Stimulate bouncing game using Pygame
Last Updated :
24 Jan, 2023
In this article, we will learn to make a bouncing game using Pygame. Pygame is a set of Python modules designed for writing video games. It adds functionality on top of the excellent SDL library. This allows you to create fully featured games and multimedia programs in the Python language. It is free and runs on nearly every platform and operating system. To install Pygame we will use pip.
Required Module
Open the terminal and type the following command:
pip install pygame
Steps to Create the Bouncing Game using Pygame
Step 1: Import Pygame and initialize it.
Python3
import pygame
pygame.init()
|
Step 2: Define the width and height of the window and create a game window.
Python3
width = 1000
height = 600
screen_res = (width, height)
pygame.display.set_caption( "GFG Bouncing game" )
screen = pygame.display.set_mode(screen_res)
|
Step 3: Define colors in RGB format. We will use these colors in our game.
Python3
red = ( 255 , 0 , 0 )
black = ( 0 , 0 , 0 )
|
Step 4: Define the ball and the speed by which it will move
This ball object will be used to move the ball in our game, and it will be used to get new coordinates of the ball and we will use these coordinates to draw the ball. Speed has 2 things, speed in the x direction and speed in the y direction. when speed in the x direction and speed in the y direction is equal in magnitude, then the ball will move in a diagonal way. Initially center of the ball is (100,100) and the speed is (1,1). Now after 1 unit of time, the center of the ball will be (101,101) and again after 1 unit of time, the center of the ball will be (102,102). In this way, our ball will move.
Python3
ball_obj = pygame.draw.circle(
surface = screen, color = red, center = [ 100 , 100 ], radius = 40 )
speed = [ 1 , 1 ]
|
Step 5: Define the game loop.
- Firstly we create an event loop, to get events from the queue.
