What are Cache Locks?
Last Updated :
18 Mar, 2024
In computer architecture, cache locks play a crucial role as shared data access coordinators in systems with several cores and processors. Cache locks are essential for preserving program accuracy and averting data corruption because they are made to guarantee data consistency and prevent race situations. Ensuring data integrity and optimizing system efficiency are interdependent and necessary for the smooth operation of contemporary computer environments.
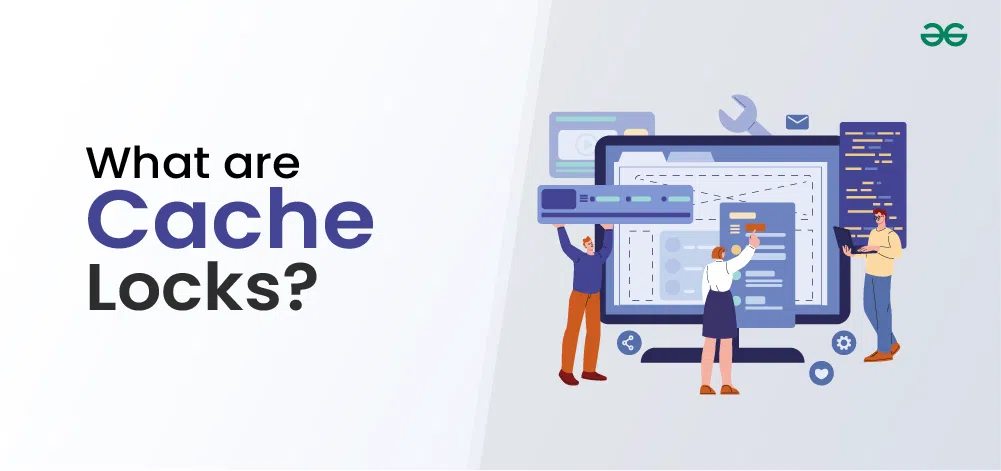
Important Topics for Cache Locks
What are Cache locks?
In the context of computer systems, cache locks typically relate to methods or devices that manage cache access, especially in contexts with many cores or processors. To expedite the CPU’s data retrieval process, caches are compact, high-speed memory modules that hold data that is recently utilized or regularly accessed. A common cache may be accessed by several cores or processors in a system with numerous cores or processors. To prevent conflicts and guarantee data consistency, cache locks are used to control and coordinate access to the cache.
Purpose of Cache locks
The purpose of cache locks, or cache coherence mechanisms in general, is to ensure the consistency of shared data in a multi-core or multi-processor system where multiple processors or cores have their caches. The main goals of cache locks include:
- Data Consistency: In a multi-core system, each core may have its cache, and copies of the same data may exist in multiple caches. Cache locks help maintain data consistency by coordinating access to shared data. They ensure that all copies of the data are kept up-to-date and coherent, preventing inconsistencies that could arise from concurrent access.
- Preventing Race Conditions: Without proper synchronization, multiple cores might attempt to read or modify the same data simultaneously, leading to race conditions. Cache locks help avoid such situations by enforcing order and coordination in accessing shared resources, ensuring that only one core at a time can perform read or write operations on a specific piece of data.
- Avoiding Data Corruption: If multiple cores are allowed to modify the same data without coordination, it can result in data corruption. Cache locks help prevent conflicting modifications by allowing only one core to have exclusive access to a cache line at any given time. This ensures that modifications are serialized and do not interfere with each other.
- Maintaining Program Correctness: In multi-threaded or multi-process applications, correct program execution depends on the proper handling of shared data. Cache locks contribute to program correctness by providing a mechanism for controlled access to shared resources, preventing unpredictable behavior and ensuring that the program behaves as intended.
- Performance Optimization: While cache locks introduce coordination overhead, they are essential for maintaining data integrity. However, efficient cache coherence mechanisms strive to minimize this overhead to avoid negatively impacting performance. Techniques such as cache invalidation, write propagation, and fine-grained locking aim to strike a balance between data consistency and system performance.
Types of Cache Locks
Below are the types of cache locks:
1. Read Locks
Read locks, also known as shared locks, allow multiple threads or processes to read data from the cache concurrently. However, they prevent any thread from writing to the cache while the lock is held. Read locks are useful when multiple threads need to read the same data without modifying it.
2. Write Locks
Write locks, also known as exclusive locks, allow only one thread or process to write to the cache at a time. Write locks prevent other threads from reading or writing to the cache while the lock is held. Write locks are used when a thread needs to modify data in the cache to ensure that no other thread can access the data concurrently.
3. Read/Write Locks (Upgradable Locks)
Read/write locks combine the functionality of read and write locks, allowing a thread to acquire a read lock to read data and then upgrade it to a write lock to modify the data. This allows for more flexible access patterns while still ensuring data consistency.
4. Optimistic Locks
Optimistic locks allow multiple threads to read and modify data concurrently without acquiring a lock. When a thread wants to modify data, it first reads the data and checks if it has changed since it was last read. If the data has not changed, the thread applies the modification and updates the cache. If the data has changed, the thread retries the operation or takes appropriate action. Optimistic locks are useful when conflicts are rare and the overhead of acquiring locks is high.
5. Pessimistic Locks
Pessimistic locks, in contrast to optimistic locks, acquire a lock before accessing data. Pessimistic locks are used when conflicts are common, and the cost of acquiring a lock is acceptable. Pessimistic locks ensure that only one thread can access data at a time, preventing conflicts and ensuring data consistency.
How Cache Locks works?
