Type Casting in Programming
Last Updated :
15 Apr, 2024
In programming, variables hold data of specific types, such as integers, floating-point numbers, strings, and more. These data types determine how the computer interprets and manipulates the information. Type casting becomes necessary when you want to perform operations or assignments involving different data types. In this blog, we will explore type casting, its importance, and the various methods used in different programming languages.
What is Type Casting:
Type casting, or type conversion, is a fundamental concept in programming that involves converting one data type into another. This process is crucial for ensuring compatibility and flexibility within a program.
Types of Type Casting:
There are two main approaches to type casting:
- Implicit or Automatic Type Casting
- Explicit or Manual Type Casting
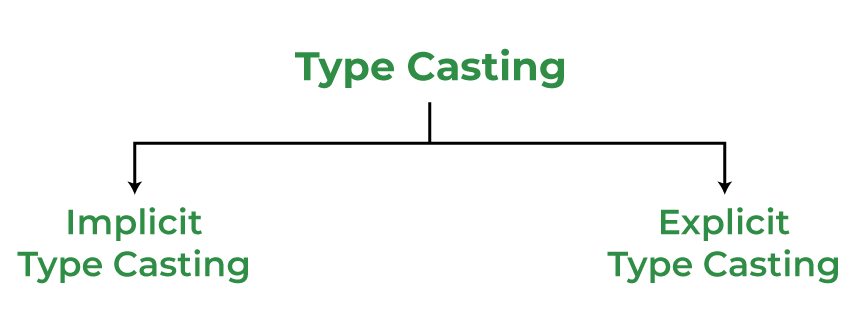
Implicit Type Casting:
Implicit Type Conversion is commonly referred to as ‘Automatic Type Conversion.’ It occurs automatically within the compiler without requiring external intervention from the user. This conversion typically happens when an expression involves more than one data type. In such scenarios, all variable data types involved are elevated to match the data type of the variable with the largest range or precision. This is also known as type promotion.
Integer promotion is the first step performed by the compiler. Subsequently, the compiler assesses whether the two operands in an expression possess different data types. In such cases, the conversion follows a hierarchy to determine the resulting data type. The hierarchy is as follows:
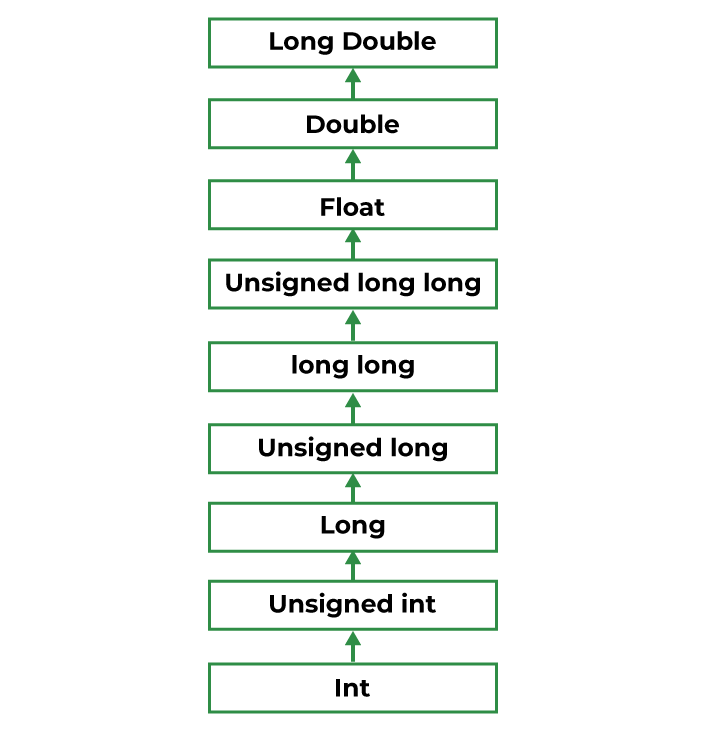
The compiler applies the conversion to match the data type based on this hierarchy, ensuring a consistent and predictable result in expressions with operands of different types.