- Then we check if a user wants to exit the game or not.
- Then we fill black color on the screen.
- Then we will move the ball with a specified speed, and update our ball(Rect) object.
- If our ball goes out of the screen in the horizontal direction, then we will change the direction of motion on the X-axis.
- And If our ball goes out of the screen in the vertical direction, then we will change the direction of motion on the Y-axis.
- Finally, we will draw our ball with the center as the center of the ball(Rect) object.
- In last we will update our screen.
Python3
while True :
for event in pygame.event.get():
if event. type = = pygame.QUIT:
exit()
screen.fill(black)
ball_obj = ball_obj.move(speed)
if ball_obj.left < = 0 or ball_obj.right > = width:
speed[ 0 ] = - speed[ 0 ]
if ball_obj.top < = 0 or ball_obj.bottom > = height:
speed[ 1 ] = - speed[ 1 ]
pygame.draw.circle(surface = screen, color = red,
center = ball_obj.center, radius = 40 )
pygame.display.flip()
|
Complete Code
Python3
import pygame
pygame.init()
width = 1000
height = 600
screen_res = (width, height)
pygame.display.set_caption( "GFG Bouncing game" )
screen = pygame.display.set_mode(screen_res)
red = ( 255 , 0 , 0 )
black = ( 0 , 0 , 0 )
ball_obj = pygame.draw.circle(
surface = screen, color = red, center = [ 100 , 100 ], radius = 40 )
speed = [ 1 , 1 ]
while True :
for event in pygame.event.get():
if event. type = = pygame.QUIT:
exit()
screen.fill(black)
ball_obj = ball_obj.move(speed)
if ball_obj.left < = 0 or ball_obj.right > = width:
speed[ 0 ] = - speed[ 0 ]
if ball_obj.top < = 0 or ball_obj.bottom > = height:
speed[ 1 ] = - speed[ 1 ]
pygame.draw.circle(surface = screen, color = red,
center = ball_obj.center, radius = 40 )
pygame.display.flip()
|
Output:
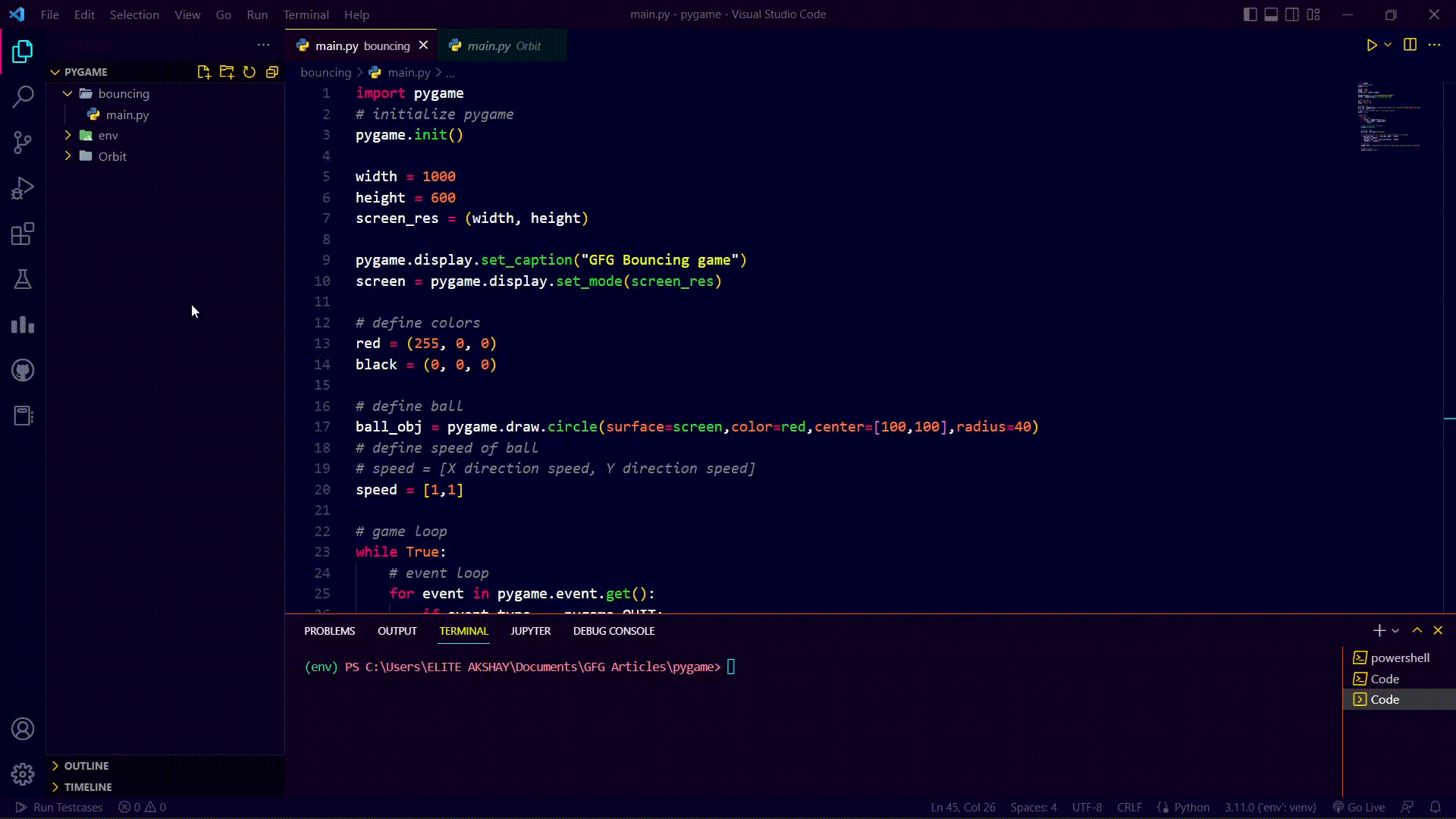
Game Output
Share your thoughts in the comments
Please Login to comment...