Cache locks work by allowing multiple threads or processes to access cached data in a controlled and synchronized manner. The goal of cache locks is to ensure that only one thread or process can modify cached data at a time, preventing data corruption and ensuring data consistency.
Here’s how cache locks typically work:
- Acquiring a Lock: When a thread or process wants to access or modify cached data, it first tries to acquire a lock on the data. The lock can be a read lock, write lock, or another type of lock depending on the access requirements.
- Checking Lock Status: Before acquiring a lock, the thread checks the status of the lock to see if it is available. If the lock is not available (i.e., another thread has already acquired it), the thread may wait (block) until the lock becomes available or take some other action based on the lock’s semantics (e.g., retrying the operation later).
- Acquiring the Lock: If the lock is available, the thread acquires the lock, allowing it to access or modify the cached data. The thread holds the lock until it completes its operation and releases the lock.
- Releasing the Lock: Once the thread has finished accessing or modifying the cached data, it releases the lock, allowing other threads to acquire the lock and access the data.
- Ensuring Data Consistency: By controlling access to cached data through locks, cache locks ensure data consistency and prevent data corruption. Only one thread can modify the data at a time, ensuring that changes are applied in a controlled and synchronized manner.
Cache locks are essential for managing concurrency in caching systems, especially in multi-threaded or distributed environments. They help prevent race conditions, ensure data integrity, and maintain consistency between the cache and the underlying data source. However, improper use of cache locks can lead to performance issues such as lock contention, so it’s important to carefully design and implement cache locking mechanisms based on the specific requirements of the system.
Example of Cache Locks?
Problem Statement:
You are designing a multi-processor system with shared memory. Each processor has its cache, and the system needs to ensure cache coherence to maintain data consistency across caches.
You decide to use a locking mechanism to coordinate access to shared data and prevent conflicts between processors.
How Cache Locks Will Help:
Cache locks will help ensure that only one thread can modify the shared account balance at a time, preventing conflicts and maintaining data integrity. By acquiring a lock before updating the balance and releasing it afterward, you can serialize write operations and prevent data corruption.
Java
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class CacheExample {
private static int sharedValue = 0;
private static Lock lock = new ReentrantLock();
public static void main(String[] args) {
Thread processorA = new Thread(() -> {
lock.lock();
try {
// Read operation
System.out.println("Processor A reads shared value: " + sharedValue);
} finally {
lock.unlock();
}
});
Thread processorB = new Thread(() -> {
lock.lock();
try {
// Write operation
sharedValue = 10;
System.out.println("Processor B writes shared value: " + sharedValue);
} finally {
lock.unlock();
}
});
processorA.start();
processorB.start();
}
}
Explanation of the above Code:
- The
Account
class represents a shared account with a balance that can be deposited to or withdrawn from. - The
deposit
method acquires the lock, updates the balance, and then releases the lock. - The
withdraw
method acquires the lock, checks if there are sufficient funds, updates the balance if possible, and then releases the lock. - The
getBalance
method returns the current balance without modifying it. - The
ReentrantLock
is used to provide reentrant locking, allowing the same thread to acquire the lock multiple times.
By using a ReentrantLock
to manage access to the balance
variable, you can ensure that only one thread can modify the balance at a time, preventing data corruption and maintaining data integrity
Benefits of Cache Locks
Below are the benefits of Cache Locks:
- Concurrency Control: Cache locks help prevent race conditions and ensure that multiple threads or processes accessing the cache do not interfere with each other. By locking access to a cached item, only one thread can modify the item at a time, ensuring data integrity.
- Consistency: Cache locks help maintain consistency between the cache and the underlying data source. When a cached item is locked for modification, other threads or processes can still read the item but cannot modify it until the lock is released. This helps prevent inconsistent data from being read or written to the cache.
- Prevention of Cache Staleness: Cache locks can help prevent cache staleness by ensuring that only one thread or process updates a cached item at a time. This reduces the likelihood of multiple threads updating the same item with potentially conflicting data.
- Improved Performance: While cache locks can introduce some overhead due to locking and unlocking operations, they can improve overall system performance by ensuring that data integrity is maintained and preventing excessive cache misses.
- Support for Transactional Operations: Cache locks can support transactional operations by allowing a group of cache operations to be performed atomically. This ensures that either all operations in the transaction are completed successfully or none of them are.
Challenges of Cache Locks
Below are the challenges of Cache Locks:
- Deadlocks: One of the primary challenges of cache locks is the potential for deadlocks. Deadlocks occur when two or more threads or processes are waiting for each other to release a lock, preventing them from progressing. Designing cache lock mechanisms to avoid deadlocks requires careful consideration of lock ordering and timeout mechanisms.
- Lock Contention: Cache locks can introduce lock contention, where multiple threads or processes compete for the same lock. This can lead to performance issues, as threads may be blocked waiting for access to a locked resource. Strategies such as fine-grained locking or lock-free data structures can help mitigate lock contention.
- Overhead: Implementing cache locks incurs overhead due to the need to acquire, release, and manage locks. This overhead can impact the overall performance of the system, especially in high-concurrency environments. Optimizing lock granularity and usage can help reduce this overhead.
- Complexity: Managing cache locks adds complexity to the system, especially in distributed or multi-threaded environments. Developers need to carefully design and implement lock mechanisms to ensure data consistency and avoid race conditions, which can be challenging and error-prone.
Conclusion
In conclusion, cache locks, or cache coherence mechanisms, are essential components in the design of multi-core and multi-processor systems to ensure data consistency and prevent race conditions. They play a crucial role in maintaining program correctness and preventing data corruption when multiple processors or cores share access to the same data.
Share your thoughts in the comments
Please Login to comment...