C++
#include <iostream>
using namespace std;
int main() {
int a = 'A';
// character to integer conversion
cout << a << "\n";
char ch = 65;
// integer to character conversion
cout << ch << "\n";
int x = 1000000;
int y = 1000000;
// Integers are multiplied and leads to overflow
long long z1 = x * y;
// Integer to Long Long conversion
long long z2 = (long long)x * y;
cout << z1 << " " << z2 << "\n";
int numerator = 15;
int denominator = 10;
// Integers are multiplied so result is an integer
int quotient1 = numerator/denominator;
// Integer to float conversion
float quotient2 = (float)numerator/denominator;
cout << quotient1 << " " << quotient2 << endl;
return 0;
}
Java
public class Main {
public static void main(String[] args) {
// Initialize integer variable 'a' with the ASCII value of 'A'
int a = 'A';
// Print the integer value of 'A' (65)
System.out.println(a);
// Initialize character variable 'ch' with ASCII value 65
char ch = 65;
// Print the character corresponding to ASCII value 65 ('A')
System.out.println(ch);
// Initialize integers x and y
int x = 1000000;
int y = 1000000;
// Integers are multiplied and leads to overflow
// Perform the multiplication and store the result in 'z1' (may cause overflow)
long z1 = x * y;
// Perform the multiplication with proper casting to long long (long) to prevent overflow
long z2 = (long)x * y;
// Print the results of both multiplications
System.out.println(z1 + " " + z2);
// Initialize numerator and denominator for division
int numerator = 15;
int denominator = 10;
// Integers are divided so result is an integer
// Perform integer division and store the result in 'quotient1'
int quotient1 = numerator / denominator;
// Perform floating-point division with proper casting to float to get the float quotient
float quotient2 = (float)numerator / denominator;
// Print both integer and float quotients
System.out.println(quotient1 + " " + quotient2);
}
}
Python3
# character to integer conversion
a = ord('A')
print(a)
# integer to character conversion
ch = chr(65)
print(ch)
# Integers are multiplied and may lead to overflow
x = 1000000
y = 1000000
z1 = x * y
# Integer to Long conversion
z2 = int(x) * y
print(z1, z2)
numerator = 15
denominator = 10
# Integers are divided so result is an integer
quotient1 = numerator // denominator
# Integer to float conversion
quotient2 = float(numerator) / denominator
print(quotient1, quotient2)
C#
using System;
public class Program {
public static void Main(string[] args) {
// Initialize integer variable 'a' with the ASCII value of 'A'
int a = 'A';
// Print the integer value of 'A' (65)
Console.WriteLine(a);
// Initialize character variable 'ch' with ASCII value 65
char ch = (char)65;
// Print the character corresponding to ASCII value 65 ('A')
Console.WriteLine(ch);
// Initialize integers x and y
int x = 1000000;
int y = 1000000;
// Integers are multiplied and may lead to overflow
// Perform the multiplication and store the result in 'z1' (may cause overflow)
long z1 = (long)x * y;
// Print the result of multiplication (may cause overflow)
Console.WriteLine(z1);
// Initialize numerator and denominator for division
int numerator = 15;
int denominator = 10;
// Integers are divided so result is an integer
// Perform integer division and store the result in 'quotient1'
int quotient1 = numerator / denominator;
// Perform floating-point division with proper casting to float to get the float quotient
float quotient2 = (float)numerator / denominator;
// Print both integer and float quotients
Console.WriteLine(quotient1 + " " + quotient2);
}
}
Javascript
class Main {
static main() {
// Initialize integer variable 'a' with the ASCII value of 'A'
let a = 'A'.charCodeAt(0);
// Print the integer value of 'A' (65)
console.log(a);
// Initialize character variable 'ch' with ASCII value 65
let ch = String.fromCharCode(65);
// Print the character corresponding to ASCII value 65 ('A')
console.log(ch);
// Initialize integers x and y
let x = 1000000;
let y = 1000000;
// Integers are multiplied and may lead to overflow
// Perform the multiplication and store the result in 'z1' (may cause overflow)
let z1 = x * y;
// Perform the multiplication with proper casting to BigInt to prevent overflow
let z2 = BigInt(x) * BigInt(y);
// Print the results of both multiplications
console.log(z1 + " " + z2);
// Initialize numerator and denominator for division
let numerator = 15;
let denominator = 10;
// Integers are divided so result is an integer
// Perform integer division and store the result in 'quotient1'
let quotient1 = Math.floor(numerator / denominator);
// Perform floating-point division to get the float quotient
let quotient2 = numerator / denominator;
// Print both integer and float quotients
console.log(quotient1 + " " + quotient2);
}
}
Main.main();
Output65
A
-727379968 1000000000000
1 1.5
Explicit Type Casting:
There are some cases where if the datatype remains unchanged, it can give incorrect output. In such cases, typecasting can help to get the correct output and reduce the time of compilation. In explicit type casting, we have to force the conversion between data types. This type of casting is explicitly defined within the program.
