TypeScript Intrinsic String Manipulation Type
Last Updated :
25 Oct, 2023
In this article, we are going to learn about Intrinsic String Manipulation Types in Typescript. TypeScript is a popular programming language used for building scalable and robust applications. In TypeScript, you can use intrinsic string manipulation types to transform string literal types to uppercase, lowercase, capitalize the first letter, or uncapitalize (lowercase the first letter). These string manipulation types are part of TypeScript’s template literal types and can be helpful for enforcing proper string formatting at the type level.
These are the following types of intrinsic string manipulation:
Syntax:
type UppercaseString = Uppercase<"stringliteral">; // for upper case
type LowercaseString = Lowercase<"StringLiteral">; // for lowercase
type CapitalizedString = Capitalize<"stringliteral">; // for capitalize
type UncapitalizedString = Uncapitalize<"StringLiteral">; // for uncapitalize
where–
- Uppercase, Lowercase, Capitalize, and Uncapitalize are the intrinsic string manipulation types.
- “stringliteral” and “StringLiteral” are examples of string literal types to which these transformations are applied.
Uppercase<StringType> Type
It helps to convert each character in the string to an uppercase equivalent.
type UppercaseString = Uppercase<"geeksforgeeks">; // "GEEKSFORGEEKS"
Example: In this example, we will see an example of using Uppercase<StringType> Template Literal. Uppercase will convert each character in the string to a uppercase equivalent resulting in GEEKSFORGEEKS
Javascript
type gfg = "Geeksforgeeks"
type geek = Uppercase<gfg>
const result: geek = "GEEKSFORGEEKS" ;
console.log(result)
|
Output:
Uncapitalize<StringType> Type
It helps to convert the first character in the string to a lowercase equivalent.
type UncapitalizedString = Uncapitalize<"Geeksforgeeks">; // "geeksforgeeks"
Example: In this example, we will see an example of using Uncapitalize<StringType> Template Literal. Uncapitalize will convert the first character in the string to a lowercase equivalent resulting in geeksforgeeks
Javascript
type gfg = "Geeksforgeeks"
type geek = Uncapitalize<gfg>
const result: geek = "geeksforgeeks" ;
console.log(result)
|
Output:

Lowercase<StringType> Type
It helps to convert each character in the string to a lowercase equivalent.
type LowercaseString = Lowercase<"GEEKSFORGEEKS">; // "geeksforgeeks"
Example: In this example, we will see an example of using Lowercase<StringType> Template Literal. Uppercase will convert each character in the string to a lowercase equivalent resulting in geeksforgeeks
Javascript
type gfg = "GEEKSFORGEEKS"
type geek = Lowercase<gfg>
const result: geek = "geeksforgeeks" ;
console.log(result)
|
Output:

Capitalize<StringType> Type
It helps to convert the first character in the string to a uppercase equivalent.
type CapitalizedString = Capitalize<"geeksforgeeks">; // "Geeksforgeeks"
Example: In this example, we will see an example of using Capitalize<StringType> Template Literal. Uncapitalize will convert the first character in the string to a uppercase equivalent resulting in Geeksforgeeks
Javascript
type gfg = "geeksforgeeks"
type geek = Capitalize<gfg>
const result: geek = "Geeksforgeeks" ;
console.log(result)
|
Output:
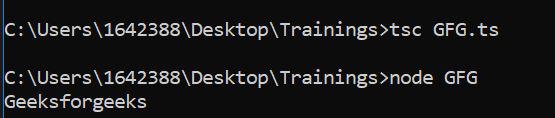
Share your thoughts in the comments
Please Login to comment...