String Manipulation in Shell Scripting
Last Updated :
24 May, 2021
String Manipulation is defined as performing several operations on a string resulting change in its contents. In Shell Scripting, this can be done in two ways: pure bash string manipulation, and string manipulation via external commands.
Basics of pure bash string manipulation:
1. Assigning content to a variable and printing its content: In bash, ‘$‘ followed by the variable name is used to print the content of the variable. Shell internally expands the variable with its value. This feature of the shell is also known as parameter expansion. Shell does not care about the type of variables and can store strings, integers, or real numbers.
Syntax:
VariableName='value'
echo $VariableName
or
VariableName="value"
echo ${VariableName}
or
VariableName=value
echo "$VariableName"
Note: There should not be any space around the “=” sign in the variable assignment. When you use VariableName=value, the shell treats the “=” as an assignment operator and assigns the value to the variable. When you use VariableName = value, the shell assumes that VariableName is the name of a command and tries to execute it.
Example:
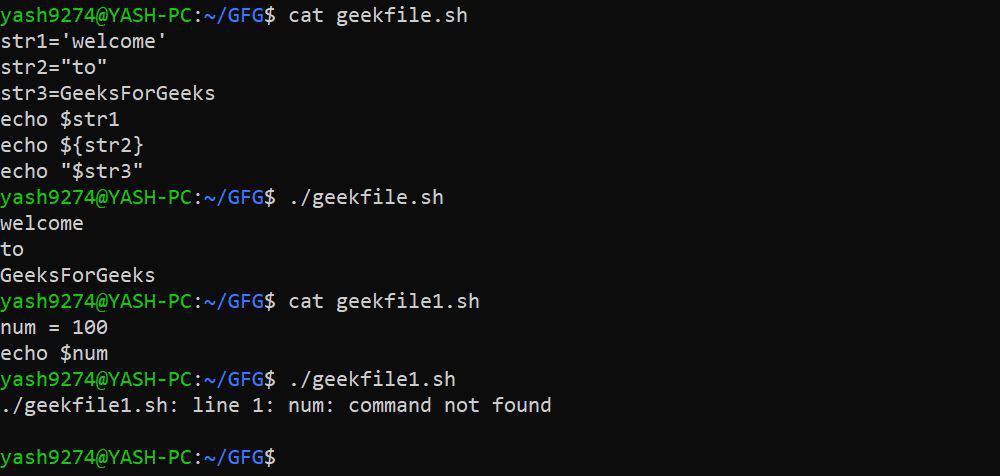
2. To print length of string inside Bash Shell: ‘#‘ symbol is used to print the length of a string.
Syntax:
variableName=value
echo ${#variablename}
Example:
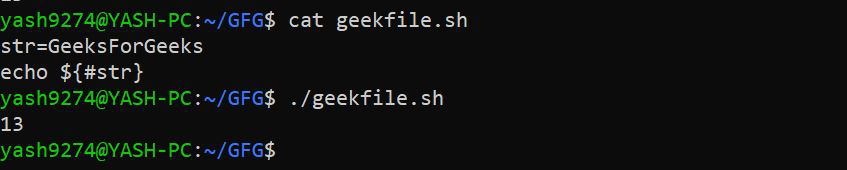
3. Concatenate strings inside Bash Shell using variables: In bash, listing the strings together concatenates the string. The resulting string so formed is a new string containing all the listed strings.
Syntax:
var=${var1}${var2}${var3}
or
var=$var1$var2$var3
or
var="$var1""$var2""$var3"
To concatenate any character between the strings:
The following will insert "**" between the strings
var=${var1}**${var2}**${var3}
or
var=$var1**$var2**$var3
or
var="$var1"**"$var2"**"$var3"
The following concatenate the strings using space:
var=${var1} ${var2} ${var3}
or
var="$var1" "$var2" "$var3"
or
echo ${var1} ${var2} ${var3}
Note: While concatenating strings via space, avoid using var=$var1 $var2 $var3. Here, the shell assumes $var2 and $var3 as commands and tries to execute them, resulting in an error.
