Text and strings Storage in Objective C
Last Updated :
01 Dec, 2023
Objective-C is an object-oriented programming language widely used to develop programs for iOS and macOS. This article focuses on discussing how text and string data can be stored in Objective-C.Â
Types and Subtypes of Strings
Objective-C has two main types of strings – NSString and NSMutableString. An NSString object is immutable and contains a sequence of Unicode characters that cannot be modified after creation. On the other hand, an NSMutableString object is mutable, which means that its contents can be modified.
There are also several subtypes of NSString, each with its specific use case. These subtypes include:
- C Strings.
- NSString Literals.
- String Variables.
1. C Strings
A C string is a sequence of characters stored in a character array. In Objective-C, a C string is represented by a pointer to the first element of the character array. C strings are null-terminated, meaning that a null character (‘\0’) marks the end of the string.
In this example we are going to understand the C string in Objective-C:
ObjectiveC
#import <Foundation/Foundation.h>
int main()
{
char myString[] = "Hello, world!" ;
NSLog ( @"%s" , myString);
return 0;
}
|
Output:

Â
2. NSString Literals
An NSString literal is a sequence of characters enclosed in double quotes. In Objective-C, NSString literals are represented by the @ symbol followed by the string enclosed in double quotes.
In this example, we will understand the NSString literal in Objective-C.
ObjectiveC
#import <Foundation/Foundation.h>
int main()
{
NSString *myString1 = @"Hello, world!" ;
NSLog ( @"myString1: %@" , myString1);
return 0;
}
|
Output:
Â
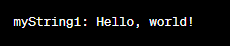
Â
3. String Variables
A string variable is a variable that stores a string value. In Objective-C, string variables are declared using the NSString or NSMutableString class.
In this example, we are going to understand string variables in Objective-C.
ObjectiveC
int main()
{
NSString *myString2 = @"Hello, world!" ;
NSMutableString *myMutableString = [ NSMutableString stringWithString: @"Hello, world!" ];
NSLog ( @"myString2: %@" , myString2);
NSLog ( @"myMutableString: %@" , myMutableString);
return 0;
}
|
Output:
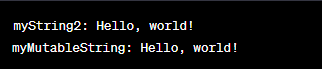
Share your thoughts in the comments
Please Login to comment...