Traversal of Singly Linked List
Last Updated :
18 Apr, 2024
Traversal of Singly Linked List is one of the fundamental operations, where we traverse or visit each node of the linked list. In this article, we will cover how to traverse all the nodes of a singly linked list along with its implementation.
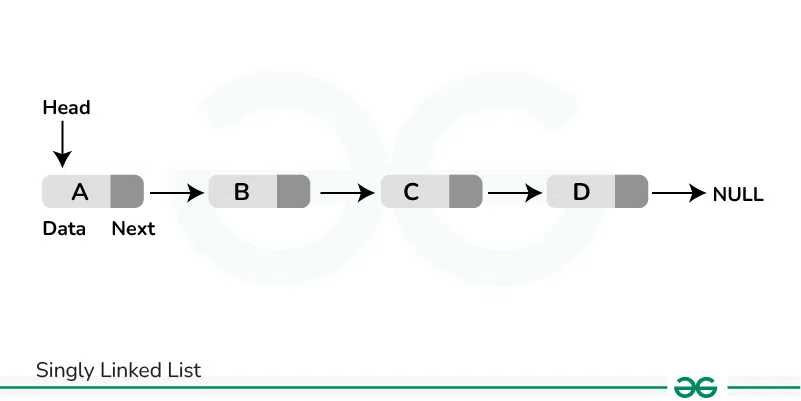
Examples:
Input: 1->2->3->4->5->null
Output: 1 2 3 4 5
Explanation: Every element of each node from head node to last node is printed which means we have traversed each node successfully.
Input: 10->20->30->40->50->null
Output: 10 20 30 40 50
Explanation: Every element of each node from head node to last node is printed which means we have traversed each node successfully.
Traversal of Singly Linked List (Iterative Approach):
The process of traversing a singly linked list involves printing the value of each node and then going on to the next node and print that node’s value also and so on, till we reach the last node in the singly linked list, whose next node is pointing towards the null.
Approach:
In order to traverse the linked list, we initialize a pointer with the head of the linked list. Now, we iterate till the pointer does not point to null. In each iteration, we print the value of the node to which the pointer currently points to and move the pointer to the next node. We keep on printing the value of nodes and updating the pointer till the pointer does not point to null.
Step-by-step algorithm:
- First, we will initialize a pointer to the head node of the singly linked list.
- Second, we will check that the pointer is null or not null, if it is null then return.
- While the pointer is not null, we will access the data or element present on that node and store it in some variable to print side by side, then we move the pointer to next node.
- we will keep on checking the pointer is null or not null, until it reaches to the end of the list.
C++
#include <iostream>
// Defining a class to represent every element of the linked list
class Node {
public:
// Data will store in this node
int data;
// Reference of the next node
Node* next;
// Constructor for creating a node with given data
Node(int data) {
this->data = data;
// there is no next node initially
this->next = nullptr;
}
};
// Main class to execute the code
int main() {
// Define the head of the linked list
Node* head = new Node(10);
// Inserting some elements in the list
head->next = new Node(20);
head->next->next = new Node(30);
head->next->next->next = new Node(40);
// Printing all elements of the singly linked list
Node* ptr = head;
while (ptr != nullptr) {
std::cout << ptr->data << " ";
ptr = ptr->next;
}
std::cout << std::endl;
// Don't forget to free memory to avoid memory leaks
ptr = head;
while (ptr != nullptr) {
Node* temp = ptr;
ptr = ptr->next;
delete temp;
}
return 0;
}
//This code is contributed by Utkasrh
Java
// Defining a class to represent every element of the linked
// list
class Node {
// Data will store in this node
int data;
// Reference of the next node
Node next;
// Constructor for creating a node with given data
Node(int data)
{
this.data = data;
// there is no next node initially
this.next = null;
}
}
// Main class to execute the code
public class Main {
public static void main(String[] args)
{
// Define the head of the linked list;
Node head = new Node(10);
// Inserting some elements in the list
head.next = new Node(20);
head.next.next = new Node(30);
head.next.next.next = new Node(40);
// Printing all elements of the singly linked list
Node ptr = head;
while (ptr != null) {
System.out.print(ptr.data + " ");
ptr = ptr.next;
}
System.out.println();
}
}
Python3
# Defining a class to represent every element of the linked
# list
class Node:
# Constructor for creating a node with given data
def __init__(self, data):
# Data will store in this node
self.data = data
# Reference of the next node
self.next = None
# Main class to execute the code
def main():
# Define the head of the linked list
head = Node(10)
# Inserting some elements in the list
head.next = Node(20)
head.next.next = Node(30)
head.next.next.next = Node(40)
# Printing all elements of the singly linked list
ptr = head
while ptr is not None:
print(ptr.data, end=" ")
ptr = ptr.next
print()
if __name__ == "__main__":
main()
JavaScript
// Defining a class to represent every element of the linked
// list
class Node {
// Data will be stored in this node
constructor(data) {
this.data = data;
// Reference of the next node
this.next = null;
}
}
// Main class to execute the code
class Main {
static main() {
// Define the head of the linked list
let head = new Node(10);
// Inserting some elements in the list
head.next = new Node(20);
head.next.next = new Node(30);
head.next.next.next = new Node(40);
// Printing all elements of the singly linked list
let ptr = head;
while (ptr != null) {
console.log(ptr.data + " ");
ptr = ptr.next;
}
console.log();
}
}
// Call the main method to execute the code
Main.main();
Time Complexity: O(N), where N is the number of nodes in the linked list.
