Struts 2 double validation
Last Updated :
16 Jan, 2024
The Struts 2 Framework’s Double Validator determines whether or not the input is double. The error message is generated if the input is not twice. The input range can also be verified using a double validator. This example shows how to check the input range using a double validator.
Plain validator Syntax of double validator
The purpose of the double validator is to verify that the double falls within a certain range.
<validators>
<validator type="double">
<param name=”fieldName">fieldName</param>
<param name="min">minDouble</param>
<param name="max">maxDouble</param>
<message>message string</message>
</validator>
</validators>
Parameters of double validator
- minExclusive:The minimal exclusive value is specified by minExclusive. By default, it is disregarded.
- minInclusive : The minimal inclusive value is specified by minInclusive. By default, it is disregarded.
- maxExclusive: The maximum inclusive value is indicated by maxInclusive. By default, it is disregarded.
- fieldName:The field name that has to be verified is specified by fieldName. Only in Plain-Validator is it necessary.
Struts 2 double validation
index.jsp for input
Using struts UI tags, this jsp website generates a form. The user provides it with their name, password, and email address.
HTML
< html >
< head >
< STYLE type = "text/css" >
.errorMessage{color:red;}
</ STYLE >
</ head >
< body >
< s:form action = "login" >
< s:textfield name = "id" label = "UserName" ></ s:textfield >
< s:textfield name = "price" label = "Amount" ></ s:textfield >
< s:submit value = "login" ></ s:submit >
</ s:form >
</ body >
</ html >
|
struts.xml
This XML file specifies an interceptor called jsonValidatorWorkflowStack and an additional result called input.
XML
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0//EN"
< struts >
< package name = "user" extends = "struts-default" >
< action name = "Login"
class = "org.geeksforgeeks.action.Login" >
< result name = "success" >/welcome.jsp</ result >
< result name = "input" >/login.jsp</ result >
</ action >
</ package >
</ struts >
|
Action class
This action class inherits the ActionSupport class and overrides the execute method.
Java
package org.geeksforgeeks;
import com.opensymphony.xwork2.ActionSupport;
public class Login extends ActionSupport{
private String userName;
private double amount;
public String execute(){
return SUCCESS;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this .userName = userName;
}
public double getAmount() {
return amount;
}
public void setAmount( double amount) {
this .amount = amount;
}
}
|
Validation file
Bundled validators are being used here to carry out the validation.
XML
<? xml version = "1.5" encoding = "UTF-8" ?>
<!DOCTYPE validators PUBLIC
"-//geeksforgeeks//XWork Validator 1.0.2//EN"
< validators >
< field name = "price" >
< field-validator type = "double" >
< param name = "minInclusive" >200.0</ param >
< param name = "maxExclusive" >9999.9</ param >
< message >Price must be between ${minInclusive} to ${maxExclusive}</ message >
</ field-validator >
</ field >
</ validators >
|
View component
The user’s information is shown in a basic JavaScript file.
XML
<%@ taglib uri="/struts-tags" prefix="s"%>
< html >
< head >
< title >Struts 2 double validator</ title >
</ head >
< body >
< h3 >This is a double validator example.</ h3 >
Hello < s:property value = "UserName" />
</ body >
</ html >
|
Output:
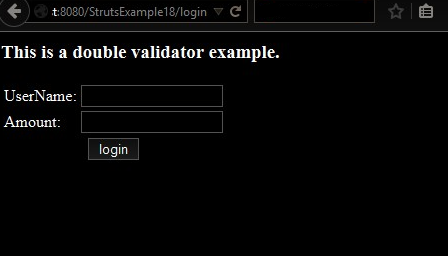
Conclusion
The Struts 2 Framework’s Double Validator determines whether or not the input is double. The error message is generated if the input is not twice. The input range can also be verified using a double validator. This example shows how to check the input range using a double validator.
Share your thoughts in the comments
Please Login to comment...