions to your Azure Cosmos DB account can further improve availability.
- Adding additional regions also helps lower latency by placing data physically closer to your users.
7. Use The.Net Sdk To Manage Azure Cosmos Db Resources.
- The.NET SDK provides a convenient way to interact with Azure Cosmos DB from your.NET applications.
- Azure SDKs for Cosmos DB are optimized for your programming language to simplify development and improve performance.
- You can use the SDK to create, read, update, and delete items in an Azure Cosmos DB container.
8. Performance & Cost Optimization
- When you created your new account, you had to choose the database operations pricing model that best meets the requirements of the application or workload.
- Select the best option between the provisioned throughput and serverless models.
These are the few best practices listed above. Backup and Disaster Recovery, Cost Management, Capacity Planning, Security and Query Optimization are some of the other best practices recommended.
Troubleshooting Common Azure Cosmos Db Problems
Here are some common issues you might encounter and steps to help resolve them:
1. Connection And Authentication Errors
Issue: Difficulty connecting to Azure Cosmos DB due to network problems or authentication errors.
Troubleshooting:
- Check your network connectivity and ensure that your firewall rules allow access to Cosmos DB.
- Verify that your authentication credentials, such as the endpoint and access key or resource token, are correct.
- If using Azure AD authentication, make sure it is correctly configured and permissions are granted.
2. High Request Unit (RU) Consumption
Issue: Unexpectedly high RU consumption, leading to increased costs.
Troubleshooting:
- Use the Query Explorer in the Azure Portal or diagnostic logs to identify and optimize inefficient queries.
- Implement correct indexing for frequently used queries.
- Consider enabling and reviewing the Query Metrics in the Azure Portal for insights into query performance.
3. Query Timeout Errors
Issue: Queries timing out or taking too long to execute.
Troubleshooting:
- Optimize queries that frequently time out by indexing, using the right partition key, or increasing RU limits.
- Adjust the client application’s timeout settings to allow for longer query execution times if necessary.
4. Resource Exhaustion
Issue: Running out of resources such as throughput, CPU, or memory.
Troubleshooting:
- Monitor resource utilization, including CPU and memory, using Azure Monitor and scale your Cosmos DB container or throughput provision as needed.
- Consider using autoscale to automatically adjust resources based on demand.
5. Resource Exhaustion
Issue: Running out of resources such as throughput, CPU, or memory.
Troubleshooting:
- Monitor resource utilization, including CPU and memory, and scale your Cosmos DB container or throughput provision as needed.
- Consider using autoscale to automatically adjust resources based on demand.
6. Client-Side Errors and Exceptions
Issue: Client applications encountering errors or exceptions when interacting with Cosmos DB.
Troubleshooting:
- Implement proper error handling mechanisms in your JavaScript application, including try-catch blocks or promises.
- Review and address error messages and codes provided by the SDK for specific issues.
- Enable diagnostic logging and monitor logs for any unusual behavior or error messages.
When troubleshooting Cosmos DB issues, it’s important to gather as much information as possible, including error messages, logs, and performance metrics. Azure’s monitoring and diagnostic tools can be invaluable for identifying and resolving problems quickly.
FAQ’s on Working with Azure Cosmos DB and the JavaScript SDK
Here are the FAQs which should help you get started with ‘working with Azure Cosmos DB and the JavaScript SDK’:
1. What is Azure Cosmos DB, and how does it relate to the JavaScript SDK?
Azure Cosmos DB is a globally distributed, multi-model database service on Microsoft Azure that supports document, key-value, wide-column, and graph databases.
The JavaScript SDK is a client library that allows you to interact with Cosmos DB from JavaScript-based applications.
2. How do I install and set up the Azure Cosmos DB JavaScript SDK?
You can install the SDK via npm or yarn: npm install @azure/cosmos.
You will need your Cosmos DB account endpoint and key to create an instance of the CosmosClient class.
You can find these in the Azure Portal or use the Azure CLI to get them.
3. How do I create a new database and container using the JavaScript SDK?
You can use the @azure/cosmos SDK to create a database and container by sending appropriate requests to the Cosmos DB service. Ensure you have the necessary permissions and authentication set up.
4. What data models does Azure Cosmos DB support, and how does the JavaScript SDK handle them?
Azure Cosmos DB supports multiple data models, including document, key-value, graph, and column-family. The JavaScript SDK allows you to work with these models seamlessly using JavaScript or TypeScript.
5. What are the different APIs supported by Azure Cosmos DB, and which one should I use with the JavaScript SDK?
Azure Cosmos DB supports several APIs, including Core (SQL), MongoDB, Cassandra, Gremlin, and Table.
You should choose the API that aligns with your application’s data model. The JavaScript SDK primarily works with the Core (SQL) API.
6. How can I secure access to my Cosmos DB resources when using the JavaScript SDK?
You can secure access using master keys, resource tokens, or Azure Active Directory (Azure AD) authentication. Always follow best practices for securely storing and managing keys or tokens.
7. What are Request Units (RUs), and how do I calculate and manage them using the JavaScript SDK?
Request Units (RUs) represent the throughput capacity in Cosmos DB. You can calculate and manage RUs using the SDK by specifying the RU consumption for each operation or by enabling automatic RU provisioning.
8. How do I perform CRUD (Create, Read, Update, Delete) operations on documents with the JavaScript SDK?
You can use the JavaScript SDK to create, read, update, and delete documents in Cosmos DB containers by invoking appropriate methods like ‘container.items.create’, ‘container.item.read’, ‘container.item.replace’, and ‘container.item.delete’.
9. What is the recommended way to handle errors and exceptions when working with the JavaScript SDK?
The SDK provides error handling mechanisms, including exception handling and error codes. Implement try-catch blocks or use promises to handle errors gracefully.
10. How do I query data in Cosmos DB using the JavaScript SDK?
You can use the ‘container.items.query’ method to perform SQL-like queries on documents within a container. Ensure you define proper indexes to optimize query performance.
11. Is there a recommended design pattern for handling Cosmos DB connections and reusing clients in JavaScript applications?
It’s recommended to use a singleton pattern to manage Cosmos DB client instances for efficiency and resource management.
12. How do I monitor and troubleshoot performance issues with the JavaScript SDK?
Utilize logging, error handling, and Azure Monitor to monitor and troubleshoot performance issues. You can enable diagnostic logs and metrics to gain insights into SDK performance.
Share your thoughts in the comments
Please Login to comment...