Auxiliary Space: O(1)
Traversal of Singly Linked List (Recursive Approach):
Approach:
We can traverse Singly Linked List using recursion by defining a recursive function with takes pointer to a node as an argument and check if the pointer points to null, then return otherwise print the value of the node to which the pointer points to and call the recursive function again with the next node as the argument.
Step-by-step algorithm:
- Firstly, we will define a recursive method to traverse the singly linked list, which take a node as a parameter.
- Secondly, we will take a base case, that is, if the node is null then we will return from the recursive method.
- After it, we will recursively call the recursive method with the next node as the parameter.
- Then, we will start the recursive traversal from the head node.
Below is the implementation of the recursive approach.
C++
#include <iostream>
// Node class
class Node {
public:
int data;
Node* next;
// Constructor for creating a new node with given data
Node(int data)
{
this->data = data;
this->next = nullptr;
}
};
// Function to traverse the linked list
void traverseList(Node* ptr)
{
if (ptr == nullptr) {
std::cout << std::endl;
return;
}
std::cout << ptr->data << " ";
traverseList(ptr->next);
}
int main()
{
// Define the head of the linked list;
Node* head = new Node(10);
// Inserting some elements in the list
head->next = new Node(20);
head->next->next = new Node(30);
head->next->next->next = new Node(40);
traverseList(head);
return 0;
}
Java
class Node {
int data;
Node next;
// Constructor for creating a new node with given data
Node(int data)
{
this.data = data;
this.next = null;
}
}
public class Main {
// Recursive function to traverse the linked list
public static void traverseList(Node ptr)
{
if (ptr == null) {
System.out.println();
return;
}
System.out.print(ptr.data + " ");
traverseList(ptr.next);
}
public static void main(String[] args)
{
// Define the head of the linked list;
Node head = new Node(10);
// Inserting some elements in the list
head.next = new Node(20);
head.next.next = new Node(30);
head.next.next.next = new Node(40);
traverseList(head);
}
}
Python3
# Node class
class Node:
def __init__(self, data):
# Constructor for creating a new node with given data
self.data = data
self.next = None
# Function to traverse the linked list
def traverse_list(node):
if node is None:
print()
return
print(node.data, end=" ")
traverse_list(node.next)
# Main function
def main():
# Define the head of the linked list
head = Node(10)
# Inserting some elements in the list
head.next = Node(20)
head.next.next = Node(30)
head.next.next.next = Node(40)
traverse_list(head)
if __name__ == "__main__":
main()
JavaScript
// Define the Node class
class Node {
// Constructor for creating a new node with given data
constructor(data) {
this.data = data;
this.next = null;
}
}
// Recursive function to traverse the linked list
function traverseList(ptr) {
if (ptr === null) {
console.log();
return;
}
process.stdout.write(ptr.data + " ");
traverseList(ptr.next);
}
// Main function
function main() {
// Define the head of the linked list
let head = new Node(10);
// Inserting some elements in the list
head.next = new Node(20);
head.next.next = new Node(30);
head.next.next.next = new Node(40);
traverseList(head);
}
main();
Time Complexity: O(N), where N is number of nodes in the linked list.
Space complexity: O(N) because of recursive stack space.
Share your thoughts in the comments
Please Login to comment...