TypeScript Working with Union Types
Last Updated :
31 Oct, 2023
In this article, we are going to learn about Working with Union Types in Typescript. TypeScript is a popular programming language used for building scalable and robust applications. In Typescript, the compiler will only allow an operation if it is valid for every member of the union.
For example, if you have the union string | number, you can’t use methods that are only available on a string or only available on a number. To handle this problem, we need to use type guards/ type assertions to narrow down the type within the union before performing the operation.
Problems Arise While using the Union Type
Suppose, a function takes a parameter which is a union type, and wants to perform an operation that is specific to one of the types in the union. TypeScript may not allow this operation because it needs to ensure that the operation is valid for all possible types within the union.
Example: In this example, TypeScript raises an error because the length property is specific to strings, and it cannot guarantee that input is always a string when working with the union type string | number.
Javascript
function printLength(input: string | number) {
console.log(input.length);
}
|
Output:
Solution of the Above Problem
To address this issue, we can use type assertions or type guards to narrow down the type within the union before performing the operation.
Example: We ae using typeof to do the narrowing for above example code.
Javascript
function printLength(input: string | number) {
if ( typeof input === 'string' ) {
console.log(input.length);
} else {
console.log( 'Not a string' );
}
}
printLength( "GeeksforGeeks" )
|
Output:
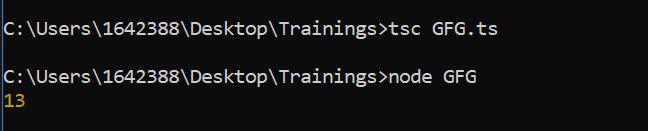
Example 2: Sometimes you’ll have a union where all the members have something in common. For example, both arrays and strings have a slice method. If every member in a union has a property in common, you can use that property without narrowing:
Javascript
function getFirstFour(x: number[] | string) {
return x.slice(0, 4);
}
console.log(getFirstFour( "GeeksforGeeks" ))
|
Output:
Share your thoughts in the comments
Please Login to comment...