Upload and Read Excel File in Flask
Last Updated :
10 Jan, 2023
In this article, we will look at how to read an Excel file in Flask. We will use the Python Pandas library to parse this excel data as HTML to make our job easier. Pandas additionally depend on openpyxl library to process Excel file formats. Before we begin, make sure that you have installed both Flask and Pandas libraries along with the openpyxl dependency.
Required Module:
pip install flask==2.2.2
pip install pandas==1.4.2
pip install openpyxl==3.0.10
File Structure
Please make sure that you name the HTML file as ‘upload-excel.html’ and place it in the ‘templates’ folder within the root directory of the application.
HTML Template
Create the HTML template which will render the user interface to view the Browse and Upload options for the file. We have allowed uploading of MS Excel and Open XML formats only through the accepted attribute of the input tag.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< title >Upload/View Excel</ title >
</ head >
< body >
< h1 >Upload Excel File (.xlsx)</ h1 >
< form action = "{{ url_for('view') }}" method = "post" enctype = "multipart/form-data" >
< input type = "file" name = "file"
accept = "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet, application/vnd.ms-excel" >
< input type = "submit" value = "Upload" >
</ form >
</ body >
</ html >
|
Upload and Read Excel File in Flask
Step 1: Upload Excel File in Flask
Import the required module
Python3
import pandas from flask import Flask, render_template, request
|
Step 2: Read Excel File in Flask
This Function returns the index page to upload the Excel file.
Python3
@app .get( '/' )
def upload():
return render_template( 'upload-excel.html' )
|
Step 3:
Here, we have created the Flask App which will contain two endpoints. One endpoint will handle a GET request from the root URL. This will return the HTML template which contains the option to upload files. The other endpoint is ‘/view’ which handles POST requests. This will read the upload file and return the HTML snippet to view the uploaded Excel in tabular format in the browser window. Also, the file will save in your local directory using the function save().
Python3
import pandas
from flask import Flask, render_template, request
from fileinput import filename
app = Flask(__name__)
@app .get( '/' )
def upload():
return render_template( 'upload-excel.html' )
@app .post( '/view' )
def view():
file = request.files[ 'file' ]
file .save( file .filename)
data = pandas.read_excel( file )
return data.to_html()
if __name__ = = '__main__' :
app.run(debug = True )
|
python main.py
Output:
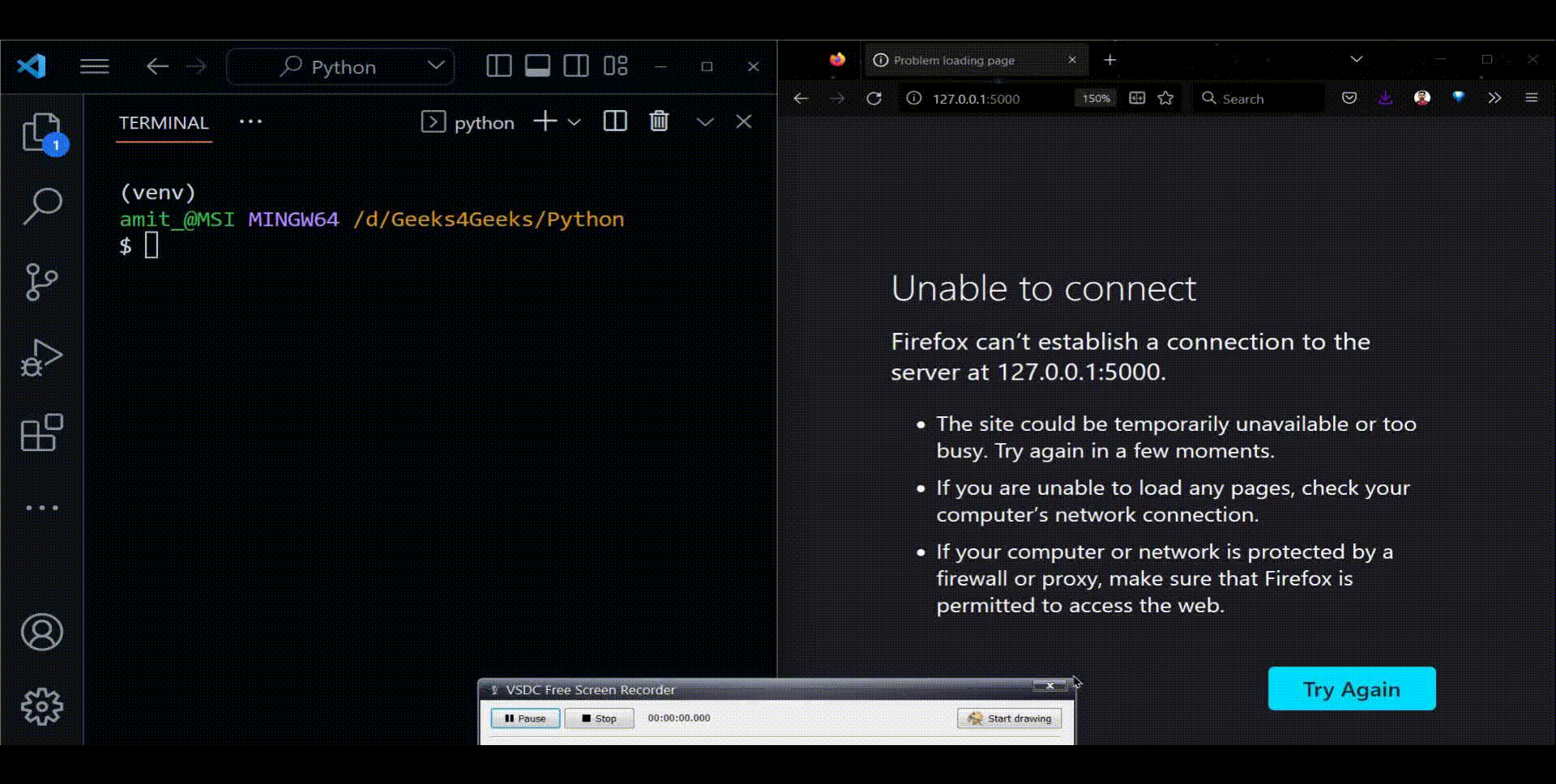
Output
Share your thoughts in the comments
Please Login to comment...