In the world of API testing, there are many tools available. Postman and cURL are two of the most popular tools for it. Let’s look at how to use these tools for testing them. We will send some HTTP requests and explore the basic syntax for both of them in this article. The article focuses on using a fake REST API named JSON placeholder.
What is REST?
REST stands for Representational state transfer. It is an architectural style that can be used in web services for scalable and modifiable communication between client and server. In this architecture, the client-server uses a standardized way to exchange resource representation. The implementation of the client side is independent of the server side. As long as both of them follow the REST architecture style both of them can communicate with each other irrespective of the tech stack of framework used to build them. For example, an API hosted on a single server can be used by both mobile and web applications.
REST API requests should consist of the following:
- An HTTP verb like GET, POST, or DELETE (denotes the kind of operation to be performed)
- Path of the requested resource.
- Headers to pass information about the request like what kind of content is passed in the request.
- Optional message body containing data (if required).
Why Test REST API?
Testing is an important stage in the development of REST APIs. It helps to verify that the APIs are:
- Working correctly and does not cause any unexpected errors.
- Functioning properly under high amounts of user requests.
- Safe and secure.
Methodology of Testing REST
The following questions needs to be asked before writing tests:
- What are the API endpoints that are needed to be tested?
- What kind of data is needed by an API?
- What kind of response is given by an API?
- How much time an API should take to respond?
- What kind of response should an API give in case a request fails?
Then create some input data for your APIs and the corresponding output for it. Send the input data to the API and test whether the response matches with the output data or not. The test case should analyse the following properties of responses:
- Status code.
- Response time.
- Response data.
- Response headers.
Suppose you have created an API for user authentication system that requires username and password. You will create some test cases consisting of multiple username and passwords and their corresponding responses that are expected by the API. Then pass the input data one by one and compare the responses with the output in the test cases.
Using cURL for API Testing
cURL (client for URL) is a command line tool that is used to transfer data using various network protocols (including HTTP). It is available on various platforms including Windows, macOS and Linux.
Open command line terminal on your computer. To send a get request, enter the command given below:
curl -X GET https://jsonplaceholder.typicode.com/posts/1
Here, -X is used to specify an HTTP verb (GET, POST, DELETE etc).
To send a POST request use the command given below:
curl -X POST https://jsonplaceholder.typicode.com/posts -H “Content-Type: application/json” -d “{\”title\”: \”foo\”,\”body\”:\”bar\”,\”userId\”:1}”
Here, -H option is for setting the headers. Here Content-Type represents the type of content of the request body (here it is json). -d denotes the request body. (\“ is used to escape the “.)
To output your requests to a file using curl command with the -o option:
curl -X GET https://jsonplaceholder.typicode.com/posts/2 -o response.json
cURL can be integrated into scripts and automated test suites by using its command-line capabilities. You can chain multiple cURL commands together to simulate complex API scenarios like user authentication.
Using Postman for API Testing
Postman is a platform for building and testing APIs. It comes with a graphical user interface which makes API testing easy and has features for automated testing and API monitoring.
Step 1: Install Postman
Download postman from here. After installing, launch the application. It will look something like this.
