TypeScript Object Extending Types
Last Updated :
18 Oct, 2023
In this article, we are going to learn about Object Extending Types in Typescript. In TypeScript, you can extend object types and interfaces using the extends keyword. This allows you to create new object types or interfaces that inherit properties and methods from one or more base types, including other interfaces. Extending interfaces is a fundamental concept in TypeScript, enabling you to compose complex and reusable type definitions.
Syntax
interface BaseInterface {
// Properties and methods of the base interface
}
interface ExtendedInterface extends BaseInterface {
// Additional properties and methods specific to the extended interface
}
Where,
- BaseInterface: This is the existing interface that serves as the base or parent interface. It contains properties and methods that you want to inherit in the extended interface.
- ExtendedInterface: This is the new interface that extends the base interface. It includes additional properties and methods that are specific to the extended interface
Example 1: In this example, We define a base interface called Person with properties name and age. We create a new interface called Employee that extends the Person interface using extends. This means that the Employee inherits the property’s name and age from Person. We can now create objects of type Employee that have the properties defined in both Employee and Person.
Javascript
interface Person {
name: string;
age: number;
}
interface Employee extends Person {
jobTitle: string;
}
const employee: Employee = {
name: "GeeksforGeeks" ,
age: 30,
jobTitle: "Manager" ,
};
console.log(employee)
|
Output:

Example 2: In this example, We have two base interfaces, Person and Address, each defining their own set of properties. The Employee interface extends both Person and Address using extends. This means Employee inherits all properties from Person and Address in addition to its own jobTitle property. We create an employee object of type Employee that includes properties from all three interfaces. When we log the employee object, it will contain properties from Person, Address, and Employee.
Javascript
interface Person {
name: string;
age: number;
}
interface Address {
address: string;
city: string;
}
interface Employee extends Person, Address {
jobTitle: string;
}
const employee: Employee = {
name: "GeeksforGeeks" ,
age: 30,
address: "A-143, 9th Floor, Sovereign Corporate Tower" ,
city: "Noida" ,
jobTitle: "Manager" ,
};
console.log(employee);
|
Output:
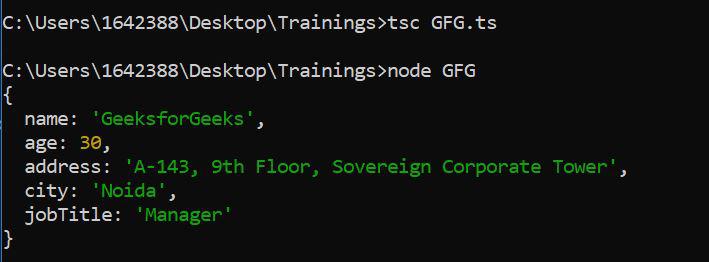
Reference: https://www.typescriptlang.org/docs/handbook/2/objects.html#extending-types
Share your thoughts in the comments
Please Login to comment...