Swift is a powerful programming language for all Apple platforms. SwiftyJSON is an open-source and free-to-use library that helps to format JSON in an easy and usable format. It is a third-party Swift library that is used to read and process JSON data from an API/Server.
To use SwiftyJSON you just need to import that library:
import SwiftyJSON
Features of SwiftyJSON
- Simplified Syntax: SwiftyJSON makes it simple to work with JSON data in Swift. Instead of using complicated code, it provides a clear and easy way to grab information from JSON.
- Type Safety: SwiftyJSON helps you avoid confusion about the type of information in the JSON.
- Error Handling: Sometimes, the JSON data might not be exactly what you expect. SwiftyJSON helps you handle these situations.
- Doing Many Things at Once: SwiftyJSON allows you to do several things one after another without making your code messy.
Why use SwiftyJSON?
Without SwiftyJSON
Below is the program to extract Employee Name from JSON Data without Using SwiftyJSON:
Swift
import Swift
if let statusesArray = try? JSONSerialization.jsonObject(with: data,
options: .allowFragments) as ? [[ String : Any ]],
let employees = statusesArray[0][ "employee" ] as ? [ String : Any ],
let employeeName = user[ "name" ] as ? String {
}
|
With SwiftyJSON
Below is the program to extract Employee Name from JSON Data Using SwiftyJSON:
Swift
import Swift
import SwiftyJSON
let json = JSON(data: dataFromNetworkingOrString)
if let employeetName = json[0][ "employees" ][ "name" ].string {
}
|
Steps to Install SwiftyJSON
Step 1: Install Cocoapods
Install cocoaPods, once that is ready, let’s create our Xcode project.
Step 2: Open Existing Project OR Create Project
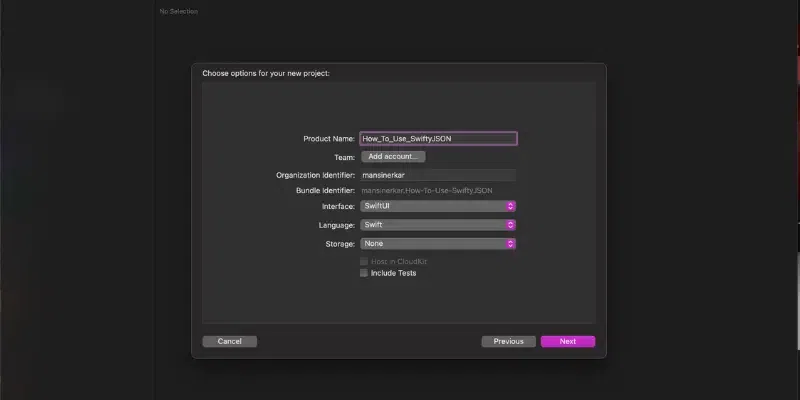
create project
Step 3: Add Project
Now navigate to your project folder and open a terminal window, also navigate the terminal window to the project location.
cd /Users/your account/Documents/GeeksForGeeks/How_To_Use_SwiftyJSON/
The above path may be different for you so Type “cd/ ” and paste that path.