C++
// C program to illustrate the use of
// typecasting
#include <stdio.h>
// Driver Code
int main()
{
// Given a & b
int a = 15, b = 2;
float div;
// Division of a and b
div = (float)a / b; // Typecasting int to float
printf("The result is %f\n", div);
return 0;
}
Java
public class Main {
public static void main(String[] args) {
// Given a & b
int a = 15, b = 2;
float div;
// Division of a and b
div = (float) a / b; // Typecasting int to float
System.out.println("The result is " + div);
}
}
Python3
# Function to perform division and print the result
def main():
# Given values of a and b
a = 15
b = 2
# Division of a and b (typecasting int to float for accurate division)
div = float(a) / b
# Printing the result
print("The result is", div)
# Calling the main function
if __name__ == "__main__":
main()
JavaScript
// Given a & b
let a = 15, b = 2;
let div;
// Division of a and b
div = parseFloat(a) / b; // Typecasting int to float
console.log("The result is " + div);
OutputThe result is 7.000000
Difference between Implicit and Explicit Type Casting:
Type Casting | Implicit (Automatic) | Explicit (Manual) |
---|
Definition | Conversion performed by the compiler without programmer’s intervention. | Conversion explicitly specified by the programmer. |
---|
Occurrence | Happens automatically during certain operations or assignments. | Programmer needs to explicitly request the conversion. |
---|
Risk | Generally safe but may lead to loss of precision or unexpected results. | Requires careful handling by the programmer to prevent data loss or errors. |
---|
Syntax | No explicit syntax needed; the conversion is done automatically. | Requires explicit syntax, such as type casting functions or operators. |
---|
Examples:
| int a = 4.5; // float to int conversion
char ch = 97; // int to char conversion
| int a = 5, b = 6; float x = (float) a/b; // int to float
int a = 1000000, b = 1000000; long long x = (long long)a * b; // int to long long
|
---|
Type Compatibility and Safety in Programming:
Type compatibility and safety are fundamental concepts in programming that play a crucial role in ensuring the correctness, reliability, and security of software. In this section, we’ll explore the significance of type compatibility, the challenges associated with type safety, and how programmers can navigate these aspects to create robust and error-resistant code.
Understanding Type Compatibility:
1. Definition:
- Type compatibility refers to the ability of different data types to interact with each other in a meaningful and well-defined manner.
- Compatible types can be used together in operations like assignments, arithmetic, and function calls without causing errors or unexpected behavior.
2. Compatibility Levels:
- Implicit Compatibility: Some languages allow automatic conversion between compatible types without explicit instructions from the programmer. This is known as implicit type casting, and it enhances code flexibility.
- Explicit Compatibility: In languages with stricter type systems, explicit type casting is required for converting between certain types. This provides more control but demands manual intervention.
Challenges in Type Safety:
1. Runtime Errors:
- Incompatible type usage can lead to runtime errors, such as type mismatch errors or data loss during conversions.
- Detecting these errors at runtime can make debugging more challenging.
2. Security Concerns:
- Type safety is closely related to security. Insecure type handling may lead to vulnerabilities like buffer overflows or injection attacks if not properly managed.
3. Maintenance Issues:
- Code that lacks type safety might be more prone to maintenance issues, as changes in one part of the code may unintentionally affect other parts, leading to unexpected behavior.
Ensuring Type Safety:
1. Strong Typing:
- Use languages with strong typing systems that enforce strict type rules at compile-time. This helps catch type-related errors before the program runs.
2. Testing and Validation:
- Implement thorough testing and validation procedures to identify and rectify type-related issues during development.
3. Explicit Type Declarations:
- Explicitly declare variable types whenever possible. This enhances code readability and reduces the likelihood of inadvertent type misuse.
Examples of Type Compatibility:
1. Numeric Compatibility:
- Compatible: Integers and floating-point numbers
- Incompatible: Strings and numeric types
int_num = 5
float_num = 2.5
result = int_num + float_num # Compatibility: int + float
2. String Compatibility:
- Compatible: Strings and characters
- Incompatible: Strings and numeric types
string_var = "Hello"
char_var = 'A'
result = string_var + char_var # Compatibility: string + char
Type Casting between Basic Data Types:
Numeric type casting is a common operation, involving the conversion between integers and floating-point numbers. String to numeric and character to numeric casting also play essential roles in handling diverse data formats.
Casting between basic data types is a common operation in programming, allowing developers to convert values from one data type to another. This process is essential when working with diverse types of data, enabling seamless integration and manipulation. In this section, we’ll explore how casting is performed between basic data types, including numeric types, strings, and characters.
1. Numeric Type Casting:
Integer to Float: Converting an integer to a floating-point number.
int_num = 5 float_num = float(int_num) # Casting integer to float
Float to Integer: Truncating the decimal part of a floating-point number to obtain an integer.
float_num = 7.8int_num = int(float_num) # Casting float to integer (truncation)
Numeric String to Integer or Float: Converting a string containing numeric characters to an integer or a float.
str_num = “10”int_num = int(str_num) # Casting string to integerfloat_num = float(str_num) # Casting string to float
2. String to Numeric Type Casting:
String to Integer: Converting a string representing an integer to an actual integer.
str_num = “42”int_num = int(str_num) # Casting string to integer
String to Float: Converting a string representing a floating-point number to an actual float.
