Types of Heap Data Structure
Last Updated :
23 Jan, 2024
Different types of heap data structures include fundamental types like min heap and max heap, binary heap and many more. In this post, we will look into their characteristics, and their use cases. Understanding the characteristics and use cases of these heap data structures helps in choosing the most suitable one for a particular algorithm or application. Each type of heap has its own advantages and trade-offs, and the choice depends on the specific requirements of the problem at hand.
1. Binary Heap:
Binary heap is the fundamental type of heap that forms the basis for many other heap structures. It is a complete binary tree with a unique property – the value of each node is either greater than or equal to (max-heap) or less than or equal to (min-heap) the values of its children. Binary heaps are often implemented as arrays, allowing for efficient manipulation and access.
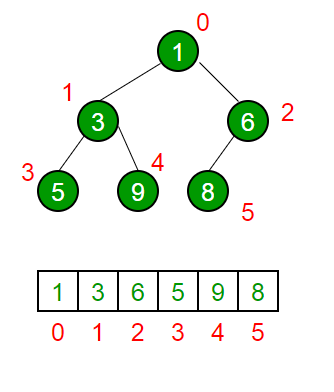
- Characteristics:
- Efficiently represented as an array.
- Parent’s value is greater than or equal to (max heap) or less than or equal to (min heap) children’s values.
- Uses:
- Priority queues.
- Heap sort algorithm.
- Applications:
- Dijkstra’s shortest path algorithm.
- Huffman coding for data compression.
Heap Sort |
Sort an array using binary heap operations. |
Priority Queue Operations |
Implement basic operations like insertion and extraction in a binary heap. |
Kth Smallest Element |
Find the Kth smallest element in an array efficiently using a binary heap. |
Merge Two Heaps |
Efficiently merge two binary heaps into a single heap. |
Heapify an Array |
Convert an array into a binary heap efficiently. |
2. Min Heap:
Min heap is a specific instance of a binary heap where the value of each node is less than or equal to the values of its children. Min heaps are particularly useful when the goal is to efficiently find and extract the minimum element from a collection.
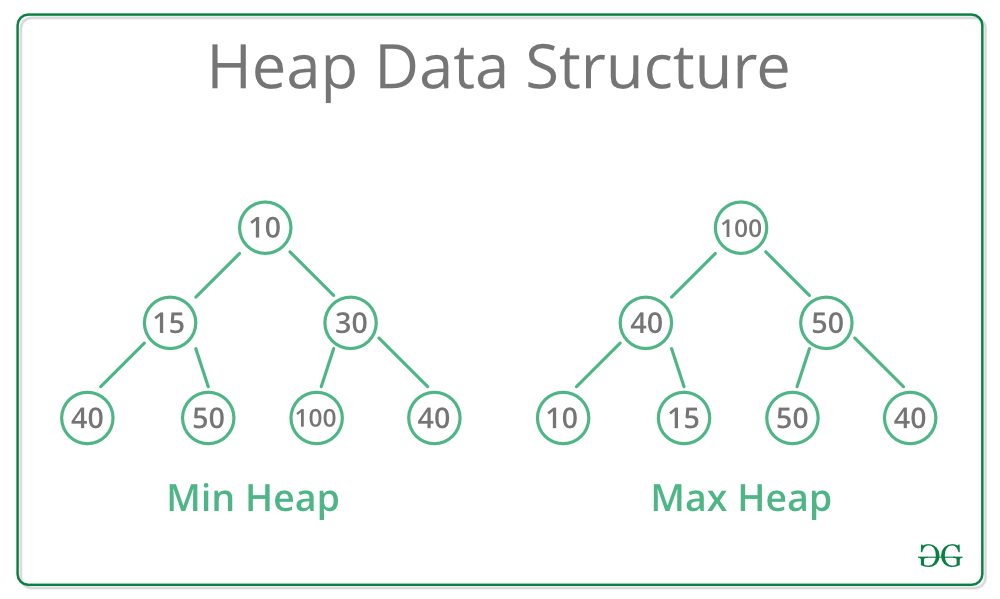
- Characteristics:
- Parent’s value is less than or equal to children’s values.
- Uses:
- Finding and extracting the minimum element efficiently.
- Applications:
- Prim’s minimum spanning tree algorithm.