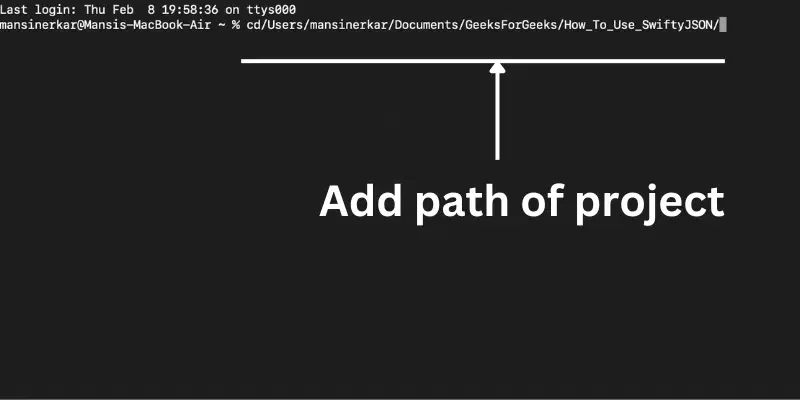
add the path to the terminal
Step 4: pod init
Navigate to your project folder using Terminal and type pod Init Once there you should do a “pod init” in your terminal:
pod init
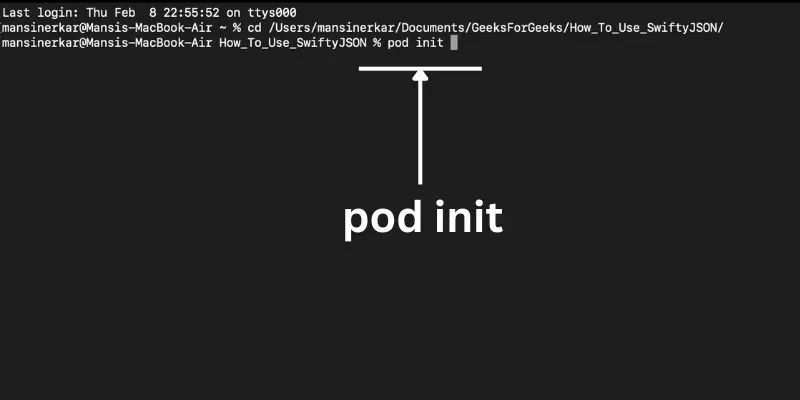
Pod init
It will then output a file named Podfile.
Step 5: Add the SwiftyJSON
Now navigate to the project using finder Open the profile and add the SwiftyJSON pod:
pod “SwiftyJSON”
pod ‘”Alamofire”
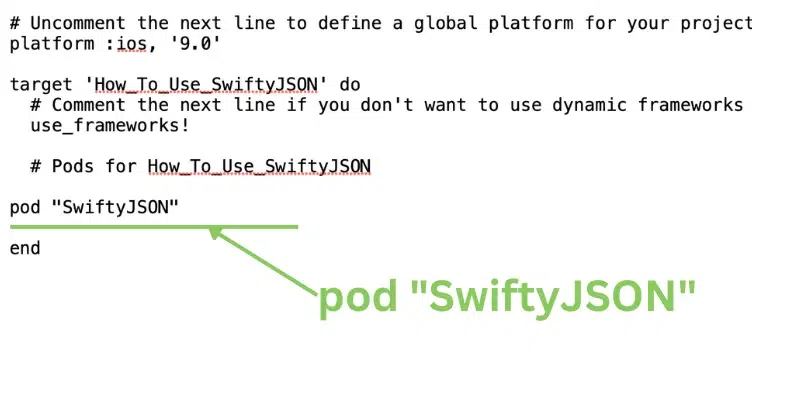
pod “SwiftyJSON”
The code will look like
# Uncomment the next line to define a global platform for your project
# platform :ios, ‘9.0’
target ‘GFG’ do
# Comment the next line if you don’t want to use dynamic frameworks
use_frameworks!
pod ‘Alamofire’
# Pods for GFG
pod “SwiftyJSON”
end
Step 6: Run pod
Save the file and in your terminal run the following command:
pod install
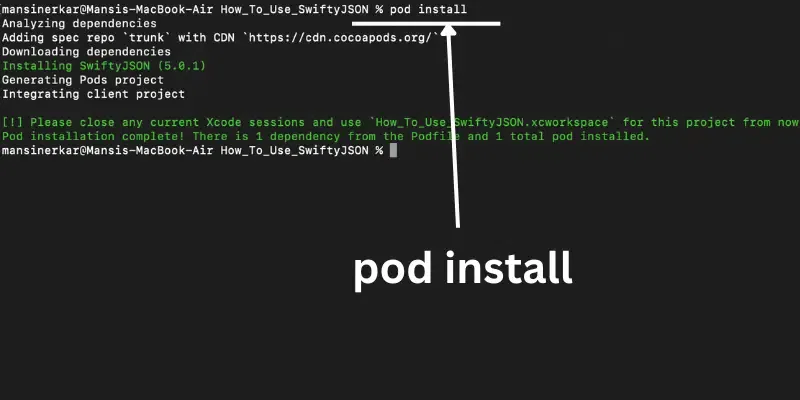
pod install
Step 7: Import
Open the file named [projectname. xcworkspace] and your project should have SwiftyJSON installed and ready to go.
