Struts 2 Int Validation
Last Updated :
05 Feb, 2024
Struts 2 integer validator is used to determine whether an integer field is inside a certain range. There are two ways to use this validator, much as other Struts validators. The Field Validator verifies if the entered number falls into the defined range.
Example of Struts 2 int validation
XML
< validators >
< validator type = "int" >
< param name = "fieldName" >age</ param >
< param name = "min" >17</ param >
< param name = "max" >24</ param >
< message >Age needs to be between ${min} and ${max}</ message >
</ validator >
< field name = "age" >
< field-validator type = "int" >
< param name = "min" >20</ param >
< param name = "max" >50</ param >
< message >Age needs to be between ${min} and ${max}</ message >
</ field-validator >
</ field >
< field name = "age" >
< field-validator type = "int" >
< param name = "minExpression" >${minValue}</ param >
< param name = "maxExpression" >${maxValue}</ param >
< message >Age needs to be between ${min} and ${max}</ message >
</ field-validator >
</ field >
</ validators >
|
Struts 2 int validation Parameters
- parse: If set to true, the expressions min and max will be compared to determine min/max.
- minExpression: The expression used to determine the minimal value is called minExpression; if it is left blank, the value will not be examined.
- maxExpression: an expression that determines the maximum value; if it is left blank, the value will not be verified.
- fieldName: This validator is validating the name of the field. Necessary when use Plain-Validator Syntax; otherwise, not necessary.
Below are the steps to implement Struts 2 integer validation:
Step 1: Create index.jsp for input
This JavaScript website uses struts UI tags to generate a form. The user provides it with their email address, password, and name.
XML
<%@ taglib uri="/struts-tags" prefix="s"%>
< html >
< head >
< title >Struts 2 int validator</ title >
</ head >
< body >
< h3 >This is an int validator.</ h3 >
< s:form action = "Login" >
< s:textfield name = "userName" label = "UserName" />
< s:textfield name = "age" label = "Age" />
< s:submit value = "login" align = "center" />
</ s:form >
</ body >
</ html >
|
Step 2: Construct the action class
Overriding the execute function, this action class derives from the ActionSupport class.
Java
package org.geeksforgeeks;
import com.opensymphony.xpwork2.ActionSupport;
public class Register extends ActionSupport {
private int id;
private double price;
public int getId() { return id; }
public void setId( int id) { this .id = id; }
public double getPrice() { return price; }
public void setPrice( double price)
{
this .price = price;
}
public String execute() { return "success" ; }
}
|
Step 3: Create the validation file
By utilizing the bundled validators, the validation is carried out.
XML
<!DOCTYPE validators PUBLIC
"-//OpenSymphony Group//XWork Validator 1.0.2//EN"
< validators >
< field name = "userName" >
< field-validator type = "requiredstring" >
< param name = "trim" >true</ param >
< message >
Username is required.
</ message >
</ field-validator >
</ field >
< field name = "age" >
< field-validator type = "int" >
< param name = "min" >17</ param >
< param name = "max" >24</ param >
< message >
Age should be between ${min} and ${max}.
</ message >
</ field-validator >
</ field >
</ validators >
|
Step 4: Put struts.xml together
This XML file specifies an interceptor named jsonValidatorWorkflowStack and an additional result named input.
XML
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.0.1//EN"
< struts >
< package name = "user" extends = "struts-default" >
< action name = "Login"
class = "org.geeksforgeeks.action.Login" >
< result name = "success" >/welcome.jsp</ result >
< result name = "input" >/login.jsp</ result >
</ action >
</ package >
</ struts >
|
Step 5: Create a view component
It is a basic JavaScript file that displays the user’s information.
XML
<%@ taglib uri="/struts-tags" prefix="s"%>
< html >
< head >
< title >Struts 2 int validator</ title >
</ head >
< body >
< h3 >This is an int validator.</ h3 >
Hello < s:property value = "userName" />
</ body >
</ html >
|
Output: int Validator log in page:
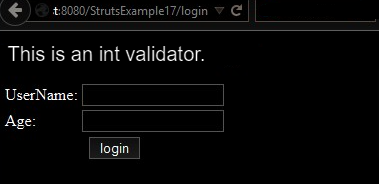
After entering values in fields:
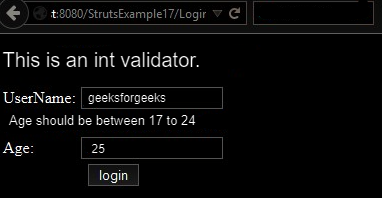
Conclusion
So, this is Struts 2 int validation. Struts 2 integer validator is used to determine whether an integer field is inside a particular range. minExpression used to decide the minimal value is called minExpression. If it is left blank, the value will now not be tested. maxExpression, an expression that determines the maximum value. If it is left blank, the value won’t be tested.
Share your thoughts in the comments
Please Login to comment...