str_float = “3.14”float_num = float(str_float) # Casting string to float
3. Character to Numeric Type Casting:
Character to Integer: Converting a character to its corresponding ASCII value.
char c = ‘A’;int ascii_value = (int)c; // Casting character to integer (ASCII value)
Here’s a simple program that demonstrates casting between basic data types:
C++
#include <iostream>
#include <string>
using namespace std;
int main() {
// Example 1: Integer to Float
int integer_value = 10;
float float_value = static_cast<float>(integer_value);
cout << "Integer to Float: " << integer_value << " -> " << float_value << endl;
// Example 2: Float to Integer
float float_value_2 = 15.5;
int integer_value_2 = static_cast<int>(float_value_2);
cout << "Float to Integer: " << float_value_2 << " -> " << integer_value_2 << endl;
// Example 3: Integer to String
int integer_value_3 = 42;
string string_value = to_string(integer_value_3);
cout << "Integer to String: " << integer_value_3 << " -> '" << string_value << "'" << endl;
// Example 4: String to Integer
string string_value_2 = "123";
int integer_value_4 = stoi(string_value_2);
cout << "String to Integer: '" << string_value_2 << "' -> " << integer_value_4 << endl;
// Example 5: String to Float
string string_value_3 = "3.14";
float float_value_3 = stof(string_value_3);
cout << "String to Float: '" << string_value_3 << "' -> " << float_value_3 << endl;
return 0;
}
Java
import java.util.*;
public class Main {
public static void main(String[] args) {
// Example 1: Integer to Float
int integer_value = 10;
float float_value = (float) integer_value;
System.out.println("Integer to Float: " + integer_value + " -> " + float_value);
// Example 2: Float to Integer
float float_value_2 = 15.5f;
int integer_value_2 = (int) float_value_2;
System.out.println("Float to Integer: " + float_value_2 + " -> " + integer_value_2);
// Example 3: Integer to String
int integer_value_3 = 42;
String string_value = Integer.toString(integer_value_3);
System.out.println("Integer to String: " + integer_value_3 + " -> '" + string_value + "'");
// Example 4: String to Integer
String string_value_2 = "123";
int integer_value_4 = Integer.parseInt(string_value_2);
System.out.println("String to Integer: '" + string_value_2 + "' -> " + integer_value_4);
// Example 5: String to Float
String string_value_3 = "3.14";
float float_value_3 = Float.parseFloat(string_value_3);
System.out.println("String to Float: '" + string_value_3 + "' -> " + float_value_3);
}
}
Python3
# Casting between Basic Data Types
# Example 1: Integer to Float
integer_value = 10
float_value = float(integer_value)
print(f"Integer to Float: {integer_value} -> {float_value}")
# Example 2: Float to Integer
float_value = 15.5
integer_value = int(float_value)
print(f"Float to Integer: {float_value} -> {integer_value}")
# Example 3: Integer to String
integer_value = 42
string_value = str(integer_value)
print(f"Integer to String: {integer_value} -> '{string_value}'")
# Example 4: String to Integer
string_value = "123"
integer_value = int(string_value)
print(f"String to Integer: '{string_value}' -> {integer_value}")
# Example 5: String to Float
string_value = "3.14"
float_value = float(string_value)
print(f"String to Float: '{string_value}' -> {float_value}")
JavaScript
// Example 1: Integer to Float
let integer_value = 10;
let float_value = parseFloat(integer_value);
console.log("Integer to Float:", integer_value, "->", float_value);
// Example 2: Float to Integer
let float_value_2 = 15.5;
let integer_value_2 = parseInt(float_value_2);
console.log("Float to Integer:", float_value_2, "->", integer_value_2);
// Example 3: Integer to String
let integer_value_3 = 42;
let string_value = integer_value_3.toString();
console.log("Integer to String:", integer_value_3, "->", "'" + string_value + "'");
// Example 4: String to Integer
let string_value_2 = "123";
let integer_value_4 = parseInt(string_value_2);
console.log("String to Integer:", "'" + string_value_2 + "'", "->", integer_value_4);
// Example 5: String to Float
let string_value_3 = "3.14";
let float_value_3 = parseFloat(string_value_3);
console.log("String to Float:", "'" + string_value_3 + "'", "->", float_value_3);
OutputInteger to Float: 10 -> 10
Float to Integer: 15.5 -> 15
Integer to String: 42 -> '42'
String to Integer: '123' -> 123
String to Float: '3.14' -> 3.14
Challenges and Best Practices of Type Casting in Programming:
- Loss of Precision: Be cautious about potential loss of precision when converting between numeric types.
- Compatibility: Ensure compatibility between data types to avoid runtime errors.
- Error Handling: Implement robust error handling mechanisms when dealing with user input or external data.
Share your thoughts in the comments
Please Login to comment...