Step 8: Add SwiftyJSON Dependencies
Navigate to the “File” option in the top left corner and click on the Add Package Dependencies.
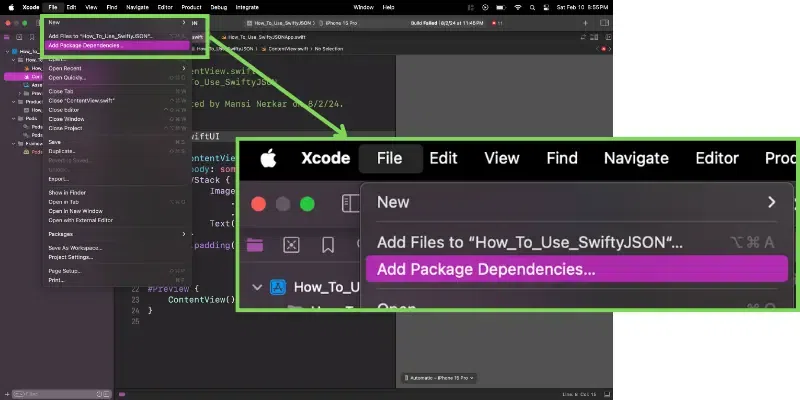
Add Package Dependencies
Now click on the search button and paste the GitHub link:
https://github.com/SwiftyJSON/SwiftyJSON
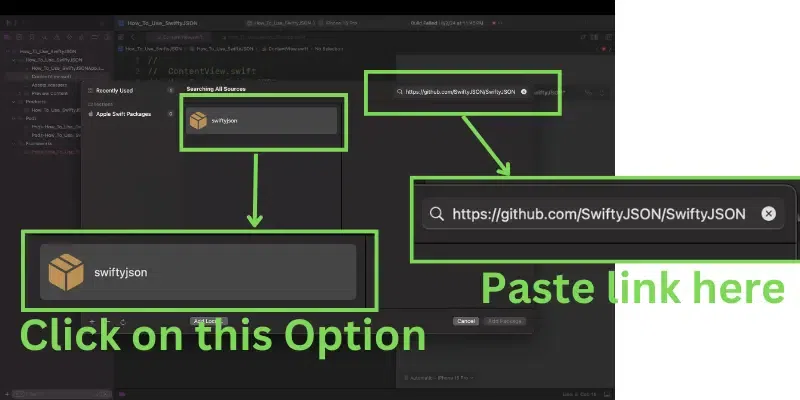
add git link
Now click on the Add Package.
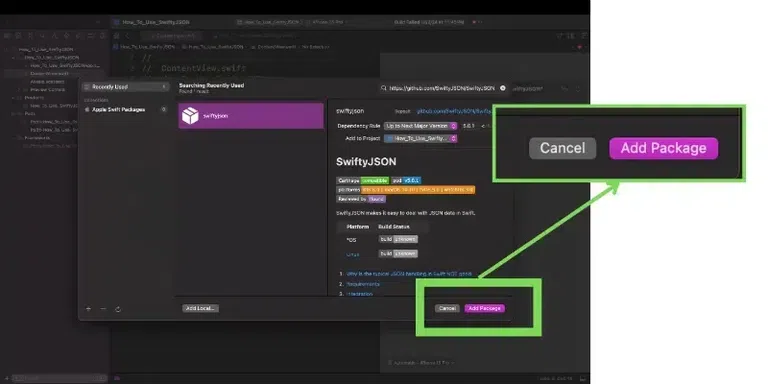
Wait until it is finished.
