What are JavaScript Events ?
Last Updated :
02 Feb, 2024
JavaScript Events are the action that happens due to the interaction of the user through the browser with the help of any input field, button, or any other interactive element present in the browser.
Events help us to create more dynamic and interactive web pages. Also, these events can be used by the eventListeners provided by the JavaScript.
These are the events that can be used directly on the HTML page for dynamic interactivity:
Mouse Events
These are the events that are triggered when there is any interaction due to the mouse.
It is mainly used for buttons, when the button is clicked this event can be used. we can directly use this event in HTML. Also, we can use this event by the use of JavaScript by using the addEventListerner.
Example: This example shows the use of onclick event to show the text on the screen.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Document</ title >
</ head >
< body >
< h2 >onclick Event in Html</ h2 >
< button onclick = "myFunction()" >Click me</ button >
< p id = "gfg" ></ p >
< script >
function myFunction() {
document.getElementById(
"gfg").innerHTML = "GeeksforGeeks onclick event";
}
</ script >
</ body >
</ html >
|
Output:
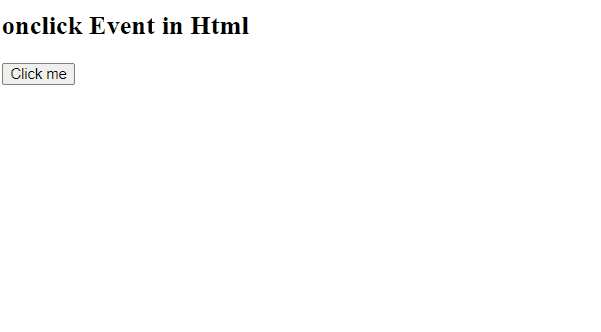
onclick event
onmouseover event triggers when there is any hovering occurring on the specific element that is under observation. and onmouseout event occurs when the user takes out the hovered mouse from the observable area or element.
Example: This example shows the use of the onmouseover and onmouseout event in JavaScript.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
DOM onmouseover event
</ title >
</ head >
< body >
< h1 id = "hID" >
GeeksforGeeks
</ h1 >
< h2 >
HTML DOM onmouseover event
</ h2 >
< script >
document.getElementById(
"hID").addEventListener(
"mouseover", over);
document.getElementById(
"hID").addEventListener(
"mouseout", out);
function over() {
document.getElementById(
"hID").style.fontSize = "40px";
}
function out() {
document.getElementById(
"hID").style.fontSize = "20px";
}
</ script >
</ body >
</ html >
|
Output:
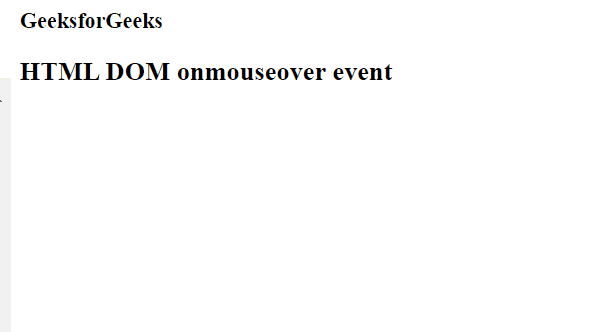
mouseover event
Keyboard Events
The events that occurs because of the keys present in the keyboard are considered as keyboard events. these events include keypress, keydown and keyup actions. They all may look like the same but their behaviour is little bit different from each other.
This event occurs when a user presss any of the key present in his keyboard that generates a character value. So, when the user will press the key it will detect as keypress event.
This event occurs when a user presses the key on keyboard it immideatly triggers the event and it does not require any kind of character production.
This event occurs just after the keydown. means when a user releases the pressed key then this events triggered.
Example: This example shows the use of keyup and keydown event.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >Document</ title >
</ head >
< body >
< body style = "text-align:center;" >
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< p >
< b >Keyup, onKeydown Events</ b >
</ p >
< input type = "text" id = "field" placeholder = "press any key!!" >
< p id = "status" ></ p >
< script >
// Script to test which key
// event gets triggered
// when a key is pressed
let key_pressed =
document.getElementById('field');
key_pressed
.addEventListener("keydown", onKeyDown);
key_pressed
.addEventListener("keyup", onKeyUp);
function onKeyDown(event) {
document.getElementById("status")
.innerHTML = 'keydown: '
+ event.key + '< br >'
}
function onKeyUp(event) {
document.getElementById("status")
.innerHTML += 'keyup: '
+ event.key + '< br >'
}
</ script >
</ body >
</ body >
</ html >
|
Output:
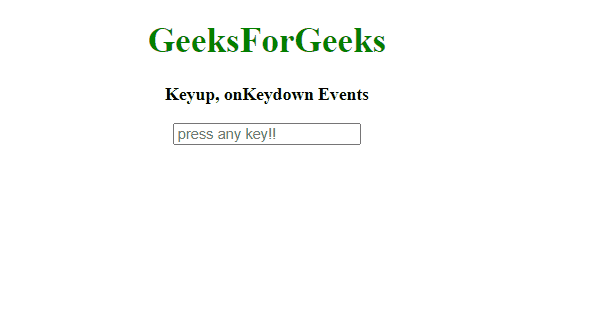
Keyboard events
Form Events
Those events that are mainly used in forms can be considered as Form Events.