Example:
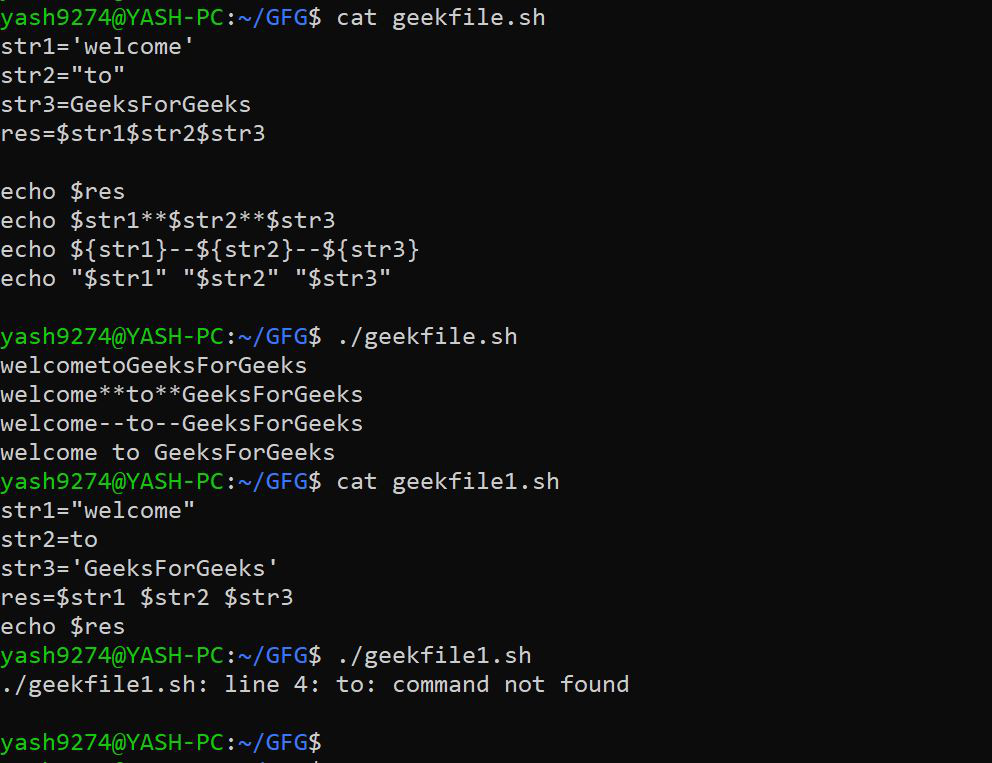
4. Concatenate strings inside Bash Shell using an array: In bash, arrays can also be used to concatenate strings.
Syntax:
To create an array:
arr=("value1" value2 $value3)
To print an array:
echo ${arr[@]}
To print length of an array:
echo ${#arr[@]}
Using indices (index starts from 0):
echo ${arr[index]}
Note: echo ${arr} is the same as echo ${arr[0]}
Example:
