Struts 2 Custom Validation – Workflow Interceptor
Last Updated :
16 Jan, 2024
Struts 2 allows us to design our validation logic, commonly known as custom validation, by implementing the action class’s Validatable Interface. As you might expect, understanding the Validatable interface is critical for understanding Struts2 custom validation.
Workflow Interceptor
The workflow interceptor verifies whether or not there are validation errors. It carries out no validation. When an action class implements the Validatable interface, it is used. For this interceptor, the default argument that decides the action or field error result to be executed is the input. We do not need to specify it directly because it is already in the default stack. Here is an example of a Workflow Interceptor:
XML
< action name = "someAction" class = "org.geeksforgeeks" >
< interceptor-ref name = "params" />
< interceptor-ref name = "validation" />
< interceptor-ref name = "workflow" />
< result name = "success" >good_result.ftl</ result >
</ action >
<-- In this case myMethod as well as mySecondMethod of the action class
will not pass through the workflow process -->
< action name = "someAction" class = "org.geeksforgeeks" >
< interceptor-ref name = "params" />
< interceptor-ref name = "validation" />
< interceptor-ref name = "workflow" >
< param name = "excludeMethods" >myMethod,mySecondMethod</ param >
</ interceptor-ref name = "workflow" >
< result name = "success" >good_result.ftl</ result >
</ action >
< action name = "someAction" class = "com.examples.SomeAction" >
< interceptor-ref name = "params" />
< interceptor-ref name = "validation" />
< interceptor-ref name = "workflow" >
< param name = "inputResultName" >error</ param >
< param name = "excludeMethods" >*</ param >
< param name = "includeMethods" >myWorkflowMethod</ param >
</ interceptor-ref >
< result name = "success" >good_result.ftl</ result >
</ action >
|
Struts 2 Custom Validation methods
- void setActionMessages(Collection<String> messages): This action’s collection of messages is set using the set action messages function.
- void addActionMessage(String message): The add action message function adds a message at the Action level to the particular action.
- void setFieldErrors(MapString,ListString>> map): Establishes a set of error messages for fields using the set field error function.
- boolean hasActionErrors(): Looks for error messages at the action level.
- boolean hasActionMessages(): This function looks for messages at the Action level.
- void setFieldErrors(MapString,ListString>> map): Establishes a set of error messages for fields using the set field error function.
- Boolean hasErrors(): Verifies if any actions or fields contain errors.
- Map<String,List<String>> getFieldErrors(): This map function retrieves all field-level error messages.
- Collection<String> getActionErrors(): This collection function retrieves all Action-level error messages.
Steps to perform Custom Validation
Step 1: Make an input index.jsp
Using struts UI tags, this jsp website generates a form. The user provides it with their name, password, and email address.
<%@ taglib uri="/struts-tags" prefix="s" %>
<s:form action="register">
<s:textfield name="name" label="Name"></s:textfield>
<s:password name="password" label="Password"></s:password>
<s:submit value="register"></s:submit>
</s:form>
Step 2: Create the action class
This action class inherits the ActionSupport class and overrides the validate method to define the validation logic.
Java
package org.geeksforgeeks;
import com.opensymphony.xwork2.ActionSupport;
public class RegisterAction extends ActionSupport{
private String name,password;
public void validate() {
if (name.length()< 1 )
addFieldError( "name" );
if (password.length()< 6 )
addFieldError( "password" );
}
public String execute(){
return "success" ;
}
}
|
Step 3: Define a struts.xml input result
This xml file defines an extra result by the name input, that will be called if any error message is discovered in the action class.
XML
<? xml version = "1.5" encoding = "UTF-8" ?>
<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts
< struts >
< package name = "default" extends = "struts-default" >
< action name = "register" class = "org.geeksforgeeks.RegisterAction" >
< result >welcome.jsp</ result >
< result name = "input" >index.jsp</ result >
</ action >
</ package >
</ struts >
|
Step 4: Make a view component
It’s a straightforward JSP file that shows the user’s data.
<%@ taglib uri="/struts-tags" prefix="s" %>
Name:<s:property value="name"/><br/>
Password:<s:property value="password"/><br/>
Output: Custom Validation Fields
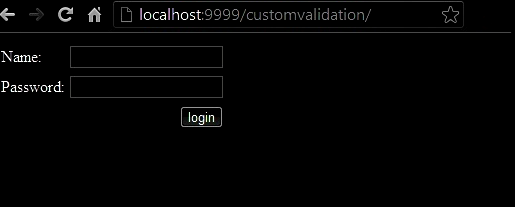
Output: FieldError Validation
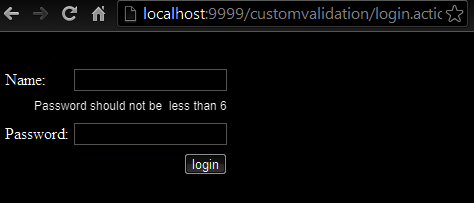
Share your thoughts in the comments
Please Login to comment...