This event occurs when users submit thier form.
Example: This example shows the use of the submit event. after submiting the form it shows the value of the input in the alert box.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >Submit Event Example</ title >
</ head >
< body >
< form id = "myForm" >
< label for = "username" >Username:</ label >
< input type = "text" id = "username" name = "username" >
< button type = "submit" >Submit</ button >
</ form >
< script >
document.getElementById("myForm").addEventListener("submit",
function (event) {
event.preventDefault();
let name = document.getElementById("username");
alert("Submitted! Username: " + name.value);
});
</ script >
</ body >
</ html >
|
Output:
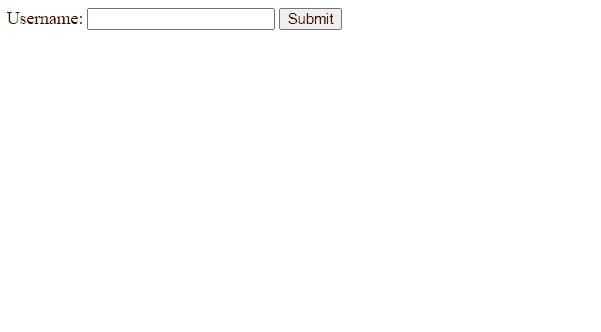
submit event
TIt keeps track of the changes made by the user on the HTML page. if we are using this event in JavaScript directly then we must use”change” in the addEventlistener. but if we are using directly it into the HTML page then we have to use “onChange” as an attribute in an element.
Example: This example shows that if the input is in all caps then it will change it into the all lowecase.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 .0">
< title >Document</ title >
</ head >
< body >
< h1 style = "color:green" >
GeeksforGeeks
</ h1 >
< h2 >HTML DOM onchange Event</ h2 > Email:
< input type = "email" id = "email" >
< script >
document.getElementById(
"email").addEventListener(
"change", GFGfun);
function GFGfun() {
let x = document.getElementById("email");
x.value = x.value.toLowerCase();
}
</ script >
</ body >
</ html >
|
Output:
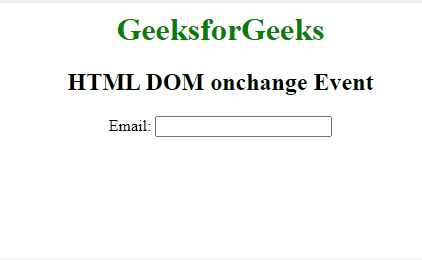
on change event
Window Events
These are the events that occurs globally means it associates with web page and windows.
This event is used when we want to load other resources on our web page. mostly it is used to load the script file on the web page.
Example: This example shows the loading of image on the HTML web page.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
onload Event
</ title >
</ head >
< body >
< img src =
alt = "GFG_logo" >
< p id = "pid" ></ p >
< script >
document.getElementById("imgid")
.addEventListener("load", GFGFun);
function GFGFun() {
document.getElementById("pid")
.innerHTML = "Image loaded";
}
</ script >
</ body >
</ html >
|
Output:
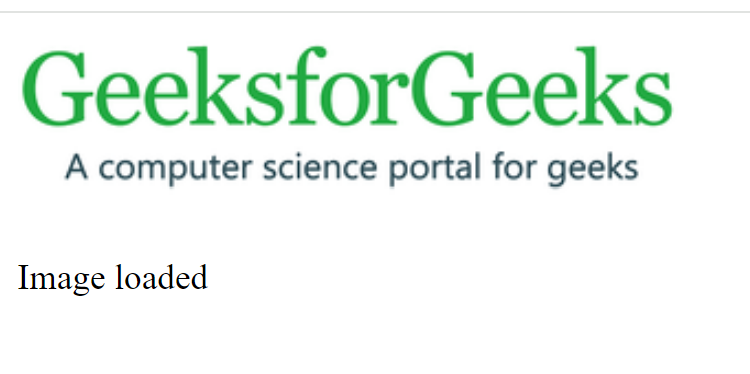
onload event
This event occurs when user resizs the browser window.
Example: This example shows the use of the onresize event. whenever there is resize occuring in web page then there is increment of a variable and we are present that on the screen.