- Efficiently solving the “k smallest/largest elements” problem.
Extract Minimum Element |
Implement the extraction of the minimum element in a min heap. |
Merge Two Min Heaps |
Efficiently merge two min heaps into a single min heap. |
Dijkstra’s Shortest Path Algorithm |
Use a min heap to implement Dijkstra’s algorithm for finding the shortest paths in a graph. |
Prim’s Minimum Spanning Tree |
Implement Prim’s algorithm using a min heap to find a minimum spanning tree in a graph. |
Running Median |
Maintain the median of a stream of numbers efficiently using a min heap. |
3. Max Heap:
Max heap, on the other hand, is a binary heap where the value of each node is greater than or equal to the values of its children. Max heaps are valuable when the objective is to efficiently find and extract the maximum element from a collection.
- Characteristics:
- Parent’s value is greater than or equal to children’s values.
- Uses:
- Finding and extracting the maximum element efficiently.
- Applications:
- Efficiently solving the “k smallest/largest elements” problem.
Extract Maximum Element |
Implement the extraction of the maximum element in a max heap. |
Merge Two Max Heaps |
Efficiently merge two max heaps into a single max heap. |
Kth Largest Element |
Find the Kth largest element in an array efficiently using a max heap. |
Running Median |
Maintain the median of a stream of numbers efficiently using a max heap. |
Heap Sort |
Sort an array using max heap operations. |
4. Binomial Heap:
Binomial heap extends the concept of binary heap by introducing the idea of binomial trees. A binomial tree of order k has 2^k nodes. Binomial heaps are known for their efficient merge operations, making them valuable in certain algorithmic scenarios.
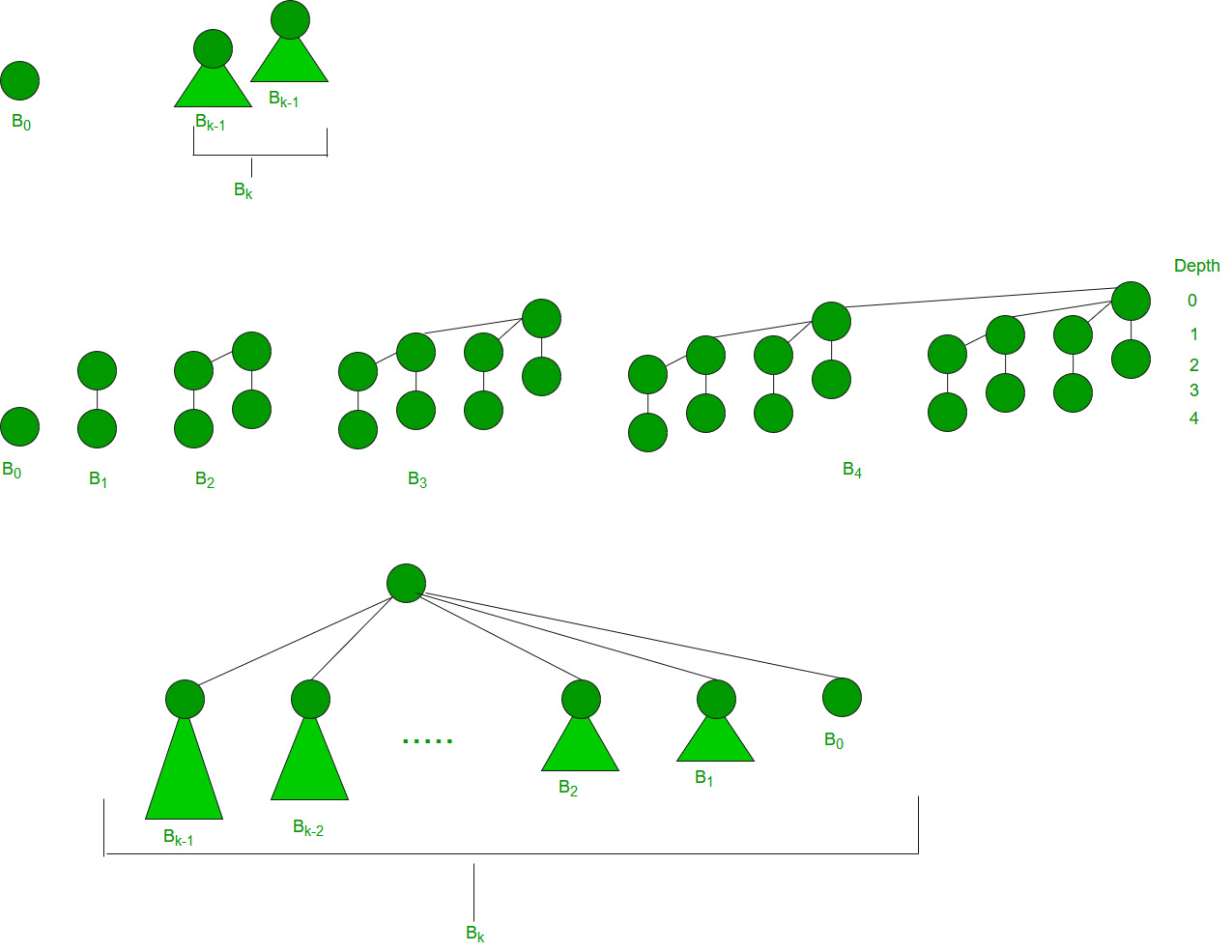
- Characteristics:
- Composed of a collection of binomial trees.