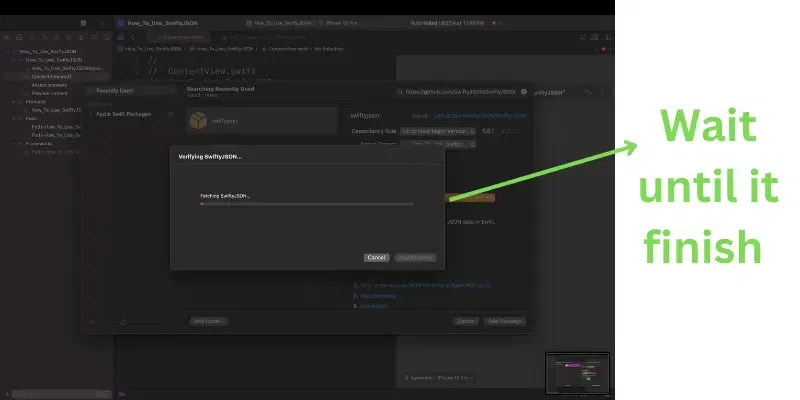
wait until it downloads the files
Click on the Add Package.
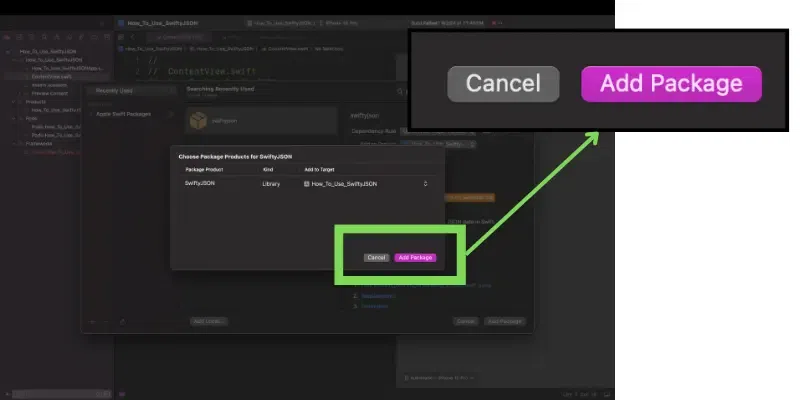
click on Add Package
If the package is visible in the Package Dependencies, you have successfully added the dependency.
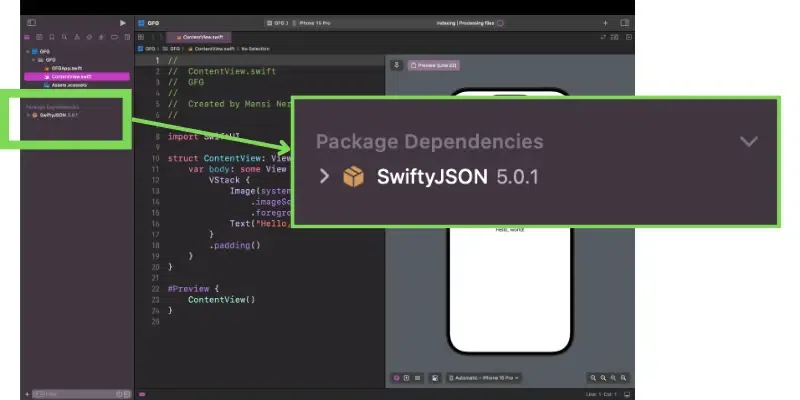
Tips
1. Import SwiftyJSON
import SwiftyJSON
2. Initializing SwiftyJSON
Create a SwiftyJSON object by initializing it with your JSON data:
if let jsonData = jsonString.data(using: .utf8) {
let json = try? JSON(data: jsonData)
// Now, ‘json’ is your SwiftyJSON object
}
3. Accessing JSON Values
Use subscript notation to access values in a clean and readable way:
let name = json[“name”].stringValue
let age = json[“age”].intValue
4. Type Safety
Leverage SwiftyJSON’s type inference to handle different types of JSON values.
let isStudent = json[“isStudent”].boolValue
5. Error Handling
Be sure to handle errors when working with JSON, especially if the structure may vary.