HTML
<!DOCTYPE html>
< html >
< head >
< title >onresize event</ title >
</ head >
< body >
< h1 style = "color:green" >GeeksforGeeks</ h1 >
< h2 >onresize event</ h2 >
< p >Resize the window</ p >
< p >Resized count: < span id = "try" >0</ span ></ p >
< script >
window.addEventListener("resize", GFGfun);
let c = 0;
function GFGfun() {
let res = c += 1;
document.getElementById("try").innerHTML = res;
}
</ script >
</ body >
</ html >
|
Output:
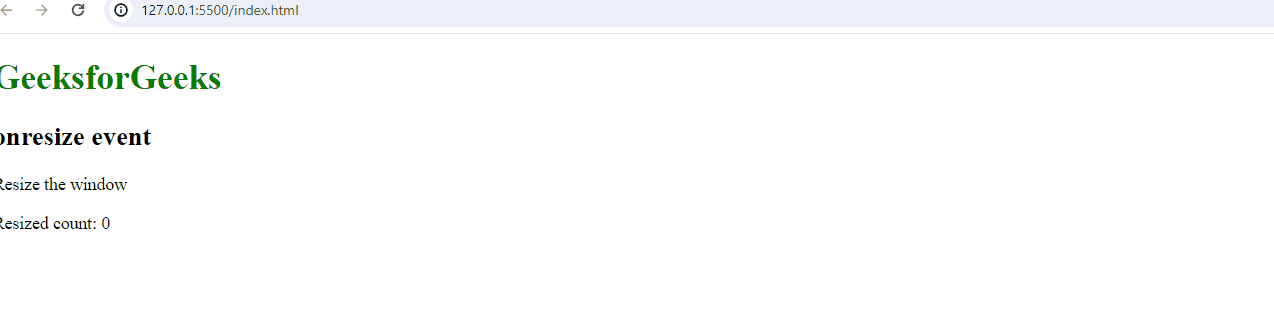
onresize event
This event occurs once at a time. when a page is unable to load it triggres the unload event.
Example: This example shows the use of the unload event.
HTML
<!DOCTYPE html>
< html >
< head >
< title > Onunload Event example </ title >
</ head >
< body onload = "myEvent()" >
< h2 > Onunload Event</ h2 >
< script >
function myEvent() {
alert("It is onunload event!!");
}
</ script >
</ body >
</ html >
|
Output:
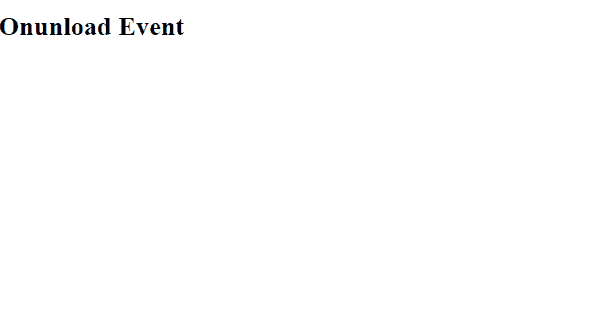
onunload event
This event triggers when a user focuses on an element. we can make any changes after focusing on that element.
Example: This example shows that on focusing on the input field it gets green background color.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 .0">
< title > onfocus Event Example</ title >
</ head >
< body >
< h1 style = "color:green" >
GeeksforGeeks
</ h1 >
< h2 >
onfocus Event
</ h2 >
< br > Name:
< input type = "text" onfocus = "geekfun(this)" >
< script >
function geekfun(gfg) {
gfg.style.background = "green";
}
</ script >
</ body >
</ html >
|
Output:
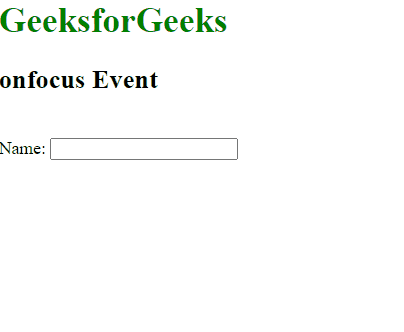
onfocus event
This event is used when we are not focusing on the element.
Example: This example shows that if we loses the focus from the input field then it shows the alert.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 .0">
< title >onblur event</ title >
</ head >
< body >
< h1 style = "color:green" >
GeeksforGeeks
</ h1 >
< h2 >onblur event</ h2 > Email:
< input type = "email" id = "email" onblur = "myFunction()" >
< script >
function myFunction() {
alert("Focus lost");
}
</ script >
</ body >
</ html >
|
Output:
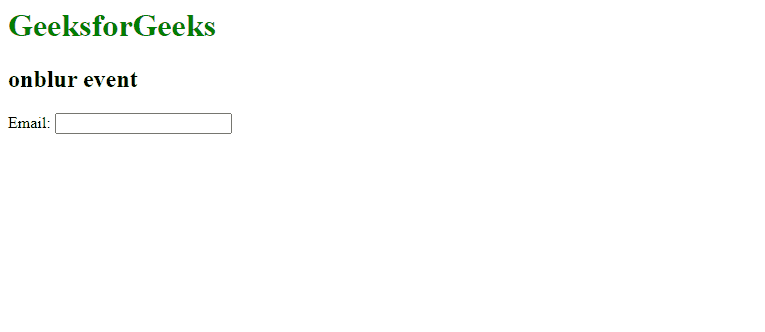
onblur event
Share your thoughts in the comments
Please Login to comment...