Swift – Methods
Last Updated :
26 Feb, 2023
Methods are functions that belong to a specific type. Instance methods, which encapsulate particular tasks and functionality for working with an instance of a given type, can be defined by classes, structures, and enumerations. Type methods, which are connected to the type itself, can also be defined by classes, structures, and enumerations. In Objective-C, type methods are comparable to class methods.
Swift differs significantly from C and Objective-C in that structures and enumerations can define methods, whereas classes are the only types in Objective-C that can. In Swift, you can define a class, structure, or enumeration and still define methods on the type you create.
Instance Methods
Classes, structures, and enumeration instances can be accessed using Swift instance methods. To access and modify instance properties, instance methods provide functionality relevant to the instance’s need. The curly braces can be used to write the instance method. It has implicit access to the type instance’s methods and properties. It will have access to that specific instance of the type when it is called.
Syntax
func funcname(Parameters) -> returntype {
Statement1
Statement2
—
Statement N
return parameters
}
Example:
Swift
class calculate
{
let x: Int
let y: Int
let res: Int
init (x: Int , y: Int )
{
self .x = x
self .y = y
res = x + y
}
func tot(z: Int ) -> Int {
return res - z
}
func result() {
print ( "Result is: \(tot(z: 70))" )
print ( "Result is: \(tot(z: 90))" )
}
}
let pri = calculate(x: 400, y: 500)
pri.result()
|
Output:
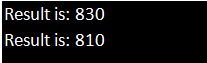
Output
Local and External Parameter Names
In Swift, functions can describe their variables’ local and global declarations. For functions and methods, the characteristics of local and global parameter name declarations vary. For convenience, the first parameter in Swift is referred to by the prepositions “with,” “for,” and “by.”
Declaring the names of the first and subsequent parameters as global parameters is now possible in Swift. By declaring the first parameter name to be a local parameter name and the remaining parameter names to be global parameter names, Swift offers flexibility in methods. In this case, Swift methods declare “no1” as a local parameter name. Throughout the programme, global declarations are made using the variable “no2.”
Swift
class division
{
var count : Int = 0
func incrementBy(no1: Int , no2: Int )
{
count = no1 / no2
print ( count )
}
}
let counter = division()
counter.incrementBy(no1: 120, no2: 2)
counter.incrementBy(no1: 220, no2: 4)
counter.incrementBy(no1: 486, no2: 6)
|
Output:

Output
Self property in Methods
All instances of the defined type have a property called “self” that is implicit in methods. To refer to the current instances of its defined methods, use the “Self” property.
Example:
Swift
class calculate
{
let x: Int
let y: Int
let res: Int
init (x: Int , y: Int )
{
self .x = x
self .y = y
res = x + y
print ( "Result Inside Self Block: \(res)" )
}
func tot(z: Int ) -> Int {
return res - z
}
func result()
{
print ( "Result is: \(tot(z: 10))" )
print ( "Result is: \(tot(z: 30))" )
}
}
let pri = calculate(x: 500, y: 100)
let sum = calculate(x: 200, y: 600)
pri.result()
sum.result()
|
Output:
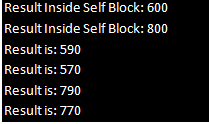
Output
Modifying Value Types from Instance Methods
Structures and enumerations are value types in the Swift 4 language, meaning that instance methods cannot change them. Swift 4 language, on the other hand, offers flexibility to change the value types by “mutating” behaviour. After the instance methods have been executed, mutate will make any changes and then revert to the initial state. Additionally, a new instance is created for the implicit function by the “self” property, which replaces the current method after it has been used.
Swift
struct area
{
var length = 1
var breadth = 1
func area() -> Int {
return length * breadth
}
mutating func scaleBy(res: Int )
{
length *= res
breadth *= res
print (length)
print (breadth)
}
}
var val = area(length: 2, breadth: 4)
val.scaleBy(res: 3)
val.scaleBy(res: 30)
val.scaleBy(res: 300)
|
Output:
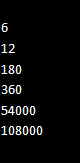
Output
Self Property for Mutating Method
A new instance of the defined method is given when mutating methods are combined with the “self” property.
Swift
struct area
{
var length = 1
var breadth = 1
func area() -> Int {
return length * breadth
}
mutating func scaleBy(res: Int ) {
self .length *= res
self .breadth *= res
print (length)
print (breadth)
}
}
var val = area(length: 6, breadth: 8)
val.scaleBy(res: 11)
|
Output:

Output
Type Methods
An instance method is used when a specific instance of a method is called; Additionally, the method is referred to as a “Type Method” when it calls a particular type of method. The “func” keyword is used to define type methods for “classes,” while the “static” keyword precedes the “func” keyword to define type methods for “structures” and “enumerations.”
The “.” syntax is used to access and call type methods, which invokes the entire method rather than just one specific instance.
Swift
class Math
{
class func abs (number: Int ) -> Int {
if number < 0
{
return (-number)
}
else
{
return number
}
}
}
struct absno
{
static func abs (number: Int ) -> Int
{
if number < 0
{
return (-number)
}
else
{
return number
}
}
}
let no = Math. abs (number: -20)
let num = absno. abs (number: -15)
print (no)
print (num)
|
Output:

Output
Share your thoughts in the comments
Please Login to comment...