if let error = json.error {
print(“Error parsing JSON: \(error)”)
}
6. Chaining Operations
Take advantage of chaining to perform multiple operations concisely.
let fullName = json[“name”].stringValue + ” ” + json[“lastName”].stringValue
7. Deal with Optional Values
SwiftyJSON returns optional values to handle situations where the JSON key may not exist.
if let optionalValue = json[“optionalKey”].string {
// Use ‘optionalValue’ here
}
8. Handling Arrays
Work with arrays using SwiftyJSON’s array methods.
for item in json[“arrayKey”].arrayValue {
// Process each item in the array
}
9. Transformation Methods
Utilize SwiftyJSON’s transformation methods to convert JSON data easily.
let intValue = json[“numericString”].intValue
Example 1
In this example, we will see how to fetch JSON data from a URL and parse it using SwiftyJSON.
File Name: data.json
{
“students”: [
{
“name”: “Geeks_1”,
“age”: 25,
“isStudent”: true
},
{
“name”: “Geeks_2”,
“age”: 22,
“isStudent”: true
},
{
“name”: “Geeks_3”,
“age”: 28,
“isStudent”: false
}
]
}
“data.json” is the JSON file in which we have the data students that we are going to display on the screen
File NameContentView.swift
Swift
import SwiftUI
struct ContentView: View {
@State private var students: [Student] = []
var body: some View {
VStack {
Text( "Student Data" ).font(.title)
if students.isEmpty {
Text( "Loading..." )
.onAppear {
fetchData()
}
} else {
List(students, id: \.name) { student in
VStack(alignment: .leading) {
Text( "Name: \(student.name)" )
Text( "Age: \(student.age)" )
Text( "Is Student: \(student.isStudent ? " Yes " : " No ")" )
}
}
}
}
}
private func fetchData() {
DataFetcher.fetchData { fetchedStudents in
if let fetchedStudents = fetchedStudents {
self .students = fetchedStudents
}
}
}
}
#Preview {
ContentView()
}
|
In the above swift code, we have created a list in which we are going to display the student data and also we wrote the function to fetch the data
File Name: DataFetcher.swift
Swift
import Foundation
import SwiftyJSON
class DataFetcher {
static func fetchData(completion: @escaping ([Student]?) -> Void ) {
guard let path = Bundle.main.path(forResource: "data" , ofType: "json" ) else {
print ( "Failed to find data.json" )
completion( nil )
return
}
do {
let data = try Data(contentsOf: URL(fileURLWithPath: path), options: .mappedIfSafe)
let json = try JSON(data: data)
var students = [Student]()
for (_, studentJson):( String , JSON) in json[ "students" ] {
let name = studentJson[ "name" ].stringValue
let age = studentJson[ "age" ].intValue
let isStudent = studentJson[ "isStudent" ].boolValue
let student = Student(name: name, age: age, isStudent: isStudent)
students.append(student)
}
completion(students)
} catch {
print ( "Failed to parse data.json:" , error)
completion( nil )
}
}
}
struct Student {
let name: String
let age: Int