- Uses:
- Mergeable priority queues.
- Applications:
- Efficiently merging sorted sequences.
- Implementing graph algorithms.
Merge Two Binomial Heaps |
Efficiently merge two binomial heaps into a single binomial heap. |
Extract Minimum Element |
Implement the extraction of the minimum element in a binomial heap. |
Decrease Key Operation |
Implement the decrease key operation efficiently in a binomial heap. |
Priority Queue with Decrease Key |
Implement a priority queue supporting decrease key efficiently using a binomial heap. |
Convert Array to Binomial Heap |
Convert an array into a binomial heap efficiently. |
5. Fibonacci Heap:
Fibonacci heap is a more advanced data structure that boasts better amortized time complexity for certain operations compared to traditional binary and binomial heaps. It comprises a collection of trees with a specific structure and excels in decrease key and merge operations.
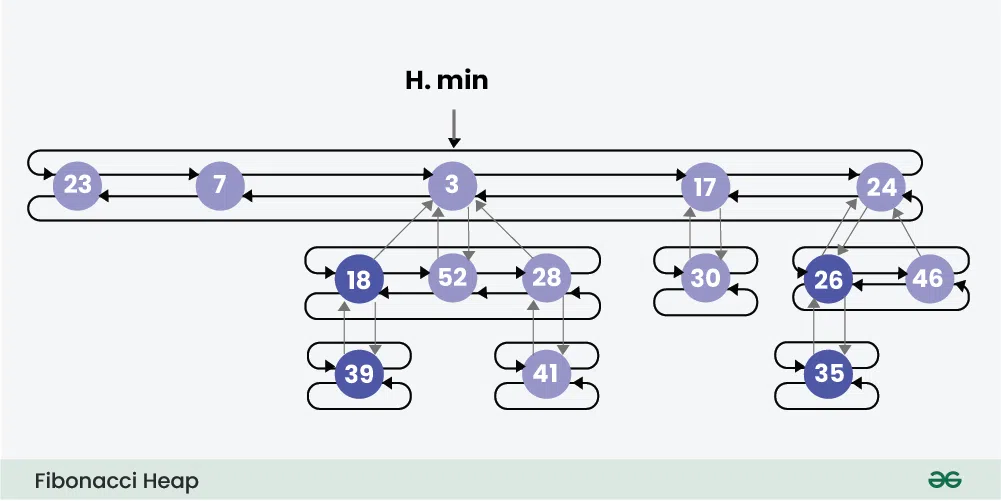
- Characteristics:
- Collection of trees with specific properties.
- Uses:
- Decrease key and merge operations efficiently.
- Applications:
- Prim’s and Kruskal’s algorithms for minimum spanning trees.
- Dijkstra’s shortest path algorithm.
