Window Object in JavaScript
Last Updated :
20 Mar, 2024
In JavaScript, the Window
object represents the window that contains a DOM document.
The Window
object provides access to various properties and methods that enable interaction with the browser environment, including manipulating the document, handling events, managing timers, displaying dialog boxes, and more.
Window Object Properties
The Window
object in JavaScript has numerous properties that provide access to various aspects of the browser environment and the window itself. Some commonly used properties include:
Property Name
| Description
|
window.document | The HTML document that is shown in the window is represented by the Document object, which is referred to by the document property. |
window.console | The console gives the window’s Console Object back. |
window.location | The location attribute makes reference to the Location object, which has data about the page’s current URL. |
window.defaultStatus | It is now Abandoned. |
window.closed | If a window is closed, it returns a true boolean value. |
window.frameElement | It gives the window’s current frame back. |
window.frame | returns every window item currently active in the window. |
window.history | Retrieves the window’s History object. |
window.length | It gives the number of iframe> elements currently present in the window. |
window.localStorage | provides the ability to store key/value pairs in a web browser. stores data without a time. |
window.innerWidth and window.innerHeight | Without including the toolbars and scrollbars, these characteristics describe the width &Â height of the browser window. |
window.opener | It returns a pointer to the window that created the window in the opener function. |
window.outerHeight | You can use outerHeight to return the height of the browser window, including toolbars and scrollbars. |
window.outerWidth | You can outerWidth to get the width of the browser window, including toolbars and scrollbars. |
window.name | Returns or sets a window’s name. |
window.parent | Brings up the current window’s parent window. |
window.sessionStorage | Provides the ability to store key/value pairs in a web browser. Contains data for a single session. |
window.scrollX | It is a pageXOffset alias. |
window.scrollY | It is a pageYOffset alias. |
window.self | It provides the window’s current state. |
window.status | It is now Deprecated. Don’t employ it. |
window.top | It provides the top-most browser window back. |
window.screen | The screen attribute makes reference to the Screen object, which stands in for the screen that the browser is shown on. |
window.history | The History object, which includes details about the current page’s browsing history, is the subject of the history property. |
window.pageXOffset | The number of pixels that the current document has been scrolled horizontally. |
window.pageYOffset | The number of pixels that the current document has been scrolled vertically. |
window.screenLeft: | The x-coordinate of the current window relative to the screen. |
window.screenTop | The y-coordinate of the current window relative to the screen. |
window.screenX | The x-coordinate of the current window relative to the screen (deprecated). |
window.screenY | The y-coordinate of the current window relative to the screen (deprecated). |
window.navigator | An object representing the browser and its capabilities |
Window Object Properties Examples
Example 1: We will use the console property in this example to show how it works.
Javascript
// This is console property
console.log(window.location)
Output:
Location {ancestorOrigins: DOMStringList, href: 'chrome://new-tab-page/', origin: 'chrome://new-tab-page', protocol: 'chrome:', host: 'new-tab-page', …}
Window Object Methods:
A method in JavaScript is a function connected to an object. You may conduct operations or compute values by calling methods on objects.
Syntax:
window.MethodName()
NOTE: Depending on the attributes, a parameter could have any value, including a string, number, object, and more.
Here is a list of some of the methods of the window object:
Property Name | Description |
window.open() | This method opens a new browser window or tab. |
window.close() | This method closes the current window or tab. |
window.alert() | This method displays an alert message to the user. |
window.prompt() | This method displays a prompt message to the user and waits for their input. |
window.confirm() | This method displays a confirm message to the user and waits for their response.window.focus: brings the current window or tab to the front. |
window.blur() | Sends the current window or tab to the back. |
window.postMessage() | Sends a message to the window or frame that is targeted by the specified WindowProxy object. |
window.scrollTo() | Scrolls the window or frame to a specific position. |
window.scrollBy() | Scrolls the window or frame by a specific number of pixels. |
window.resizeTo() | Resizes the window to a specific size. |
window.resizeBy() | Resizes the window by a specific number of pixels. |
window.atob() | A base-64 encoded string is decoded via atob(). |
window.btoa() | Base-64 encoding is done with btoa(). |
window.clearInterval() | A timer set with setInterval() is reset. |
window.clearTimeout() | The function clearTimeout() resets a timer specified with setTimeout(). |
window.focus() | It switches the focus to the active window. |
window.getComputedStyle() | This function returns the element’s current computed CSS styles. |
window.getSelection() | It provides a Selection object corresponding to the user-selected text selection range. |
window.matchMedia() | The provided CSS media query string is represented by a MediaQueryList object created by the matchMedia() function. |
window.moveBy() | Relocates a window with respect to its present location. |
window.moveTo() | Relocates a window to the given location. |
window.print() | Displays what is currently displayed in the window. |
window.requestAnimationFrame() | Before the subsequent repaint, the browser is asked to invoke a function to update an animation using the requestAnimationFrame() method. |
window.setInterval() | At predetermined intervals, setInterval() calls a function or evaluates an expression (in milliseconds). |
window.setTimeout() | When a certain amount of milliseconds have passed, the setTimeout() method calls a function or evaluates an expression. |
window.stop() | It halts the loading of the window. |
Window Object Method Example
Example 1: We’ll use the window.alert method in this example to show how it works:
Javascript
// This is window.alert method
console.log(window.alert("GeeksforGeeks"))
Output:
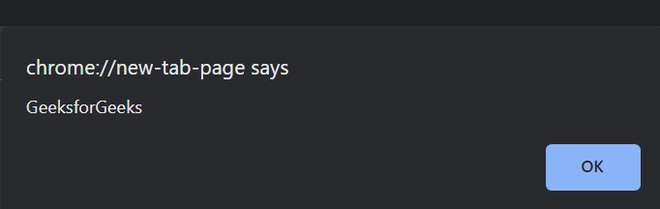
Example 2: In this example, we’ll use the setInterval() Method:
HTML
<!DOCTYPE html>
<html>
<body>
<h2>The setInterval() Method</h2>
<p id="demo"></p>
<script>
const element = document.getElementById("demo");
setInterval(function () {
element.innerHTML += "GeeksforGeeks</br>"
}, 500);
</script>
</body>
</html>
Output:
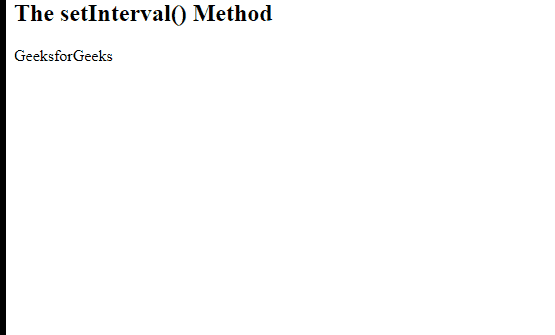
setInterval() Method Example Output
Conclusion:
The window object in JavaScript represents the browser window or frame. It offers properties like innerHeight, innerWidth, screen, and methods like alert(), confirm(), open(), enabling interaction and control over the window and document.
Share your thoughts in the comments
Please Login to comment...