Mutex in C++
Last Updated :
20 Nov, 2023
Mutex stands for Mutual Exclusion. In C++, std::mutex class is a synchronization primitive that is used to protect the shared data from being accessed by multiple threads simultaneously. The shared data can be in the form of variables, data structures, etc.
std::mutex class implements mutex in C++. It is defined inside <mutex> header file.
Need for Mutex in C++
In C++, when multiple threads modify the same shared resources simultaneously may cause race conditions. It may produce unpredictable output or unexpected behavior while executing the program. Mutex is used to avoid race conditions by locking the current thread so that all the other threads cannot access the shared resources at that time and unlocking it when the current thread is done.
Syntax for Mutex in C++
The use of mutex can be divided into three steps:
1. Create a std::mutex Object
std::mutex mutex_object_name;
2. Lock the Thread
The lock() function of the std::mutex class locks the thread and allows only the current thread to run until it is unlocked. It prevents the shared resource from being accessed by multiple threads simultaneously.
mutex_object_name.lock()
3. Unlock the thread
The unlock() of the std::mutex function is used to release the lock after execution of the code piece containing the possibility of race condition occurrence. It resumes all the waiting threads.
mutex_object_name.unlock()
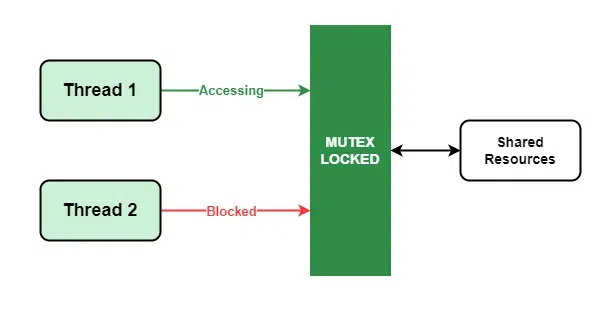
Working of Mutex in C++
Example of Mutex in C++
Let’s create a shared integer variable, which can be accessed globally inside the program. Create a function to increment the number by 1 for 1000000 times using a for loop. Create two threads named thread1 and thread2 to run the same increment() function.
In this case, thread1 will increment the number by 1 for 1000000 times and thread2 will increment the number by 1 for 1000000 times. So the expected output is 2000000.
However, there is a possibility of occurrences of race conditions when multiple threads try to modify the same resource simultaneously. So the value of the number cannot be predicted.
Code Without Mutex Synchronization
C++
#include <iostream>
#include <thread>
using namespace std;
int number = 0;
void increment(){
for ( int i=0; i<1000000; i++){
number++;
}
}
int main()
{
thread t1(increment);
thread t2(increment);
t1.join();
t2.join();
cout << "Number after execution of t1 and t2 is " << number;
return 0;
}
|
Output
The same program is executed three times to observe the behavior when modifying shared resources without thread synchronization.
Run 1:
Number after execution of t1 and t2 is 1058072

Without Mutex Output 1
Run 2:
Number after execution of t1 and t2 is 1456656

Without Mutex Output 2
Run 3:
Number after execution of t1 and t2 is 2000000

Without Mutex Output 3
Explanation
It is clearly visible that the output of the program is unpredictable. When two threads are running at the same time causes race cases that create unpredictable output. There is no guarantee that the output to be 2000000. This unpredictable behavior is happening because of the concurrent modification of the same shared variable simultaneously using multiple threads.
Code with Mutex Synchronization
C++
#include <iostream>
#include <thread>
using namespace std;
#include <mutex>
mutex mtx;
int number = 0;
void increment(){
mtx.lock();
for ( int i=0; i<1000000; i++){
number++;
}
mtx.unlock();
}
int main()
{
thread t1(increment);
thread t2(increment);
t1.join();
t2.join();
std::cout<< "Number after execution of t1 and t2 is " <<number;
return 0;
}
|
Output
The same program is executed three times to observe the behavior when modifying shared resource with thread synchronization using mutex.
Run 1:
Number after execution of t1 and t2 is 2000000

Mutex Output 1
Run 2:
Number after execution of t1 and t2 is 2000000

Mutex Output 2
Run 3:
Number after execution of t1 and t2 is 2000000
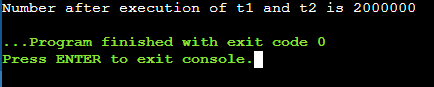
Mutex Output 3
Explanation
Here, the output is stable. The threads are synchronous. Mutex object pauses all other thread than current thread using lock(). Now, only it allows one thread (current thread) at a time until it is unlocked using unlock() function.
Share your thoughts in the comments
Please Login to comment...