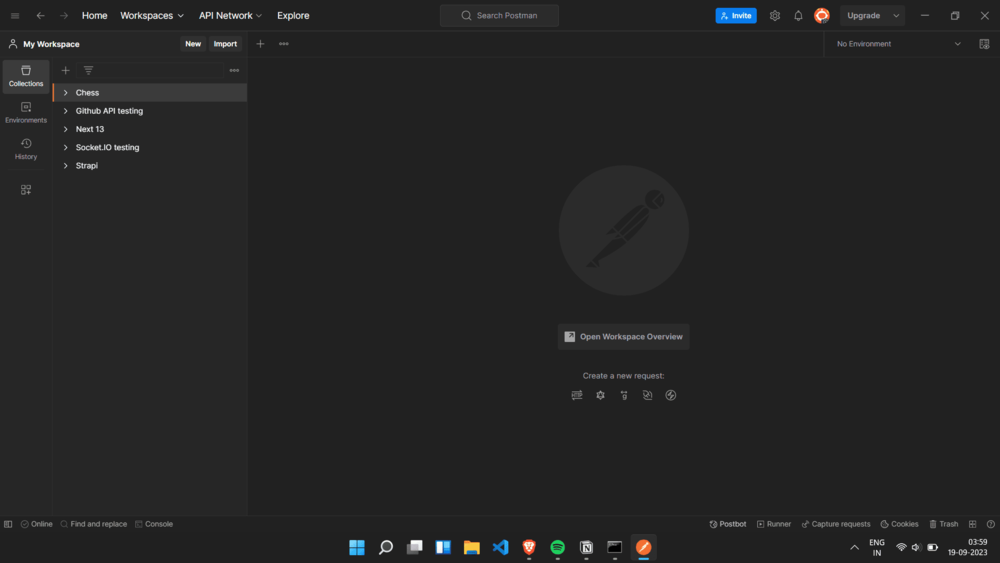
postman desktop application
Step 2: Create a new collection
On the top left corner, press the + icon to create a new collection. Name it Postman testing. A collection in Postman is a group of requests.
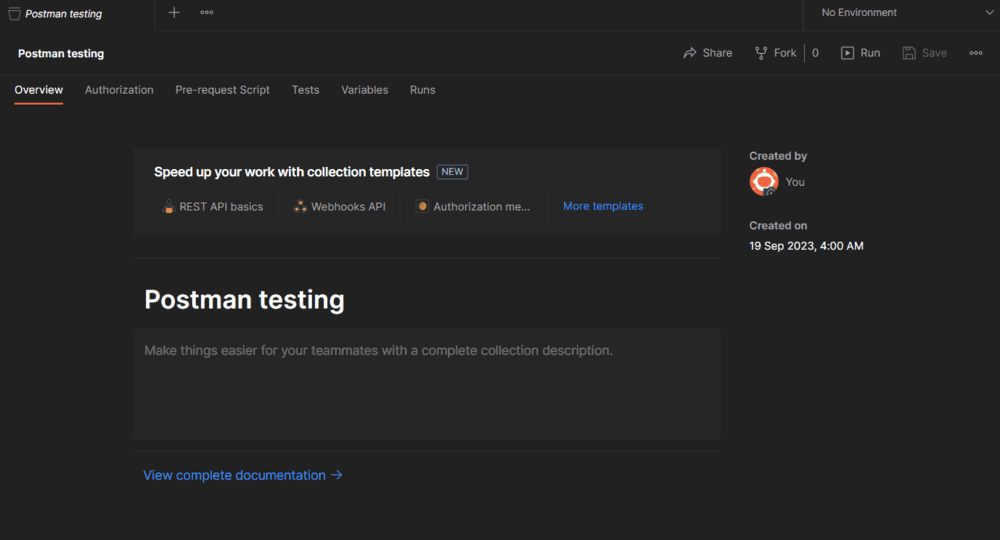
Step 3: Create a new request
On the left side click on the three dots on the collection tab and click Add Request to create a new request. Name the request get request.
