Upload File With Selenium in Python
Last Updated :
01 Feb, 2024
Uploading files using Selenium in Python allows developers to automate the process of interacting with file input elements on web pages. Leveraging the Selenium WebDriver, this tutorial guides users through the steps of locating file input elements, providing the file path for upload, and handling the submission process.
What is Selenium?
Selenium is an open-source framework designed for automating web browsers. It provides a suite of tools for controlling and interacting with web browsers through programs written in various programming languages. Selenium supports multiple web browsers, including Chrome, Firefox, Safari, and Edge, making it versatile for cross-browser testing.
How To Upload File With Selenium?
Below, is the Guide to How To Upload File with Selenium ().
Create a Virtual Environment
First, create the virtual environment using the below commands
python -m venv env
.\env\Scripts\activate.ps1
Install the Selenium
To begin using Selenium, the first step is to install the Selenium package. This can be achieved by running the following command in the terminal:
pip install selenium
Implement the Logic
In this example , below Python code uses Selenium to automate file uploading on a web page. The fileUploader
function initializes a Chrome WebDriver, navigates to a specified URL, and uploads a file by locating the relevant input elements. It simulates user interactions, such as entering file paths and submitting the form. Upon successful upload, it prints a confirmation message, waits for 5 seconds, and closes the WebDriver. Any runtime errors during the process trigger an error message. The script demonstrates a practical approach to automating file uploads using Selenium.
Python3
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
def fileUploader(url):
dr = webdriver.Chrome()
try :
dr.get(url)
file = dr.find_element(By.NAME, "upfile" )
file .send_keys(r 'YOUR/FILE/PATH' )
fileNotes = dr.find_element(By.NAME, "note" )
fileNotes.send_keys( "A file for testing purpose" )
submit = dr.find_element(By.CSS_SELECTOR, 'input[type="submit"]' )
submit.click()
print ( "File Uploaded Successfully" )
time.sleep( 5 )
dr.quit()
except RuntimeError as e:
print ( "An error occurred while fetching and uploading the file {}" . format (e))
if __name__ = = "__main__" :
fileUploader(url)
|
Run the Server
Run the below command for run the server .
python script_name.py
Output
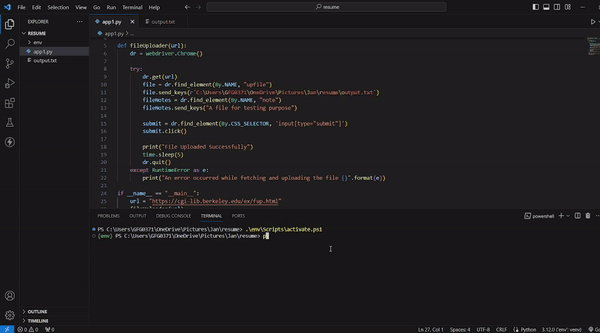
Share your thoughts in the comments
Please Login to comment...