std::shared_mutex in C++
Last Updated :
25 Nov, 2023
In C++, std::mutex is a mechanism that locks access to the shared resource when some other thread is working on it so that errors such as race conditions can be avoided and threads can be synchronized. But in some cases, several threads need to read the data from shared resources at the same time. Here, the std::shared_mutex comes into play. In this article, we will discuss the std::shared_mutex, its associated methods, and how it is different from the std::mutex in C++.
Prerequisite: C++ Multithreading, std::mutex in C++.
std::shared_mutex in C++
In C++, std::shared_mutex is a synchronization primitive that lets several threads use a shared resource simultaneously for reading while guaranteeing exclusive writing access. It is helpful in situations where many threads need read-only access to the same data structure, but write operations aren’t often used.
Types of Locks in std::shared_mutex
The std::shared_mutex provides two types of locks:
- Unique Lock: It is a unique lock that can only be acquired by a single thread at a time. This lock only allows a single thread to modify the shared resources while blocking the other threads. It is similar to the lock provided by the std::mutex.
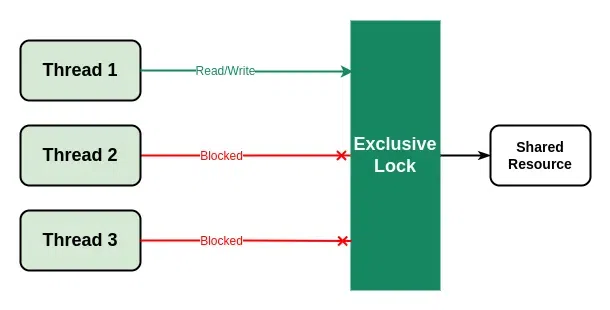
Exclusive Lock in C++
- Shared Lock: The shared lock is a non-exclusive lock that several threads can acquire at once. This gives multiple threads read-only access to the shared resource.
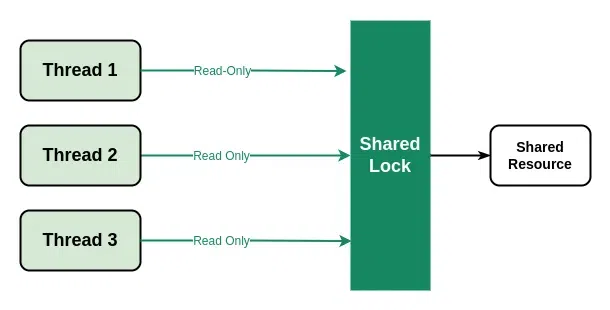
Shared Lock in C++
Note: Multiple threads can aquire the shared lock simultaneously but only one thread can aquire the exclusive lock at a time.
Methods Associated with std::shared_mutex
The std::shared_mutex contains several member methods that are required to perform different operations. Some of the commonly used functions are:
S. No.
|
Function
|
Description
|
1
|
lock() |
Exclusively locks the mutex and blocks if it fails. |
2
|
unlock() |
Unlock the exclusive lock. |
3
|
try_lock() |
Tries to uniquely lock the mutex. This function doesn’t block and returns false if the mutex is already locked for exclusive access. |
4
|
lock_shared() |
Gets the mutex to use a shared lock. |
5
|
unlock_shared() |
Unlocks the shared lock for the current thread. |
6
|
try_lock_shared() |
Tries for shared lock. This function doesn’t block and returns false if fails. |
Example of std::shared_lock
C++
#include <iostream>
#include <shared_mutex>
#include <mutex>
#include <thread>
using namespace std;
shared_mutex mutx;
int shared_data = 11;
void readData() {
shared_lock<shared_mutex> lock(mutx);
cout << "Thread " << this_thread::get_id() << ": " ;
cout << shared_data << endl;
}
void writeData( int n) {
unique_lock<shared_mutex> lock(mutx);
shared_data = n;
cout << "Thread" << this_thread::get_id() << ": \n" ;
}
int main()
{
thread t1(readData);
thread t2(writeData, 128);
thread t3(writeData, 10);
thread t4 (readData);
t1.join();
t2.join();
t3.join();
t4.join();
return 0;
}
|
Output
Read Thread 140122977658432: 11
Write Thread 140122960873024
Write Thread 140122969265728
Read Thread 140122952480320: 128
std::shared_mutex vs std::mutex
Similar to std::mutex, std::shared_mutex is a synchronization primitive that prevents concurrent access to shared resources. Both have their own advantages and disadvantages.
Advantages of std::shared_mutex over std::mutex
The std::shared_mutex has the following advantages over std::mutex:
- Enhanced Parallelism: Multiple threads can access the shared data for reading operation at once enhancing the parallelism of the program where only read operation is required.
Advantages of std::shared_mutex over std::mutex
- Limited Flexibility: There are only two types of locks that the std::shared_mutex supports: shared locks and unique locks. Additional lock types, such as recursive and postponed locks, are supported via the std::mutex.
- Increased Complexity: Compared to std::mutex, std::shared_mutex has greater complexity. It may become more challenging to understand and apply.
Applications
Following are some main applications of std::shared_mutex:
- When reading data is the primary operation and writing data is uncommon, shared mutexes offer effective parallelism.
- Shared mutexes can be used in caching algorithms to enable simultaneous updating of the cache by one thread while allowing several threads to read data that has been cached.
- Database connection pooling allows for simultaneous reading and updating of connection information by several threads, but not for simultaneous modification.
Conclusion
std::shared_mutex is one of the useful mechanisms for access synchronization in multithreaded environments. It works especially effectively in scenarios where the shared data is mostly read-only. But before utilizing the std::shared_mutex, it’s crucial to be aware of its limitations.
Share your thoughts in the comments
Please Login to comment...