Decrease Key Operation |
Implement the decrease key operation efficiently in a Fibonacci heap. |
Merge Two Fibonacci Heaps |
Efficiently merge two Fibonacci heaps into a single Fibonacci heap. |
Dijkstra’s Shortest Path Algorithm |
Use a Fibonacci heap to implement Dijkstra’s algorithm for finding shortest paths. |
Prim’s Minimum Spanning Tree |
Implement Prim’s algorithm using a Fibonacci heap to find a minimum spanning tree. |
Extract Minimum Element |
Implement the extraction of the minimum element in a Fibonacci heap. |
6. D-ary Heap:
D-ary heap is a generalization of the binary heap, allowing each node to have D children instead of 2. The value of D determines the arity of the heap, with binary heap being a special case (D=2).
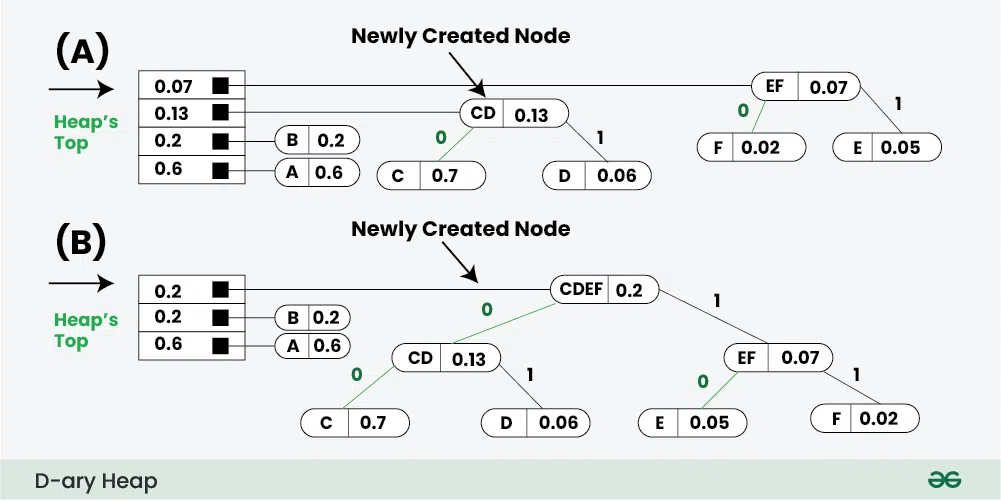
- Characteristics:
- Generalization of binary heap with D children per node.
- Uses:
- Varying arity based on specific needs.
- Applications:
- D-ary heaps can be tailored for specific applications requiring a particular arity.
Decrease Key Operation |
Implement the decrease key operation efficiently in a Fibonacci heap. |
Merge Two Fibonacci Heaps |
Efficiently merge two Fibonacci heaps into a single Fibonacci heap. |
Dijkstra’s Shortest Path Algorithm |
Use a Fibonacci heap to implement Dijkstra’s algorithm for finding shortest paths. |
Prim’s Minimum Spanning Tree |
Implement Prim’s algorithm using a Fibonacci heap to find a minimum spanning tree. |
Extract Minimum Element |
Implement the extraction of the minimum element in a Fibonacci heap. |
7. Pairing Heap:
Pairing heap is a simpler alternative to Fibonacci heap while still maintaining efficient time bounds for key operations. Its structure is based on a pairing mechanism, making it a suitable choice for various applications.
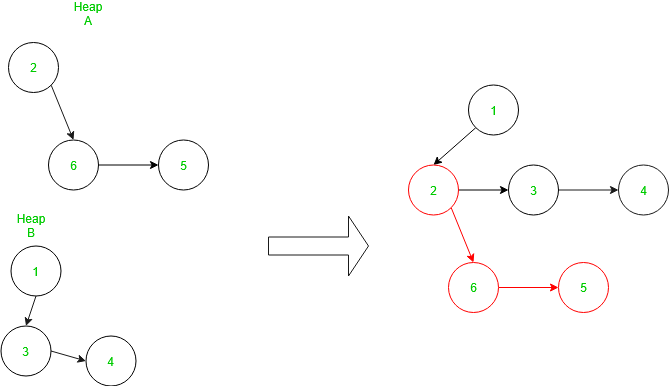
- Characteristics:
- Trees are paired together during the merge operation.
- Uses:
- Simplicity with reasonable time bounds.
- Applications:
- Network optimization problems.
- Union-find data structure.
