Pointer Events in Javascript DOM
Last Updated :
10 Feb, 2023
Pointer events are a set of DOM (Document Object Model) events that provide a unified way of handling inputs from a variety of devices, such as touchscreens, mouse, and pen/stylus. These events are supported by modern browsers and allow developers to write code that responds to user interactions with the page in a consistent and predictable way, regardless of the type of input device being used.
Some of the most commonly used pointer events include:
- pointerdown: The event fired when the user presses their pointer on an element
- pointerup: The event fired when the user releases their pointer from an element
- pointermove: The event fired when the user moves their pointer over an element
- pointerover: The event fired when the user moves their pointer over an element
- pointerout: The event fired when the user moves their pointer out of an element
- pointercancel: The event fired when the user’s pointer interaction is interrupted (such as by switching to a different application
Syntax:
<element pointerdown ="ID">
<element pointerup ="ID">
<element pointermove ="ID">
<element pointerover= "ID">
<element pointerout = "ID">
<element pointercancel= "ID">
Why pointer events are better than mouse events?
Pointer events and mouse events are both used for handling user interactions with a webpage, such as clicks, hover, and scrolling. However, pointer events are generally considered to be better than mouse events for several reasons:
- Pointer events provide a more consistent experience across devices: Pointer events are designed to work with a wide range of input devices, including touchscreens, mouse, and stylus.
- Pointer events provide more information: Pointer events provide more information about the input device, such as its type (e.g. mouse, touch, pen), pressure, and tilt.
- Pointer events are more efficient: Pointer events are designed to be more memory-efficient and have lower overhead than mouse events.
- Pointer events are more flexible: Pointer events are more flexible than mouse events in that they can be used to handle both single and multi-touch interactions.
Example 1: Below program illustrate pointermove and pointerout property:
HTML
<!DOCTYPE html>
< html >
< head >
< title >Pointer events Javascript DOM</ title >
</ head >
< body >
< center >
< div >
< h1 >
Welcome to GeeksforGeeks
</ h1 >
< p id = "myP" onpointermove = "pointerMove()"
onpointerout = "pointerOut()" >
The pointerMove() function works when the
pointer is go over text and this paragraph
sets the color of the text to 'red'.
The pointerOut() function works when the
pointer goes out of text zone then and the
color of the text sets to 'blue'.
</ p >
</ div >
< script >
function pointerMove() {
document.getElementById("myP")
.style.color = "red";
}
function pointerOut() {
document.getElementById("myP")
.style.color = "blue";
}
</ script >
</ center >
</ body >
</ html >
|
Output:

Pointer events Javascript DOM
Example 2: Below program illustrate pointerup and pointerdown and pointerover properties:
HTML
<!DOCTYPE html>
< html >
< head >
< title >Pointer events Javascript DOM</ title >
</ head >
< body >
< center >
< div >
< h1 >
Welcome to GeeksforGeeks
</ h1 >
< p id = "myP" onpointerup = "pointerUp()"
onpointerdown = "pointerDown()"
onpointerover = "pointerOver()" >
Pointer events are a set of DOM (Document
Object Model) events that provide a unified
way of handling inputs from a variety of
devices, such as touchscreens, mouse, and
pen/stylus. These events are supported by
modern browsers and allow developers to
write code that responds to user
interactions with the page in a consistent
and predictable way, regardless of the type
of input device being used.
</ p >
< script >
function pointerUp() {
document.getElementById("myP")
.style.color = "red";
}
function pointerDown() {
document.getElementById("myP")
.style.color = "blue";
}
function pointerOver() {
document.getElementById("myP")
.style.color = "yellow";
}
</ script >
</ center >
</ body >
</ html >
|
Output:
- When the pointer is moved over the text, the pointerOver() function is called. This function changes the color of the text to “yellow”.
- Similarly, when the pointer is clicked on the text, the pointerDown() function is called. This function sets the color “blue” to the text.
- Lastly, when the pointer is released after clicking on the text, the pointerUp() function is called. This function sets the color “red” to the text.
- So, The overall effect is when the pointer is moved over the text, the text color changes to yellow, when the pointer is pressed on the text, the text color changes to blue and when the pointer is released on the text, the text color changes to red.
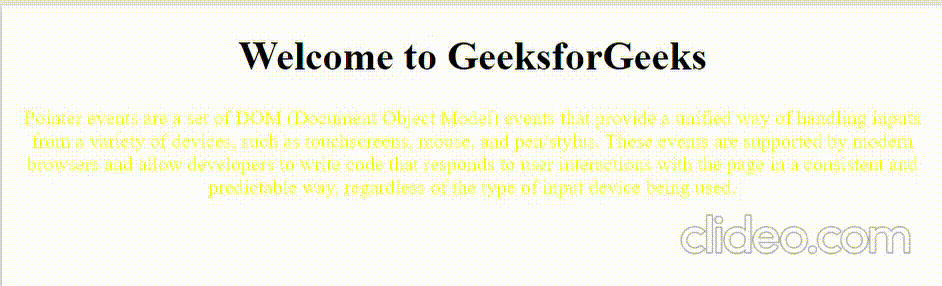
Pointer events Javascript DOM
Share your thoughts in the comments
Please Login to comment...