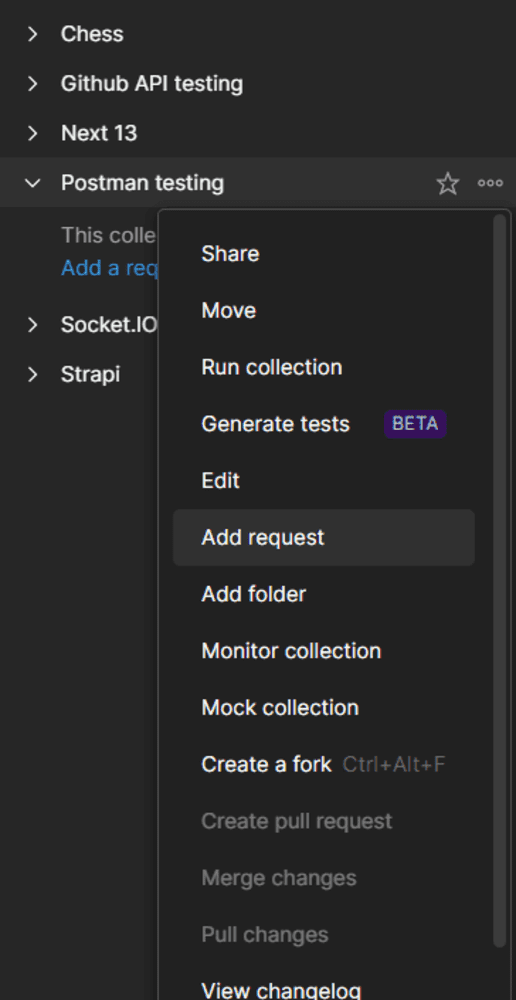
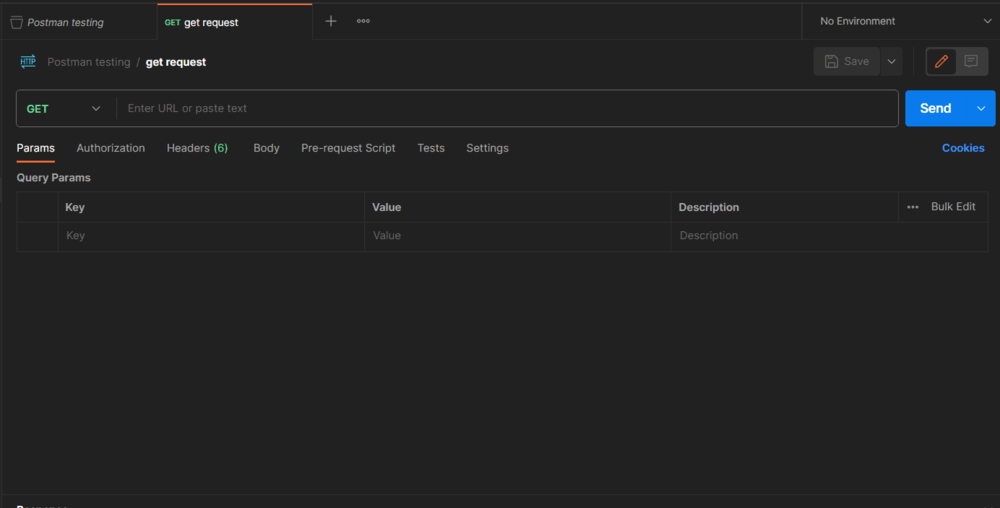
Step 4: Sending HTTP requests
Set the HTTP method to GET on the top. Enter https://jsonplaceholder.typicode.com/posts/1 in the URL field. Now, hit the send button. You will get a response in the response tab below with status code 200 meaning the response was successful.
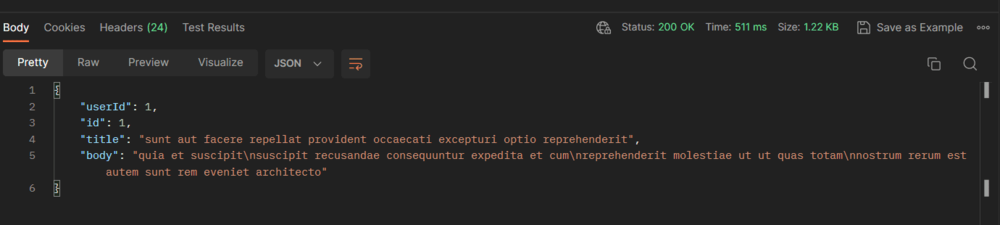
Congratulations, you completed your first REST API test. In this way, you can test REST APIs to make sure the APIs are working well. Of course, there is much more in API testing, but this is the basic workflow.
Sending POST Request
1. Create a new request in the collection.
2. Set the method to POST.
3. Enter https://jsonplaceholder.typicode.com/posts in the URL field.
4. Now we need to send a body with a POST request. Select Body tab below the URL field.
5. Select Raw and JavaScript instead of Text. Paste this JSON object in the text area and hit send.
{ title: ‘foo’, body: ‘bar’, userId: 1, }
6. You will get a JSON object in response denoting the id of the newly created object and a 201-status code which means your object was created successfully in the backend.