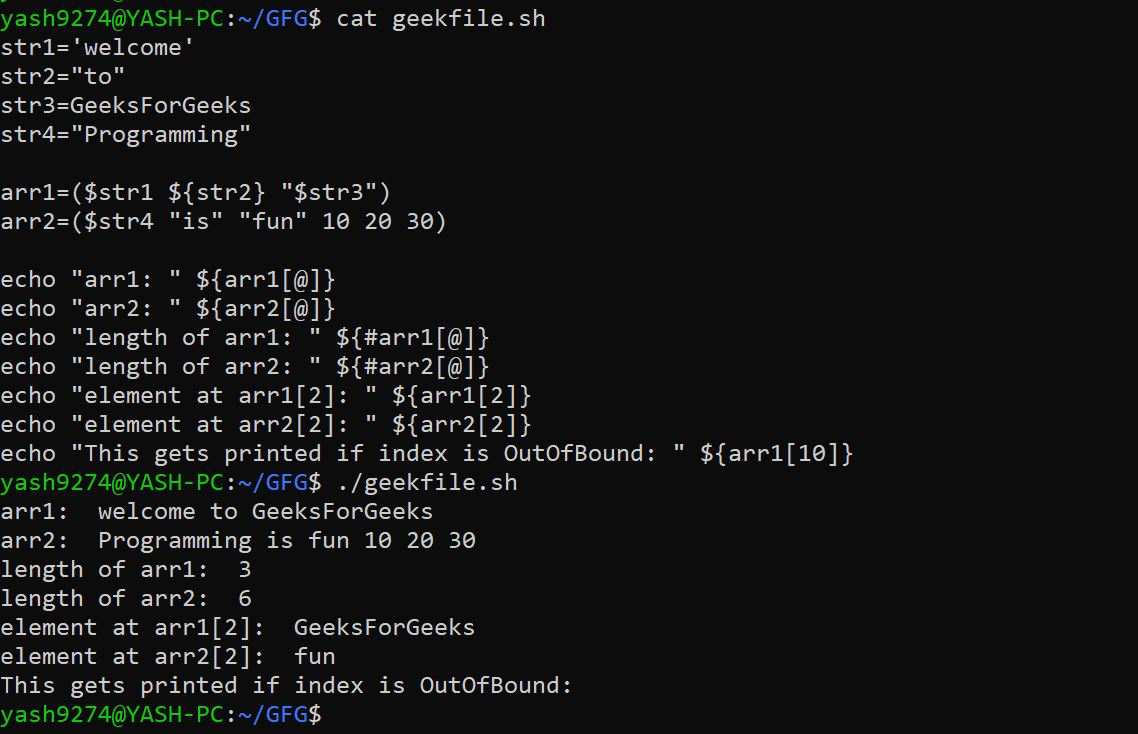
5. Extract a substring from a string: In Bash, a substring of characters can be extracted from a string.
Syntax:
${string:position} --> returns a substring starting from $position till end
${string:position:length} --> returns a substring of $length characters starting from $position.
Note: $length and $position must be always greater than or equal to zero.
If the $position is less than 0, it will print the complete string.
If the $length is less than 0, it will raise an error and will not execute.
Example:
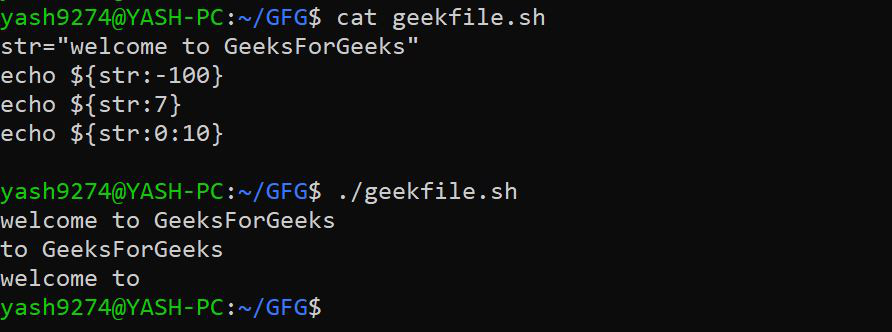
6. Substring matching: In Bash, the shortest and longest possible match of a substring can be found and deleted from either front or back.
Syntax:
To delete the shortest substring match from front of $string:
${string#substring}
To delete the shortest substring match from back of $string:
${string%substring}
To delete the longest substring match from front of $string:
${string##substring}
To delete the shortest substring match from back of $string of $string:
${string%%substring}
Example:
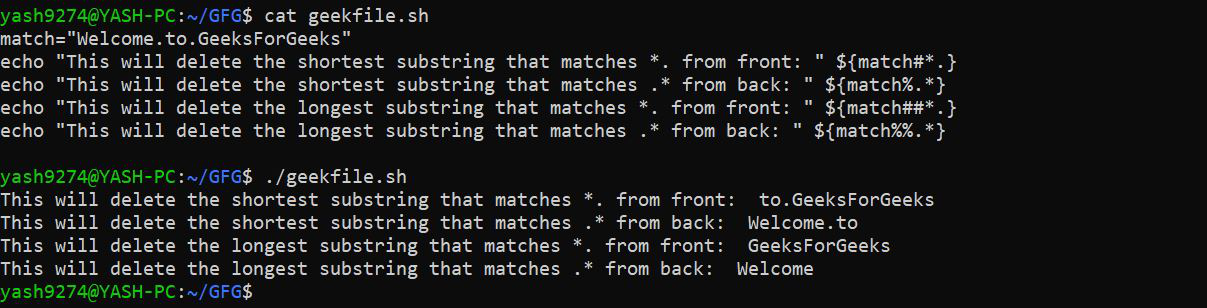
In the above example:
- The first echo statement substring ‘*.‘ matches the characters ending with a dot, and # deletes the shortest match of the substring from the front of the string, so it strips the substring ‘Welcome.‘.
- The second echo statement substring ‘.*‘ matches the substring starting with a dot and ending with characters, and % deletes the shortest match of the substring from the back of the string, so it strips the substring ‘.GeeksForGeeks‘
- The third echo statement substring ‘*.‘ matches the characters ending with a dot, and ## deletes the longest match of the substring from the front of the string, so it strips the substring ‘Welcome.to.‘
- The fourth echo statement substring ‘.*‘ matches the substring starting with a dot and ending with characters, and %% deletes the longest match of the substring from the back of the string, so it strips the substring ‘.to.GeeksForGeeks‘.
Share your thoughts in the comments
Please Login to comment...