Using Python Environment Variables with Python Dotenv
Last Updated :
19 Mar, 2024
Python dotenv is a powerful tool that makes it easy to handle environment variables in Python applications from start to finish. It lets you easily load configuration settings from a special file (usually named .env) instead of hardcoding them. This makes your code more secure, easier to manage, and better organized. In this article, we’ll see over the most important parts of Python dotenv and show you how to use it effectively through examples.
What Is the Use of Python Dotenv?
Below are the uses of the Python dotenv module in Python:
- Configuration Management: Dotenv helps manage these settings by storing them in a special file (usually called .env). This keeps your code tidy and makes it easier to handle different settings for different situations.
- Security Measures: Dotenv lets you keep sensitive information, like passwords or API keys, in a separate file. This file isn’t shared with others through tools like Git, so your secrets stay safe.
- Consistency Across Environments: Using Dotenv ensures that your program behaves consistently, no matter where it’s running. This is especially helpful for teams working on the same project with different setups, as it prevents problems caused by different settings
Python Dotenv Examples and Uses
Below are the examples of Python Dotenv in Python:
Installation of Python Dotenv Module
Install the Python Dotenv library by running the following command in your terminal or integrated terminal within your Python IDE.
pip install python-dotenv
.env file
SECRET_KEY=mysecretkey
DATABASE_URL=postgres://user:password@localhost/db
API_KEY=your-api-key
DEBUG=True
File Structure
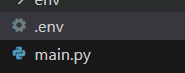
File Structure
Example 1: Environment Variable Handling Using Dotenv
main.py : In this example, the Python script is utilizing Python-Dotenv to load environment variables, specifically SECRET_KEY and DATABASE_URL, from a .env file. The script then prints the values of these variables, showcasing a basic usage scenario. This practice enhances security by keeping sensitive information external to the code and allows for easy configuration management.
Python3
# Import the necessary module
from dotenv import load_dotenv
import os
# Load environment variables from the .env file (if present)
load_dotenv()
# Access environment variables as if they came from the actual environment
SECRET_KEY = os.getenv('SECRET_KEY')
DATABASE_URL = os.getenv('DATABASE_URL')
# Example usage
print(f'SECRET_KEY: {SECRET_KEY}')
print(f'DATABASE_URL: {DATABASE_URL}')
Output:
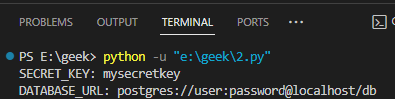
Example 2: Flask App with Dotenv For Environment Variable Management
main.py : Below Flask app imports necessary modules, including Flask and dotenv for managing environment variables. It loads environment variables from a .env file using load_dotenv()
. The app defines a route ‘/’ that returns a message including a secret key retrieved from the environment variables. If executed directly, the app runs.
Python3
# app.py
from flask import Flask
from dotenv import load_dotenv
import os
load_dotenv()
app = Flask(__name__)
@app.route('/')
def hello():
secret_key = os.getenv('SECRET_KEY')
return f'Hello, Flask! Secret Key: {secret_key}'
if __name__ == '__main__':
app.run()
Output:
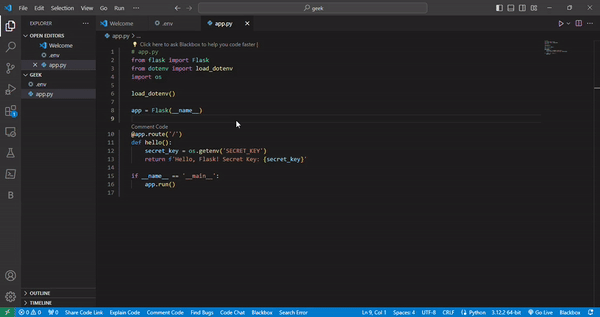
Conclusion
In conclusion, Python-dotenv is a powerful tool for Python developers that makes it easy to work with environment variables. It streamlines the process of loading configuration settings from environment variables, securely storing sensitive data, and managing complex configurations. Examples are included to show how Python-dotenv can be used in different situations to improve the flexibility and security of your projects.
Share your thoughts in the comments
Please Login to comment...