let isStudent: Bool
}
|
In this Swift code, we have created the variables that are present in the JSON file and created the extractor of json
Output:
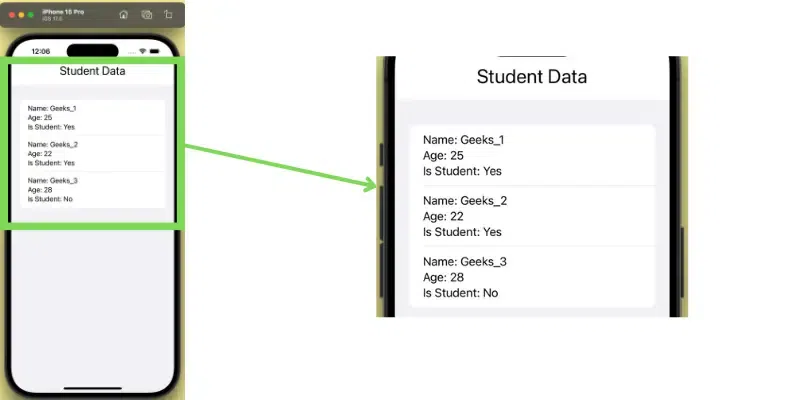
Output of Example 1
Example 2
File Name: Data.json
{
“movies”: [
{
“title”: “Interstellar”,
“director”: “Christopher Nolan”,
“year”: 2014,
“rating”: 8.6
},
{
“title”: “The BFG”,
“director”: “Steven Spielberg”,
“year”: 2016,
“rating”: 6.4
},
{
“title”: “Frozen”,
“director”: “Chris Buck, Jennifer Lee”,
“year”: 2013,
“rating”: 7.4
}
]
}
File Name: ContentView.swift
Swift
import SwiftUI
struct ContentView: View {
@State private var movies: [Movie] = []
var body: some View {
NavigationView {
List(movies, id: \.title) { movie in
VStack(alignment: .leading, spacing: 8) {
Text(movie.title)
.font(.headline)
Text( "Directed by: \(movie.director)" )
.font(.subheadline)
.foregroundColor(.secondary)
Text( "Year: \(movie.year)" )
.font(.subheadline)
.foregroundColor(.secondary)
Text( "Rating: \(movie.rating)" )
.font(.subheadline)
.foregroundColor(.secondary)
}
}
.navigationTitle( "Movies" )
}
.onAppear {
fetchData()
}
}
private func fetchData() {
if let fetchedMovies = DataFetcher.fetchData() {
movies = fetchedMovies
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
|
In the above swift code, we have created a list in which we are going to display the student data and also we wrote the function to fetch the data
File Name: Movie. swift
Swift
import Foundation
import SwiftyJSON
struct Movie {
let title: String
let director: String
let year: Int
let rating: Double
}
|
In the above swift code, we have created the structure named movies in which we initialize the data type of the variables that are present in the json file
File Name: DataFetcher.swift
Swift
import Foundation
import SwiftyJSON
class DataFetcher {
static func fetchData() -> [Movie]? {
guard let path = Bundle.main.path(forResource: "data" , ofType: "json" ) else {
print ( "Failed to find data.json" )
return nil
}
do {
let data = try Data(contentsOf: URL(fileURLWithPath: path), options: .mappedIfSafe)
let json = try JSON(data: data)
var movies = [Movie]()
for (_, movieJson):( String , JSON) in json[ "movies" ] {
let title = movieJson[ "title" ].stringValue
let director = movieJson[ "director" ].stringValue
let year = movieJson[ "year" ].intValue
let rating = movieJson[ "rating" ].doubleValue
let movie = Movie(title: title, director: director, year: year, rating: rating)
movies.append(movie)
}
return movies
} catch {
print ( "Failed to parse data.json:" , error)
return nil
}
}
}
|
In the above swift code, we have created a list in which we are going to display the student data and also we wrote the function to fetch the data
Output:
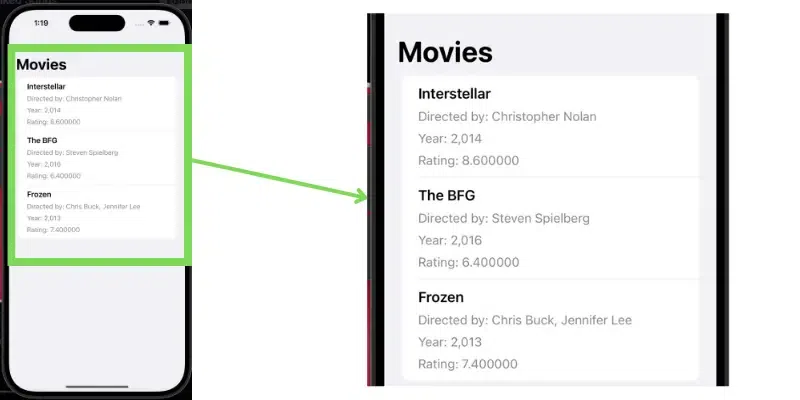
Output of Example 2
Share your thoughts in the comments
Please Login to comment...