Decrease Key Operation |
Implement the decrease key operation efficiently in a Fibonacci heap. |
Merge Two Fibonacci Heaps |
Efficiently merge two Fibonacci heaps into a single Fibonacci heap. |
Dijkstra’s Shortest Path Algorithm |
Use a Fibonacci heap to implement Dijkstra’s algorithm for finding shortest paths. |
Prim’s Minimum Spanning Tree |
Implement Prim’s algorithm using a Fibonacci heap to find a minimum spanning tree. |
Extract Minimum Element |
Implement the extraction of the minimum element in a Fibonacci heap. |
8. Leftist Heap:
Leftist heap is a variant of the binary heap that introduces a “null path length” property. This property aids in efficiently maintaining the heap structure during merge operations.
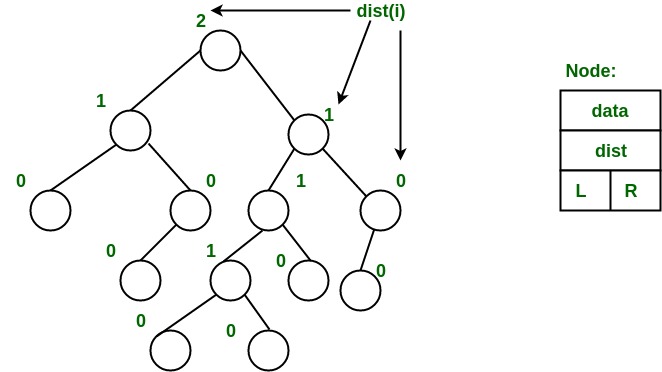
- Characteristics:
- Maintains a “null path length” property.
- Uses:
- Efficient merge operations.
- Applications:
- Mergeable priority queues.
- Huffman coding.
Merge Two Leftist Heaps |
Efficiently merge two leftist heaps into a single leftist heap. |
Priority Queue Operations (Leftist Heap) |
Implement basic operations like insertion and extraction in a leftist heap. |
Convert Array to Leftist Heap |
Convert an array into a leftist heap efficiently. |
Delete Operation (Leftist Heap) |
Implement the delete operation in a leftist heap. |
Shortest Path Algorithms (Leftist Heap) |
Use a leftist heap to implement efficient shortest path algorithms. |
9. Skew Heap:
Skew heap is another variation of the binary heap, employing a specific skew-link operation to combine two heaps. It provides an interesting approach to heap management.
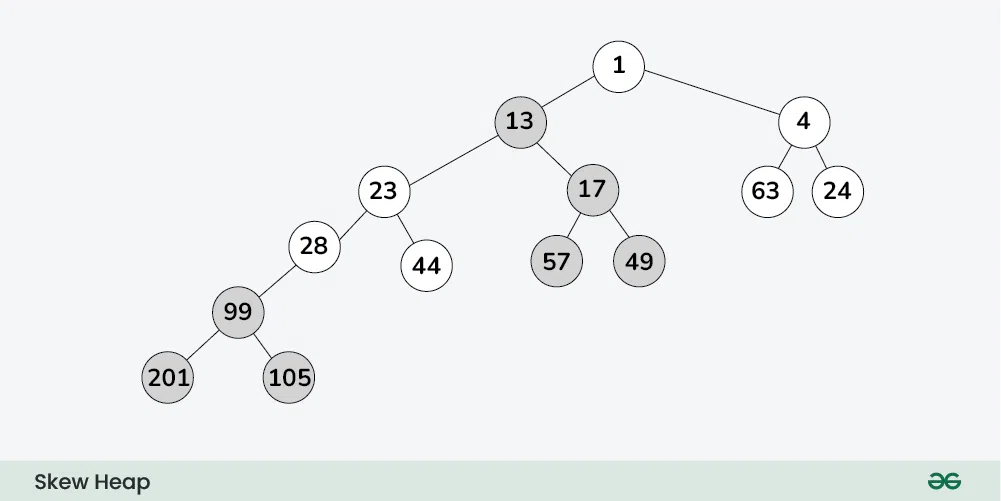
- Characteristics:
- Uses skew-link operation for merging.
- Uses:
- Simplicity with reasonable time bounds.
- Applications:
- Priority queue operations.