Sending a DELETE Request
1. Create a new request for DELETE. Select DELETE method and send the request to this URL https://jsonplaceholder.typicode.com/posts/50
2. You will get a response similar to this meaning that the object with id 1 was successfully deleted.

Testing using Script in Postman
While testing real world APIs there would be many APIs and manually checking the status codes and responses of each API would be a cumbersome task. Postman has a solution for this – test scripts. Postman allows writing test scripts to automate the testing of API.
To write a test script go to the get request.
1. Select the Tests tab under it.
2. You can write scripts in JavaScript to assert your API response.
3. Paste the following code into the Tests tab and hit send.
Javascript
pm.test( "Status code is 200" , function () {
pm.response.to.have.status(200);
});
const jsonData = pm.response.json();
pm.test( "Response contains userId" , function () {
pm.expect(jsonData.userId).to.exist;
});
pm.test( "Response contains id" , function () {
pm.expect(jsonData.id).to.exist;
});
pm.test( "Response contains title" , function () {
pm.expect(jsonData.title).to.exist;
});
pm.test( "Response contains body" , function () {
pm.expect(jsonData.body).to.exist;
});
|
4. Go to Test Results tab in the response. You can see that all of our test cases passed.
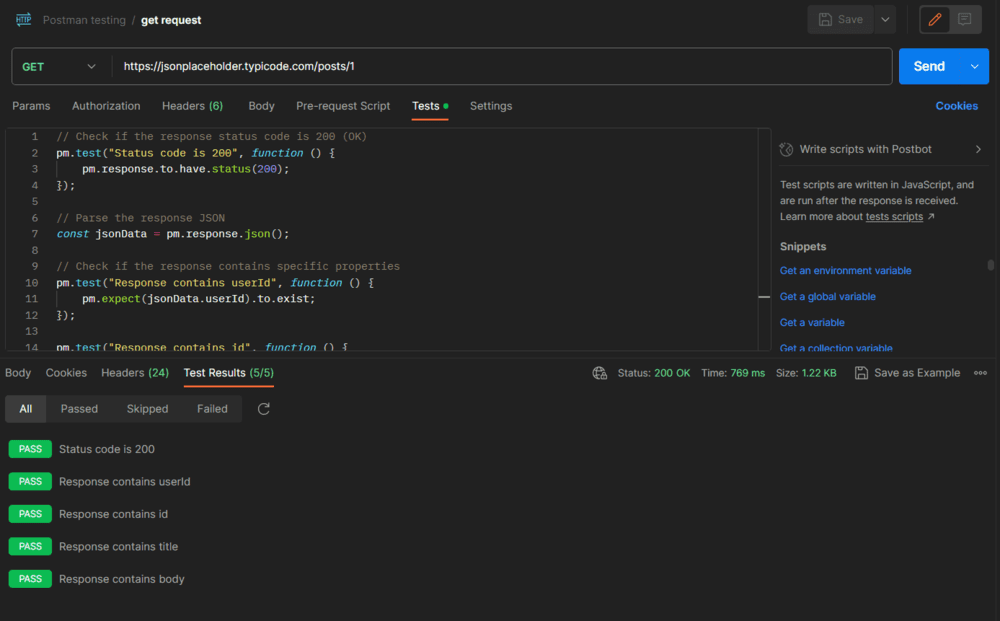
Congratulations, you just created an API test script.
Postman vs cURL
Postman is a platform for building and testing REST APIs. It comes with a graphical user interface and has many useful features like organizing tests into collections, automated testing and API monitoring. Postman allows you to create environments, set variables and so on. You can write scripts through which developers can build automated tests. It also has version control and collaboration features which are useful for developers working in teams.
cURL is a command-line tool for transferring data using many network protocols and HTTP is one of them. It is useful in situations where you don’t require a graphical interface. It comes mostly pre-installed with many operating systems. It can also be easily integrated into scripts.
Conclusion
We looked at the process of testing REST APIs with cURL and Postman in this article. You can choose either of them based on your testing requirements. Some developers also use both of them to have the best of both worlds.
Share your thoughts in the comments
Please Login to comment...