Swift – Functions and its types
Last Updated :
12 Dec, 2023
A function in Swift is a standalone section of code that completes a particular job. Code is organized into functions that can be called repeatedly throughout a program to make it simpler to understand and maintain.
Here’s the basic syntax for defining a function in Swift:
Swift
func functionName(argument1: Type , argument2: Type , ...) -> ReturnType {
return returnValue
}
|
Parameters passed to functions:
- func: keyword to define a function.
- functionName: name of the function.
- argument1, argument2: arguments passed to the function. Each argument consists of a name and a type.
- ReturnType: the data type of the value returned by the function.
- function body: the code that is executed when the function is called.
- return: statement used to return a value from the function.
For example, here’s a simple function that takes two integer arguments and returns their sum:
Swift
func addNumbers(number1: Int , number2: Int ) -> Int {
let result = number1 + number2
return result
}
let sum = addNumbers(number1: 5, number2: 7)
print (sum)
|
Swift functions can have multiple return values using tuples:
Swift
func calculateStatistics(numbers: [ Int ]) -> ( min : Int , max : Int , sum: Int ) {
var min = numbers[0]
var max = numbers[0]
var sum = 0
for number in numbers {
if number < min {
min = number
} else if number > max {
max = number
}
sum += number
}
return ( min , max , sum)
}
let statistics = calculateStatistics(numbers: [5, 3, 10, 2, 7])
print (statistics. min )
print (statistics. max )
print (statistics.sum)
|
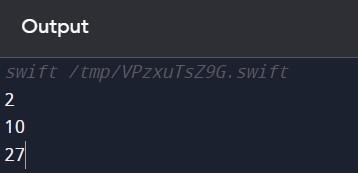
Â
Types of Functions:
In Swift, there are several types of functions. Let’s take a look at each one:
1. Global Functions: These are functions that are defined outside of any class, structure or enumeration. Global functions can be called from anywhere within the program.
Swift
func calculateSum(_ numbers: [ Int ]) -> Int {
var sum = 0
for number in numbers {
sum += number
}
return sum
}
let numbers = [1, 2, 3, 4, 5]
let sum = calculateSum(numbers)
print (sum)
|
Output:
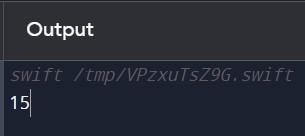
Â
2. Nested Function: These are functions that are defined within another function. Nested functions can only be called from within the enclosing function.
Â
Swift
func outerFunction(_ number1: Int , _ number2: Int ) -> Int {
func innerFunction(_ number: Int ) -> Int {
return number * 2
}
let result1 = innerFunction(number1)
let result2 = innerFunction(number2)
return result1 + result2
}
let number1 = 3
let number2 = 5
let result = outerFunction(number1, number2)
print (result)
|
Output:
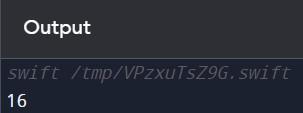
Â
3. Methods: These functions are defined as part of a class, structure, or enumeration. Methods can access and modify the properties of that type.
Swift
class Calculator {
func addNumbers(_ number1: Int , _ number2: Int ) -> Int {
return number1 + number2
}
}
let calculator = Calculator()
let result = calculator.addNumbers(5, 7)
print (result)
|
Output:
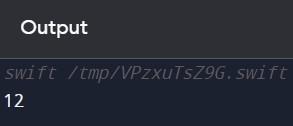
Â
4. Closures: These are self-contained blocks of code that can be assigned to a variable or passed as an argument to a function.
Swift
let numbers = [1, 2, 3, 4, 5]
let filteredNumbers = numbers. filter { $0 % 2 == 0 }
print (filteredNumbers)
|
Output:
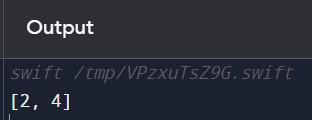
Â
5. Higher-order Functions: These are functions that take other functions as arguments or return functions as their result.
Swift
func filterIntegers(_ numbers: [ Int ], _ isIncluded: ( Int ) -> Bool ) -> [ Int ] {
var result = [ Int ]()
for number in numbers {
if isIncluded(number) {
result.append(number)
}
}
return result
}
let numbers = [1, 2, 3, 4, 5]
let isEven: ( Int ) -> Bool = { $0 % 2 == 0 }
let filteredNumbers = filterIntegers(numbers, isEven)
print (filteredNumbers)
|
Output:
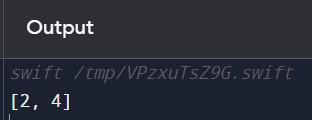
Â
Share your thoughts in the comments
Please Login to comment...