Merge Two Skew Heaps |
Efficiently merge two skew heaps into a single skew heap. |
Priority Queue Operations (Skew Heap) |
Implement basic operations like insertion and extraction in a skew heap. |
Convert Array to Skew Heap |
Convert an array into a skew heap efficiently. |
Delete Operation (Skew Heap) |
Implement the delete operation in a skew heap. |
Shortest Path Algorithms (Skew Heap) |
Use a skew heap to implement efficient shortest path algorithms. |
10. B-Heap:
B-heap is a generalization of the binary heap, allowing for trees with more than two children at each node. This flexibility in the number of children can be advantageous in certain scenarios.
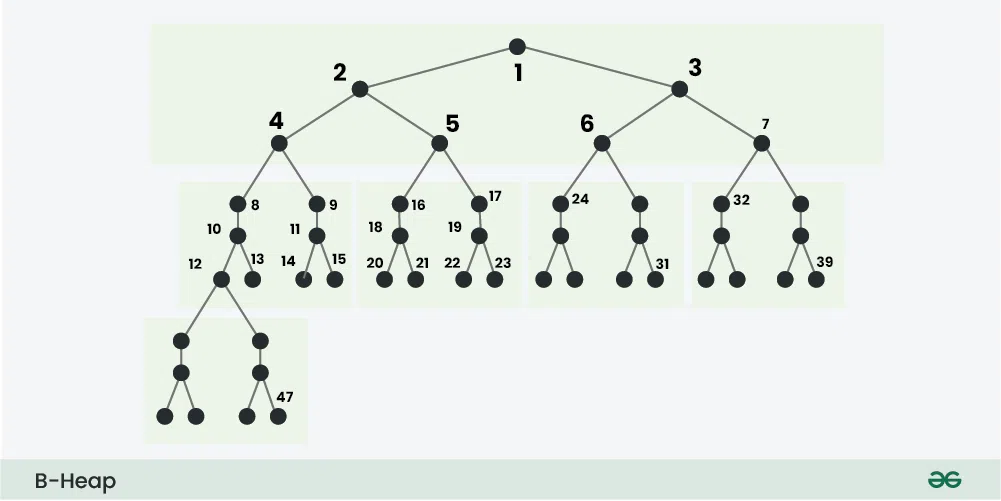
- Characteristics:
- Generalization of binary heap allowing more than two children per node.
- Uses:
- Balanced trees with varying arity.
- Applications:
- Database systems for indexing.
- File systems for efficient storage management.
Decrease Key Operation (B-Heap) |
Implement the decrease key operation efficiently in a B-heap. |
Priority Queue Operations (B-Heap) |
Implement basic operations like insertion and extraction in a B-heap. |
Convert Binary Heap to B-Heap |
Convert a binary heap into a B-heap efficiently. |
Kth Smallest Element (B-Heap) |
Find the Kth smallest element in an array efficiently using a B-heap. |
Mergeable Priority Queue (B-Heap) |
Implement a mergeable priority queue using B-heaps. |
Comparison between different types of Heap:
Here’s a simplified comparison table highlighting key characteristics of different types of Heap data structures:
Min or Max |
Complete Binary Tree |
O(log n) |
O(log n) |
O(1) (for insert) |
Min |
Complete Binary Tree |
O(log n) |
O(log n) |
O(1) (for insert) |
Max |
Complete Binary Tree |
O(log n) |
O(log n) |
O(1) (for insert) |
Min |
Forest of Binomial Trees |
O(1) (amortized) |
O(log n) |
O(log n) |
Min |
Collection of Trees |
O(1) (amortized) |
O(1) (amortized) |
O(1) (amortized) |
Min or Max |
Complete D-ary Tree |
O(log_d n) |
O(log_d n) |
O(1) (for insert) |
Min |
Pairing of Trees |
O(1) (amortized) |
O(1) (amortized) |
O(1) (amortized) |
Min |
Leftist Tree |
O(log n) |
O(log n) |
O(1) (for insert) |
Min or Max |
Skewed Tree |
O(log n) |
O(log n) |
O(1) (for insert) |
Min or Max |
B-ary Tree |
O(log_b n) |
O(log_b n) |
O(1) (for insert) |
Share your thoughts in the comments
Please Login to comment...